o3-mini has confirmed to be OpenAI’s most superior mannequin for coding and reasoning. The o3-mini (excessive) mannequin has single-handedly outperformed different current fashions like DeepSeek-R1 and Claude 3.5 in most traditional benchmark exams. Owing to this, ChatGPT powered by o3-mini has now turn out to be an on a regular basis companion for builders. It provides them an clever and environment friendly solution to sort out programming challenges—be it debugging, code technology, documentation, or knowledge cleansing. This text lists 10 ChatGPT prompts that may provide help to unlock the total potential of o3-mini on your coding duties. So, let’s get began!
1. Debugging Code Errors
Suppose you might be engaged on a Python script for an online app, and instantly, you encounter an error that you simply don’t perceive. The traceback message is lengthy and complicated, and you might be not sure how you can repair it. o3-mini provides a fast solution to debug the difficulty and perceive what went flawed.
Template Immediate: “I’ve a bit of code in [language] that’s throwing an error: [error message]. Are you able to assist me debug it? [insert code]”
Pattern Immediate:
“I’ve a bit of Python code that’s throwing an error: AttributeError: ‘NoneType’ object has no attribute ‘group’. Are you able to assist me debug it?”
import pandas as pd
# Pattern knowledge
knowledge = {
"Product": ["Laptop", "Headphones", "Smartphone", "Monitor", "Mouse"],
"Class": ["Electronics", "Electronics", "Electronics", "Accessories", "Accessories"],
"Gross sales": ["$1000", "$200", "$800", "$300", "$50"] # Gross sales values comprise a '$' signal
}
df = pd.DataFrame(knowledge)
# Convert Gross sales column to drift
df["Sales"] = df["Sales"].astype(float) # 🚨 This line throws a ValueError
# Calculate complete gross sales per class
total_sales = df.groupby("Class")["Sales"].sum()
print(total_sales)
Output of Code:
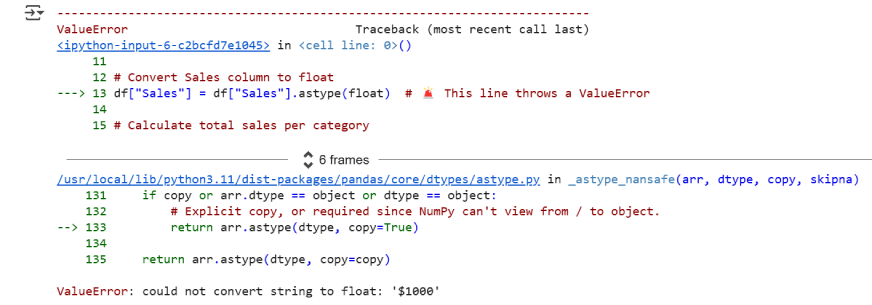
o3-mini’s Response:
import pandas as pd
# Pattern knowledge
knowledge = {
"Product": ["Laptop", "Headphones", "Smartphone", "Monitor", "Mouse"],
"Class": ["Electronics", "Electronics", "Electronics", "Accessories", "Accessories"],
"Gross sales": ["$1000", "$200", "$800", "$300", "$50"] # Gross sales values comprise a '$' signal
}
df = pd.DataFrame(knowledge)
# Convert Gross sales column to drift after stripping '$'
df["Sales"] = df["Sales"].str.exchange("$", "").astype(float) # ✅ Mounted: Eliminated foreign money symbols
# Calculate complete gross sales per class
total_sales = df.groupby("Class")["Sales"].sum()
print(total_sales)
Output of Code:

2. Making a Code Template
When you could have a brand new mission at hand, it could possibly usually be complicated on the place to start out. There could also be totally different approaches, or a number of steps concerned, or it might require libraries you aren’t aware of. In such instances, o3-mini can assist you get began with a fundamental code template and information you thru writing your entire code.
Template Immediate: “Are you able to present a structured template and information for writing a [language] mission that does [task]?”
Pattern Immediate:
“Are you able to present a structured template and information for organising a Python Flask mission that connects to a PostgreSQL database?”
o3-mini’s Response:
3. Changing Code Between Languages
Let’s say you could have a bit of code written in a single programming language, however your mission requires it in one other. As an alternative of rewriting it manually, o3-mini can translate the code for you, preserving the logic and performance.
Template Immediate: “Can you exchange this [source language] code to [target language] whereas sustaining logic and performance?”
Pattern Immediate:
“Can you exchange this Python code to JavaScript (Node.js) whereas sustaining its logic and performance?”
from collections import Counter
# Pattern textual content enter
textual content = """
It is a pattern textual content. This textual content is supposed to check phrase frequency.
Some phrases seem greater than as soon as, some phrases seem solely as soon as.
"""
# Convert to lowercase and break up into phrases
phrases = textual content.decrease().break up()
# Depend phrase occurrences
word_counts = Counter(phrases)
# Print outcomes
for phrase, rely in word_counts.gadgets():
print(f"{phrase}: {rely}")
Output of Code:
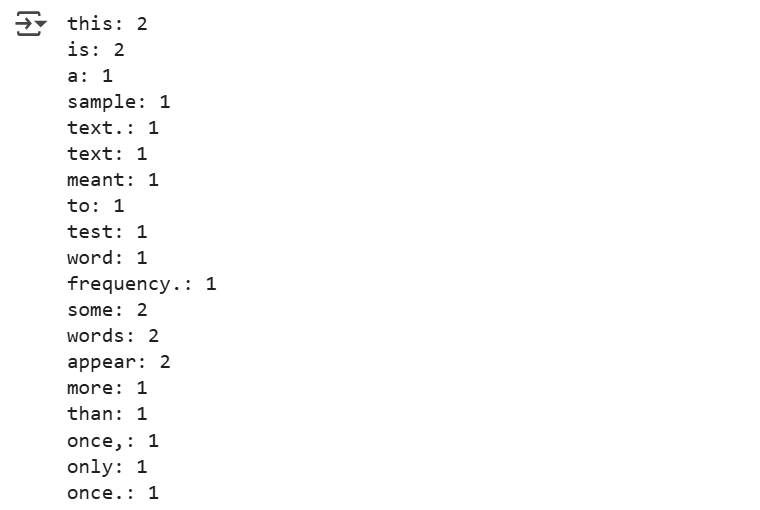
o3-mini’s Response:
// Pattern textual content enter
let textual content = `
It is a pattern textual content. This textual content is supposed to check phrase frequency.
Some phrases seem greater than as soon as, some phrases seem solely as soon as.
`;
// Convert to lowercase and break up into phrases
let phrases = textual content.toLowerCase().exchange(/[^ws]/g, "").break up(/s+/);
// Depend phrase occurrences
let wordCounts = new Map();
phrases.forEach(phrase => 0) + 1);
);
// Print outcomes
wordCounts.forEach((rely, phrase) => {
console.log(`${phrase}: ${rely}`);
});
Output of Code:
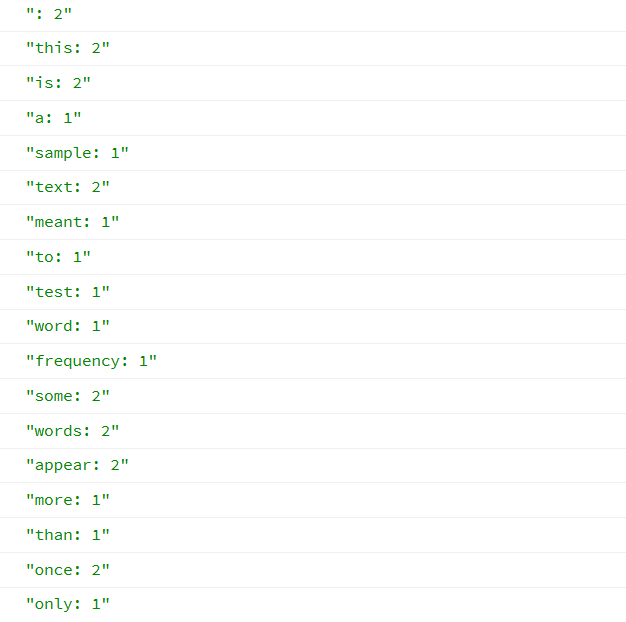
4. Enhancing Code Readability
Whereas writing code for work, it is crucial that the code be readable and simple for all stakeholders to grasp. For this, you’ll have to put in writing documentation, add code feedback, or refactor the code for higher readability. In such instances, o3-mini might be of nice assist.
Template Immediate: “Are you able to generate docstrings and feedback for this [language] operate?[insert code]”
OR “Are you able to refactor this [language] code to make it extra readable?[insert code]”
Pattern Immediate:
“Are you able to add docstrings and feedback to this Python operate that processes consumer enter and updates a database?”
import sqlite3
def update_user_balance(user_id, quantity):
conn = sqlite3.join("database.db")
cursor = conn.cursor()
current_balance = cursor.execute("SELECT steadiness FROM customers WHERE id = ?", (user_id,)).fetchone()
if current_balance:
new_balance = current_balance[0] + quantity
cursor.execute("UPDATE customers SET steadiness = ? WHERE id = ?", (new_balance, user_id))
conn.commit()
conn.shut()
return new_balance
else:
conn.shut()
return None
o3-mini’s Response:
import sqlite3
def update_user_balance(user_id, quantity):
"""
Updates the steadiness of a consumer within the database.
Parameters:
user_id (int): The distinctive ID of the consumer whose steadiness must be up to date.
quantity (float): The quantity so as to add to (or subtract from) the consumer's steadiness.
Returns:
float: The up to date steadiness if the consumer exists.
None: If the consumer doesn't exist within the database.
"""
# Connect with the SQLite database
conn = sqlite3.join("database.db")
cursor = conn.cursor()
# Fetch the present steadiness of the consumer
current_balance = cursor.execute(
"SELECT steadiness FROM customers WHERE id = ?", (user_id,)
).fetchone()
if current_balance:
# Calculate the brand new steadiness
new_balance = current_balance[0] + quantity
# Replace the consumer's steadiness within the database
cursor.execute(
"UPDATE customers SET steadiness = ? WHERE id = ?", (new_balance, user_id)
)
# Commit the transaction
conn.commit()
# Shut the connection
conn.shut()
return new_balance # Return the up to date steadiness
else:
# Shut the connection if consumer doesn't exist
conn.shut()
return None # Return None if consumer ID shouldn't be discovered
5. Writing Code for Edge Circumstances and Error Dealing with
You’re making an API request in Python, however your code doesn’t deal with instances the place the request fails. You have to add error dealing with to make it extra strong.
Template Immediate: “Are you able to add correct error dealing with to this [language] operate? [insert function]”
Pattern Immediate:
“Are you able to add correct error dealing with to this Python operate making an API request?”
import requests
response = requests.get("https://api.instance.com/knowledge")
knowledge = response.json()
o3-mini’s Response:
import requests
def fetch_api_data(url):
"""
Fetches knowledge from the given API URL with correct error dealing with.
Parameters:
url (str): The API endpoint to request knowledge from.
Returns:
dict or None: The JSON response as a dictionary if profitable, or None if an error happens.
"""
attempt:
response = requests.get(url, timeout=10) # Set a timeout to keep away from hanging requests
response.raise_for_status() # Elevate an error for HTTP errors (4xx and 5xx)
return response.json() # Parse and return JSON response
besides requests.exceptions.Timeout:
print("Error: The request timed out.")
besides requests.exceptions.ConnectionError:
print("Error: Failed to hook up with the server.")
besides requests.exceptions.HTTPError as err:
print(f"HTTP Error: {err}")
besides requests.exceptions.RequestException as err:
print(f"Request Error: {err}")
besides ValueError:
print("Error: Unable to decode JSON response.")
return None # Return None if any error happens
# Instance utilization
api_url = "https://api.instance.com/knowledge"
knowledge = fetch_api_data(api_url)
if knowledge:
print("API Knowledge Retrieved Efficiently!")
else:
print("Did not retrieve API knowledge.")
6. Writing CI/CD Pipeline Configurations
Let’s suppose your workforce is deploying a Node.js utility, and also you’ve been assigned the duty of organising an automatic CI/CD pipeline. You have to configure GitHub Actions to run exams and deploy the app each time adjustments are pushed to the primary department. Right here’s a easy immediate you should utilize on o3-mini to arrange the deployment pipeline.
Template Immediate: “Are you able to generate a CI/CD pipeline configuration for a [technology stack: React, MongoDB, Node.js, etc.] mission utilizing [CI/CD tool: GitHub Actions, Jenkins, etc.] that runs exams and deploys to [deployment environment: AWS, AKS, GKE, etc.]?”
Pattern Immediate:
“Are you able to generate a CI/CD pipeline configuration for a Node.js mission utilizing GitHub Actions that runs exams and deploys to AWS S3 on each push to the primary department?”
o3-mini’s Response:
title: Node.js CI/CD Pipeline
on:
push:
branches:
- fundamental # Runs the workflow on pushes to the primary department
jobs:
build-and-test:
title: Construct and Check
runs-on: ubuntu-latest
steps:
- title: Checkout repository
makes use of: actions/checkout@v3
- title: Arrange Node.js
makes use of: actions/setup-node@v3
with:
node-version: 18
- title: Set up dependencies
run: npm set up
- title: Run exams
run: npm take a look at
- title: Construct mission
run: npm run construct
- title: Add construct artifacts
makes use of: actions/upload-artifact@v3
with:
title: build-output
path: dist/
- title: Deploy to S3
env:
AWS_ACCESS_KEY_ID: ${{ secrets and techniques.AWS_ACCESS_KEY_ID }}
AWS_SECRET_ACCESS_KEY: ${{ secrets and techniques.AWS_SECRET_ACCESS_KEY }}
AWS_REGION: "us-east-1" # Change to your AWS area
S3_BUCKET: "your-s3-bucket-name"
run: |
aws s3 sync dist/ s3://$S3_BUCKET --delete
7. Code Optimization and Efficiency Enchancment
Have you ever ever been in conditions the place your Python operate works effective, however runs slowly on giant datasets? If this has been a roadblock in getting your job accomplished, right here’s how o3-mini can assist you optimize your code for extra environment friendly execution.
Template Immediate: “Are you able to optimize this code for efficiency? It at the moment has points with [mention inefficiencies] and runs slowly for [mention scenario]. [insert code]”
Pattern Immediate:
“Are you able to optimize this Python code for efficiency? It at the moment has inefficiencies in checking for prime numbers and runs slowly when looking for primes in a wide range (e.g., 1 to 100,000).”
def is_prime(n):
if n < 2:
return False
for i in vary(2, n):
if n % i == 0:
return False
return True
def find_primes(begin, finish):
primes = []
for num in vary(begin, finish + 1):
if is_prime(num):
primes.append(num)
return primes
# Instance utilization
start_range = 1
end_range = 10000
primes = find_primes(start_range, end_range)
print(f"Discovered {len(primes)} prime numbers.")
o3-mini’s Response:
import math
def is_prime(n):
"""Verify if a quantity is prime."""
if n < 2:
return False
if n == 2:
return True # 2 is the one even prime quantity
if n % 2 == 0:
return False # Remove even numbers > 2
# Verify divisibility as much as √n
for i in vary(3, int(math.sqrt(n)) + 1, 2):
if n % i == 0:
return False
return True
def find_primes(begin, finish):
"""Discover all prime numbers in a given vary."""
primes = []
for num in vary(begin, finish + 1):
if is_prime(num):
primes.append(num)
return primes
# Instance utilization
start_range = 1
end_range = 10000
primes = find_primes(start_range, end_range)
print(f"Discovered {len(primes)} prime numbers.")
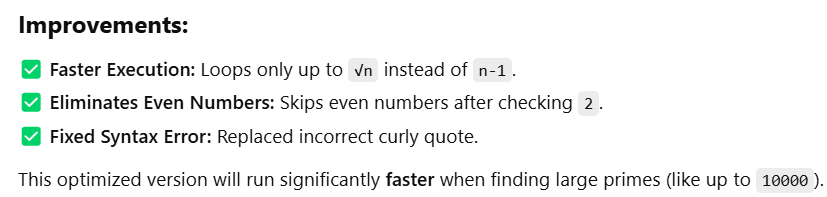
Output of Code:

8. Producing Common Expressions (Regex)
o3-mini might be very useful in producing common expressions in code. Suppose you’re constructing a kind validation system for a web site and wish a regex sample to validate electronic mail addresses. Simply ask o3-mini to generate a regex sample utilizing this immediate.
Template Immediate: “Are you able to generate a regex sample in [language] for [task]?”
Pattern Immediate:
“Are you able to generate a regex sample in Python for validating electronic mail addresses?”
o3-mini’s Response:
import re
# Common expression for electronic mail validation
email_pattern = r"^[a-zA-Z0-9_.+-]+@[a-zA-Z0-9-]+.[a-zA-Z0-9-.]+$"
# Perform to validate emails
def is_valid_email(electronic mail):
return re.match(email_pattern, electronic mail) shouldn't be None
Output of Code:
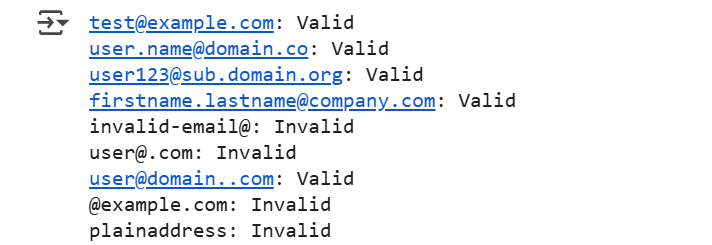
9. Helping with Knowledge Cleansing and Processing
Whereas analyzing buyer gross sales knowledge in Pandas, you usually come throughout messy datasets, lacking values, duplicate entries, and knowledge in a number of codecs. Utilizing the next immediate on o3-mini is an environment friendly solution to clear the info earlier than operating your evaluation.
Template Immediate: “Are you able to assist clear and course of a dataset with points resembling [list data issues] utilizing [programming language and library]? [insert dataset]”
Pattern Immediate:
“Are you able to assist clear and course of a dataset with lacking values, duplicate rows, incorrect date codecs, and inconsistent capitalization utilizing Python and Pandas? clients.csv”
o3-mini’s Response:
import pandas as pd
# Load the dataset
df = pd.read_csv("clients.csv")
# Standardize column names (take away areas and convert to lowercase)
df.columns = df.columns.str.strip().str.decrease().str.exchange(" ", "_")
# Deal with lacking values
df["age"].fillna(df["age"].median(), inplace=True) # Fill lacking ages with median
df["purchase_amount"].fillna(df["purchase_amount"].imply(), inplace=True) # Fill lacking purchases with imply
# Take away duplicate rows
df = df.drop_duplicates()
# Standardize textual content knowledge (capitalize names and cities)
df["name"] = df["name"].str.title()
df["city"] = df["city"].str.title()
df["country"] = df["country"].str.higher()
# Convert dates to a constant format
df["join_date"] = pd.to_datetime(df["join_date"], errors="coerce", dayfirst=False)
# Save the cleaned dataset
df.to_csv("cleaned_customers.csv", index=False)
print("Knowledge cleansing accomplished efficiently!")
Output of Code:
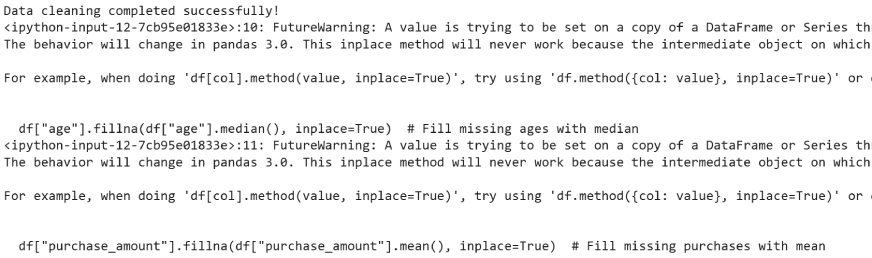
10. Writing Shell Scripts for Automation
I’m positive you’ll agree with me after I say plenty of our work is repetitive in nature – be it ceaselessly deploying functions or backing up recordsdata. As an alternative of operating these instructions manually each time, o3-mini can assist you automate them by coding. It may well write you shell scripts to automate repetitive terminal instructions based mostly in your necessities.
Template Immediate: “Are you able to write a shell script to automate [task] utilizing [specific tools or commands]?”
Pattern Immediate:
“Are you able to write a shell script to mechanically again up a listing (/residence/consumer/paperwork) to a different location (/mnt/backup) on daily basis at midnight utilizing cron?”
o3-mini’s Response:
1️. Shell Script (backup.sh)
<pre class="wp-block-code"><code>#!/bin/bash
# Outline supply and vacation spot directories
SOURCE="/residence/consumer/paperwork"
DESTINATION="/mnt/backup"
TIMESTAMP=$(date +"%Y-%m-%d_percentH-%M-%S")
BACKUP_DIR="$DESTINATION/backup_$TIMESTAMP"
# Create backup listing
mkdir -p "$BACKUP_DIR"
#Copy recordsdata whereas preserving attributes
rsync -av --delete "$SOURCE/" "$BACKUP_DIR/"
# Log the backup
2️. Make the Script Executable
Run the next command to present the script execution permissions:
chmod +x /path/to/backup.sh
3️. Schedule Day by day Backup at Midnight with Cron
Open the crontab editor:
crontab -e
Add this line on the finish:
0 0 * * * /path/to/backup.sh
This schedules the script to run every day at midnight (00:00).
Conclusion
As we have now on this article, o3-mini is able to dealing with all types of coding duties from debugging advanced errors and optimizing code for higher efficiency, to writing CI/CD configurations, and changing code between languages. In a approach, o3-mini has redefined how builders method coding challenges by offering clever, environment friendly, and correct options. So go forward, check out these prompts, and let o3-mini provide help to work smarter, not more durable!
Ceaselessly Requested Questions
A. o3-mini is OpenAI’s most superior mannequin for coding and reasoning. It outperforms fashions like DeepSeek-R1 and Claude 3.5 in benchmark exams, making it a dependable selection for builders.
A. Sure, o3-mini can analyze error messages, determine the foundation trigger, and counsel fixes for varied programming languages. The above coding prompts can assist you leverage o3-mini for these duties.
A. Completely! o3-mini can help with Python, JavaScript, Java, C++, Rust, Go, and lots of extra languages.
A. Sure, you possibly can ask o3-mini to generate structured templates for initiatives, together with frameworks like Flask, Django, React, and Node.js.
A. o3-mini gives extremely correct code translations whereas sustaining logic and performance, making it simpler to adapt code for various tech stacks.
A. Sure, it could possibly analyze your code and counsel optimizations to enhance velocity, reminiscence utilization, and effectivity. The o3-mini immediate template given within the above article can assist you with such coding duties.
A. It may well generate docstrings, add significant feedback, and refactor messy code to make it extra readable and maintainable.
A. Sure, it could possibly generate CI/CD pipeline scripts for instruments like GitHub Actions, Jenkins, and GitLab CI/CD. You should use the o3-mini immediate template given within the above article on ChatGPT for this.