Retrievers play an important position within the LangChain framework by offering a versatile interface that returns paperwork based mostly on unstructured queries. Not like vector shops, retrievers aren’t required to retailer paperwork; their major perform is to retrieve related info. Whereas vector shops can function the spine of a retriever, varied forms of retrievers exist, every tailor-made to particular use instances.
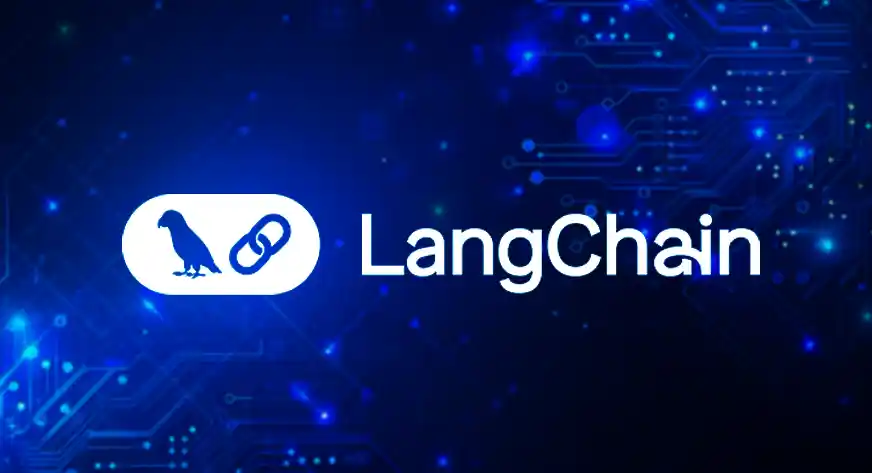
Studying Goal
- Discover the pivotal position of retrievers in LangChain, enabling environment friendly and versatile doc retrieval for various functions.
- Learn the way LangChain’s retrievers, from vector shops to MultiQuery and Contextual Compression, streamline entry to related info.
- This information covers varied retriever varieties in LangChain and illustrates how every is tailor-made to optimize question dealing with and knowledge entry.
- Dive into LangChain’s retriever performance, analyzing instruments for enhancing doc retrieval precision and relevance.
- Perceive how LangChain’s customized retrievers adapt to particular wants, empowering builders to create extremely responsive functions.
- Uncover LangChain’s retrieval strategies that combine language fashions and vector databases for extra correct and environment friendly search outcomes.
Retrievers in LangChain
Retrievers settle for a string question as enter and output an inventory of Doc objects. This mechanism permits functions to fetch pertinent info effectively, enabling superior interactions with massive datasets or information bases.
1. Utilizing a Vectorstore as a Retriever
A vector retailer retriever effectively retrieves paperwork by leveraging vector representations. It serves as a light-weight wrapper across the vector retailer class, conforming to the retriever interface and using strategies like similarity search and Most Marginal Relevance (MMR).
To create a retriever from a vector retailer, use the .as_retriever methodology. For instance, with a Pinecone vector retailer based mostly on buyer opinions, we are able to set it up as follows:
from langchain_community.document_loaders import CSVLoader
from langchain_community.vectorstores import Pinecone
from langchain_openai import OpenAIEmbeddings
from langchain_text_splitters import CharacterTextSplitter
loader = CSVLoader("customer_reviews.csv")
paperwork = loader.load()
text_splitter = CharacterTextSplitter(chunk_size=500, chunk_overlap=50)
texts = text_splitter.split_documents(paperwork)
embeddings = OpenAIEmbeddings()
vectorstore = Pinecone.from_documents(texts, embeddings)
retriever = vectorstore.as_retriever()
We will now use this retriever to question related opinions:
docs = retriever.invoke("What do prospects take into consideration the battery life?")
By default, the retriever makes use of similarity search, however we are able to specify MMR because the search kind:
retriever = vectorstore.as_retriever(search_type="mmr")
Moreover, we are able to go parameters like a similarity rating threshold or restrict the variety of outcomes with top-k:
retriever = vectorstore.as_retriever(search_kwargs={"ok": 2, "score_threshold": 0.6})
Output:

Utilizing a vector retailer as a retriever enhances doc retrieval by guaranteeing environment friendly entry to related info.
2. Utilizing the MultiQueryRetriever
The MultiQueryRetriever enhances distance-based vector database retrieval by addressing frequent limitations, akin to variations in question wording and suboptimal embeddings. Automating immediate tuning with a massive language mannequin (LLM) generates a number of queries from completely different views for a given consumer enter. This course of permits for retrieving related paperwork for every question and mixing the outcomes to yield a richer set of potential paperwork.
Constructing a Pattern Vector Database
To exhibit the MultiQueryRetriever, let’s create a vector retailer utilizing product descriptions from a CSV file:
from langchain_community.document_loaders import CSVLoader
from langchain_community.vectorstores import FAISS
from langchain_openai import OpenAIEmbeddings
from langchain_text_splitters import CharacterTextSplitter
# Load product descriptions
loader = CSVLoader("product_descriptions.csv")
knowledge = loader.load()
# Cut up the textual content into chunks
text_splitter = CharacterTextSplitter(chunk_size=300, chunk_overlap=50)
paperwork = text_splitter.split_documents(knowledge)
# Create the vector retailer
embeddings = OpenAIEmbeddings()
vectordb = FAISS.from_documents(paperwork, embeddings)
Easy Utilization
To make the most of the MultiQueryRetriever, specify the LLM for question technology:
from langchain.retrievers.multi_query import MultiQueryRetriever
from langchain_openai import ChatOpenAI
query = "What options do prospects worth in smartphones?"
llm = ChatOpenAI(temperature=0)
retriever_from_llm = MultiQueryRetriever.from_llm(
retriever=vectordb.as_retriever(), llm=llm
)
unique_docs = retriever_from_llm.invoke(query)
len(unique_docs) # Variety of distinctive paperwork retrieved
Output:

The MultiQueryRetriever generates a number of queries, enhancing the range and relevance of the retrieved paperwork.
Customizing Your Immediate
To tailor the generated queries, you’ll be able to create a customized PromptTemplate and an output parser:
from langchain_core.output_parsers import BaseOutputParser
from langchain_core.prompts import PromptTemplate
from typing import Checklist
# Customized output parser
class LineListOutputParser(BaseOutputParser[List[str]]):
def parse(self, textual content: str) -> Checklist[str]:
return record(filter(None, textual content.strip().cut up("n")))
output_parser = LineListOutputParser()
# Customized immediate for question technology
QUERY_PROMPT = PromptTemplate(
input_variables=["question"],
template="""Generate 5 completely different variations of the query: {query}"""
)
llm_chain = QUERY_PROMPT | llm | output_parser
# Initialize the retriever
retriever = MultiQueryRetriever(
retriever=vectordb.as_retriever(), llm_chain=llm_chain, parser_key="strains"
)
unique_docs = retriever.invoke("What options do prospects worth in smartphones?")
len(unique_docs) # Variety of distinctive paperwork retrieved
Output

Utilizing the MultiQueryRetriever permits for simpler retrieval processes, guaranteeing various and complete outcomes based mostly on consumer queries
3. Learn how to Carry out Retrieval with Contextual Compression
Retrieving related info from massive doc collections will be difficult, particularly when the precise queries customers will pose are unknown on the time of knowledge ingestion. Typically, invaluable insights are buried in prolonged paperwork, resulting in inefficient and expensive calls to language fashions (LLMs) whereas offering less-than-ideal responses. Contextual compression addresses this challenge by refining the retrieval course of, guaranteeing that solely pertinent info is returned based mostly on the consumer’s question.
Overview of Contextual Compression
The Contextual Compression Retriever operates by integrating a base retriever with a Doc Compressor. As a substitute of returning paperwork of their entirety, this method compresses them based on the context supplied by the question. This compression includes each decreasing the content material of particular person paperwork and filtering out irrelevant ones.
Implementation Steps
1. Initialize the Base Retriever: Start by organising a vanilla vector retailer retriever. For instance, think about a information article on local weather change coverage:
from langchain_community.document_loaders import TextLoader
from langchain_community.vectorstores import FAISS
from langchain_openai import OpenAIEmbeddings
from langchain_text_splitters import CharacterTextSplitter
# Load and cut up the article
paperwork = TextLoader("climate_change_policy.txt").load()
text_splitter = CharacterTextSplitter(chunk_size=1000, chunk_overlap=0)
texts = text_splitter.split_documents(paperwork)
# Initialize the vector retailer retriever
retriever = FAISS.from_documents(texts, OpenAIEmbeddings()).as_retriever()
2. Carry out an Preliminary Question: Execute a question to see the outcomes returned by the bottom retriever, which can embrace related in addition to irrelevant info.
docs = retriever.invoke("What actions are being proposed to fight local weather change?")
3. Improve Retrieval with Contextual Compression: Wrap the bottom retriever with a ContextualCompressionRetriever, using an LLMChainExtractor to extract related content material:
from langchain.retrievers import ContextualCompressionRetriever
from langchain.retrievers.document_compressors import LLMChainExtractor
from langchain_openai import OpenAI
llm = OpenAI(temperature=0)
compressor = LLMChainExtractor.from_llm(llm)
compression_retriever = ContextualCompressionRetriever(
base_compressor=compressor, base_retriever=retriever
)
# Carry out the compressed retrieval
compressed_docs = compression_retriever.invoke("What actions are being proposed to fight local weather change?")
Evaluate the Compressed Outcomes: The ContextualCompressionRetriever processes the preliminary paperwork and extracts solely the related info associated to the question, optimizing the response.
Making a Customized Retriever
A retriever is crucial in lots of LLM functions. It’s tasked with fetching related paperwork based mostly on consumer queries. These paperwork are formatted into prompts for the LLM, enabling it to generate applicable responses.
Interface
To create a customized retriever, lengthen the BaseRetriever class and implement the next strategies:
Methodology | Description | Required/Non-compulsory |
_get_relevant_documents | Retrieve paperwork related to a question. | Required |
_aget_relevant_documents | Asynchronous implementation for native help. | Non-compulsory |
Inheriting from BaseRetriever grants your retriever the usual Runnable performance.
Instance
Right here’s an instance of a easy retriever:
from typing import Checklist
from langchain_core.paperwork import Doc
from langchain_core.retrievers import BaseRetriever
class ToyRetriever(BaseRetriever):
"""A easy retriever that returns prime ok paperwork containing the consumer question."""
paperwork: Checklist[Document]
ok: int
def _get_relevant_documents(self, question: str) -> Checklist[Document]:
matching_documents = [doc for doc in self.documents if query.lower() in doc.page_content.lower()]
return matching_documents[:self.k]
# Instance utilization
paperwork = [
Document("Dogs are great companions.", {"type": "dog"}),
Document("Cats are independent pets.", {"type": "cat"}),
]
retriever = ToyRetriever(paperwork=paperwork, ok=1)
consequence = retriever.invoke("canine")
print(consequence[0].page_content)
Output

This implementation gives a simple method to retrieve paperwork based mostly on consumer enter, illustrating the core performance of a customized retriever in LangChain.
Conclusion
Within the LangChain framework, retrievers are highly effective instruments that allow environment friendly entry to related info throughout varied doc varieties and use instances. By understanding and implementing completely different retriever varieties—akin to vector retailer retrievers, the MultiQueryRetriever, and the Contextual Compression Retriever—builders can tailor doc retrieval to their utility’s particular wants.
Every retriever kind affords distinctive benefits, from dealing with advanced queries with MultiQueryRetriever to optimizing responses with Contextual Compression. Moreover, creating customized retrievers permits for even larger flexibility, accommodating specialised necessities that in-built choices might not meet. Mastering these retrieval strategies empowers builders to construct simpler and responsive functions, harnessing the complete potential of language fashions and enormous datasets.
In case you’re trying to grasp LangChain and different Generative AI ideas, don’t miss out on our GenAI Pinnacle Program.
Regularly Requested Questions
Ans. A retriever’s major position is to fetch related paperwork in response to a question. This helps functions effectively entry essential info from massive datasets with no need to retailer the paperwork themselves.
Ans. A vector retailer is used for storing paperwork in a method that enables similarity-based retrieval, whereas a retriever is an interface designed to retrieve paperwork based mostly on queries. Though vector shops will be a part of a retriever, the retriever’s job is concentrated on fetching related info.
Ans. The MultiQueryRetriever improves search outcomes by creating a number of variations of a question utilizing a language mannequin. This methodology captures a broader vary of paperwork that is perhaps related to in another way phrased questions, enhancing the range of retrieved info.
Ans. Contextual compression refines retrieval outcomes by decreasing doc content material to solely the related sections and filtering out unrelated info. That is particularly helpful in massive collections the place full paperwork would possibly include extraneous particulars, saving sources and offering extra centered responses.
Ans. To arrange a MultiQueryRetriever, you want a vector retailer for doc storage, a language mannequin (LLM) to generate a number of question views, and, optionally, a customized immediate template to refine question technology additional.