Introduction
Think about you’re looking for a particular piece of data from a large library the place some books produce other smaller books inside them. To search out the appropriate reply, you might have to first take a look at the smaller books, then use that data to search out the bigger one. That is precisely how nested queries in SQL work! By putting one question inside one other, you may extract advanced knowledge with ease. On this information, we’ll discover how nested queries perform and how one can harness their energy in SQL for extra environment friendly database administration.
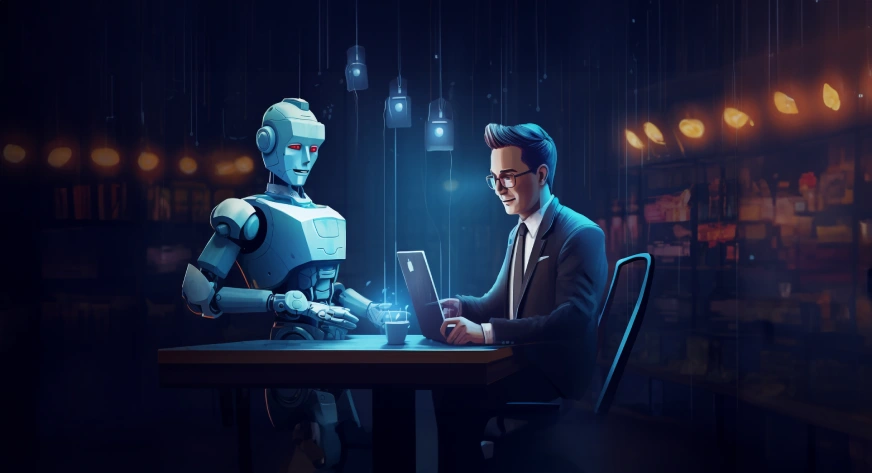
Studying End result
- Perceive what nested queries (subqueries) are in SQL.
- Write and implement nested queries inside numerous SQL statements.
- Differentiate between correlated and non-correlated nested queries.
- Optimize SQL queries utilizing nested constructions for improved efficiency.
What Are Nested Queries in SQL?
A nested question, also referred to as a subquery, is an SQL question positioned inside one other SQL question. The results of the internal question (the subquery) is utilized by the outer question to attain the specified end result. This method is especially helpful when the outcomes from the internal question depend upon the info retrieved by the outer question.
Primary Syntax
SELECT column_name(s)
FROM table_name
WHERE column_name = (SELECT column_name FROM table_name WHERE situation);
Forms of Nested Queries in SQL
Nested queries, also referred to as subqueries, help you carry out advanced knowledge retrieval by embedding one SQL question inside one other. This performance is important for writing environment friendly SQL code and dealing with intricate database operations. On this part, we’ll discover the various kinds of nested queries, full with examples and anticipated outputs.
Single-row Subquery in SQL
A single-row subquery is a nested kind of question that leads to a number of columns in only a single row. It is extremely widespread with SQL statements the place you wish to use a comparability operator or a situation in opposition to a single worth, comparable to =, <, >, and so forth.
Key Traits of Single-row Subqueries
- Returns One Row: Owing to the title assigned, one can count on a single row of knowledge.
- Often Used with Comparability Operators: Often used with operators comparable to =, >, <, >=, <= and so forth.
- Can Return One or Extra Columns: Although it returns a single row, but it might return a number of columns.
Instance: Discover Workers Incomes Extra Than the Common Wage
Desk: staff
employee_id | first_name | last_name | wage | department_id |
---|---|---|---|---|
1 | John | Doe | 90000 | 1 |
2 | Jane | Smith | 95000 | 1 |
3 | Alice | Johnson | 60000 | 2 |
4 | Bob | Brown | 65000 | 2 |
5 | Charlie | Davis | 40000 | 3 |
6 | Eve | Adams | 75000 | 3 |
Desk: departments
department_id | department_name | location_id |
---|---|---|
1 | Gross sales | 1700 |
2 | Advertising | 1700 |
3 | IT | 1800 |
4 | HR | 1900 |
SELECT first_name, last_name, wage
FROM staff
WHERE wage > (SELECT AVG(wage) FROM staff);
Output:
| first_name | last_name | wage |
|------------|-----------|--------|
| John | Doe | 90000 |
| Jane | Smith | 95000 |
For example, within the instance, the internal question (SELECT AVG(wage) FROM staff) finds what all the staff’ common salaries are. The outer question will get the primary title, final title and wage of all staff whose earnings are above this quantity.
Multi-row Subquery in SQL
Multi-row subquery is a type of nested question that returns therefore, multiple row of knowledge. It Is normally used with IN, ANY, or ALL operators to check a column with a set of values returned by the subquery. One of many benefits of utilizing multi-row subquery is that it combines the outcomes on an inventory of values and applies a number of rows in making computations.
Instance: Discover Workers in Sure Departments
SELECT first_name, last_name
FROM staff
WHERE department_id IN (SELECT department_id FROM departments WHERE location_id = 1700);
Output:
| first_name | last_name |
|------------|-----------|
| Alice | Johnson |
| Bob | Brown |
Right here, the internal question retrieves department_id
s from the departments
desk the place the location_id
is 1700. The outer question then finds staff who work in these departments.
Correlated Subquery in SQL
A correlated subquery is a kind of nested question in SQL. It depends upon the outer question for its values. Whereas an everyday subquery can execute independently, a correlated subquery calculates in relation to each row processed by the outer question, therefore dynamic and context-sensitive.
Traits of Correlated Subqueries
- Dependency: The internal question references columns from the outer question, establishing a direct dependency.
- Row-by-row Execution: The internal question runs a number of occasions—as soon as for every row processed by the outer question.
- Efficiency Issues: As a result of the internal question runs repeatedly, correlated subqueries could be slower than their non-correlated counterparts, particularly on massive datasets.
Instance: Discover Workers with Salaries Above Their Division’s Common
SELECT first_name, wage
FROM staff e1
WHERE wage > (SELECT AVG(wage) FROM staff e2 WHERE e1.department_id = e2.department_id);
Output:
| first_name | wage |
|------------|--------|
| John | 90000 |
| Jane | 95000 |
On this case, the internal question calculates the common wage for every division because the outer question processes every worker. The outer question selects staff who earn greater than their division’s common wage.
Nested Subqueries in SQL
A nested subquery is also referred to as a nested question. That is an internal question or a question positioned inside one other question the place one question seems inside one other. Such queries turn into fairly helpful for accessing troublesome knowledge and remodeling it in reasonably very particular methods, permitting advanced issues to interrupt into extra constituent, manageable elements, making it a lot simpler to question relational databases.
Construction of Nested Subqueries
A nested subquery sometimes consists of two principal elements:
- Outer Question: That is the principle question that incorporates the subquery. It makes use of the results of the subquery to filter or manipulate knowledge.
- Interior Question (Subquery): This question is embedded inside the outer question and gives a consequence set that may be utilized by the outer question.
Instance: Discover Departments with Workers Incomes Extra Than the Common Wage
SELECT department_id, department_name
FROM departments
WHERE department_id IN (
SELECT department_id
FROM staff
WHERE wage > (SELECT AVG(wage) FROM staff)
);
Output:
| department_id | department_name |
|---------------|------------------|
| 1 | Gross sales |
| 2 | Advertising |
On this instance, the innermost question (SELECT AVG(wage) FROM staff) is taking the common. The center question will fetch department_ids of staff making above that common and the outer question will retrieve the division names assigned to it.
Scalar Subquery
A scalar subquery is outlined as a subquery which provides out a single worth, a single row and a single column. So scalar subqueries are fairly helpful to make use of wherever there’s a requirement of a single worth in the principle question. Scalar subqueries could be utilized inside many SQL clauses like SELECT, WHERE and HAVING.
Traits of Scalar Subqueries
- Returns One Worth: As its title suggests, scalar subquery solely returns a single worth. Any subquery which tries to return a row apart from a single one or a column apart from one will result in an error.
- Utilized in Varied Clauses: Derived columns could be calculated within the SELECT statements, narrowing down the leads to WHERE clauses, and including situations on a set of knowledge inside a HAVING clause all with the assistance of those scalar subqueries inside this clause.
- Environment friendly for Comparisons: They’re typically used for making comparisons in opposition to a single worth derived from one other question.
Instance: Retrieve Workers and Their Wage Distinction from the Common Wage
SELECT first_name, last_name, wage - (SELECT AVG(wage) FROM staff) AS salary_difference
FROM staff;
Output:
| first_name | last_name | salary_difference |
|------------|-----------|-------------------|
| John | Doe | 10000 |
| Jane | Smith | 15000 |
On this case, the scalar subquery computes the common wage as soon as, and the outer question calculates the distinction for every worker’s wage from the common.
Use Instances for Nested Queries
Nested queries, or subqueries, are highly effective instruments in SQL that may clear up quite a lot of advanced knowledge retrieval challenges. Listed below are some widespread use instances:
Information Filtering
Nested queries can be utilized to filter outcomes primarily based on values derived from one other desk.
Instance: Discover staff whose salaries are above the common wage of their respective departments.
SELECT first_name, last_name, wage
FROM staff e1
WHERE wage > (SELECT AVG(wage) FROM staff e2 WHERE e1.department_id = e2.department_id);
Calculating Aggregates
You possibly can calculate aggregates in a nested question and use these leads to the outer question.
Instance: Retrieve departments with a median wage larger than the general common wage.
SELECT department_id, AVG(wage) AS average_salary
FROM staff
GROUP BY department_id
HAVING AVG(wage) > (SELECT AVG(wage) FROM staff);
Conditional Logic
Nested queries help you implement conditional logic inside your SQL statements.
Instance: Listing staff who belong to departments positioned in a particular metropolis.
SELECT first_name, last_name
FROM staff
WHERE department_id IN (SELECT department_id FROM departments WHERE metropolis = 'New York');
Correlated Subqueries for Row-Degree Calculations
Correlated subqueries allow row-level calculations primarily based on values from the present row within the outer question.
Instance: Get an inventory of merchandise with a value greater than the common value of merchandise in the identical class.
SELECT product_name, value
FROM merchandise p1
WHERE value > (SELECT AVG(value) FROM merchandise p2 WHERE p1.category_id = p2.category_id);
Variations Between Nested Queries and Different SQL Queries
Allow us to now look into the distinction between nested queries and different SQL queries beneath:
Characteristic | Nested Queries | Joins | Easy Queries |
---|---|---|---|
Definition | A question positioned inside one other question | Combines rows from two or extra tables primarily based on a associated column | A single SQL assertion that retrieves knowledge |
Execution | Executes the internal question for every row processed by the outer question | Executes concurrently for all rows from each tables | Executes independently with none dependencies |
Use Case | Helpful for advanced calculations and filtering primarily based on one other question | Preferrred for combining associated knowledge from a number of tables | Appropriate for simple knowledge retrieval |
Efficiency | Could result in slower efficiency resulting from repeated execution of the internal question | Typically extra environment friendly because it processes knowledge in a single go | Quickest for easy knowledge retrieval |
Complexity | Can turn into advanced and troublesome to learn | May also be advanced however sometimes clearer with express relationships | Easy and straightforward to know |
Information Dependency | The internal question can depend upon the outer question’s consequence | Information from joined tables is impartial of one another | Information retrieved is impartial, no subqueries concerned |
Instance | SELECT first_name FROM staff WHERE wage > (SELECT AVG(wage) FROM staff); |
SELECT e.first_name, d.department_name FROM staff e JOIN departments d ON e.department_id = d.department_id; |
SELECT * FROM staff; |
Widespread Errors with Nested Queries
Whereas nested queries could be extremely helpful, additionally they include pitfalls. Listed below are some widespread errors to be careful for:
Returning A number of Rows
A scalar subquery should return a single worth; if it returns a number of rows, it would trigger an error.
Mistake:
SELECT first_name
FROM staff
WHERE wage = (SELECT wage FROM staff);
Answer: Make sure the internal question makes use of aggregation or filtering to return a single worth.
Efficiency Points
Nested queries can generally result in efficiency bottlenecks, particularly if they’re executed for every row within the outer question.
Mistake: Utilizing a nested question inside a big outer question with out contemplating efficiency implications.
Answer: Analyze question execution plans and take into account various strategies, like joins, when coping with massive datasets.
Improper Use of Parentheses
Incorrect placement of parentheses can result in surprising outcomes or errors.
Mistake:
SELECT first_name
FROM staff
WHERE wage > (SELECT AVG(wage) FROM staff WHERE department_id);
Answer: Make sure the logic of your question is evident, and parentheses are used appropriately to group situations.
Not Contemplating NULL Values
Nested queries can produce surprising outcomes when NULL values are current within the knowledge.
SELECT first_name
FROM staff
WHERE wage > (SELECT AVG(wage) FROM staff WHERE department_id IS NOT NULL);
Answer: Deal with NULL values explicitly utilizing capabilities like COALESCE
to keep away from unintended filtering.
Conclusion
SQL nested queries, also referred to as subqueries, are very helpful in finishing up extremely advanced knowledge retrieval operations effectively. You possibly can embed a question inside one other, to do any calculations on knowledge that can not be carried out by easy queries alone. Having the information of 4 principal forms of these will probably be useful: single-row, multi-row, correlated, and scalar subqueries. Making use of finest practices and avoiding some widespread pitfalls, you may faucet into the complete potential of nested queries to enhance your database administration and efficiency.
Steadily Requested Questions
A. A nested question, or subquery, is an SQL question positioned inside one other question. The internal question’s result’s utilized by the outer question to carry out advanced knowledge retrieval.
A. The primary sorts embrace single-row subqueries, multi-row subqueries, correlated subqueries, and scalar subqueries, every serving totally different use instances.
A. Use a correlated subquery when the internal question must reference a column from the outer question, permitting for dynamic row-by-row evaluations.
A. Sure, nested queries can result in efficiency points, particularly if they’re executed for each row within the outer question. Analyzing execution plans and contemplating options like joins can assist enhance effectivity.