Introduction
On the planet of databases, NULL values can typically really feel just like the proverbial black sheep. They characterize lacking, undefined, or unknown knowledge, and might pose distinctive challenges in knowledge administration and evaluation. Think about you’re analyzing a gross sales database, and a few entries lack buyer suggestions or order portions. Understanding successfully deal with NULL values in SQL is essential for making certain correct knowledge retrieval and significant evaluation. On this information, we’ll delve into the nuances of NULL values, discover how they have an effect on SQL operations, and supply sensible methods for managing them.
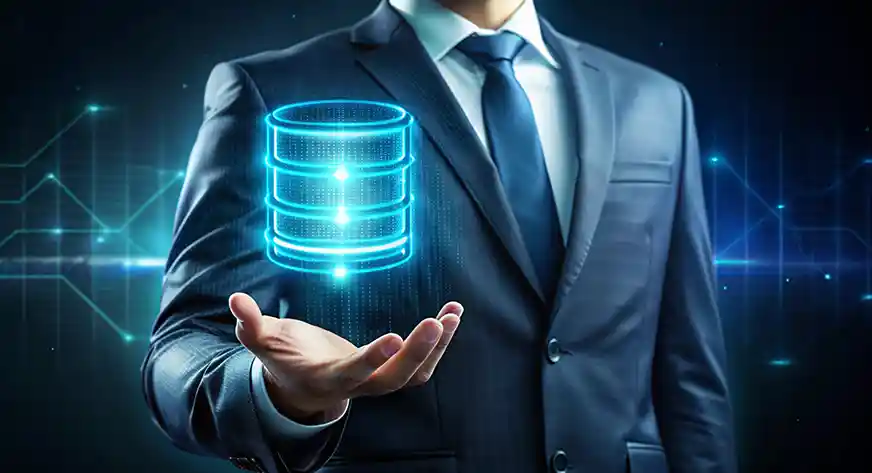
Studying Outcomes
- Perceive what NULL values characterize in SQL.
- Establish the impression of NULL values on knowledge queries and calculations.
- Make the most of SQL capabilities and methods to deal with NULL values successfully.
- Implement finest practices for managing NULLs in database design and querying.
What Are NULL Values in SQL?
NULL is a particular marker in SQL that’s used to level to the truth that worth for some issue isn’t recognized. It must also be understood that NULL isn’t equal to ‘’, 0 and different such values, whereas as a substitute it factors in direction of the absence of worth. In SQL, NULL can be utilized in any sort of an attribute, whether or not integer, string, or date.
Instance of NULL Values
Think about a desk named workers
:
On this desk, the department_id
for John and Bob is NULL, indicating that their division is unknown. Alice’s electronic mail can be NULL, that means there isn’t any electronic mail recorded.
Impression of NULL Values on SQL Queries
SQL NULL has outlined any columns that don’t comprise knowledge and its use influences how queries carry out and what outcomes are delivered. One of many issues that everybody must know to be able to write good queries and have the ability to work with knowledge appropriately is the conduct of NULL values. On this weblog, I’ll clarify some approaches, relying on whether or not fields comprise the NULL worth and the angle wherein the fields are thought of, for SQL queries for comparability, calculation, logical operations, and so forth.
Comparisons with NULL
When performing comparisons in SQL, it’s important to know that NULL values don’t equate to zero or an empty string. As an alternative, NULL represents an unknown worth. In consequence, any direct comparability involving NULL will yield an UNKNOWN end result, slightly than TRUE or FALSE.
Instance:
SELECT * FROM workers WHERE department_id = NULL;
Output: No rows can be returned as a result of comparisons to NULL utilizing =
don’t consider to TRUE.
To appropriately examine for NULL values, use:
SELECT * FROM workers WHERE department_id IS NULL;
Assuming the workers
desk has:
employee_id | first_name | department_id |
---|---|---|
1 | John | 101 |
2 | Jane | NULL |
3 | Bob | 102 |
4 | Alice | NULL |
Output:
employee_id | first_name | department_id |
---|---|---|
2 | Jane | NULL |
4 | Alice | NULL |
Boolean Logic and NULLs
NULL values have an effect on boolean logic in SQL queries. When NULL is concerned in logical operations, the end result can typically result in sudden outcomes. In SQL, the three-valued logic (TRUE, FALSE, UNKNOWN) signifies that if any operand in a logical expression is NULL, the whole expression may consider to UNKNOWN.
Instance:
SELECT * FROM workers WHERE first_name="John" AND department_id = NULL;
Output: This question will return no outcomes, because the situation involving NULL
will consider to UNKNOWN.
For proper logical operations, explicitly examine for NULL:
SELECT * FROM workers WHERE first_name="John" AND department_id IS NULL;
Output:
employee_id | first_name | department_id |
---|---|---|
No output |
Aggregation Features
NULL values have a novel impression on mixture capabilities reminiscent of SUM
, AVG
, COUNT
, and others. Most mixture capabilities ignore NULL values, which implies they won’t contribute to the results of calculations. This conduct can result in deceptive conclusions in case you are not conscious of the NULLs current in your dataset.
Instance:
SELECT AVG(wage) FROM workers;
Assuming the workers
desk has:
employee_id | wage |
---|---|
1 | 50000 |
2 | NULL |
3 | 60000 |
4 | NULL |
Output:
The common is calculated from the non-NULL salaries (50000 and 60000).
If all values in a column are NULL:
SELECT COUNT(wage) FROM workers;
Output:
On this case, COUNT solely counts non-NULL values.
DISTINCT and NULL Values
When utilizing the DISTINCT
key phrase, NULL values are handled as a single distinctive worth. Thus, in case you have a number of rows with NULLs in a column, the DISTINCT
question will return just one occasion of NULL.
Instance:
SELECT DISTINCT department_id FROM workers;
Assuming the workers
desk has:
employee_id | department_id |
---|---|
1 | 101 |
2 | NULL |
3 | 102 |
4 | NULL |
Output:
Even when there are a number of NULLs, just one NULL seems within the end result.
Strategies for Dealing with NULL Values
Dealing with NULL values is essential for sustaining knowledge integrity and making certain correct question outcomes. Listed here are some efficient methods:
Utilizing IS NULL and IS NOT NULL
Probably the most easy option to filter out NULL values is through the use of the IS NULL
and IS NOT NULL
predicates. This lets you explicitly examine for NULL values in your queries.
Instance:
SELECT * FROM workers WHERE department_id IS NULL;
Output:
employee_id | first_name | department_id |
---|---|---|
2 | Jane | NULL |
4 | Alice | NULL |
To seek out workers with a division assigned:
SELECT * FROM workers WHERE department_id IS NOT NULL;
Output:
employee_id | first_name | department_id |
---|---|---|
1 | John | 101 |
3 | Bob | 102 |
Utilizing COALESCE Perform
The COALESCE
perform returns the primary non-NULL worth within the listing of arguments. That is helpful for offering default values when NULL is encountered.
Instance:
SELECT first_name, COALESCE(department_id, 'No Division') AS division FROM workers;
Output:
first_name | division |
---|---|
John | 101 |
Jane | No Division |
Bob | 102 |
Alice | No Division |
Utilizing NULLIF Perform
The NULLIF
perform returns NULL if the 2 arguments are equal; in any other case, it returns the primary argument. This may help keep away from undesirable comparisons and deal with defaults elegantly.
Instance:
SELECT first_name, NULLIF(department_id, 0) AS department_id FROM workers;
Assuming department_id
is usually set to 0 as a substitute of NULL:
Output:
first_name | department_id |
---|---|
John | 101 |
Jane | NULL |
Bob | 102 |
Alice | NULL |
Utilizing the CASE Assertion
The CASE
assertion permits for conditional logic in SQL queries. You should use it to interchange NULL values with significant substitutes based mostly on particular circumstances.
Instance:
SELECT first_name,
CASE
WHEN department_id IS NULL THEN 'Unknown Division'
ELSE department_id
END AS division
FROM workers;
Output:
first_name | division |
---|---|
John | 101 |
Jane | Unknown Division |
Bob | 102 |
Alice | Unknown Division |
Utilizing Mixture Features with NULL Dealing with
When utilizing mixture capabilities like COUNT
, SUM
, AVG
, and so on., it’s important to keep in mind that they ignore NULL values. You possibly can mix these capabilities with COALESCE
or related methods to handle NULLs in mixture outcomes.
Instance:
To rely what number of workers have a division assigned:
SELECT COUNT(department_id) AS AssignedDepartments FROM workers;
Output:
If you wish to embrace a rely of NULL values:
SELECT COUNT(*) AS TotalEmployees,
COUNT(department_id) AS AssignedDepartments,
COUNT(*) - COUNT(department_id) AS UnassignedDepartments
FROM workers;
Output:
TotalEmployees | AssignedDepartments | UnassignedDepartments |
---|---|---|
4 | 2 | 2 |
Finest Practices for Managing NULL Values
We’ll now look into the most effective practices for managing NULL Worth.
- Use NULL Purposefully: Solely use NULL to point the absence of a worth. This distinction is essential; NULL shouldn’t be confused with zero or an empty string, as every has its personal that means in knowledge context.
- Set up Database Constraints: Implement NOT NULL constraints wherever relevant to stop unintentional NULL entries in vital fields. This helps implement knowledge integrity and ensures that important data is all the time current.
- Normalize Your Database Schema: Correctly design your database schema to attenuate the prevalence of NULL values. By organizing knowledge into acceptable tables and relationships, you possibly can cut back the necessity for NULLs and promote clearer knowledge illustration.
- Make the most of Smart Default Values: When designing tables, think about using wise default values to fill in for potential NULL entries. This method helps keep away from confusion and ensures that customers perceive the info’s context with out encountering NULL.
- Doc NULL Dealing with Methods: Clearly doc your method to dealing with NULL values inside your group. This consists of establishing tips for knowledge entry, reporting, and evaluation to advertise consistency and understanding amongst group members.
- Repeatedly Evaluation and Audit Knowledge: Conduct periodic critiques and audits of your knowledge to establish and handle NULL values successfully. This follow helps keep knowledge high quality and integrity over time.
- Educate Crew Members: Acknowledge and clarify NULL values to the workers in order that they perceive their significance and correct dealing with. Informing the group with the right information is essential for making the correct selections concerning knowledge and reporting.
Frequent Errors to Keep away from with NULLs
Allow us to now discover the widespread errors that we will keep away from with NULLs.
- Complicated NULL with Zero or Empty Strings: The primary and most steadily encountered anti-patterns are NULL used as the identical as zero or an empty string. Recognising that NULL is used to indicate the absence of worth is essential to be able to keep away from misinterpretations of knowledge.
- Utilizing the Equality Operator for NULL Comparisons: Don’t use equality operators (=) when testing NULL values, this can end result to an UNKNOWN situation. In stead of this, you need to use predicates IS NULL or IS NOT NULL for comparability.
- Neglecting NULLs in Mixture Features: Among the widespread points embrace the truth that most customers appear to disregard the truth that mixture capabilities like SUM, AVG and COUNT will all the time omit NULL values ensuing to unsuitable indicators. Use care of mixture knowledge and NULLs exist even in information containing solely entire numbers.
- Not Contemplating NULLs in Enterprise Logic: Failing to account for NULL values in enterprise logic can result in sudden outcomes in functions and experiences. All the time embrace checks for NULL when performing logical operations.
- Overusing NULLs: Whereas NULLs may be helpful, overusing them can complicate knowledge evaluation and reporting. Try for a stability, making certain that NULLs are used appropriately with out cluttering the dataset.
- Ignoring Documentation: Neglecting to doc your methods for managing NULL values can result in confusion and inconsistency amongst group members. Clear documentation is crucial for efficient knowledge administration.
- Neglecting Common Audits of NULL Values: Common audits of NULL values assist keep knowledge integrity and high quality. Ignoring this step may end up in accumulating errors and misinterpretations in your knowledge evaluation.
Conclusion
Dealing with NULL values in SQL requires cautious consideration to keep away from skewing and affecting knowledge evaluation. You possibly can remedy points with NULLs by deliberately utilizing NULL, organising constraints within the database, and auditing data every day. Additional, there are particular pitfalls that, if familiarized with—reminiscent of complicated NULL with zero or failure to account for NULLs in logical operations—will enhance knowledge manipulation skilled strategies. Lastly and extra importantly an acceptable administration of NULL values enhances question and reporting credibility and encourages appreciation of knowledge environments and thus the formation of the correct selections/insights a couple of explicit knowledge.
Regularly Requested Questions
A. NULL represents a lacking or undefined worth in SQL, indicating the absence of knowledge.
A. Use IS NULL
or IS NOT NULL
to examine for NULL values in SQL queries.
A. Sure, mixture capabilities ignore NULL values, which might impression the outcomes.
A. You should use the COALESCE
, IFNULL
, or ISNULL
capabilities to interchange NULL values with a specified default.
A. Whereas NULLs may be obligatory, it’s typically finest to attenuate their use by implementing NOT NULL constraints and offering default values the place acceptable.