Introduction
As the necessity for environment friendly software program growth continues, synthetic intelligence turns into a precious colleague for programmers. AI-powered coding assistants are altering the sport by making it simpler for builders to write down, debug, and optimize their code like pair programmers. On this article, we’ll discover ways to construct an AI pair programmer utilizing CrewAI brokers to simplify your coding duties and enhance your productiveness in software program growth.
Overview
- Grasp the fundamentals of CrewAI and its position in aiding coding duties.
- Acknowledge key elements—Brokers, Duties, Instruments, and Crews—and their interactions.
- Achieve hands-on expertise in establishing AI brokers for code technology and overview.
- Be taught to configure a number of AI brokers to work collectively on coding duties.
- Develop expertise to make use of CrewAI to guage and optimize code high quality.
What Can an AI Pair Programmer Do?
Let’s discover just a few use circumstances of what an AI pair programmer can do.
- Write code: We will generate the code for a given downside utilizing an AI agent and overview it with one other agent.
- Enhance current code: If we write the code, our pair programmer can consider it based mostly on the analysis necessities.
- Optimize code: We will ask for any modifications to the code to optimize it, comparable to including feedback, a doc string, and so on.
- Debug code: Errors are inevitable within the code. However not like rubber duck, our pair programmer could make ideas.
- Write take a look at circumstances: Let the AI agent write the take a look at circumstances for each edge case. We will even use it for test-driven growth.
On this article, we are going to cowl the primary two duties.
What’s CrewAI?
CrewAI is a well-liked framework for constructing AI brokers. It consists of key elements comparable to Brokers, Duties, Instruments, and Crews.
- Agent: At its core, an agent makes use of a big language mannequin (LLM) to generate outputs based mostly on enter prompts. It will possibly name varied instruments, settle for human enter, and talk with different brokers to finish duties.
- Job: A process outlines what the agent will accomplish, specifying the outline, the agent to make use of, and the instruments that may be known as upon.
- Instrument: Brokers can make the most of varied instruments to carry out duties like net searches, studying recordsdata, and executing code enhancing the capabilities of the AI Agent.
- Crew: A crew is a gaggle of brokers collaborating to finish a set of duties. Every crew defines how brokers work together, share info, and delegate tasks.
Additionally Learn: Constructing Collaborative AI Brokers With CrewAI
Now, let’s construct an agent to realize a greater understanding!
What are the Stipulations?
Earlier than constructing an AI Pair Programmer with a CrewAI agent, guarantee you will have the API keys for LLMs.
Accessing an LLM through API
Begin by producing an API key for the LLM you propose to make use of. Then, create a .env file to retailer this key securely. It will maintain it personal whereas making it simply accessible inside your mission.
Instance of a .env File
Right here’s an instance of what a .env file seems like:

Libraries Required
We now have used the next variations for the foremost libraries:
- crewai – 0.66.0
- crewai-tools – 0.12.1
Automating Code Creation with CrewAI
On this part, we are going to import the required libraries and outline brokers to generate and overview code. This hands-on strategy will enable you to perceive the right way to make the most of CrewAI successfully.
Import the Mandatory Libraries
from dotenv import load_dotenv
load_dotenv('/.env')
from crewai import Agent, Job, Crew
Defining the Code Author Agent
We will use one agent to generate the code and one other agent to overview the code.
code_writer_agent = Agent(position="Software program Engineer",
purpose="Write optimized code for a given process",
backstory="""You're a software program engineer who writes code for a given process.
The code needs to be optimized, and maintainable and embody doc string, feedback, and so on.""",
llm='gpt-4o-mini',
verbose=True)
Clarification of Agent Parameters
- position: Defines what the agent is and defines it based mostly on the duties we would like the agent to finish.
- Purpose: Defines what the agent tries to realize. The agent makes the choices to realize this purpose.
- backstory: Gives context to the position and purpose of the agent. This can be utilized for higher interplay with the agent as that is despatched because the immediate to the LLM.
- llm: LLM used within the agent. CrewAI makes use of LiteLLM to name the LLMs, so seek advice from its documentation for the out there fashions.
- verbose: we will set verbose=True to take a look at the enter and output of the agent.
Defining the Code Author Job
code_writer_task = Job(description='Write the code to resolve the given downside within the {language} programming language.'
'Downside: {downside}',
expected_output="Effectively formatted code to resolve the issue. Embody sort hinting",
agent=code_writer_agent)
Clarification of Job Parameters
- description: write a transparent and detailed assertion of aims for the duty that must be accomplished. curly braces {} are used to point variables. On this case, we move the ‘downside’ and ‘language’ values to the duty.
- expected_output: How the output of the duty ought to appear to be. We will additionally get structured output utilizing Pydantic lessons.
- agent: defines the agent that needs to be used to realize this process.
Defining the Code Reviewer Agent and Job
Equally, let’s outline code_reviewer_agent and code_reviewer_task.
code_reviewer_agent = Agent(position="Senior Software program Engineer",
purpose="Make sure that the code written is optimized and maintainable",
backstory="""You're a Senior software program engineer who critiques the code written for a given process.
It is best to verify the code for readability, maintainability, and efficiency.""",
llm='gpt-4o-mini',
verbose=True)
code_reviewer_task = Job(description="""A software program engineer has written this code for the given downside
within the {language} programming language.' Assessment the code critically and
make any modifications to the code if vital.
'Downside: {downside}""",
expected_output="Effectively formatted code after the overview",
agent=code_reviewer_agent)
Constructing and Operating the Crew
Now, we will construct the crew and run it:
crew = Crew(brokers=[code_writer_agent, code_reviewer_agent],
duties=[code_writer_task, code_reviewer_task],
verbose=True)
outcome = crew.kickoff(inputs={'downside': 'create a recreation of tic-tac-toe', 'language': 'Python'})
The pattern output will likely be as follows:
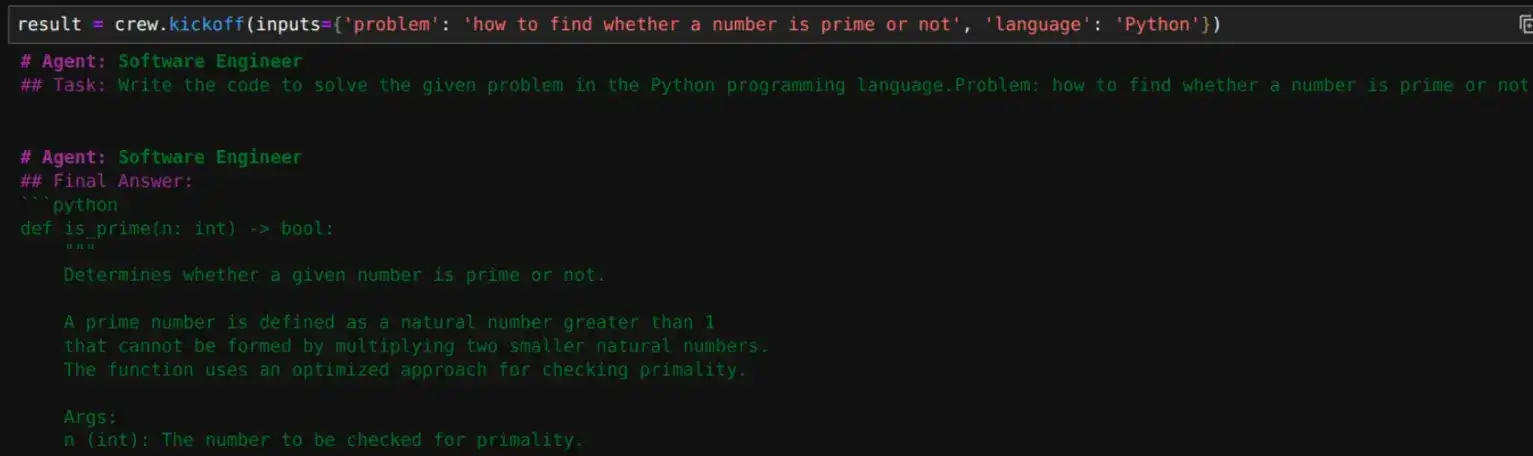
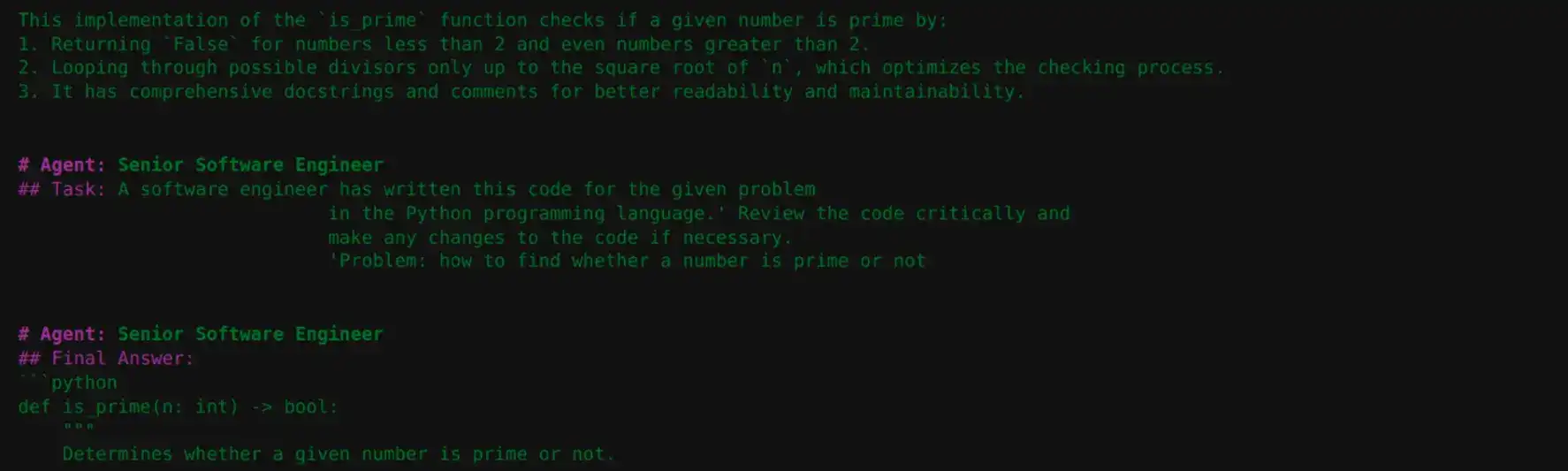
End result
The outcome may have the next
outcome.dict().keys()
>>> dict_keys(['raw', 'pydantic', 'json_dict', 'tasks_output', 'token_usage'])
# we will additionally verify token utilization
outcome.dict()['token_usage']
>>> {'total_tokens': 2656,
'prompt_tokens': 1425,
'completion_tokens': 1231,
'successful_requests': 3}
# we will print the ultimate outcome
print(outcome.uncooked)
We will run the code generated by the Agent:
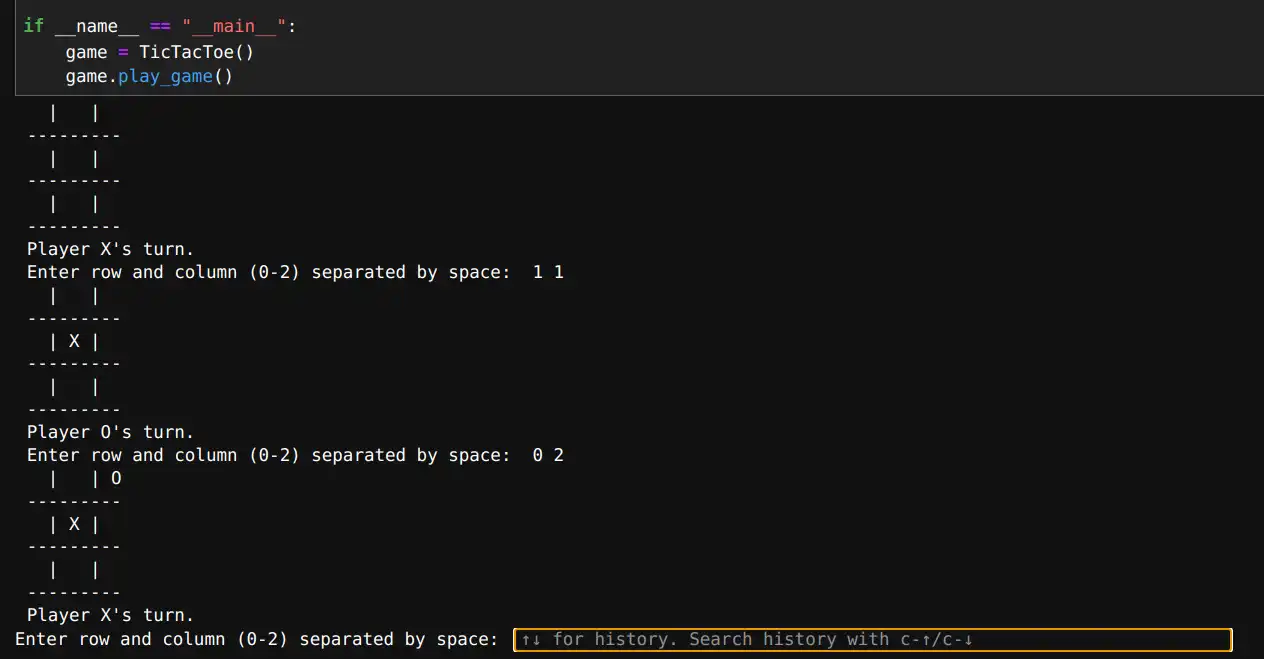
Automated Code Analysis with Crew AI
After constructing the code technology and overview brokers, we are going to now consider an current code file.
Gathering Necessities
First, we are going to collect analysis necessities for an issue utilizing an agent after which consider the code based mostly on these necessities utilizing one other agent.
Utilizing Instruments for Enhanced Capabilities
We are going to use the FileReadTool to learn recordsdata from the system. Instruments improve agent capabilities by enabling actions like studying recordsdata or looking the Web.
We will assign instruments to each duties and brokers. Instruments assigned in Duties will override instruments within the agent.
Initialize Agent and Job for Requirement Gathering
code_requirements_agent = Agent(position="Knowledge Scientist",
purpose="present are all issues that needs to be required within the code to resolve the given downside.",
backstory="""You're a Knowledge Scientist who decides what are all issues required
within the code to resolve a given downside/process. The code will likely be written based mostly on
the necessities supplied by you.""",
llm='gpt-4o-mini',
verbose=True)
code_requirement_task = Job(description='Write the necessities for the given downside step-by-step.'
'Downside: {downside}',
expected_output="Effectively formatted textual content which specifies what's required to resolve the issue.",
agent=code_requirements_agent,
human_input=True)
Within the above process, we’ve got assigned human_input to True. So, the agent asks for enter from the consumer as soon as it generates the necessities. We will ask it to make any modifications if required.
Evaluating the Code
Now, do the identical analysis. Right here, we use the instrument to learn the file. We additionally use GPT-4o for higher output because the context measurement is bigger.
from crewai_tools import DirectoryReadTool, FileReadTool
file_read_tool = FileReadTool('EDA.py')
code_evaluator_agent = Agent(position="Knowledge Science Evaluator",
purpose="Consider the given code file based mostly on the necessities supplied for a given downside",
backstory="""You're a Knowledge Science evaluator who critiques and evaluates the code.
It is best to verify the code based mostly on the necessities given to you""",
llm='gpt-4o',
verbose=True)
code_evaluator_task = Job(description="""A code file is given to you.
Consider the file based mostly on the necessities given because the context.
Present the one overview and analysis of the code because the output, not the code.
""",
expected_output="Detailed analysis outcomes of the code file based mostly on the necessities."
'Assessment the code file for every level of the necessities given to you'
'Present analysis outcomes as textual content',
instruments=[file_read_tool],
agent=code_evaluator_agent)
Constructing the Crew for Analysis
Allow us to construct the crew and outline the issue to get the necessities for it.
crew = Crew(brokers=[code_requirements_agent, code_evaluator_agent],
duties=[code_requirement_task, code_evaluator_task],
verbose=True)
downside = """
Carry out EDA on the NYC taxi journey length dataset.
Right here is the outline of all of the variables/options out there within the dataset which is able to enable you to to carry out EDA:
id - a singular identifier for every journey
vendor_id - a code indicating the supplier related to the journey document
pickup_datetime - date and time when the meter was engaged
dropoff_datetime - date and time when the meter was disengaged
passenger_count - the variety of passengers within the car (driver entered worth)
pickup_longitude - the longitude the place the meter was engaged
pickup_latitude - the latitude the place the meter was engaged
dropoff_longitude - the longitude the place the meter was disengaged
dropoff_latitude - the latitude the place the meter was disengaged
store_and_fwd_flag - This flag signifies whether or not the journey document was held in car reminiscence earlier than sending to the seller as a result of the car didn't have a connection to the server (Y=retailer and ahead; N=not a retailer and ahead journey)
trip_duration - (goal) length of the journey in seconds
"""
outcome = crew.kickoff(inputs={'downside': downside})
Output from Duties
Right here’s how the outcome will appear to be whereas asking for human enter:
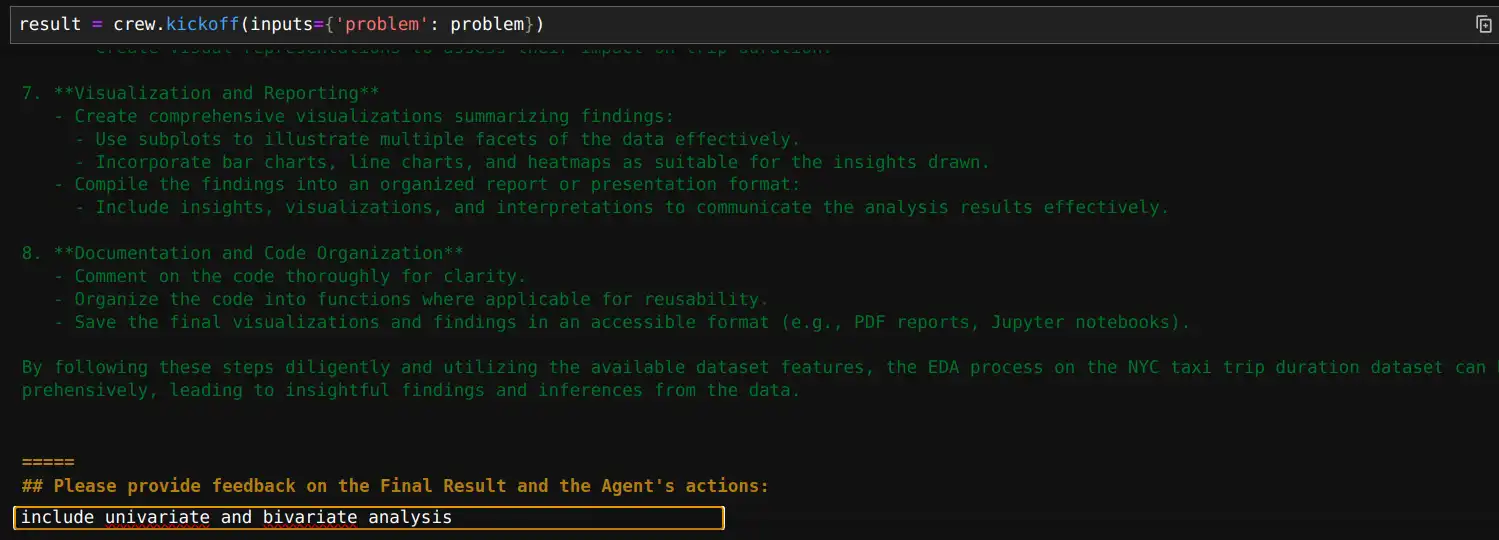
We will additionally get the output of any process as follows:
print(code_requirement_task.output.uncooked)
# closing output
print(outcome.uncooked)
On this method, we will construct versatile crews to make our personal AI pair programmer.
Conclusion
CrewAI affords a robust framework for enhancing software program growth by leveraging AI brokers to automate code technology, overview, and analysis duties. Builders can streamline their workflow and enhance productiveness by defining clear roles, objectives, and duties for every agent. Incorporating an AI Pair Programmer with CrewAI into your software program growth workflow can considerably improve productiveness and code high quality.
CrewAI’s versatile framework permits for seamless collaboration between AI brokers, guaranteeing your code is optimized, maintainable, and error-free. As AI expertise evolves, leveraging instruments like CrewAI for pair programming will turn out to be a vital technique for builders to streamline their work and enhance effectivity. With its versatile instruments and collaborative options, CrewAI has the potential to revolutionize how we strategy programming, making the method extra environment friendly and efficient.
Often Requested Questions
A. CrewAI is a framework that leverages AI brokers. It may be used to help builders with duties like writing, reviewing, and evaluating code. It enhances productiveness by automating repetitive duties, permitting builders to give attention to extra complicated elements of growth.
A. The core elements of CrewAI embody Brokers, Duties, Instruments, and Crews. Brokers carry out actions based mostly on their outlined roles, Duties specify the aims, Instruments lengthen the brokers’ capabilities, and Crews enable a number of brokers to collaborate on complicated workflows.
A. To arrange an AI agent, you outline its position (e.g., “Software program Engineer”), purpose (e.g., “Write optimized code”), and backstory for context, and specify the LLM (language mannequin) for use. You additionally create a corresponding Job that particulars the issue and anticipated output.
A. Sure, CrewAI brokers can collaborate on duties by being a part of a “Crew.” Every agent within the crew can have a selected process, comparable to writing code or reviewing it, permitting for environment friendly teamwork amongst AI brokers.
A. CrewAI brokers can use varied instruments to boost their capabilities, comparable to studying recordsdata, conducting net searches, or working code. These instruments will be assigned to brokers and duties, permitting for extra dynamic and highly effective workflows.