Introduction
Python is extensively utilized by builders since it’s a simple language to be taught and implement. One its sturdy sides is that there are lots of samples of helpful and concise code that will assist to resolve particular issues. No matter whether or not you’re coping with recordsdata, knowledge, or internet scraping these snippets will aid you save half of the time you’ll have dedicated to typing code. Right here we’ll talk about 30 Python code by explaining them intimately as a way to clear up day after day programming issues successfully.
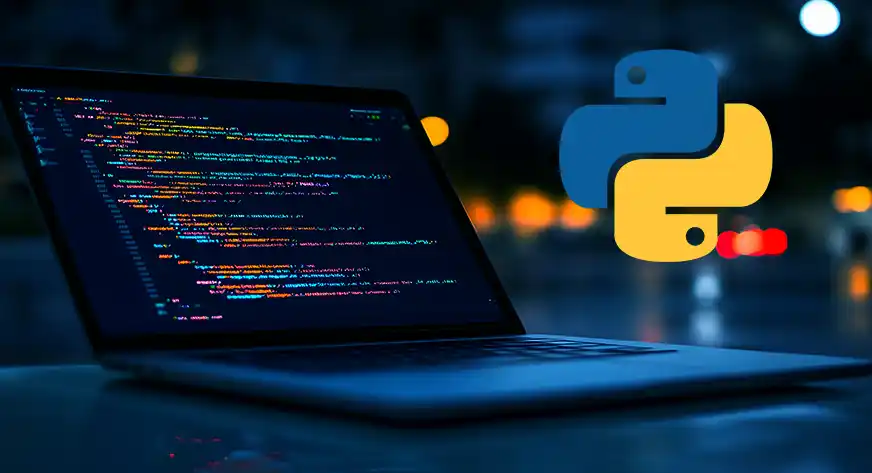
Studying Outcomes
- Study to implement widespread Python code snippets for on a regular basis duties.
- Perceive key Python ideas akin to file dealing with, string manipulation, and knowledge processing.
- Develop into aware of environment friendly Python methods like record comprehensions, lambda features, and dictionary operations.
- Achieve confidence in writing clear, reusable code for fixing widespread issues rapidly.
Why You Ought to Use Python Code Snippets
Each programmer is aware of that Python code snippets are efficient in any venture. This manner of easily integrating new components arrives from immediately making use of pre-written code block templates for various duties. Snippets aid you to focus on sure venture facets with out typing monotonous code line by line. They’re Most worthy to be used instances like record processing, file I/O, and string formatting—issues you will do with close to certainty in virtually any venture that you just’ll write utilizing Python.
As well as, snippets are helpful in you can check with them as you write your code, thus stopping the errors that accrue from writing comparable primary code time and again. That’s the reason, having discovered and repeated snippets, it’s doable to realize the development of functions which might be cleaner, extra sparing of system sources, and extra sturdy.
30 Python Code Snippets for Your On a regular basis Use
Allow us to discover 30 python code snippets in your on a regular basis use under:
Studying a File Line by Line
This snippet opens a file and reads it line by line utilizing a for
loop. The with
assertion ensures the file is correctly closed after studying. strip()
removes any further whitespace or newline characters from every line.
with open('filename.txt', 'r') as file:
for line in file:
print(line.strip())
Writing to a File
We open a file for writing utilizing the w
mode. If the file doesn’t exist, Python creates it. The write()
methodology provides content material to the file. This method is environment friendly for logging or writing structured output.
with open('output.txt', 'w') as file:
file.write('Good day, World!')
Checklist Comprehension for Filtering
This snippet makes using record comprehension the place a brand new record is created with solely even numbers. The situation n % 2 == 0 appears for even numbers and Python creates a brand new record containing these numbers.
numbers = [1, 2, 3, 4, 5, 6]
even_numbers = [n for n in numbers if n % 2 == 0]
print(even_numbers)
Lambda Operate for Fast Math Operations
Lambda features are these perform that are outlined as inline features utilizing the lambda key phrase. On this instance, a full perform will not be outlined and a lambda perform merely provides two numbers immediately.
add = lambda x, y: x + y
print(add(5, 3))
Reversing a String
This snippet reverses a string utilizing slicing. The [::-1]
syntax slices the string with a step of -1
, which means it begins from the tip and strikes backward.
string = "Python"
reversed_string = string[::-1]
print(reversed_string)
Merging Two Dictionaries
We merge two dictionaries utilizing the **
unpacking operator. This methodology is clear and environment friendly, significantly for contemporary Python variations (3.5+).
dict1 = {'a': 1, 'b': 2}
dict2 = {'c': 3, 'd': 4}
merged_dict = {**dict1, **dict2}
print(merged_dict)
Sorting a Checklist of Tuples
The next snippet kinds the given record of tuples with the assistance of dictionary key perform set to lambda perform. Particularly, the sorted() perform type an inventory in ascending or in descending order, in accordance with the parameter handed to it; the brand new record was created for brand spanking new sorted values.
tuples = [(2, 'banana'), (1, 'apple'), (3, 'cherry')]
sorted_tuples = sorted(tuples, key=lambda x: x[0])
print(sorted_tuples)
Fibonacci Sequence Generator
The generator perform yields the primary n
numbers within the Fibonacci sequence. Turbines are memory-efficient as they produce objects on the fly moderately than storing your entire sequence in reminiscence.
def fibonacci(n):
a, b = 0, 1
for _ in vary(n):
yield a
a, b = b, a + b
for num in fibonacci(10):
print(num)
Examine for Prime Quantity
On this snippet it’s counting that if the quantity is a chief quantity utilizing for loop from 2 to quantity sq. root. This being the case the quantity will be divided by any of the numbers on this vary, then the quantity will not be prime.
def is_prime(num):
if num < 2:
return False
for i in vary(2, int(num ** 0.5) + 1):
if num % i == 0:
return False
return True
print(is_prime(29))
Take away Duplicates from a Checklist
Most programming languages have a built-in perform to transform an inventory right into a set by which you eradicate duplicates as a result of units can’t let two objects be the identical. Lastly, the result’s transformed again to record from the iterable that’s obtained from utilizing the rely perform.
objects = [1, 2, 2, 3, 4, 4, 5]
unique_items = record(set(objects))
print(unique_items)
Easy Net Scraper with requests
and BeautifulSoup
This internet scraper makes use of Python’s ‘requests library’ the retrieves the web page and BeautifulSoup to investigate the returned HTML. It additionally extracts the title of the web page and prints it.
import requests
from bs4 import BeautifulSoup
response = requests.get('https://instance.com')
soup = BeautifulSoup(response.textual content, 'html.parser')
print(soup.title.textual content)
Convert Checklist to String
The be part of() methodology combines components in an inventory in to type a string that’s delimited by a selected string (in our case it’s ‘, ‘).
objects = ['apple', 'banana', 'cherry']
outcome=", ".be part of(objects)
print(outcome)
Get Present Date and Time
The datetime.now() perform return present date and time. The strftime() methodology codecs it right into a readable string format (YYYY-MM-DD HH:MM:SS).
from datetime import datetime
now = datetime.now()
print(now.strftime('%Y-%m-%d %H:%M:%S'))
Random Quantity Technology
This snippet generates a random integer between 1 and 100 utilizing the random.randint()
perform, helpful in situations like simulations, video games, or testing.
import random
print(random.randint(1, 100))
Flatten a Checklist of Lists
Checklist comprehension flattens an inventory of lists by iterating by means of every sublist and extracting the weather right into a single record.
list_of_lists = [[1, 2], [3, 4], [5, 6]]
flattened = [item for sublist in list_of_lists for item in sublist]
print(flattened)
Calculate Factorial Utilizing Recursion
Recursive perform calculates the factorial of a quantity by calling itself. It terminates when n
equals zero, returning 1 (the bottom case).
def factorial(n):
return 1 if n == 0 else n * factorial(n - 1)
print(factorial(5))
Swap Two Variables
Python trick lets you interchange the values saved in two variables with out utilizing a 3rd or temporary variable. It’s a easy one-liner.
a, b = 5, 10
a, b = b, a
print(a, b)
Take away Whitespace from String
The strip() is the usual methodology that, when referred to as, strips all whitespaces that seem earlier than and after a string. It’s useful when one desires to tidy up their enter knowledge earlier than evaluation.
textual content = " Good day, World! "
cleaned_text = textual content.strip()
print(cleaned_text)
Discover the Most Aspect in a Checklist
The max() perform find and return the largest worth inside a given record. It’s an efficient methodology to get the utmost of record of numbers, as a result of it’s quick.
numbers = [10, 20, 30, 40, 50]
max_value = max(numbers)
print(max_value)
Examine If a String is Palindrome
This snippet checks if a string is a palindrome by evaluating the string to its reverse ([::-1]
), returning True
if they’re similar.
def is_palindrome(string):
return string == string[::-1]
print(is_palindrome('madam'))
Depend Occurrences of an Aspect in a Checklist
The rely()
methodology returns the variety of occasions a component seems in an inventory. On this instance, it counts what number of occasions the quantity 3
seems.
objects = [1, 2, 2, 3, 3, 3, 4, 4, 4, 4]
rely = objects.rely(3)
print(rely)
Create a Dictionary from Two Lists
The zip()
perform pairs components from two lists into tuples, and the dict()
constructor converts these tuples right into a dictionary. It’s a easy technique to map keys to values.
keys = ['name', 'age', 'job']
values = ['John', 28, 'Developer']
dictionary = dict(zip(keys, values))
print(dictionary)
Shuffle a Checklist
This snippet shuffles an inventory in place utilizing the random.shuffle()
methodology, which randomly reorders the record’s components.
import random
objects = [1, 2, 3, 4, 5]
random.shuffle(objects)
print(objects)
Filter Parts Utilizing filter()
Filter() perform works on an inventory and takes a situation that may be a lambda perform on this case then it should return solely these components of the record for which the situation is true (all of the even numbers right here).
numbers = [1, 2, 3, 4, 5, 6]
even_numbers = record(filter(lambda x: x % 2 == 0, numbers))
print(even_numbers)
Measure Execution Time of a Code Block
This snippet measures the execution time of a block of code utilizing time.time()
, which returns the present time in seconds. That is helpful for optimizing efficiency.
import time
start_time = time.time()
# Some code block
end_time = time.time()
print(f"Execution Time: {end_time - start_time} seconds")
Convert Dictionary to JSON
The json.dumps() perform take Python dictionary and returns JSON formatted string. That is particularly helpful with regards to making API requests or when storing info in a JSON object.
import json
knowledge = {'identify': 'John', 'age': 30}
json_data = json.dumps(knowledge)
print(json_data)
Examine If a Key Exists in a Dictionary
This snippet checks if a key exists in a dictionary utilizing the in
key phrase. If the secret’s discovered, it executes the code within the if
block.
particular person = {'identify': 'Alice', 'age': 25}
if 'identify' in particular person:
print('Key exists')
Zip A number of Lists
The zip()
perform combines a number of lists by pairing components with the identical index. This may be helpful for creating structured knowledge from a number of lists.
names = ['Alice', 'Bob', 'Charlie']
ages = [25, 30, 35]
zipped = record(zip(names, ages))
print(zipped)
Generate Checklist of Numbers Utilizing vary()
The vary()
perform generates a sequence of numbers, which is transformed into an inventory utilizing record()
. On this instance, it generates numbers from 1 to 10.
numbers = record(vary(1, 11))
print(numbers)
Examine If a Checklist is Empty
This snippet checks if an inventory is empty by utilizing not
. If the record accommodates no components, the situation evaluates to True
.
objects = []
if not objects:
print("Checklist is empty")
Greatest Practices for Reusing Code Snippets
When reusing code snippets, following a couple of finest practices will assist make sure that your initiatives preserve high quality and effectivity:
- Perceive Earlier than Utilizing: Keep away from blindly copying snippets. Take the time to grasp how every snippet works, its inputs, and its outputs. This may aid you keep away from unintended bugs.
- Take a look at in Isolation: Take a look at snippets in isolation to make sure they carry out as anticipated. It’s important to confirm that the snippet works appropriately within the context of your venture.
- Remark and Doc: When modifying a snippet in your wants, all the time add feedback. Documenting adjustments will aid you or different builders perceive the logic afterward.
- Observe Coding Requirements: Be certain that snippets adhere to your coding requirements. They need to be written clearly and observe conventions like correct variable naming and formatting.
- Adapt to Your Use Case: Don’t hesitate to adapt a snippet to suit your particular venture wants. Modify it to make sure it really works optimally along with your codebase.
Creating a private library of Python code snippets is beneficial and may eradicate the necessity to seek for codes in different venture. A number of instruments can be found that will help you retailer, set up, and rapidly entry your snippets:
- GitHub Gists: By means of use of Gists, GitHub gives an exemplary method of storing and sharing snippets of code effortlessly. These snippets will be both public or non-public in nature which makes it may be accessed from any location and machine.
- VS Code Snippets: Visible Studio Code additionally built-in a strong snippet supervisor in which you’ll create your individual snippet units with assignable shortcuts to be utilized throughout numerous initiatives. It additionally provides snippets the flexibility to categorize them by language.
- SnipperApp: For Mac customers, SnipperApp gives an intuitive interface to handle and categorize your code snippets, making them searchable and simply accessible when wanted.
- Chic Textual content Snippets: Furthermore, Chic Textual content additionally provides you the choice to grasp and even customise the snippets built-in your growth surroundings.
- Snippet Supervisor for Home windows: This device can help builders on home windows platforms to categorize, tag, and seek for snippets simply.
Suggestions for Optimizing Snippets for Efficiency
Whereas code snippets assist streamline growth, it’s necessary to make sure that they’re optimized for efficiency. Listed below are some tricks to hold your snippets quick and environment friendly:
- Reduce Loops: If that is doable, eradicate loops and use record comprehensions that are quicker in Python.
- Use Constructed-in Capabilities: Python connected features, sum(), max() or min(), are written in C and are normally quicker than these carried out by hand.
- Keep away from International Variables: I additionally got here throughout some particular points when growing snippets, one in all which is the utilization of world variables, which leads to efficiency issues. Reasonably attempt to use native variables or to cross the variables as parameters.
- Environment friendly Knowledge Buildings: When retrieving data it necessary take the correct construction to finish the duty. As an illustration, use units to guarantee that membership examine is completed in a short while whereas utilizing dictionaries to acquire fast lookups.
- Benchmark Your Snippets: Time your snippets and use debugging and profiling instruments and if doable use time it to indicate you the bottlenecks. It helps in ensuring that your code will not be solely right but in addition right when it comes to, how briskly it might probably execute in your pc.
Widespread Errors to Keep away from When Utilizing Python Snippets
Whereas code snippets can save time, there are widespread errors that builders ought to keep away from when reusing them:
- Copy-Pasting With out Understanding: One fault was talked about to be particularly devastating – that of lifting the code and probably not realizing what one does. This ends in an misguided resolution when the snippet is then taken and utilized in a brand new setting.
- Ignoring Edge Instances: Snippets are good for a common case when the standard values are entered, however they don’t think about particular instances (for instance, an empty subject, however there could also be numerous different values). While you write your snippets, all the time attempt to run them with completely different inputs.
- Overusing Snippets: Nonetheless, if one makes use of snippets loads, he/she’s going to by no means actually get the language and simply be a Outsource Monkey, utilizing translation software program on each day foundation. Attempt to learn the way the snippets are carried out.
- Not Refactoring for Particular Wants: As one may guess from their identify, snippets are supposed to be versatile. If a snippet will not be custom-made to suit the precise venture it’s getting used on, then there’s a excessive chance that the venture will expertise some inefficiencies, or bugs it.
- Not Checking for Compatibility: Be certain that the snippet you’re utilizing is suitable with the model of Python you’re working with. Some snippets could use options or libraries which might be deprecated or version-specific.
Conclusion
These 30 Python code snippets cowl a big selection of widespread duties and challenges that builders face of their day-to-day coding. From dealing with recordsdata, and dealing with strings, to internet scraping and knowledge processing, these snippets save time and simplify coding efforts. Hold these snippets in your toolkit, modify them in your wants, and apply them in real-world initiatives to grow to be a extra environment friendly Python developer.
If you wish to be taught python without cost, right here is our introduction to python course!
Regularly Requested Questions
A. Observe commonly, discover Python documentation, and contribute to initiatives on GitHub.
A. Sure, they’re simple to grasp and useful for novices in addition to skilled builders.
A. Observe by utilizing them in real-world initiatives. Repetition will aid you recall them simply.
A. Completely! These snippets present a strong basis, however you’ll be able to construct extra advanced logic round them primarily based in your wants.