Within the age of data overload, it’s straightforward to get misplaced within the great amount of content material accessible on-line. YouTube gives billions of movies, and the web is full of articles, blogs, and educational papers. With such a big quantity of knowledge, it’s usually tough to extract helpful insights with out spending hours studying and watching. That’s the place AI-powered internet summarizer involves the assistance.
On this article, Let’s make a Streamlit-based app utilizing NLP and AI that summarizes YouTube movies and web sites in very detailed summaries. This app makes use of Groq’s Llama-3.2 mannequin and LangChain’s summarization chains to supply very detailed summaries, saving the reader time with out lacking any focal point.
Studying Outcomes
- Perceive the challenges of data overload and the advantages of AI-powered summarization.
- Discover ways to construct a Streamlit app that summarizes content material from YouTube and web sites.
- Discover the position of LangChain and Llama 3.2 in producing detailed content material summaries.
- Uncover easy methods to combine instruments like yt-dlp and UnstructuredURLLoader for multimedia content material processing.
- Construct a robust internet summarizer utilizing Streamlit and LangChain to immediately summarize YouTube movies and web sites.
- Create an internet summarizer with LangChain for concise, correct content material summaries from URLs and movies.
This text was printed as part of the Information Science Blogathon.
Goal and Advantages of the Summarizer App
From YouTube to webpage publications, or in-depth analysis articles, this huge repository of data is actually simply across the nook. Nevertheless, for many of us, the time issue guidelines out shopping by way of movies that run into a number of minutes or studying long-form articles. In accordance with research, an individual spends just some seconds on an internet site earlier than deciding to proceed to learn it or not. Now, right here is the issue that wants an answer.
Enter AI-powered summarization: a method that enables AI fashions to digest massive quantities of content material and supply concise, human-readable summaries. This may be significantly helpful for busy professionals, college students, or anybody who needs to shortly get the gist of a bit of content material with out spending hours on it.
Elements of the Summarization App
Earlier than diving into the code, let’s break down the important thing parts that make this utility work:
- LangChain: This highly effective framework simplifies the method of interacting with massive language fashions (LLMs). It offers a standardized solution to handle prompts, chain collectively completely different language mannequin operations, and entry a wide range of LLMs.
- Streamlit: This open-source Python library permits us to shortly construct interactive internet functions. It’s user-friendly and that make it excellent for creating the frontend of our summarizer.
- yt-dlp: When summarizing YouTube movies, yt_dlp is used to extract metadata just like the title and outline. In contrast to different YouTube downloaders, yt_dlp is extra versatile and helps a variety of codecs. It’s the perfect selection for extracting video particulars, that are then fed into the LLM for summarization.
- UnstructuredURLLoader: This LangChain utility helps us load and course of content material from web sites. It handles the complexities of fetching internet pages and extracting their textual info.
Constructing the App: Step-by-Step Information
On this part, we’ll stroll by way of every stage of creating your AI summarization app. We’ll cowl organising the surroundings, designing the consumer interface, implementing the summarization mannequin, and testing the app to make sure optimum efficiency.”
Word: Get the Necessities.txt file and Full code on GitHub right here.
Importing Libraries and Loading Atmosphere Variables
This step entails organising the important libraries wanted for the app, together with any machine studying and NLP frameworks. We’ll additionally load surroundings variables to securely handle API keys, credentials, and configuration settings required all through the event course of.
import os
import validators
import streamlit as st
from langchain.prompts import PromptTemplate
from langchain_groq import ChatGroq
from langchain.chains.summarize import load_summarize_chain
from langchain_community.document_loaders import UnstructuredURLLoader
from yt_dlp import YoutubeDL
from dotenv import load_dotenv
from langchain.schema import Doc
load_dotenv()
groq_api_key = os.getenv("GROQ_API_KEY")
This part import Libraries and masses the API key from an .env file, which retains delicate info like API keys safe.
Designing the Frontend with Streamlit
On this step, we’ll create an interactive and user-friendly interface for the app utilizing Streamlit. This contains including enter varieties, buttons, and displaying outputs, permitting customers to seamlessly work together with the backend functionalities.
st.set_page_config(page_title="LangChain Enhanced Summarizer", page_icon="🌟")
st.title("YouTube or Web site Summarizer")
st.write("Welcome! Summarize content material from YouTube movies or web sites in a extra detailed method.")
st.sidebar.title("About This App")
st.sidebar.information(
"This app makes use of LangChain and the Llama 3.2 mannequin from Groq API to offer detailed summaries. "
"Merely enter a URL (YouTube or web site) and get a concise abstract!"
)
st.header("Find out how to Use:")
st.write("1. Enter the URL of a YouTube video or web site you want to summarize.")
st.write("2. Click on **Summarize** to get an in depth abstract.")
st.write("3. Benefit from the outcomes!")
These traces set the web page configuration, title, and welcome textual content for the principle UI of the app.
Textual content Enter for URL and Mannequin Loading
Right here, we’ll arrange a textual content enter discipline the place customers can enter a URL to research. Moreover, we’ll combine the required mannequin loading performance to make sure that the app can course of the URL effectively and apply the machine studying mannequin as wanted for evaluation.
st.subheader("Enter the URL:")
generic_url = st.text_input("URL", label_visibility="collapsed", placeholder="https://instance.com")
Customers can enter the URL (YouTube or web site) they need summarized in a textual content enter discipline.
llm = ChatGroq(mannequin="llama-3.2-11b-text-preview", groq_api_key=groq_api_key)
prompt_template = """
Present an in depth abstract of the next content material in 300 phrases:
Content material: {textual content}
"""
immediate = PromptTemplate(template=prompt_template, input_variables=["text"])
The mannequin makes use of a immediate template to generate a 300-word abstract of the offered content material. This template is integrated into the summarization chain to information the method.
Defining Operate to Load YouTube Content material
On this step, we’ll outline a perform that handles fetching and loading content material from YouTube. This perform will take the offered URL, extract related video information, and put together it for evaluation by the machine studying mannequin built-in into the app.
def load_youtube_content(url):
ydl_opts = {'format': 'bestaudio/greatest', 'quiet': True}
with YoutubeDL(ydl_opts) as ydl:
information = ydl.extract_info(url, obtain=False)
title = information.get("title", "Video")
description = information.get("description", "No description accessible.")
return f"{title}nn{description}"
This perform makes use of yt_dlp to extract YouTube video info with out downloading it. It returns the video’s title and outline, which can be summarized by the LLM.
Dealing with the Summarization Logic
if st.button("Summarize"):
if not generic_url.strip():
st.error("Please present a URL to proceed.")
elif not validators.url(generic_url):
st.error("Please enter a legitimate URL (YouTube or web site).")
else:
attempt:
with st.spinner("Processing..."):
# Load content material from URL
if "youtube.com" in generic_url:
# Load YouTube content material as a string
text_content = load_youtube_content(generic_url)
docs = [Document(page_content=text_content)]
else:
loader = UnstructuredURLLoader(
urls=[generic_url],
ssl_verify=False,
headers={"Person-Agent": "Mozilla/5.0"}
)
docs = loader.load()
# Summarize utilizing LangChain
chain = load_summarize_chain(llm, chain_type="stuff", immediate=immediate)
output_summary = chain.run(docs)
st.subheader("Detailed Abstract:")
st.success(output_summary)
besides Exception as e:
st.exception(f"Exception occurred: {e}")
- If it’s a YouTube hyperlink, load_youtube_content extracts the content material, wraps it in a Doc, and shops it in docs.
- If it’s an internet site, UnstructuredURLLoader fetches the content material as docs.
Operating the Summarization Chain: The LangChain summarization chain processes the loaded content material, utilizing the immediate template and LLM to generate a abstract.
To provide your app a cultured look and supply important info, we’ll add a customized footer utilizing Streamlit. This footer can show essential hyperlinks, acknowledgments, or contact particulars, making certain a clear {and professional} consumer interface.
st.sidebar.header("Options Coming Quickly")
st.sidebar.write("- Choice to obtain summaries")
st.sidebar.write("- Language choice for summaries")
st.sidebar.write("- Abstract size customization")
st.sidebar.write("- Integration with different content material platforms")
st.sidebar.markdown("---")
st.sidebar.write("Developed with ❤️ by Gourav Lohar")
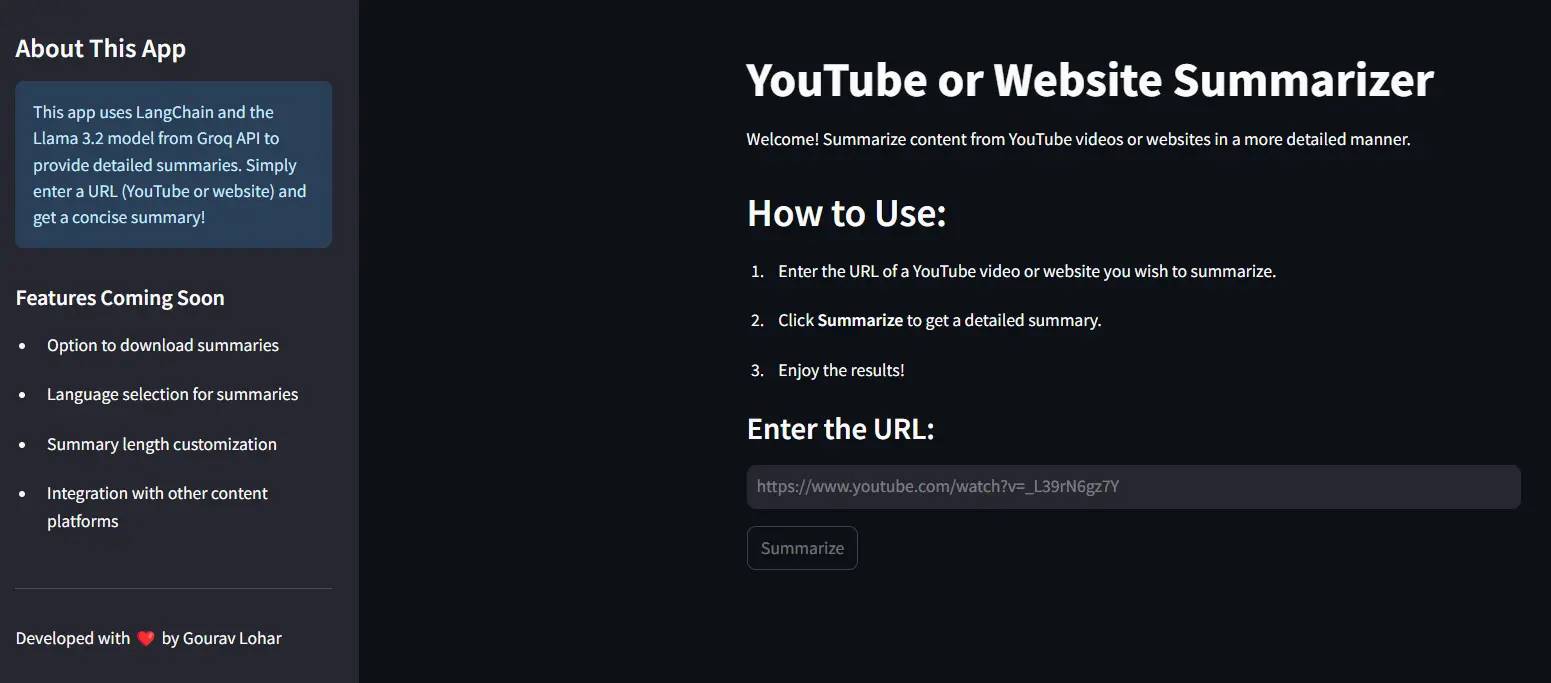
Output
Enter: https://www.analyticsvidhya.com/weblog/2024/10/nvidia-nim/
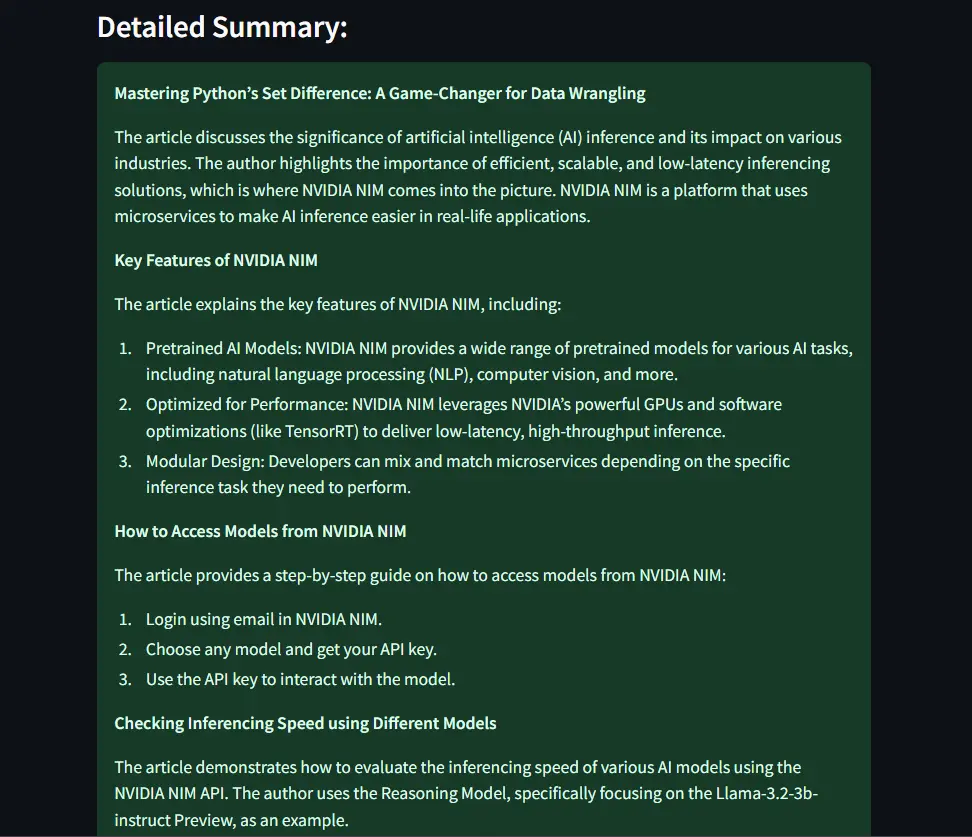
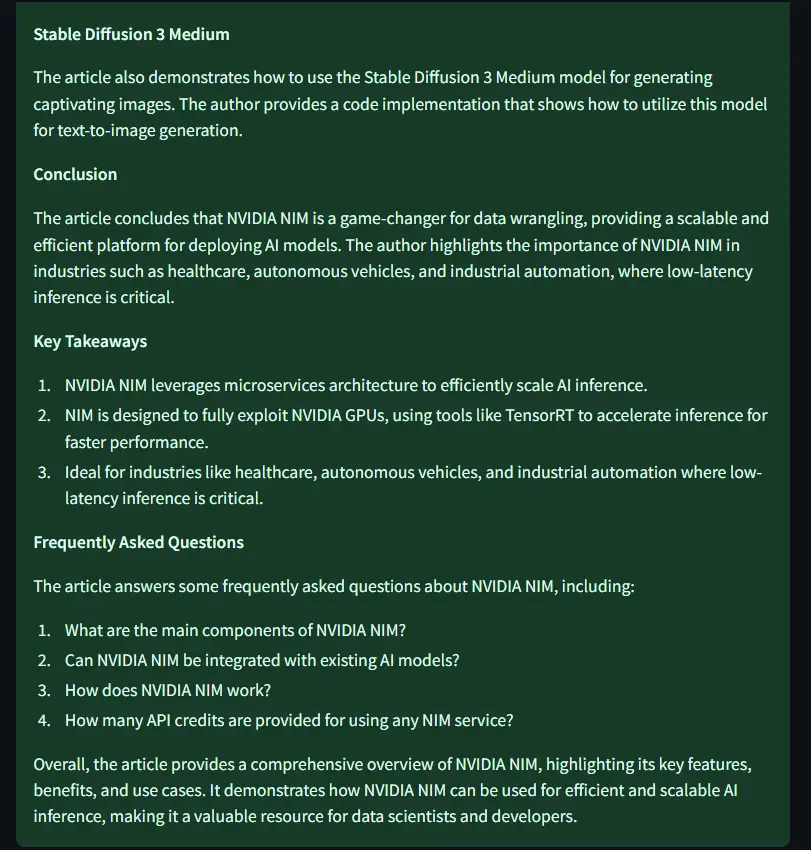
YouTube Video Summarizer
Enter Video:
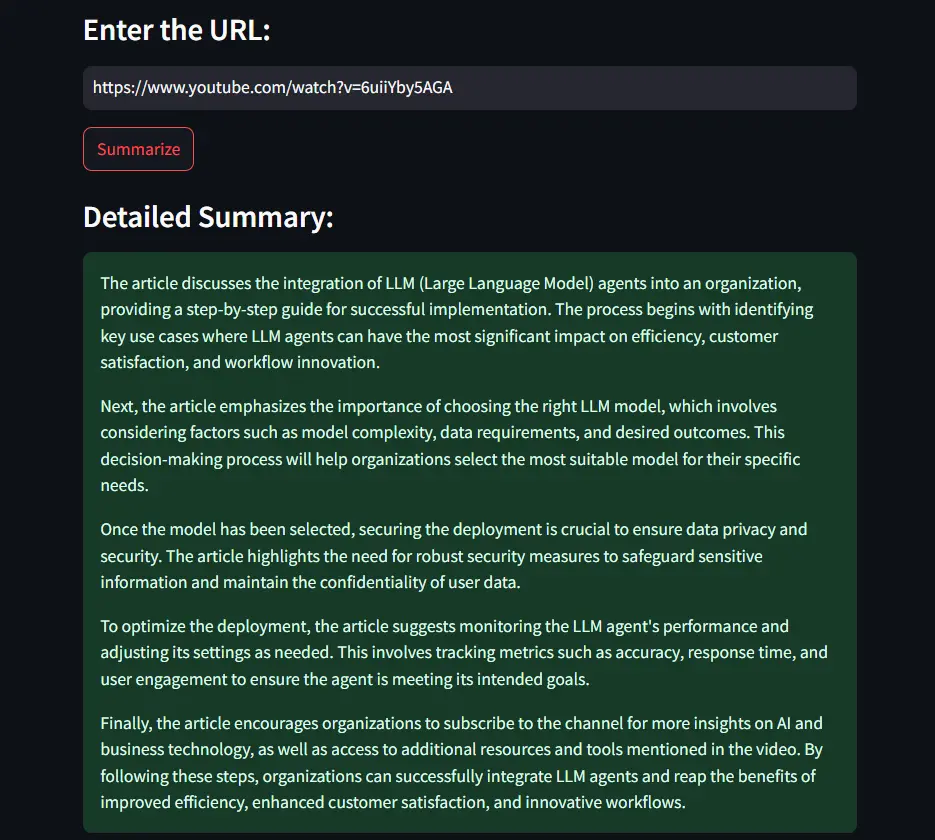
Conclusion
By leveraging LangChain’s framework, we streamlined the interplay with the highly effective Llama 3.2 language mannequin, enabling the era of high-quality summaries. Streamlit facilitated the event of an intuitive and user-friendly internet utility, making the summarization software accessible and interesting.
In conclusion, the article gives a sensible strategy and helpful concepts into making a complete abstract software. By combining cutting-edge language fashions with environment friendly frameworks and user-friendly interfaces, we will open up contemporary prospects for relieving info consumption and enhancing data acquisition in at the moment’s content-rich world.
Key Takeaways
- LangChain makes improvement simpler by providing a constant strategy to work together with language fashions, handle prompts, and chain processes.
- The Llama 3.2 mannequin from Groq API demonstrates robust capabilities in understanding and condensing info, leading to correct and concise summaries.
- Integrating instruments like yt-dlp and UnstructuredURLLoader permits the applying to deal with content material from numerous sources like YouTube and internet articles simply.
- The net summarizer makes use of LangChain and Streamlit to offer fast and correct summaries from YouTube movies and web sites.
- By leveraging the Llama 3.2 mannequin, the online summarizer effectively condenses advanced content material into easy-to-understand summaries.
Regularly Requested Questions
A. LangChain is a framework that simplifies interacting with massive language fashions. It helps handle prompts, chain operations, and entry numerous LLMs, making it simpler to construct functions like this summarizer.
A. Llama 3.2 generates high-quality textual content and excels at understanding and condensing info, making it well-suited for summarization duties. It’s also an open-source mannequin.
A. Whereas it could actually deal with a variety of content material, limitations exist. Extraordinarily lengthy movies or articles may require extra options like audio transcription or textual content splitting for optimum summaries.
A. At the moment, sure. Nevertheless, future enhancements might embody language choice for broader applicability.
A. It’s good to run the offered code in a Python surroundings with the required libraries put in. Examine GitHub for full code and necessities.txt.
The media proven on this article is just not owned by Analytics Vidhya and is used on the Writer’s discretion.