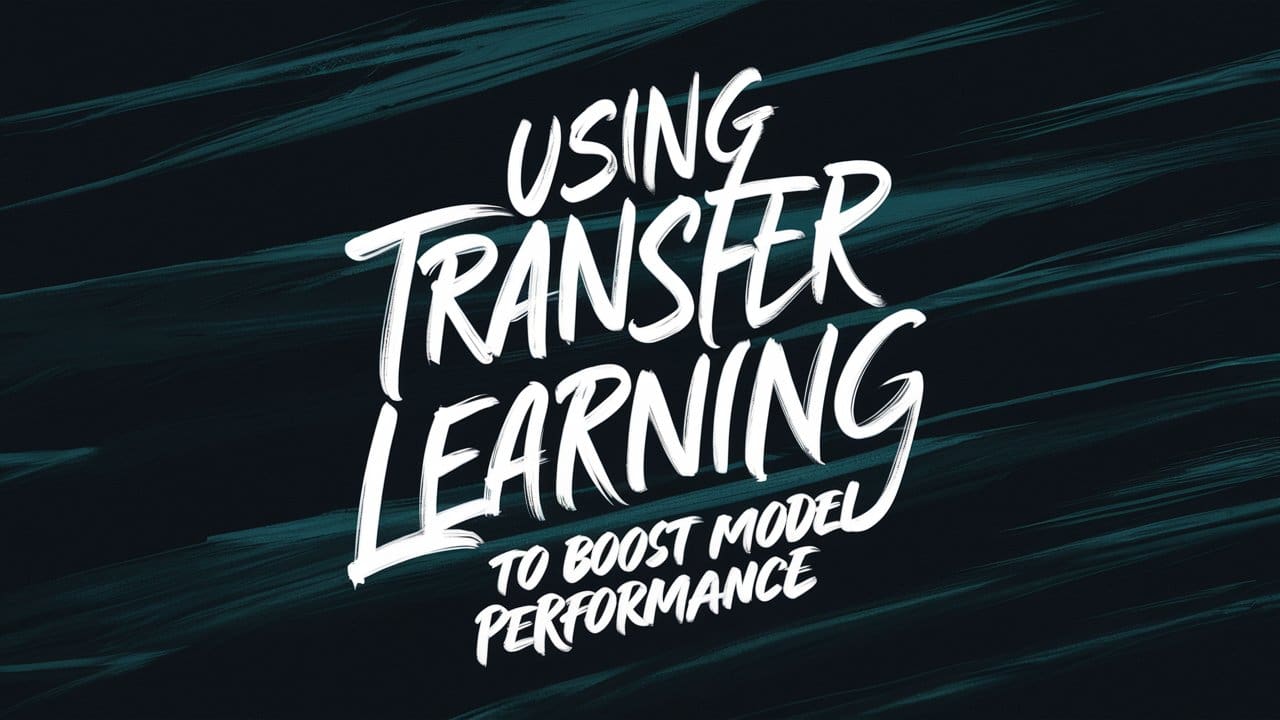
Have you considered how the efficiency of your ML fashions may be enhanced with out creating new fashions? That’s the place switch studying comes into play. On this article, we’ll present an outline of switch studying together with its advantages and challenges.
What’s Switch Studying?
Switch studying signifies that a mannequin educated for one process can be utilized for an additional comparable process. You possibly can then use a pre-trained mannequin and make modifications in it in keeping with the required process. Let’s focus on the phases in switch studying.


- Select a Pre-trained mannequin: Choose a mannequin that has been educated on a big dataset for the same process to the one you wish to work on.
- Modify mannequin structure: Modify the ultimate layers of the pre-trained mannequin in keeping with your particular process. Additionally, add new layers if wanted.
- Re-train the mannequin: Practice the modified mannequin in your new dataset. This permits the mannequin to be taught the main points of your particular process. It additionally advantages from the options it discovered in the course of the authentic coaching.
- High quality-tune the mannequin: Unfreeze among the pre-trained layers and proceed coaching your mannequin. This permits the mannequin to raised adapt to the brand new process by fine-tuning its weights.
Advantages of Switch Studying
Switch studying affords a number of vital benefits:
- Saves Time and Assets: High quality-tuning wants lesser time and computational sources because the pre-trained mannequin has been initially educated for a lot of iterations for a selected dataset. This course of has already captured important options, so it reduces the workload for the brand new process.
- Improves Efficiency: Pre-trained fashions have discovered from intensive datasets, in order that they generalize higher. This results in improved efficiency on new duties, even when the brand new dataset is comparatively small. The data gained from the preliminary coaching helps in attaining greater accuracy and higher outcomes.
- Wants Much less Knowledge: One of many main advantages of switch studying is its effectiveness with smaller datasets. The pre-trained mannequin has already acquired helpful sample and options info. Thus, it could possibly carry out pretty even whether it is given few new information.
Kinds of Switch Studying
Switch studying may be categorized into three varieties:
Function extraction
Function extraction means utilizing options discovered by a mannequin on new information. As an example, in picture classification, we will make the most of options from a predefined Convolutional Neural Community to seek for vital options in pictures. Right here’s an instance utilizing a pre-trained VGG16 mannequin from Keras for picture characteristic extraction:
import numpy as np
from tensorflow.keras.purposes import VGG16
from tensorflow.keras.preprocessing import picture
from tensorflow.keras.purposes.vgg16 import preprocess_input
# Load pre-trained VGG16 mannequin (with out the highest layers for classification)
base_model = VGG16(weights="imagenet", include_top=False)
# Operate to extract options from a picture
def extract_features(img_path):
img = picture.load_img(img_path, target_size=(224, 224)) # Load picture and resize
x = picture.img_to_array(img) # Convert picture to numpy array
x = np.expand_dims(x, axis=0) # Add batch dimension
x = preprocess_input(x) # Preprocess enter in keeping with mannequin's necessities
options = base_model.predict(x) # Extract options utilizing VGG16 mannequin
return options.flatten() # Flatten to a 1D array for simplicity
# Instance utilization
image_path="path_to_your_image.jpg"
image_features = extract_features(image_path)
print(f"Extracted options form: {image_features.form}")
High quality-tuning
High quality-tuning includes tweaking the characteristic extraction steps and the elements of a brand new mannequin matching the precise process. This methodology is most helpful with a mid-sized information set and the place you want to improve a selected task-related means of the mannequin. For instance, in NLP, a normal BERT mannequin is likely to be adjusted or additional educated on a small amount of medical texts to perform medical entity recognition higher. Right here’s an instance utilizing BERT for sentiment evaluation with fine-tuning on a customized dataset:
from transformers import BertTokenizer, BertForSequenceClassification, AdamW
import torch
from torch.utils.information import DataLoader, TensorDataset
# Instance information (change together with your dataset)
texts = ["I love this product!", "This is not what I expected.", ...]
labels = [1, 0, ...] # 1 for optimistic sentiment, 0 for adverse sentiment, and so forth.
# Load pre-trained BERT mannequin and tokenizer
model_name="bert-base-uncased"
tokenizer = BertTokenizer.from_pretrained(model_name)
mannequin = BertForSequenceClassification.from_pretrained(model_name, num_labels=2) # Instance: binary classification
# Tokenize enter texts and create DataLoader
inputs = tokenizer(texts, padding=True, truncation=True, return_tensors="pt")
dataset = TensorDataset(inputs['input_ids'], inputs['attention_mask'], torch.tensor(labels))
dataloader = DataLoader(dataset, batch_size=16, shuffle=True)
# High quality-tuning parameters
optimizer = AdamW(mannequin.parameters(), lr=1e-5)
# High quality-tune BERT mannequin
mannequin.prepare()
for epoch in vary(3): # Instance: 3 epochs
for batch in dataloader:
optimizer.zero_grad()
input_ids, attention_mask, goal = batch
outputs = mannequin(input_ids, attention_mask=attention_mask, labels=goal)
loss = outputs.loss
loss.backward()
optimizer.step()
Area adaptation
Area adaptation offers an perception on how one can make the most of data gained from the supply area that the pre-trained mannequin was educated on to the completely different goal area. That is required when the supply and goal domains differ on the options, the information distribution, and even on the language. As an example, in sentiment evaluation we might apply a sentiment classifier discovered from product critiques into social media posts as a result of the 2 makes use of very completely different language. Right here’s an instance utilizing sentiment evaluation, adapting from product critiques to social media posts:
# Operate to adapt textual content model
def adapt_text_style(textual content):
# Instance: change social media language with product review-like language
adapted_text = textual content.change("excited", "optimistic").change("#innovation", "new know-how")
return adapted_text
# Instance utilization of area adaptation
social_media_post = "Excited in regards to the new tech! #innovation"
adapted_text = adapt_text_style(social_media_post)
print(f"Tailored textual content: {adapted_text}")
# Use sentiment classifier educated on product critiques
# Instance: sentiment_score = sentiment_classifier.predict(adapted_text)
Pre-trained Fashions
Pretrained fashions are fashions already educated on massive datasets. They seize data and patterns from intensive information. These fashions are used as a place to begin for different duties. Let’s focus on among the widespread pre-trained fashions utilized in machine studying: purposes.
VGG (Visible Geometry Group)
The structure of VGG embody a number of layers of three×3 convolutional filters and pooling layers. It is ready to determine detailed options like edges and shapes in pictures. By coaching on massive datasets, VGG learns to acknowledge completely different objects inside pictures. It may used for object detection and picture segmentation.


ResNet (Residual Community)
ResNet makes use of residual connections to coach fashions. These connections make it simpler for gradients to movement by way of the community. This prevents the vanishing gradient downside, serving to the community prepare successfully. ResNet can efficiently prepare fashions with tons of of layers. ResNet is great for duties reminiscent of picture classification and face recognition.


BERT (Bidirectional Encoder Representations from Transformers)
BERT is used for pure language processing purposes. It makes use of a transformer-based mannequin to know the context of phrases in a sentence. It learns to guess lacking phrases and perceive sentence meanings. BERT can be utilized for sentiment evaluation, query answering and named entity recognition.


High quality-tuning Strategies
Layer Freezing
Layer freezing means selecting sure layers of a pre-trained mannequin and stopping them from altering throughout coaching with new information. That is achieved to protect the helpful patterns and options the mannequin discovered from its authentic coaching. Usually, we freeze early layers that seize common options like edges in pictures or primary constructions in textual content.
Studying Price Adjustment
Tuning the training fee is essential to stability what the mannequin has discovered and new information. Normally, fine-tuning includes utilizing a decrease studying fee than within the preliminary coaching with massive datasets. This helps the mannequin adapt to new information whereas preserving most of its discovered weights.
Challenges and Concerns
Let’s focus on the challenges of switch studying and methods to deal with them.
- Dataset Measurement and Area Shift: When fine-tuning, there needs to be ample of knowledge for the duty involved whereas fine-tuning generalized fashions. The disadvantage of this strategy is that in case the brand new dataset is both small or considerably completely different from what matches the mannequin initially. To cope with this, one can put extra information which will likely be extra related to what the mannequin already educated on.
- Hyperparameter Tuning: Altering hyperparameters is essential when working with pre educated fashions. These parameters are depending on one another and decide how good the mannequin goes to be. Strategies reminiscent of grid search or automated instruments to seek for essentially the most optimum settings for hyperparameters that might yield excessive efficiency on validation information.
- Computational Assets: High quality-tuning of deep neural networks is computationally demanding as a result of such fashions can have tens of millions of parameters. For coaching and predicting the output, highly effective accelerators like GPU or TPU are required. These calls for are often addressed by the cloud computing platforms.
Wrapping Up
In conclusion, switch studying stands as a cornerstone within the quest to reinforce mannequin efficiency throughout various purposes of synthetic intelligence. By leveraging pretrained fashions like VGG, ResNet, BERT, and others, practitioners can effectively harness present data to deal with advanced duties in picture classification, pure language processing, healthcare, autonomous methods, and past.
Jayita Gulati is a machine studying fanatic and technical author pushed by her ardour for constructing machine studying fashions. She holds a Grasp’s diploma in Pc Science from the College of Liverpool.