Introduction
Give it some thought such as you’re fixing a puzzle the place every of these SQL queries is part of the picture and you are attempting to get the entire image out of it. Listed below are the practices described on this information that educate you the best way to learn and write SQL queries. Whether or not you might be studying SQL from a learners perspective or from an expert programmer seeking to study new methods, decoding SQL queries will provide help to get via it and get the solutions sooner and with a lot ease. Start looking, and you’ll rapidly come to understand how the usage of SQL can revolutionize your considering course of by way of databases.
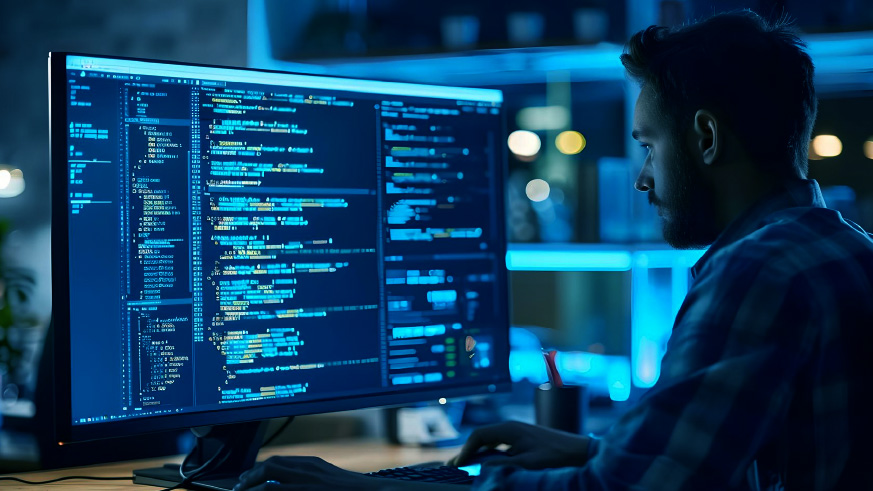
Overview
- Grasp the essential construction of SQL queries.
- Interpret varied SQL clauses and capabilities.
- Analyze and perceive complicated SQL queries.
- Debug and optimize SQL queries effectively.
- Apply superior strategies to understand intricate queries.
Fundamentals of SQL Question Construction
Earlier than diving into complicated queries, it’s important to grasp the elemental construction of an SQL question. SQL queries use varied clauses to outline what information to retrieve and the best way to course of it.
Parts of an SQL Question
- Statements: SQL statements carry out actions resembling retrieving, including, modifying, or eradicating information. Examples embrace SELECT, INSERT, UPDATE, and DELETE.
- Clauses: Clauses specify actions and circumstances inside statements. Widespread clauses embrace FROM (specifying tables), WHERE (filtering rows), GROUP BY (grouping rows), and ORDER BY (sorting outcomes).
- Operators: Operators carry out comparisons and specify circumstances inside clauses. These embrace comparability operators (=, <>, >, <), logical operators (AND, OR, NOT), and arithmetic operators (+, -, *, /).
- Features: Features carry out operations on information, resembling combination capabilities (COUNT, SUM, AVG), string capabilities (CONCAT), and date capabilities (NOW, DATEDIFF).
- Expressions: Expressions are mixtures of symbols, identifiers, operators, and capabilities that consider to a worth. They’re utilized in varied elements of a question, like arithmetic and conditional expressions.
- Subqueries: Subqueries are nested queries inside one other question, permitting for complicated information manipulation and filtering. They can be utilized in clauses like WHERE and FROM.
- Widespread Desk Expressions (CTEs): CTEs outline non permanent outcome units that may be referenced inside the primary question, bettering readability and group.
- Feedback: Feedback clarify SQL code, making it extra comprehensible. They’re ignored by the SQL engine and may be single-line or multi-line.
Key SQL Clauses
- SELECT: Specifies the columns to retrieve.
- FROM: Signifies the desk(s) from which to retrieve the information.
- JOIN: Combines rows from two or extra tables based mostly on a associated column.
- WHERE: Filters data based mostly on specified circumstances.
- GROUP BY: Teams rows which have the identical values in specified columns.
- HAVING: Filters teams based mostly on a situation.
- ORDER BY: Types the outcome set by a number of columns.
Instance
SELECT
staff.identify,
departments.identify,
SUM(wage) as total_salary
FROM
staff
JOIN departments ON staff.dept_id = departments.id
WHERE
staff.standing="lively"
GROUP BY
staff.identify,
departments.identify
HAVING
total_salary > 50000
ORDER BY
total_salary DESC;
This question retrieves the names of staff and their departments, the entire wage of lively staff, and teams the information by worker and division names. It filters for lively staff and orders the outcomes by complete wage in descending order.
Studying Easy SQL Queries
Beginning with easy SQL queries helps construct a stable basis. Deal with figuring out the core parts and understanding their roles.
Instance
SELECT identify, age FROM customers WHERE age > 30;
Steps to Perceive
- Determine the SELECT clause: Specifies the columns to retrieve (identify and age).
- Determine the FROM clause: Signifies the desk (customers).
- Determine the WHERE clause: Units the situation (age > 30).
Clarification
- SELECT: The columns to be retrieved are identify and age.
- FROM: The desk from which the information is retrieved is customers.
- WHERE: The situation is age > 30, so solely customers older than 30 are chosen.
Easy queries usually contain simply these three clauses. They’re simple and straightforward to learn, making them an ideal place to begin for learners.
Intermediate queries usually embrace further clauses like JOIN and GROUP BY. Understanding these queries requires recognizing how tables are mixed and the way information is aggregated.
Instance
SELECT
orders.order_id,
clients.customer_name,
SUM(orders.quantity) as total_amount
FROM
orders
JOIN clients ON orders.customer_id = clients.id
GROUP BY
orders.order_id,
clients.customer_name;
Steps to Perceive
- Determine the SELECT clause: Columns to retrieve (order_id, customer_name, and aggregated
total_amount
). - Determine the FROM clause: Most important desk (orders).
- Determine the JOIN clause: Combines orders and clients tables.
- Determine the GROUP BY clause: Teams the outcomes by order_id and customer_name.
Clarification
- JOIN: Combines rows from the orders and clients tables the place orders.customer_id matches
clients.id
. - GROUP BY: Aggregates information based mostly on order_id and customer_name.
- SUM: Calculates the entire quantity of orders for every group.
Intermediate queries are extra complicated than easy queries and infrequently contain combining information from a number of tables and aggregating information.
Analyzing Superior SQL Queries
Superior queries can contain a number of subqueries, nested SELECT statements, and superior capabilities. Understanding these queries requires breaking them down into manageable elements.
Instance
WITH TotalSales AS (
SELECT
salesperson_id,
SUM(sales_amount) as total_sales
FROM
gross sales
GROUP BY
salesperson_id
)
SELECT
salespeople.identify,
TotalSales.total_sales
FROM
TotalSales
JOIN salespeople ON TotalSales.salesperson_id = salespeople.id
WHERE
TotalSales.total_sales > 100000;
Steps to Perceive
- Determine the CTE (Widespread Desk Expression): TotalSales subquery calculates complete gross sales per salesperson.
- Determine the primary SELECT clause: Retrieves identify and total_sales.
- Determine the JOIN clause: Combines TotalSales with salespeople.
- Determine the WHERE clause: Filters for salespeople with total_sales > 100000.
Clarification
- WITH: Defines a Widespread Desk Expression (CTE) that may be referenced later within the question.
- CTE (TotalSales): Calculates complete gross sales for every salesperson.
- JOIN: Combines the TotalSales CTE with the salespeople desk.
- WHERE: Filters the outcomes to incorporate solely these with total_sales higher than 100,000.
Break down superior queries into a number of steps utilizing subqueries or CTEs to simplify complicated operations.
Writing SQL Queries
Writing SQL queries entails crafting instructions to retrieve and manipulate information from a database. The method begins with defining what information you want after which translating that want into SQL syntax.
Steps to Write SQL Queries
- Outline Your Goal: Decide the information you want and the way you wish to current it.
- Choose the Tables: Determine the tables that include the information.
- Specify the Columns: Determine which columns you wish to retrieve.
- Apply Filters: Use the WHERE clause to filter the information.
- Be part of Tables: Mix information from a number of tables utilizing JOIN clauses.
- Group and Mixture: Use GROUP BY and aggregation capabilities to summarize information.
- Order Outcomes: Use ORDER BY to kind the information in a selected order.
Instance
SELECT
staff.identify,
departments.identify,
COUNT(orders.order_id) as order_count
FROM
staff
JOIN departments ON staff.dept_id = departments.id
LEFT JOIN orders ON staff.id = orders.employee_id
GROUP BY
staff.identify,
departments.identify
ORDER BY
order_count DESC;
This question retrieves worker names, division names, and the variety of orders related to every worker, teams the outcomes by worker and division, and orders the outcomes by the variety of orders in descending order.
Circulation of SQL Queries
Understanding the circulation of SQL question execution is essential for writing environment friendly and efficient queries. The execution follows a selected logical order, also known as the logical question processing phases.
Right here’s the final order during which a SQL question is processed:
- FROM: Specifies the tables from which to retrieve the information. It consists of JOIN operations and any subqueries within the FROM clause.
SELECT *
FROM staff
- WHERE: Filters the rows based mostly on a situation.
SELECT *
FROM staff
WHERE wage > 50000
- GROUP BY: Teams the rows which have the identical values in specified columns into combination information. Mixture capabilities (e.g., COUNT, SUM) are sometimes used right here.
SELECT division, COUNT(*)
FROM staff
WHERE wage > 50000
GROUP BY division
- HAVING: Filters teams based mostly on a situation. It’s much like the WHERE clause however used for teams created by the GROUP BY clause.
SELECT division, COUNT(*)
FROM staff
WHERE wage > 50000
GROUP BY division
HAVING COUNT(*) > 10
- SELECT: Specifies the columns to be retrieved from the tables. It might additionally embrace computed columns.
SELECT division, COUNT(*)
FROM staff
WHERE wage > 50000
GROUP BY division
HAVING COUNT(*) > 10
- DISTINCT: Removes duplicate rows from the outcome set.
SELECT DISTINCT division
FROM staff
- ORDER BY: Types the outcome set based mostly on a number of columns.
SELECT division, COUNT(*)
FROM staff
WHERE wage > 50000
GROUP BY division
HAVING COUNT(*) > 10
ORDER BY COUNT(*) DESC
- LIMIT/OFFSET: Restricts the variety of rows returned by the question and/or skips a specified variety of rows earlier than starting to return rows.
SELECT division, COUNT(*)
FROM staff
WHERE wage > 50000
GROUP BY division
HAVING COUNT(*) > 10
ORDER BY COUNT(*) DESC
LIMIT 5
OFFSET 10
By understanding this order, you’ll be able to construction your queries appropriately to make sure they return the specified outcomes.
Debugging SQL Queries
Debugging SQL queries entails figuring out and resolving errors or efficiency points. Widespread strategies embrace checking for syntax errors, verifying information sorts, and optimizing question efficiency.
Instance
SELECT identify, age FROM customers WHERE age="thirty";
Steps to Debug
- Examine for syntax errors: Guarantee all clauses are appropriately written.
- Confirm information sorts: Right the situation to make use of the suitable information kind (age = 30).
Clarification
- Syntax Errors: Search for lacking commas, incorrect key phrases, or mismatched parentheses.
- Knowledge Sorts: Guarantee circumstances use the right information sorts (e.g., evaluating numeric values with numeric values).
Debugging usually requires cautious examination of the question and its logic, making certain every half capabilities as anticipated.
Superior Ideas for Mastering SQL
Allow us to now look into some superior suggestions for mastering SQL.
Use Subqueries Properly
It is because the usage of subqueries may help within the simplification of the question for the reason that extra difficult elements of the question may be achieved in sections. Nonetheless, when they’re applied in a lot of occurrences, issues can come up regarding efficiency. Make use of them properly with a view to enhance readability whereas ensuring that they won’t an excessive amount of of a pressure relating to efficiency points.
Indexing for Efficiency
Indexes improve question efficiency by lowering the quantity of knowledge learn. Study when to create indexes, the best way to do it, and when to drop them. Pre-schedule audits to measure efficiency good points from indexes.
Optimize Joins
Joins are highly effective however may be pricey by way of efficiency. Use INNER JOINs if you want rows which have matching values in each tables. Use LEFT JOINs sparingly and solely when needed.
Perceive Execution Plans
Execution plans provide data pertaining to how the SQL engine processes an announcement. Use the services like EXPLAIN in MySQL or EXPLAIN PLAN in Oracle to determine the efficiency issues associated to the queries you might be utilizing.
Common Follow
As some other ability, it requires observe and the extra you observe the higher you change into at it so far as SQL is worried. Clear up precise issues, interact in on-line instances, and all the time attempt to replace your information and efficiency.
Conclusion
Each information skilled ought to know the best way to learn and particularly the best way to write SQL queries as these are highly effective instruments for information evaluation. Following the outlined pointers on this information, you can be in a greater place to grasp and analyze SQL queries, a lot as offered in equation. The extra you observe, the higher you get and utilizing SQL will change into second nature to you and an everyday a part of your work.
Regularly Requested Questions
A. The fundamental parts embrace SELECT, FROM, JOIN, WHERE, GROUP BY, HAVING, and ORDER BY clauses.
A. Break down the question into smaller elements, perceive every clause, and observe the information circulation from subqueries to the primary question.
A. Examine for syntax errors, confirm information sorts, and use debugging instruments to determine and resolve points.
A. Optimize your queries by indexing, avoiding pointless subqueries, and utilizing environment friendly be a part of operations.
A. On-line platforms like LeetCode, HackerRank, and SQLZoo provide observe issues to enhance your SQL expertise.