Introduction
Suppose you’re a developer anticipated to hold out testing on a big net software. It’s unattainable to undergo every function and all of the interactions one after the other which can take days and even weeks. Enter Selenium, the sport altering device which automates net browser interplay and thus is extra environment friendly with regards to testing. As urged within the title of the information we’re about to have a look at, Selenium and Python are a robust workforce with regards to net automation. A lot of its content material is centered on problem fixing and by the top you ought to be prepared figuring out your atmosphere setup, efficient scripting of assessments in addition to frequent net take a look at points altering the best way you observe net automation and testing.
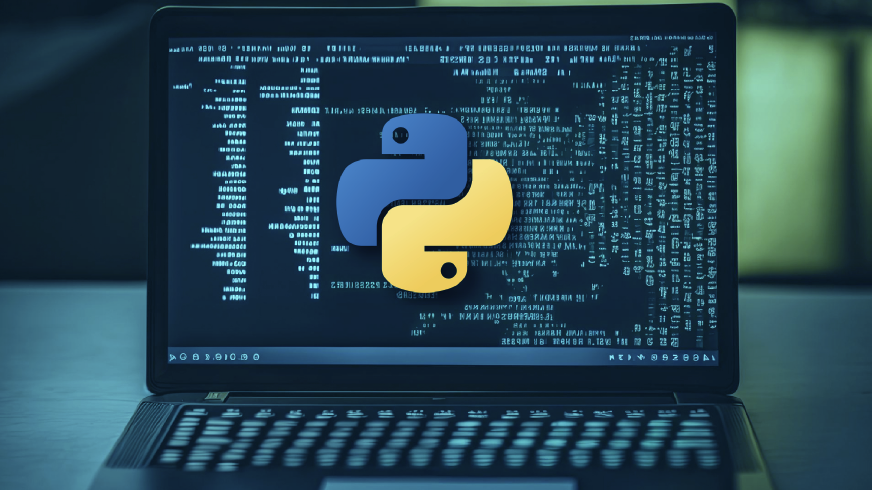
Studying Outcomes
- Grasp the fundamentals of Selenium and its integration with Python.
- Arrange a Python atmosphere for Selenium and set up obligatory packages.
- Write, run, and debug Selenium take a look at scripts for net functions.
- Perceive superior Selenium options, together with dealing with dynamic content material and interacting with net components.
- Troubleshoot frequent points encountered in net automation with sensible options.
Why Study Selenium Python?
Selenium mixed with Python affords a robust toolkit for net automation. Right here’s why it’s value studying:
- Ease of Use: Python is a perfect language to make use of when writing take a look at scripts as is made simple subsequently easing the automation of duties.
- Extensively Supported: Selenium helps completely different browsers in addition to completely different working methods.
- Strong Neighborhood: A big neighborhood and large documentation assure that you could all the time discover the help and supplies to repair points and research extra.
- Enhanced Testing Effectivity: Selenium helps automate assessments which cuts down on guide work, testing takes lesser time and it’s extremely correct.
Pre-requisite to Study Selenium Python Tutorial
Earlier than diving into Selenium with Python, it’s essential to have a foundational understanding of each Python programming and net applied sciences. Right here’s what it is best to know:
- Fundamental Python Information: The fundamental information of Python syntax, features, and Object-oriented precept will go a good distance in writing and decoding Selenium scripts.
- HTML/CSS Fundamentals: Attributable to HTML and CSS information one can work together with the Net components and search them efficiently.
- Fundamental Net Ideas: Understanding of how web-pages operate, kind submission, buttons, hyperlinks, and many others. will assist with automating browser performance.
Getting Began with Selenium and Python
Selenium might be described as a way of automating net browsers the place you may create scripts of which might carry out features just like a human. Python is simple to study and extremely simple to learn making it very appropriate to make use of whereas scripting with Selenium. Initially Selenium must be put in, along with a WebDriver for the specified browser.
Putting in Selenium
Start by putting in the Selenium package deal by way of pip:
pip set up selenium
Setting Up WebDriver
Selenium requires a WebDriver for the browser you plan to automate. For Chrome, you’ll use ChromeDriver, whereas for Firefox, it’s GeckoDriver. Obtain the suitable driver and guarantee it’s in your system’s PATH or specify its location in your script.
For different browsers, they’ve their very own supported net drivers. A few of them are –
Writing Your First Selenium Script
As soon as the set up is full, one is able to write his or her first script. Right here’s a easy instance of a Selenium script in Python that opens a webpage and interacts with it:
from selenium import webdriver
# Initialize the Chrome driver
driver = webdriver.Chrome()
# Open a web site
driver.get('https://www.instance.com')
# Discover a component by its title and ship some textual content
search_box = driver.find_element_by_name('q')
search_box.send_keys('Selenium with Python')
# Submit the shape
search_box.submit()
# Shut the browser
driver.stop()
Superior Selenium Options
As you change into extra conversant in Selenium, you’ll encounter extra superior options:
- Dealing with Dynamic Content material: Use WebDriverWait to deal with components that take time to load.
from selenium.webdriver.frequent.by import By
from selenium.webdriver.help.ui import WebDriverWait
from selenium.webdriver.help import expected_conditions as EC
wait = WebDriverWait(driver, 10)
ingredient = wait.till(EC.presence_of_element_located((By.ID, 'dynamic-element')))
- Interacting with Net Parts: Learn to deal with several types of components like dropdowns, checkboxes, and alerts.
# Dealing with a dropdown
from selenium.webdriver.help.ui import Choose
dropdown = Choose(driver.find_element_by_id('dropdown'))
dropdown.select_by_visible_text('Choice 1')
Varied Strategies One Can Use in Selenium Python
Selenium WebDriver is a robust device for automating web-based functions. It gives a set of strategies to work together with net components, management browser habits, and deal with varied web-related duties.
Selenium Strategies for Browser Administration
Technique | Description |
---|---|
get(url) | Navigates to the required URL. |
getTitle() | Returns the title of the present web page. |
getCurrentUrl() | Returns the present URL of the web page. |
getPageSource() | Returns the supply code of the present web page. |
shut() | Closes the present browser window. |
stop() | Quits the WebDriver occasion and closes all browser home windows. |
getWindowHandle() | Returns the deal with of the present window. |
getWindowHandles() | Returns a set of handles of all open home windows. |
Selenium Strategies for Net Parts
Selenium gives a variety of strategies to work together with net components. A few of the generally used strategies embody:
Technique | Description |
---|---|
find_element_by_id |
Locates a component by its distinctive id attribute. Supreme for components with distinctive identifiers, making certain fast and exact entry. |
find_element_by_name |
Locates a component by its title attribute. Helpful for kind components or fields with distinct names. |
find_element_by_class_name |
Locates a component by its CSS class title. Greatest for focusing on components that share a typical class. |
find_element_by_tag_name |
Locates a component by its HTML tag. Efficient for normal searches throughout tags like <enter> , <div> , and many others. |
find_element_by_link_text |
Locates a hyperlink ingredient by its full seen textual content. Helpful for locating and interacting with particular hyperlinks. |
find_element_by_partial_link_text |
Locates a hyperlink ingredient by a partial match of its seen textual content. Useful for locating hyperlinks with dynamic or variable textual content. |
find_element_by_xpath |
Locates a component utilizing XPath expressions. Provides exact management and suppleness for advanced queries and hierarchical searches. |
find_element_by_css_selector |
Locates a component utilizing CSS selectors. Gives highly effective choice capabilities based mostly on CSS guidelines and attributes. |
What’s Selenium Used For in Python Programming?
Selenium is primarily used for automating net browser interactions and testing net functions. In Python programming, Selenium might be employed for:
- Net Scraping: Extracting knowledge from net pages.
- Automated Testing: Working take a look at circumstances to confirm that net functions behave as anticipated.
- Kind Filling: Automating repetitive knowledge entry duties.
- Interplay Simulation: Mimicking consumer actions like clicking, scrolling, and navigating.
Greatest Practices to Comply with When Utilizing Selenium in Python
To make sure environment friendly and efficient Selenium automation, adhere to those greatest practices:
- Use Specific Waits: As an alternative of hardcoding delays, use WebDriverWait to attend for particular situations.
- Keep away from Hardcoding Knowledge: Use configuration recordsdata or atmosphere variables to handle take a look at knowledge and settings.
- Set up Take a look at Circumstances: Construction your take a look at circumstances utilizing frameworks like pytest or unittest for higher readability and upkeep.
- Deal with Exceptions: Implement error dealing with to handle surprising conditions and guarantee scripts don’t fail abruptly.
- Maintain WebDriver Up to date: Guarantee your WebDriver model is appropriate together with your browser model to keep away from compatibility points.
Troubleshooting Frequent Points
Whereas working with Selenium, you may run into points. Listed here are some frequent issues and options:
- ElementNotFoundException: Be certain that the ingredient is current on the web page and that you just’re utilizing the proper selector.
- TimeoutException: Improve the wait time in WebDriverWait or verify if the web page is loading accurately.
- WebDriver Model Mismatch: Be certain that the WebDriver model matches your browser model.
Conclusion
Selenium when used with Python is definitely fairly a potent package deal that may drastically pace up and improve net testing and automation. Mastering even the essential in addition to the varied options of Selenium will allow the developer to cut back time and automate assessments, do in-depth testing. By the information you’ve got gained from this information, now you can be assured to deal with completely different net automation duties.
Regularly Requested Questions
A. Selenium is an open-source device for automating net browsers, permitting you to jot down scripts that may carry out duties and take a look at net functions robotically.
A. Set up Selenium utilizing pip with the command pip set up selenium
.
A. A WebDriver is a device that permits Selenium to manage an online browser by interacting with it programmatically. Examples embody ChromeDriver for Chrome and GeckoDriver for Firefox.
A. Use WebDriverWait to attend for components to look or change states earlier than interacting with them.
A. Obtain the WebDriver model that matches your browser’s model or replace your browser to match the WebDriver model.