Introduction
We’ve got been discussing Python and its versatility. Now could be the time to grasp one other performance of this highly effective programming language: it enhances code effectivity and readability. Sustaining the modularity of your code logic whereas engaged on a production-level program is necessary.
Python Operate definition permits the builders to realize this by encapsulating the codes. Alternatively, lambda features present a compact strategy to outline easy features in Python.
On this information, we are going to discover the syntaxes, usages, and finest practices for each varieties of Python features to construct a stable basis for leveraging these instruments in your Python initiatives within the trade. Whether or not you need to break complicated duties into easier features or make the most of lambda features for concise operations, these strategies will assist you to write environment friendly code.
To refresh your Python primary to advance, undergo these –
- Complete Information to Superior Python Programming- Hyperlink
- Complete Information to Python Constructed-in Knowledge Buildings – Hyperlink
- Fundamentals of Python Programming for Rookies- Hyperlink
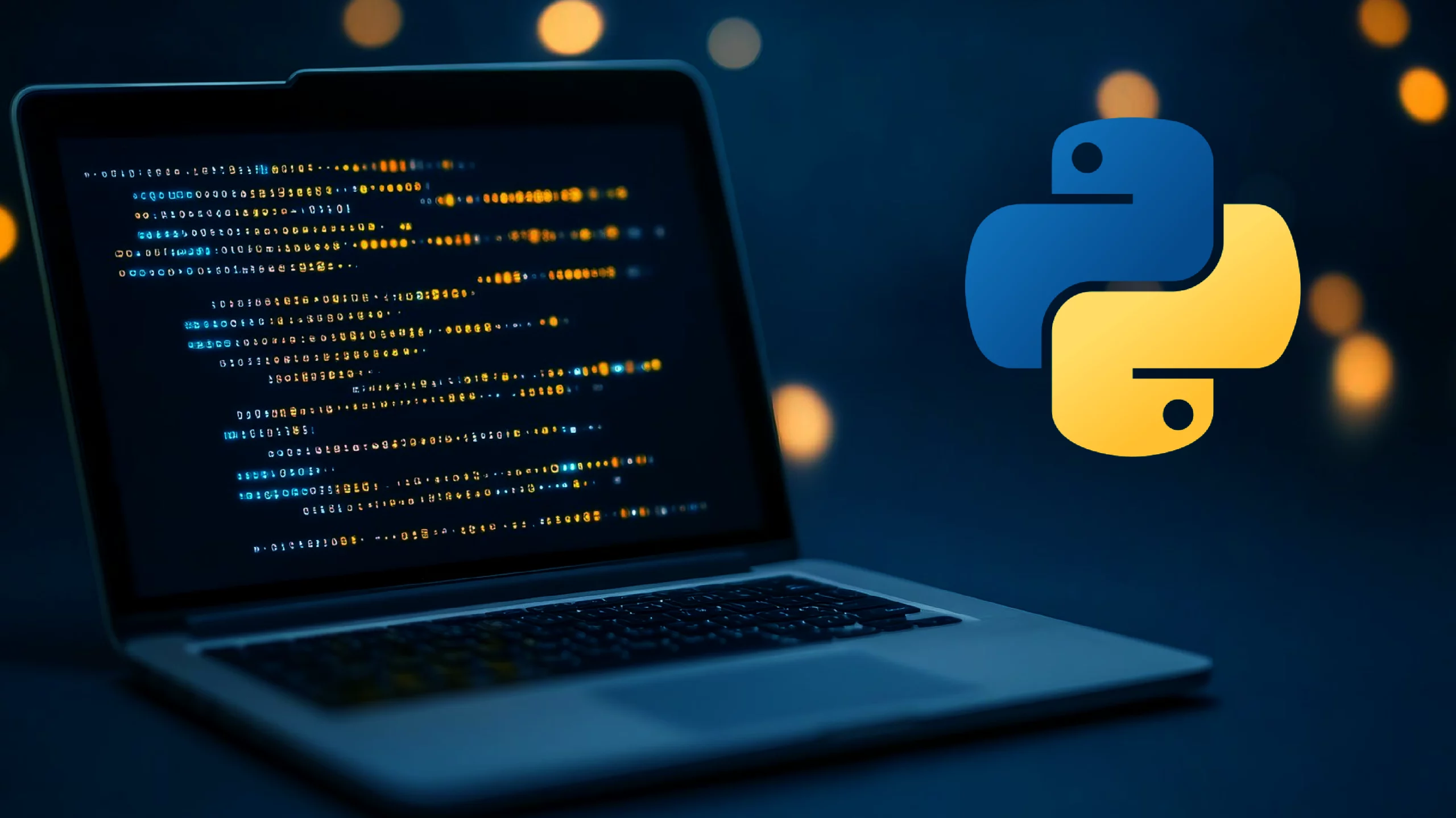
What’s a Operate?
A operate in Python is a reusable block of code that performs a selected activity relying on this system’s logic. They’ll take inputs (often called parameters or arguments), carry out sure operations, and return outputs.
Capabilities are actually useful in organizing code, making it extra readable, maintainable, and environment friendly in manufacturing.
Python Operate makes use of two Most Vital Rules:
- Abstraction: This precept makes the operate cover the small print of the complicated implementation whereas displaying solely the important options (i.e., no matter is returned as output).
- Decomposition: This precept entails breaking down an enormous activity into smaller, extra manageable operate blocks to keep away from redundancy and facilitate simpler debugging.
Syntax:
The operate syntax entails two issues:
On this half, you’ll write a logic, together with a docstring, utilizing the `def` key phrase.
def function_name(paramters):
"""
doc-string
"""
operate logic (physique)
return output
The above operate doesn’t return any output by itself. To print the output on the display, you’ll want to name the operate utilizing this syntax.
function_name(arguments)
Let’s discover an instance of how you can create a operate.
Creating Operate
Now, let’s create our first operate, together with a docstring.
# Operate physique
def is_even(num:int):
"""
Test if a quantity is even or odd.
Parameters:
num (int): The quantity to test.
Returns:
str: "even" for the even quantity and, "odd" if the quantity is odd.
"""
# Operate logic
if kind(num) == int:
if num % 2 == 0:
return "even"
else:
return "odd"
else:
return "Operate wants an integer aruguement"
# Calling operate
for i in vary(1,11):
print(i, "is", is_even(i))
Output
1 is odd2 is even
3 is odd
4 is even
5 is odd
6 is even
7 is odd
8 is even
9 is odd
10 is even
How do you run documentation?
You should utilize `.__doc__` to entry the docstring of your operate (or any built-in operate, which we’ve mentioned right here).
print(is_even.__doc__)
Output
Test if a quantity is even or odd.Parameters:
num (int): The quantity to test.
Returns:
str: "even" for the even quantity and, "odd" if the quantity is odd.
To Observe:
Programmers usually confuse the parameter/s and the argument/s and use them interchangeably whereas talking. However let’s perceive the distinction between them so that you just by no means get into this dilemma.
- Parameter: A parameter is a variable named within the operate or methodology definition (in `class`). It acts as a placeholder for the information the operate will use sooner or later.
- Argument: The precise worth is handed to the operate or methodology when it’s referred to as to return the output in accordance with the operate logic.
Kinds of Arguments in Python
Because of their versatility, Python features can settle for various kinds of arguments, offering flexibility in how you can name them.
The principle varieties of arguments are:
- Default Arguments
- Positional Arguments
- Key phrase Arguments
- Arbitrary Positional Arguments (*args)
- Arbitrary Key phrase Arguments (**kwargs)
Let’s perceive them one after the other:
1. Default Arguments
- Arguments that assume a default worth whereas writing the operate, if a worth isn’t offered in the course of the operate name.
- Helpful for offering elective parameters when person doesn’t enter the worth.
def greet(identify, message="Good day"):
return f"{message}, {identify}!"
print(greet("Nikita"))
print(greet("Nikita", "Hello"))
Outputs
Good day, Nikita!
Hello, Nikita!
2. Positional Arguments
- Arguments handed to a operate in a selected order are referred to as positional arguments.
- The order wherein the arguments are handed issues, or else it could return the unsuitable output or error.
def add(a, b):
return a + b
print(add(2, 3))
Output
Outputs: 5
3. Key phrase Arguments
- Arguments which might be handed to a operate utilizing the parameter identify as a reference are often called Key phrase Arguments.
- The order doesn’t matter herein, as every argument is assigned to the corresponding parameter.
def greet(identify, message):
return f"{message}, {identify}!"
print(greet(message="Good day", identify="Nikita"))
Output
Outputs: Good day, Nikita!
4. Variable-Size Arguments
`*args` and `**kwargs` are particular python key phrases which might be used to cross the variable size of arguments to operate.
- Arbitrary Positional Arguments (*args): This permits a operate to just accept any variety of non-keyword positional arguments.
def sum_all(*args):
print(kind(args), args)
return sum(args)
print(sum_all(1, 2, 3, 4))
Output
<class 'tuple'> (1, 2, 3, 4)
# 10
- Arbitrary Key phrase Arguments (**kwargs): This permits a operate to just accept any variety of key phrase arguments.
def print_details(**kwargs):
for key, worth in kwargs.gadgets():
print(f"{key}: {worth}")
print_details(identify="Nikita", age=20)
Output
identify: Alice
age: 30
Observe: Key phrase arguments imply that they include a key-value pair, like a Python dictionary.
Level to recollect
The order of the arguments issues whereas writing a operate to get the right output:
def function_name(parameter_name, *args, **kwargs):
"""
Logic
"""
Kinds of Capabilities in Python
There are a number of varieties of features Python presents the builders, akin to:
Operate Kind | Description | Instance |
---|---|---|
Constructed-in Capabilities | Predefined features accessible in Python. | print(), len(), kind() |
Consumer-Outlined Capabilities | Capabilities created by the person to carry out particular duties. | def greet(identify): |
Lambda Capabilities | Small, nameless features with a single expression. | lambda x, y: x + y |
Recursive Capabilities | Capabilities that decision themselves to unravel an issue. | def factorial(n): |
Greater-Order Capabilities | Capabilities that take different features as arguments or return them. | map(), filter(), cut back() |
Generator Capabilities | Capabilities that return a sequence of values separately utilizing yield. | def count_up_to(max): |
Capabilities in Python are the first Class Citizen
I do know it is a very heavy assertion for those who’ve by no means heard it earlier than, however let’s focus on it.
Capabilities in Python are entities that help all of the operations usually accessible to different objects, akin to lists, tuples, and many others.
Being first-class residents means features in Python can:
- Be assigned to variables.
- Be handed as arguments to different features.
- Be returned from different features.
- Be saved in information buildings.
This flexibility permits for highly effective and dynamic programming.
kind() and id() of Operate
By now, chances are you’ll be excited to know in regards to the operate’s kind() and id(). So, let’s code it to grasp higher:
def sum(num1, num2):
return num1 + num2
print(kind(sum))
print(id(sum))
Output
<class 'operate'>134474514428928
Like different objects, this operate additionally has a category of features and an ID tackle the place it’s saved in reminiscence.
Reassign Operate to the Variable
You may also assign a operate to a variable, permitting you to name the operate utilizing that variable.
x = sum
print(id(x))
x(3,9)
Output
13447451442892812
Observe: x could have the similar tackle as sum.
Capabilities Can Additionally Be Saved within the Knowledge Buildings
You may also retailer features in information buildings like lists, dictionaries, and many others., enabling dynamic operate dispatch.
l1 = [sum, print, type]
l1[0](2,3)
# Calling operate inside an inventory
Output
5
Capabilities are Immutable information sorts
Let’s retailer a operate `sum` in a set to show this. As set won’t ever enable mutable datatypes.
s = {sum}
s
Output
{<operate __main__.sum(num1, num2)>}
Since we received an output, this confirms that the set is the immutable information sorts.
Capabilities Can Additionally Be Handed as Arguments to Different Capabilities
You may also cross features as arguments to different features, enabling higher-order features and callbacks.
def shout(textual content):
return textual content.higher()
def whisper(textual content):
return textual content.decrease()
def greet(func, identify):
return func(f"Good day, {identify}!")
print(greet(shout, "Nikita")) # Outputs: HELLO, NIKITA!
print(greet(whisper, "Nikita")) # Outputs: howdy, nikita
Output
HELLO, NIKITA!
howdy, nikita
We’ll cowl higher-order features intimately later on this article. So, keep tuned till the top!
Capabilities Can Additionally Be Returned from Different Capabilities
A operate may return different features, permitting the creation of a number of features or decorators.
def create_multiplier(n):
def multiplier(x):
return x * n
return multiplier
double = create_multiplier(2)
print(double(5)) # Outputs: 10
triple = create_multiplier(3)
print(triple(5)) # Outputs: 15
Outputs
10
15
Benefits of utilizing Capabilities
Python features provide 3 main benefits, akin to
- Code Modularity: Capabilities mean you can encapsulate the logic inside named blocks, breaking down complicated issues into smaller, extra organized items.
- Code Readability: Capabilities make code a lot cleaner and simpler for others (or your self) to grasp whereas reviewing and debugging it sooner or later.
- Code Reusability: As soon as the logic is created, it may be referred to as a number of instances in a program, lowering code redundancy.
Additionally learn: What are Capabilities in Python and How you can Create Them?
What’s a Lambda Operate?
A lambda operate, additionally referred to as an inline operate is a small nameless operate. It will possibly take any variety of arguments, however can solely have one-line expression. These features are significantly helpful for a brief interval.
Syntax:
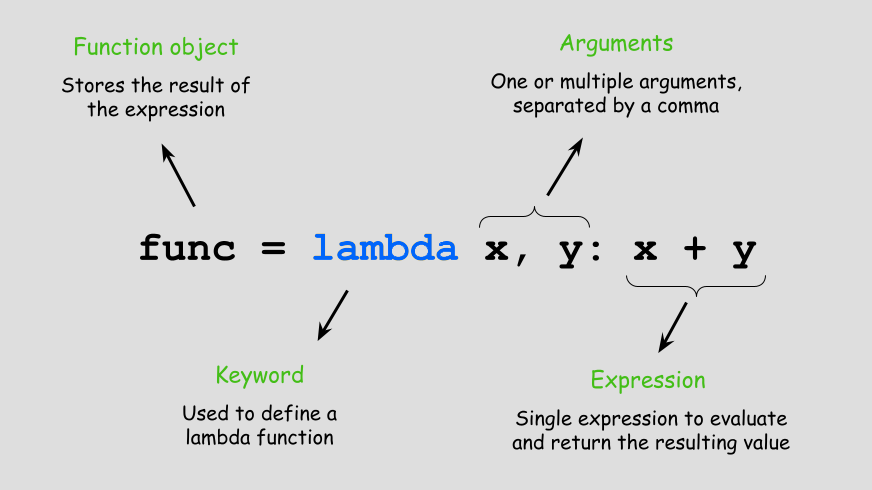
Let’s test some examples:
1. Lambda Operate with one variable
# sq. a worth
func = lambda x : x**2
func(5)
Output
25
2. Lambda Operate with two variables
# Subtracting a worth
func = lambda x=0, y=0: x-y
func(5)
Output
5
3. Lambda Operate with `if-else` assertion
# Odd or Even
func = lambda x : "even" if xpercent2==0 else "odd"
func(1418236418)
Output
'even'
Lambda features vs. Regular features
Function | Lambda Operate | Regular Operate |
---|---|---|
Definition Syntax | Outlined utilizing the lambda key phrase | Outlined utilizing the def key phrase |
Syntax Instance | lambda x, y: x + y | def add(x, y):n return x + y |
Operate Identify | Nameless (no identify) | Named operate |
Use Case | Quick, easy features | Complicated features |
Return Assertion | Implicit return (single expression) | Specific return |
Readability | Much less readable for complicated logic | Extra readable |
Scoping | Restricted to a single expression | Can include a number of statements |
Decorators | Can’t be adorned | Could be adorned |
Docstrings | Can not include docstrings | Can include docstrings |
Code Reusability | Sometimes used for brief, throwaway features | Reusable and maintainable code blocks |
Why use the Lambda Operate?
Lambda features don’t exist independently. The perfect strategy to utilizing them is with higher-order features (HOF) like map, filter, and cut back.
Whereas these features have a restricted scope in comparison with common features, they will provide a succinct strategy to streamline your code, particularly in sorting operations.
Additionally learn: 15 Python Constructed-in Capabilities which You Ought to Know whereas studying Knowledge Science
What are Greater Order Capabilities(HOF) in Python?
The next-order operate, generally often called an HOF, can settle for different features as arguments, return features, or each.
As an example, that is how you need to use a HOF:
# HOF
def remodel(lambda_func, list_of_elements):
output = []
for i in L:
output.append(f(i))
print(output)
L = [1, 2, 3, 4, 5]
# Calling operate
remodel(lambda x: x**2, L)
Output
[1, 4, 9, 16, 25]
The principle operate on this code snippet is to take a lambda operate and an inventory of parts.
Observe: As per the issue assertion, you’ll be able to apply any particular logic utilizing this lambda operate.
Now, let’s dive deep into the Kinds of HOFs.
What are 3 HOF in Python?
Listed here are 3 HOF in Python:
1. map()
It applies a given operate to every merchandise of an iterable (e.g., checklist, dictionary, tuple) and returns an inventory of the outcomes.
As an example,
# Fetch names from an inventory of dict
folks = [
{"name": "Alice", "age": 25},
{"name": "Bob", "age": 30},
{"name": "Charlie", "age": 35},
{"name": "David", "age": 40}
]
checklist(map(lambda particular person: particular person["name"], folks))
Output
['Alice', 'Bob', 'Charlie', 'David']
2. filter()
It creates an inventory of parts for which a given operate returns `True`, much like any filter operation in numerous programming languages.
As an example,
# filter: fetch names of individuals older than 30
filtered_names = filter(lambda particular person: particular person["age"] > 30, folks)
filtered_names_list = map(lambda particular person: particular person["name"], filtered_names)
print(checklist(filtered_names_list))
Output
['Charlie', 'David']
3. cut back()
It applies a operate cumulatively to the gadgets of an iterable, lowering it to a single worth.
As an example,
# cut back: concatenate all names right into a single string
concatenated_names = cut back(lambda x, y: x + ", " + y, map(lambda particular person: particular person["name"], folks))
print(concatenated_names)
Output
Alice, Bob, Charlie, David
Observe: All of those features anticipate a lambda operate and an iterable.
Conclusion
To conclude this text on Python Capabilities Definition and Lambda Capabilities, I might say that for those who purpose to write down strong and scalable code, it’s actually necessary to grasp each of those functionalities to work in real-life industries.
Moreover, this follow helps in writing cleaner code and enhances collaboration all through the group, as different programmers can simply perceive and use the predefined features to scale back redundancy.
Continuously Requested Questions
Ans. Operate definitions, also known as regular features in Python, enable programmers to encapsulate code into reusable blocks to advertise modularity, improve readability, and make it simpler to debug.
Ans. Lambda features, also known as nameless or inline features, present a compact strategy to outline easy features as wanted for a brief interval, akin to in sorting operations or inside higher-order features like map(), filter(), and cut back().
Ans. Right here’s the distinction:
`map()`: Applies a given operate to every merchandise of an iterable and returns an inventory of the outcomes.
`filter()`: creates an inventory of parts for which a given operate returns `True`.
`cut back()`: Applies a operate cumulatively to the gadgets of an iterable, lowering it to a single worth.