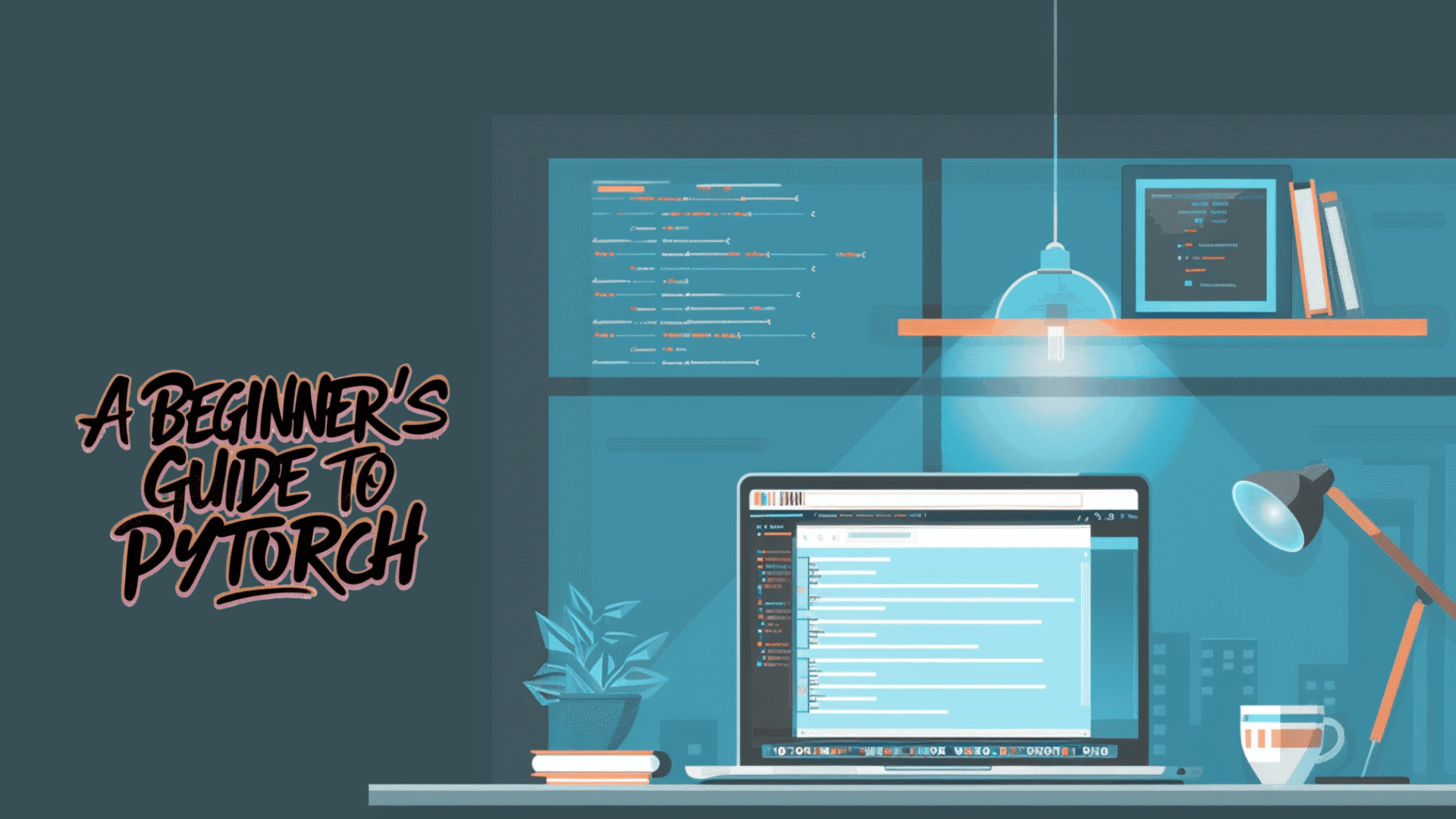
Picture by Editor | Midjourney & Canva
Deep Studying is broadly utilized in many areas of Synthetic Intelligence analysis and has contributed to technological developments. For instance, textual content technology, facial recognition, and voice synthesis purposes are based mostly on deep studying analysis.
One of the used Deep Studying packages is PyTorch. It’s an open-source package deal created by Meta AI in 2016 and has since been utilized by many.
There are lots of PyTorch benefits, together with:
- Versatile mannequin structure
- Native Help for CUDA (Can use GPU)
- Python-based
- Offering lower-level controls, that are helpful for analysis and plenty of use circumstances
- Lively improvement by the developer and neighborhood
Let’s discover PyTorch with this text that will help you get began.
Preparation
It is best to go to their set up webpage and choose the one which fits your surroundings’s necessities. The beneath code is the set up instance.
pip3 set up torch torchvision torchaudio --index-url https://obtain.pytorch.org/whl/cpu
With the PyTorch prepared, let’s get into the central half.
PyTorch Tensor
Tensor is the constructing block in PyTorch. It’s just like the NumPy array however has entry to a GPU. We are able to attempt to create a PyTorch Tensor utilizing the next code:
a = torch.tensor([2, 4, 5])
print(a)
Output>>
tensor([2, 4, 5])
Just like the NumPy array Tensor, it permits matrix operations.
e = torch.tensor([[1, 2, 3],
[4, 5, 6]])
f = torch.tensor([7, 8, 9])
print(e * f)
Output>>
tensor([[ 7, 16, 27],
[28, 40, 54]])
It’s additionally attainable to carry out the matrix multiplication.
g = torch.randn(2, 3)
h = torch.randn(3, 2)
print( g @ h)
Output>>
tensor([[-0.8357, 0.0583],
[-2.7121, 2.1980]])
We are able to entry the Tensor info through the use of the code beneath.
x = torch.rand(3,4)
print("Form:", x.form)
print("Information kind:", x.dtype)
print("Machine:", x.gadget)
Output>>
Form: torch.Measurement([3, 4])
Information kind: torch.float32
Machine: cpu
Neural Community Coaching with PyTorch
By defining the NN utilizing the nn.Module class, we will develop a easy mannequin. Let’s strive it out with the code beneath.
import torch
class SimpleNet(nn.Module):
def __init__(self, enter, hidden, output):
tremendous(SimpleNet, self).__init__()
self.fc1 = torch.nn.Linear(enter, hidden)
self.fc2 = torch.nn.Linear(hidden, output)
def ahead(self, x):
x = torch.nn.useful.relu(self.fc1(x))
x = self.fc2(x)
return x
inp = 10
hid = 10
outp = 2
mannequin = SimpleNet(inp, hid, out)
print(mannequin)
Output>>
SimpleNet(
(fc1): Linear(in_features=10, out_features=10, bias=True)
(fc2): Linear(in_features=10, out_features=2, bias=True)
)
The above code defines a SimpleNet
class that inherits from nn.Module
, which units up the layers. We use nn.Linear
is for the layers, and relu
because the activation operate.
We are able to add extra layers or use totally different layers like Conv2D or CNN. However we’d not use that.
Subsequent, we’d prepare the SimpleNet
we developed with pattern Tensor information.
import torch
inp = torch.randn(100, 10)
tar = torch.randint(0, 2, (100,))
criterion = torch.nn.CrossEntropyLoss()
optimizr = torch.optim.SGD(mannequin.parameters(), lr=0.01)
epochs = 100
batchsize = 10
for epoch in vary(numepochs):
mannequin.prepare()
for i in vary(0, inp.measurement(0), batchsize):
batch_inp = inputs[i:i+batch_size]
batch_tar = targets[i:i+batch_size]
out = mannequin(batch_inp)
loss = criterion(out, batch_tar)
optimizer.zero_grad()
loss.backward()
optimizr.step()
if (epoch + 1) % 10 == 0:
print(f'Epoch [{epoch+1}/{num_epochs}], Loss: {spherical(loss.merchandise(),4})')
Throughout the coaching above, we use random Tensor information and provoke the loss operate referred to as CrossEntropyLoss
. Additionally, we provoke the SGD optimizer to handle the mannequin parameters to attenuate the loss.
The coaching course of runs a number of instances in response to the epoch numbers after which performs the optimization course of. That is the standard deep-learning course of.
We are able to add a number of steps to extra complicated coaching to enhance coaching, like early stopping, studying fee, and different methods.
Lastly, we will consider the mannequin we’ve educated with the unseen information. The next code permits us to try this.
from sklearn.metrics import classification_report
mannequin.eval()
test_inputs = torch.randn(20, 10)
test_targets = torch.randint(0, 2, (20,))
with torch.no_grad():
test_outputs = mannequin(test_inputs)
_, predicted = torch.max(test_outputs, 1)
print(classification_report(test_targets, predicted))
What occurred above is that we switched the mannequin into the analysis mode, which turned off dropout and batch normalization updates. Moreover, we disable the gradient computation course of to hurry up the method.
You may go to the PyTorch documentation to be taught additional about what you are able to do.
Conclusion
On this article, we are going to undergo the fundamentals of PyTorch. From tensor creation to tensor operations and creating a easy NN mannequin. The article is an introductory stage that each newbie ought to be capable to comply with shortly.
Cornellius Yudha Wijaya is a knowledge science assistant supervisor and information author. Whereas working full-time at Allianz Indonesia, he likes to share Python and information ideas by way of social media and writing media. Cornellius writes on a wide range of AI and machine studying subjects.