Introduction
By no means would have the inventor of e mail –Ray Tomlinson– considered how far this piece of tech would attain sooner or later. As we speak, e mail is the prime pillar of company {and professional} communications and is utilized in innumerable sides of the working world. And this has propelled the creation of an entire set of instruments and plugins to optimise your e mail inbox! With the appearance of generative AI, the newest discuss on the town is in regards to the widespread use of AI Brokers to optimise emails. On this weblog, I’ll clarify the craft of automating e mail sorting and labelling by constructing brokers utilizing crewAI.
Overview
- Discover ways to give your purposes entry to your Gmail utilizing Google Cloud Console’s OAuth 2.0
- Perceive the code and construct an agent that makes use of an LLM to learn and categorise mails into pre-defined classes.
- Discover ways to automate the method of e mail sorting and labelling utilizing crewAI by merely working a Python script.
Understanding the Context
In case you are not dwelling underneath a rock and have an e mail deal with, you know the way flooded your inbox tends to get after an evening’s sleep. Advertising and marketing emails, private messages, skilled communications, and so forth, particularly if you’re a busy working skilled. The frustration of a cluttered inbox will be felt within the picture beneath:
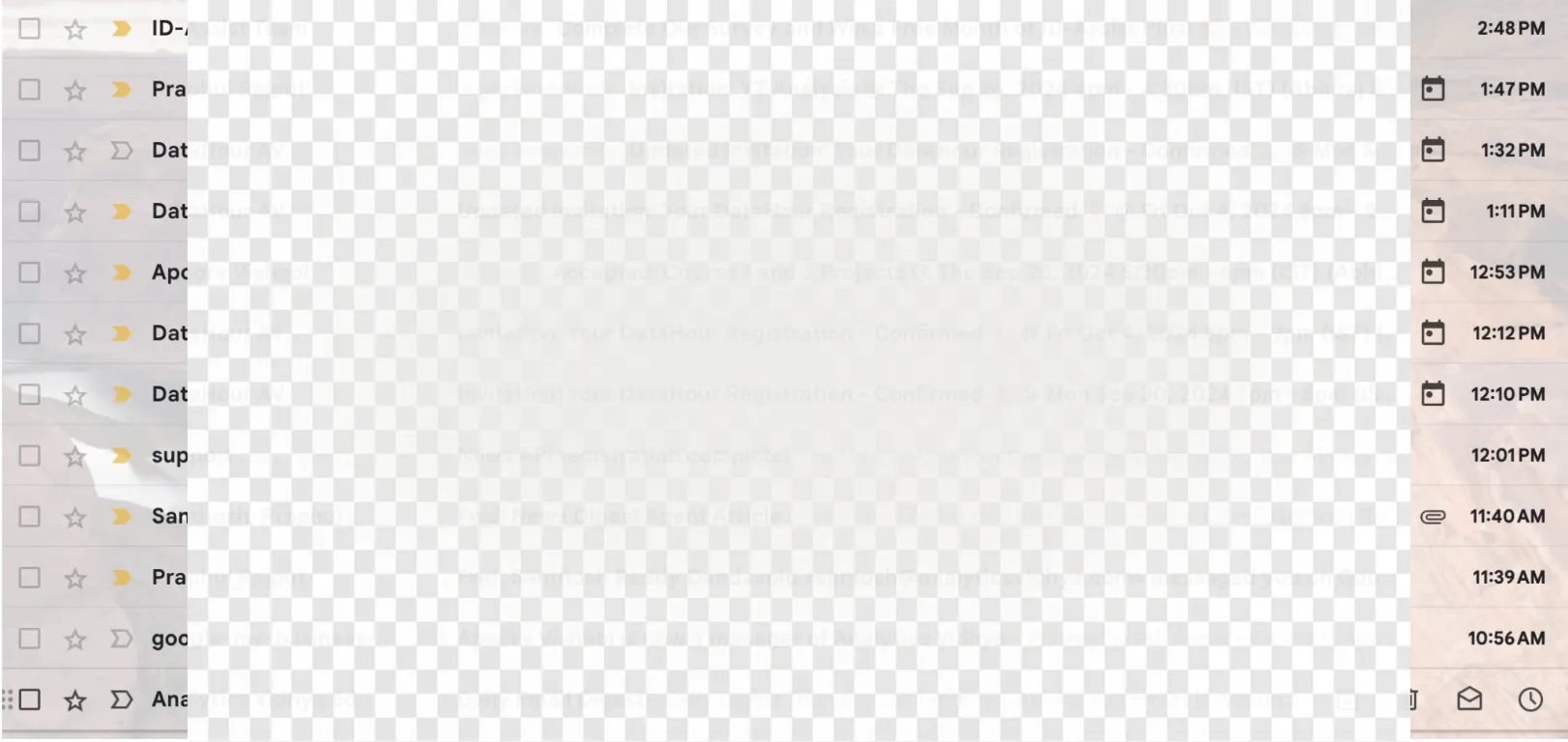
The worst half is that even after creating related labels within the e mail (Gmail within the case of the creator), one has to take the time to assign these emails to the proper label. With the developments in generative AI, shouldn’t it’s a lot simpler to type and label emails in Gmail? Why don’t we scale back the steps of sorting related emails to a single click on?
Let me illustrate how we are able to use crewAI to construct an LLM agent and automate this course of. The top aim is mechanically sorting our unread emails utilizing crewAI into three classes: ‘Reply Instantly’, ‘No Reply’, and ‘Irrelevant’. We then add these categorised emails to Gmail and the respective labels.
Observe: We manually created these labels – ‘Reply Instantly’, ‘No Reply’, and ‘Irrelevant’ – in Gmail.
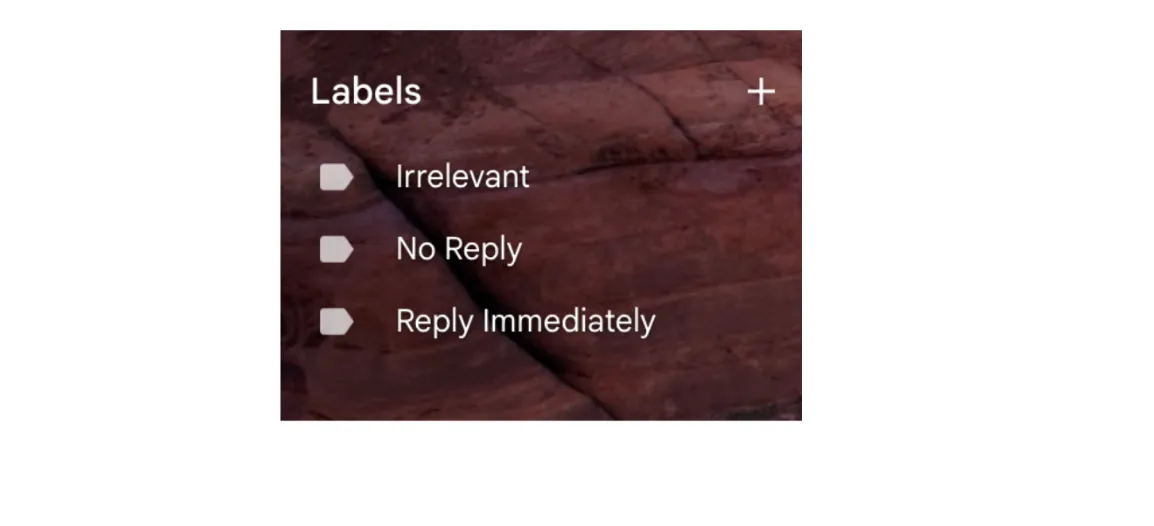
Additionally Learn: High 10 AI E mail Automation Instruments to Use in 2024
Steps for Google Authentication
Earlier than we leap to the code to type emails in Gmail, it is advisable allow the Gmail API and generate the OAuth 2.0 credentials. This can assist your e mail sorting agentic system entry your emails. Listed below are the steps to do that.
Step 1: Create a New Venture in Google Cloud
Step one in a Google authentication course of is to create a brand new undertaking in Google Cloud.
- 1. Go to the Google Cloud console and log in together with your e mail deal with. First-time customers should create an account.
- Then choose “New Venture” within the dropdown, give it a reputation, and click on Create. This undertaking can have the required API-related configurations. Whereas including the brand new undertaking, select your organisation identify as the situation, as we’ve chosen analyticsvidhya.com
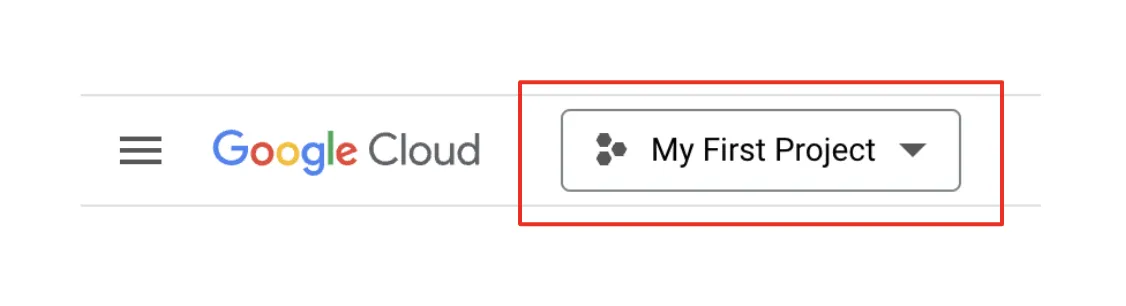
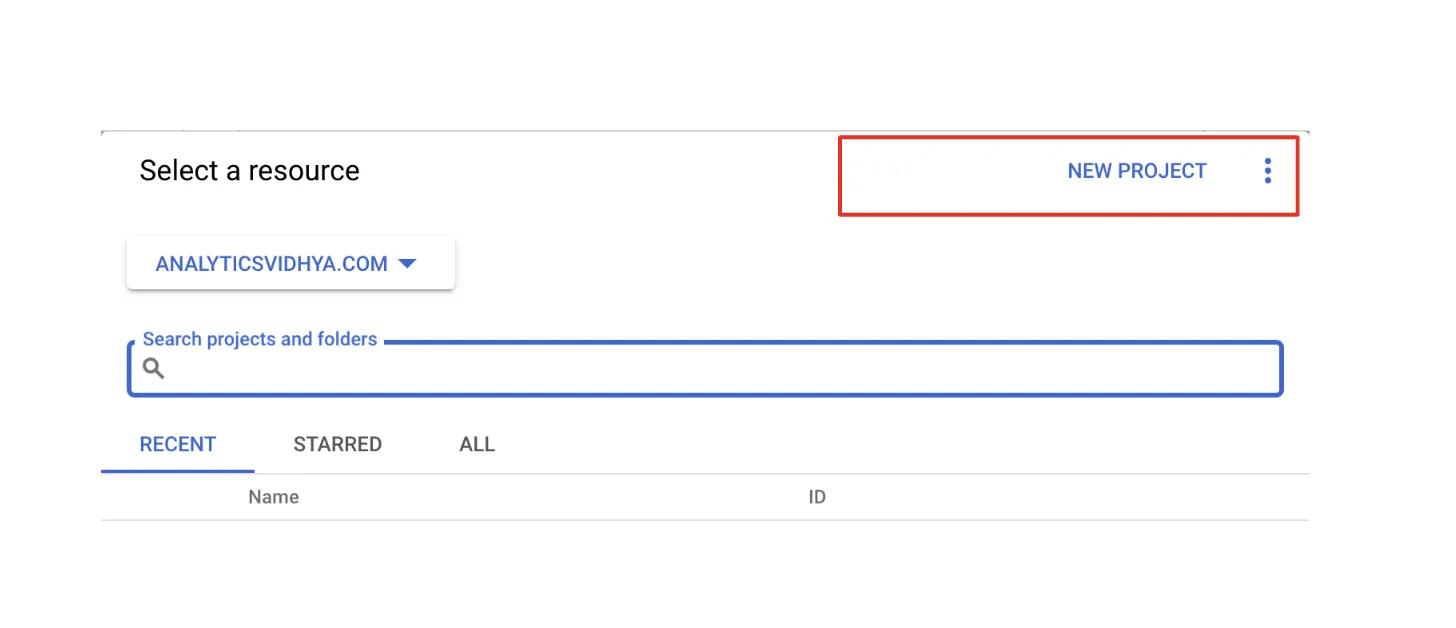
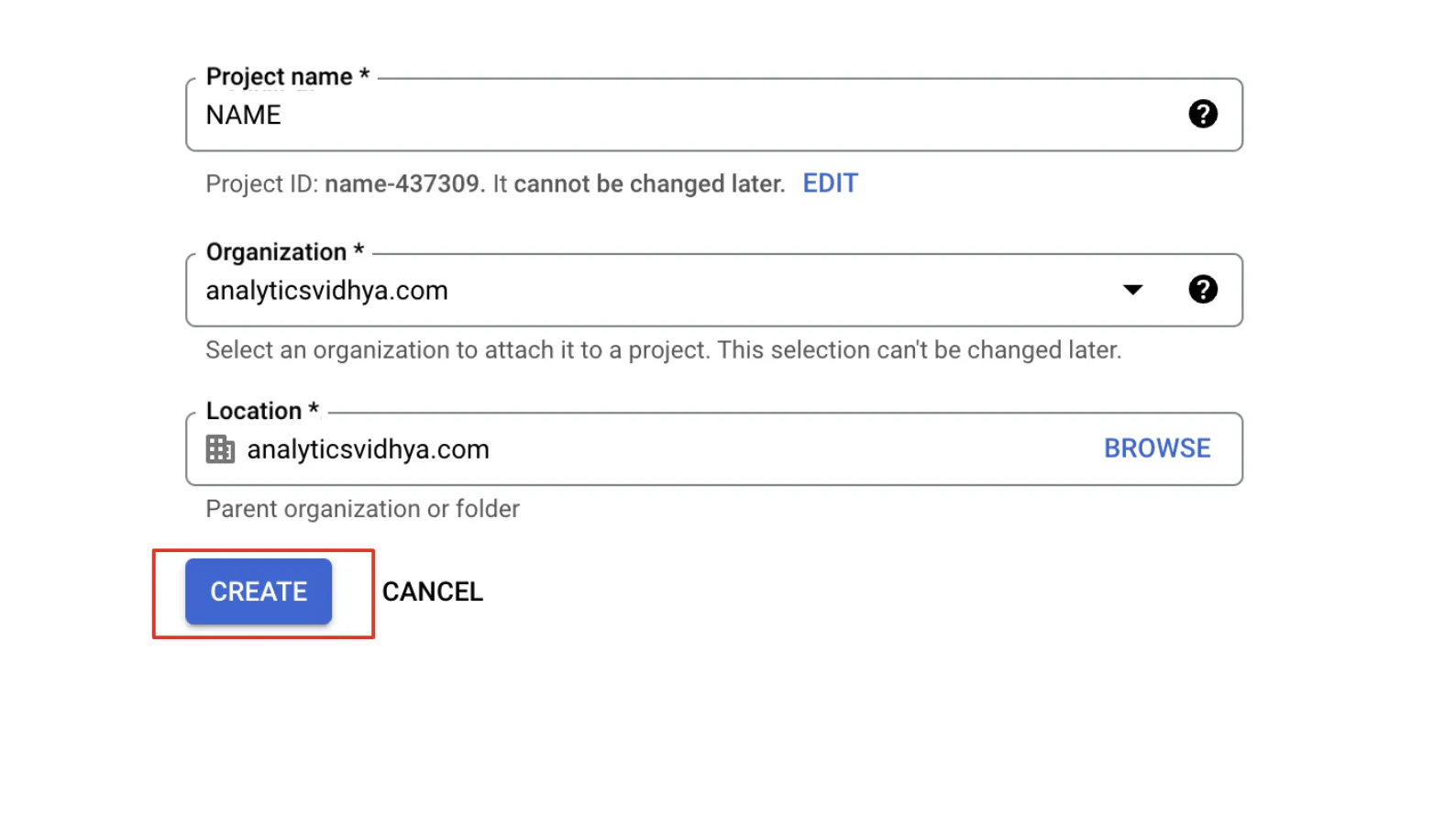
Step 2: Allow Gmail API
The subsequent step is to allow Gmail API.
- 1. Click on the Navigation Menu from the console’s Dashboard to Discover and Allow APIs underneath “Getting Began.“
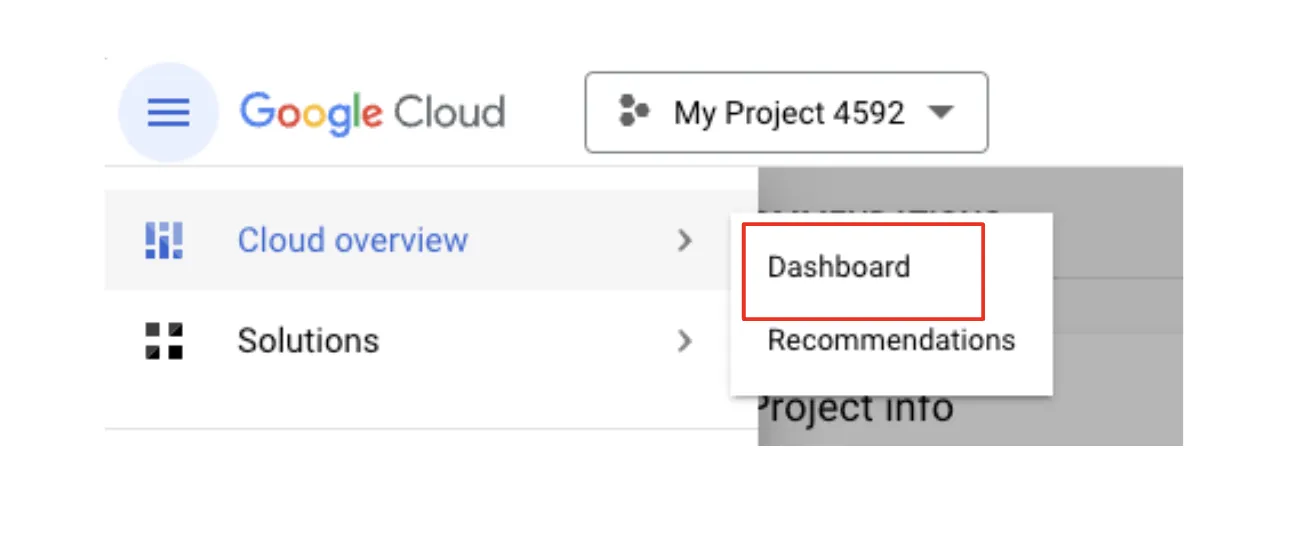
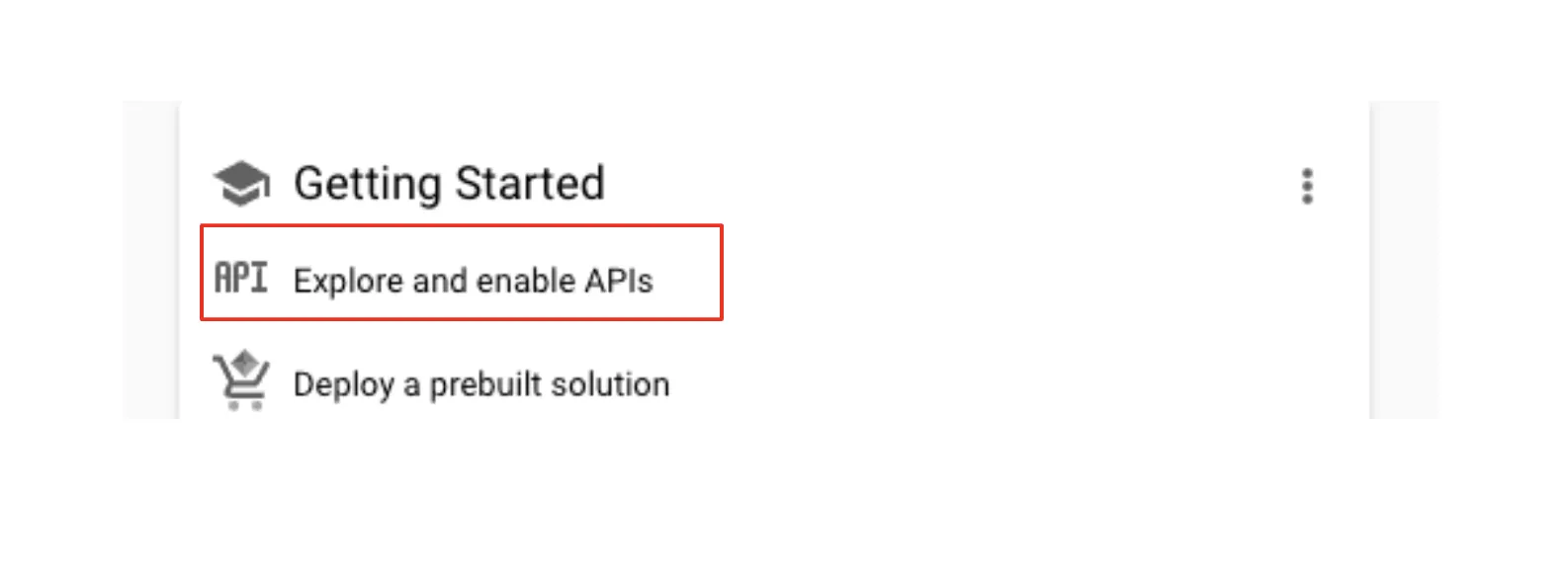
- On the left aspect of the display screen, choose Library and seek for “Gmail API.” Then, please allow it for the undertaking you created.
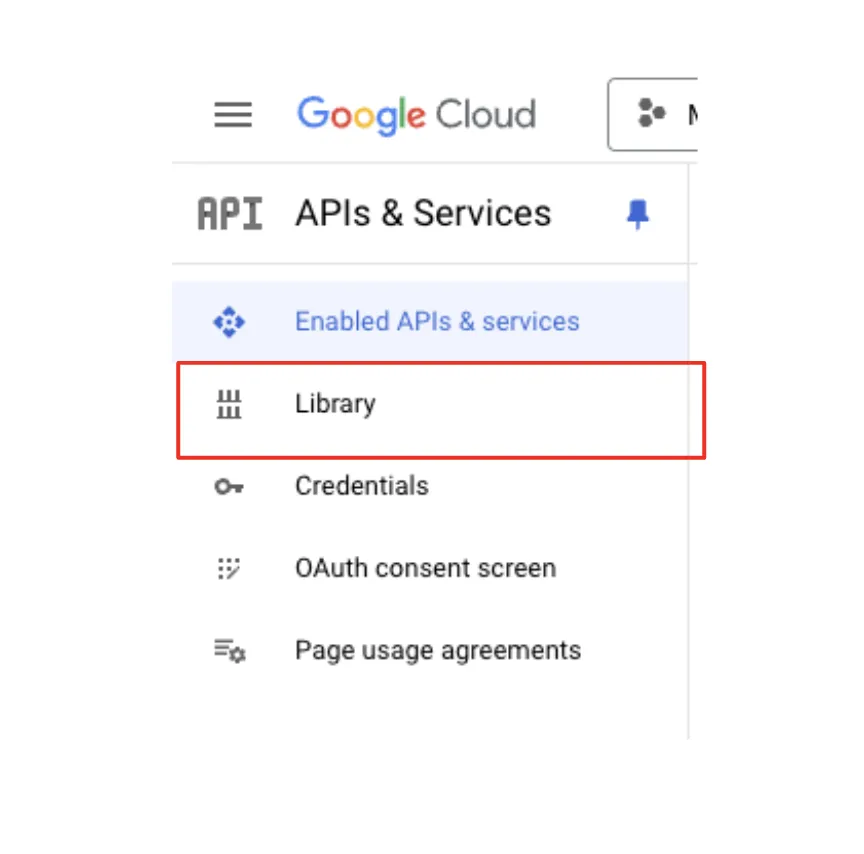
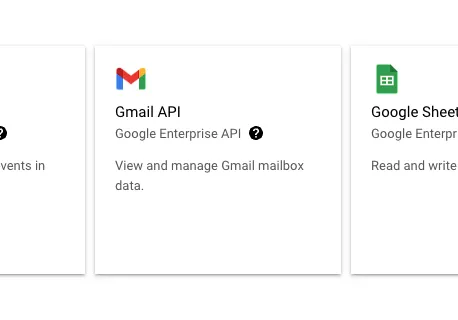
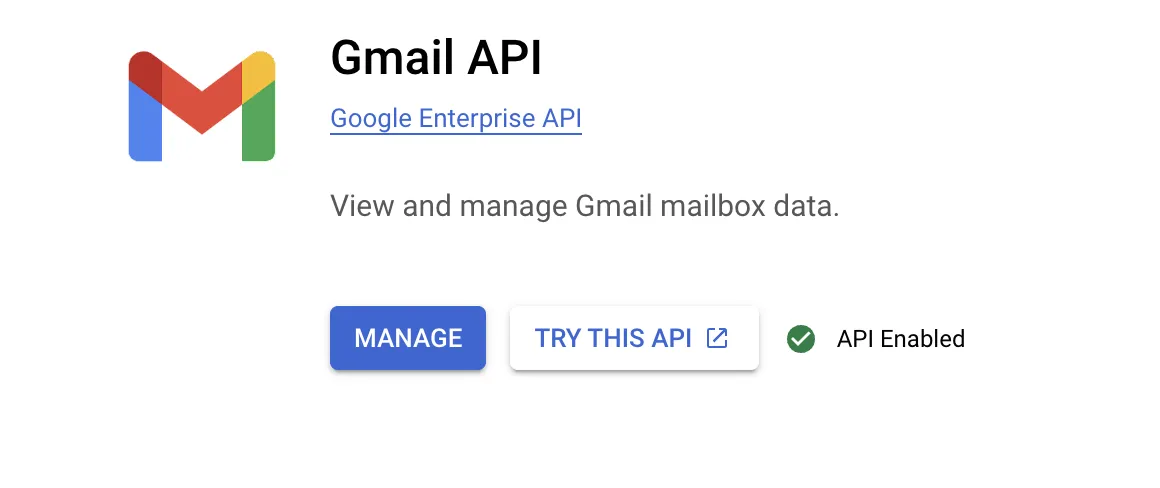
Step 3: Set Up OAuth 2.0 Credentials
The final step in Google Authentication is to arrange OAuth 2.0 credentials.
- First, arrange the OAuth consent display screen underneath APIs & Providers >. Click on Configure Consent Display.
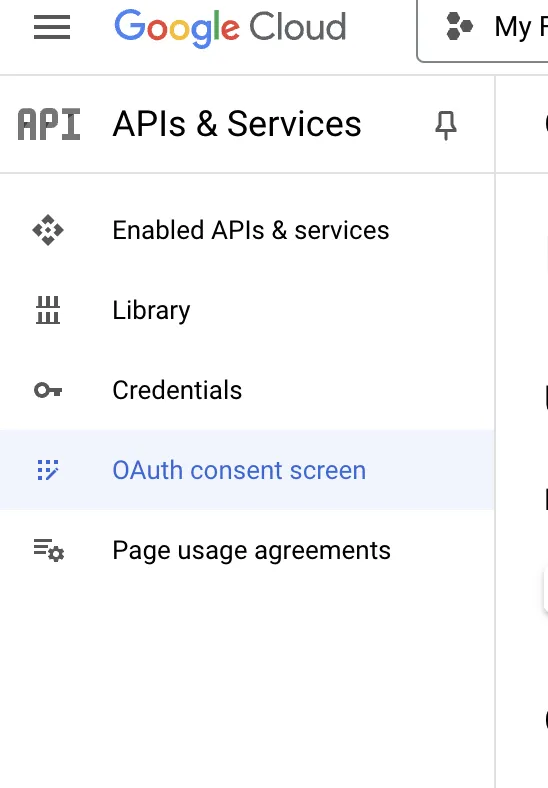

- Select the sort (e.g., Exterior for apps utilized by anybody). We selected Inside since we’re utilizing it for our e mail ID. Then Click on Create.
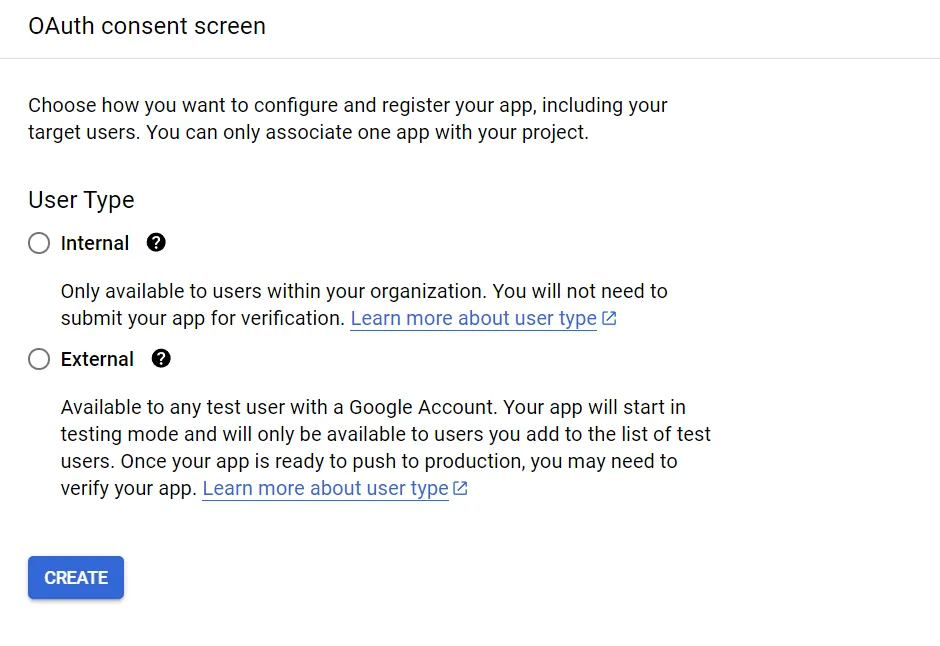
- Title your app and add the Person help e mail and Developer contact data. Ideally, you must add your work e mail ID. Click on SAVE AND CONTINUE on the backside of the display screen.
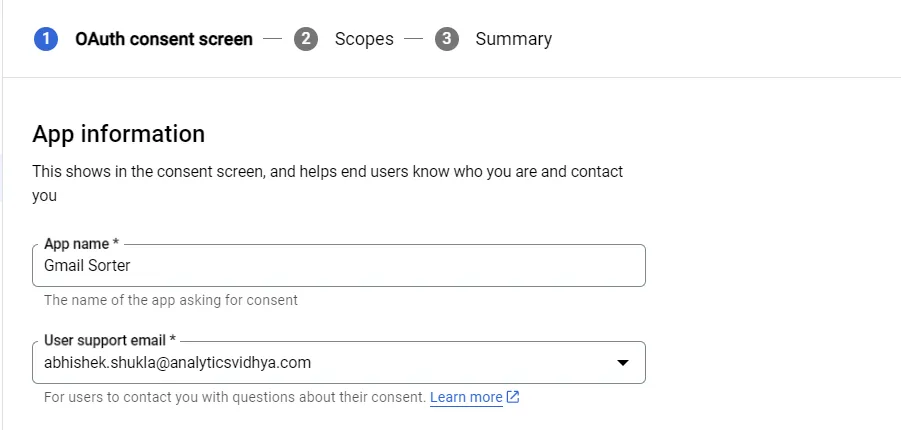
- Now, Outline the scopes within the consent display screen setup. Scopes within the context of Google Console dictate what the API can entry. For email-related duties, you’ll want the next: ‘https://www.googleapis.com/auth/gmail.modify‘. This scope will permit our e mail sorting and labelling system to ship and modify emails in your Gmail account.
Click on on ADD OR REMOVE SCOPES after which choose the scope talked about above.
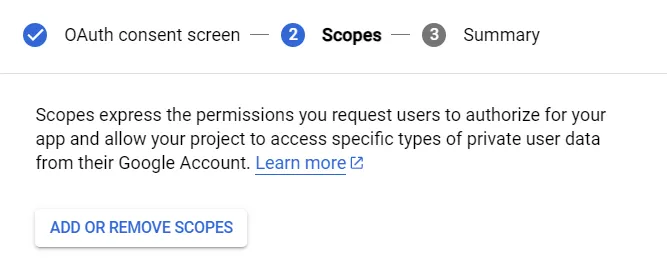
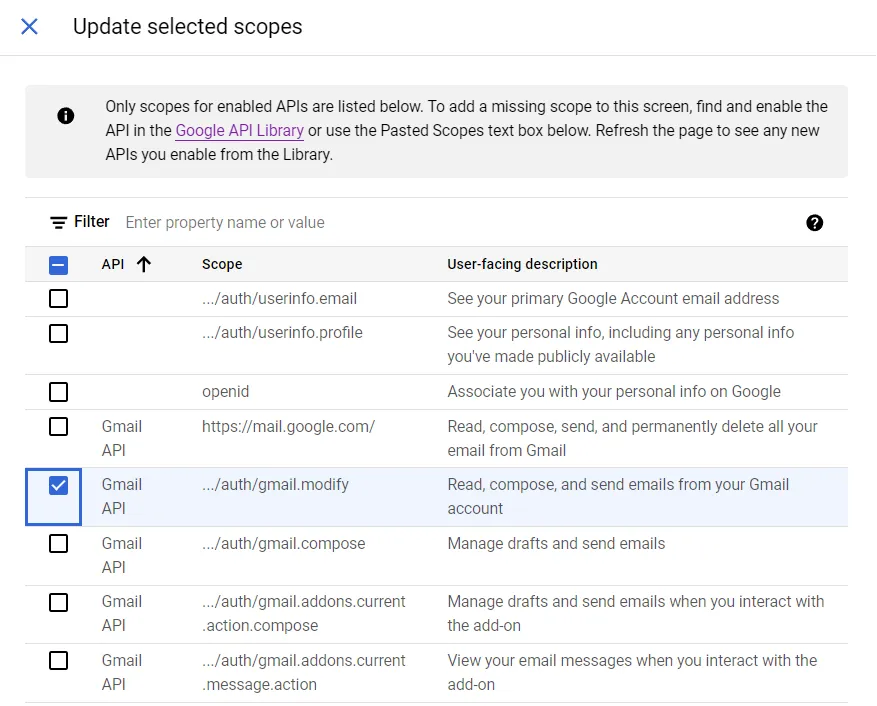
- Click on on Replace. You possibly can see the scope has been added. Press SAVE AND CONTINUE.
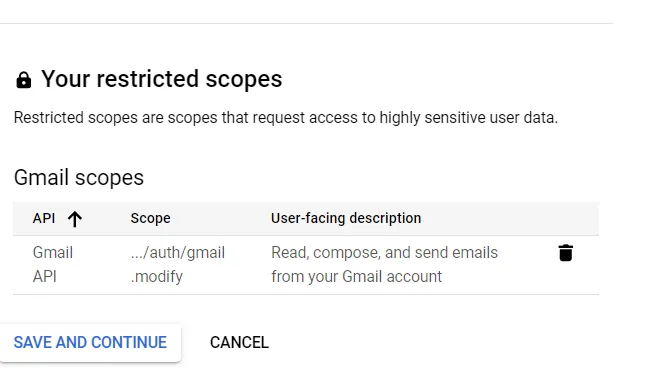
- Undergo the abstract and click on BACK TO DASHBOARD.
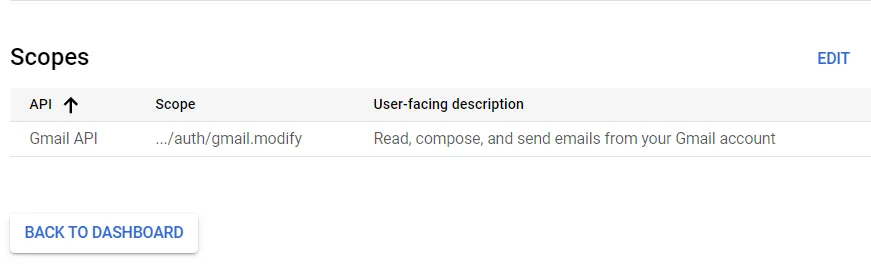
Step 4: Creating Credentials
- Now, select Credentials underneath APIs & Providers and click on CREATE CREDENTIALS.
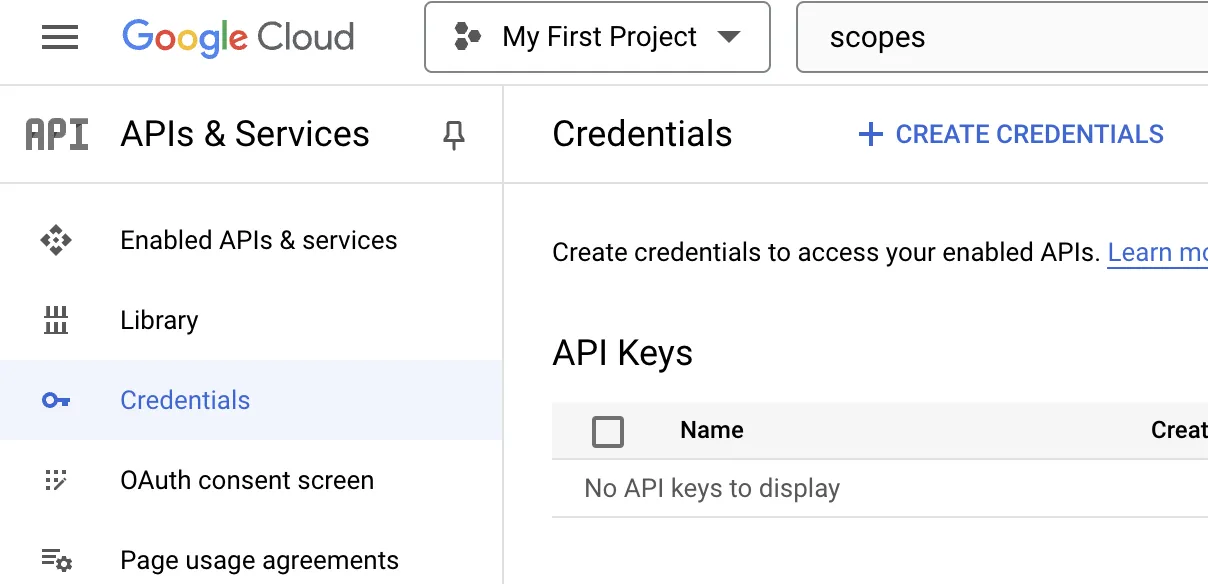
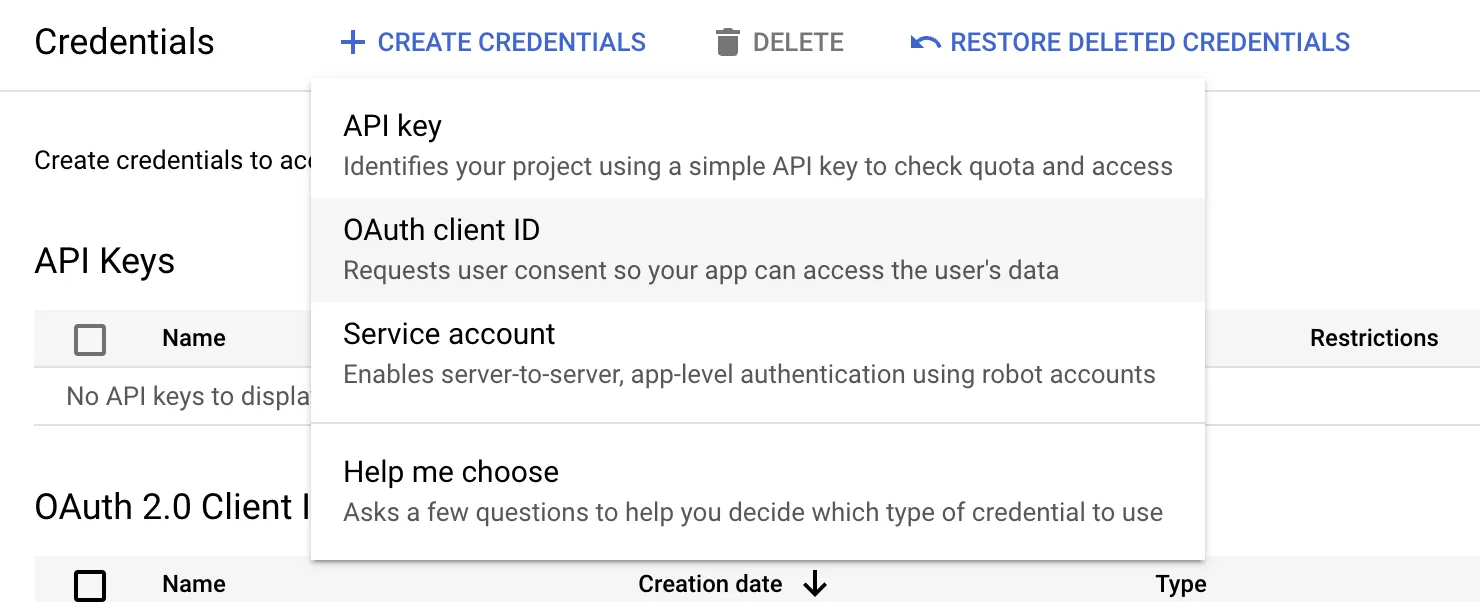
- For native improvement, we are going to select the Desktop App possibility. After which press CREATE.
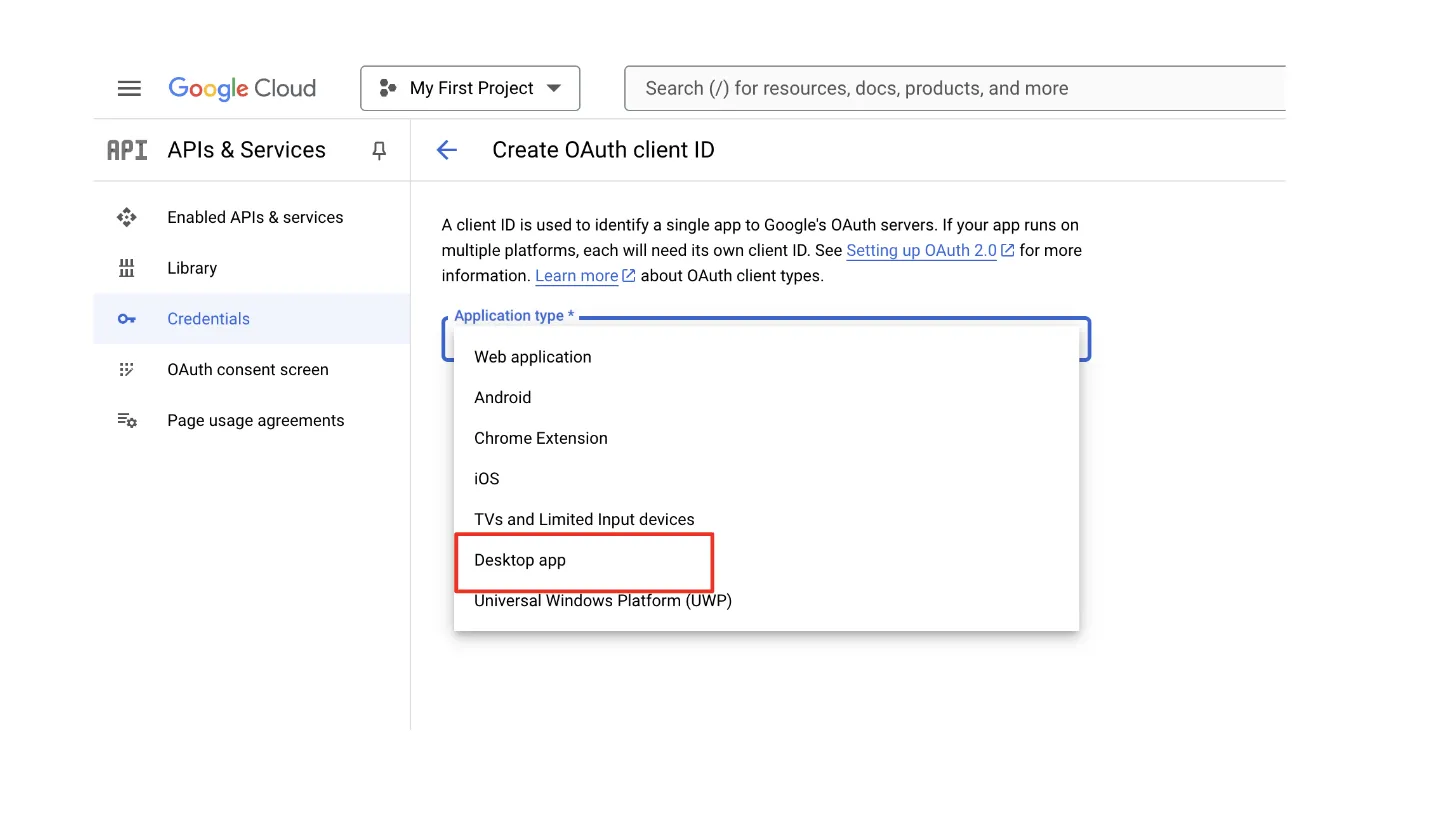
Step 5: Obtain the Credential.json
- Now obtain the JSON file and put it aside regionally at your most well-liked location.
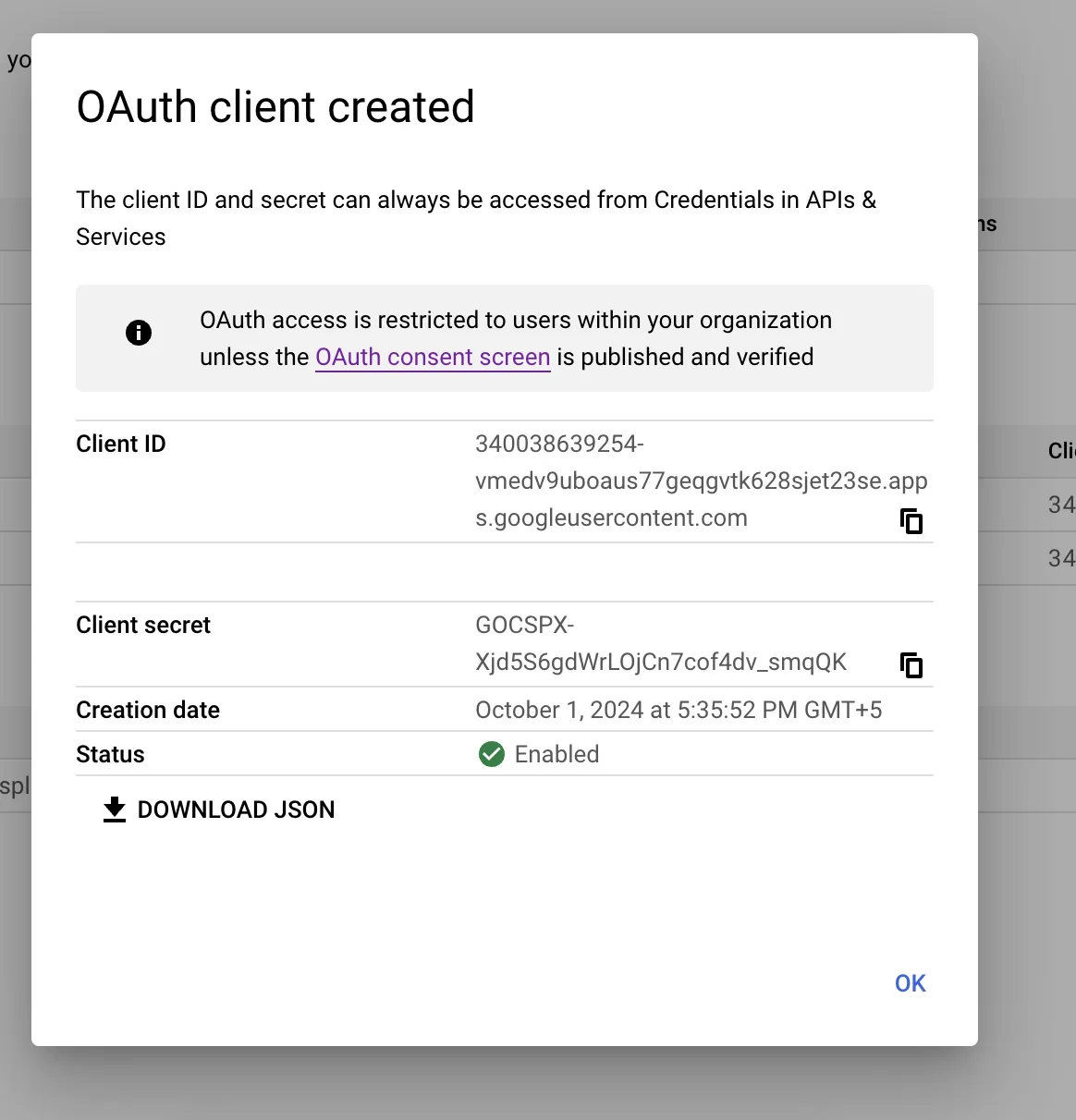
Python Code for Sorting and Labelling Emails Utilizing crewAI
We are going to now start coding the e-mail sorting and labelling system utilizing crewAI. Guarantee you could have put in crewAI and utils.
Set up crewAI
#pip set up crewai==0.28.8 crewai_tools==0.1.6
#pip set up utils
#pip set up google-auth-oauthlib
#pip set up google-api-python-client
Import Libraries
Now, we are going to import the related libraries.
# Import customary libraries
import json # For working with JSON knowledge
import os # For interacting with the working system
import os.path # For manipulating filesystem pathnames
import pickle # For serializing and deserializing Python objects
# Import Google API consumer libraries for authentication and API requests
from google.auth.transport.requests import Request # For making authenticated HTTP requests
from google.oauth2.credentials import Credentials # For dealing with OAuth 2.0 credentials
from google_auth_oauthlib.stream import InstalledAppFlow # For managing OAuth 2.0 authorization flows
from googleapiclient.discovery import construct # For establishing a Useful resource object for interacting with an API
from googleapiclient.errors import HttpError # For dealing with HTTP errors from API requests
# Import customized libraries (assuming these are your personal modules or third-party packages)
from crewai import Agent, Process, Crew # For managing brokers, duties, and crews
from crewai_tools import Instrument # For added instruments and utilities
# Import pandas library
import pandas as pd # For knowledge manipulation and evaluation
Entry to LLM
After this, we are going to give our e mail sorter and labeller entry to an LLM. I’m utilizing the GPT 4o mannequin for this process, however you may select the LLM of your selection.
# Set OpenAI API key
os.environ['OPENAI_API_KEY'] = "Your API Key"
os.environ["OPENAI_MODEL_NAME"] = 'Mannequin Title’'
Now, we are going to create the EmailCollector process, which makes use of Google’s API to work together with Gmail and fetch unread emails.
class EmailCollector:
def __init__(self):
self.creds = None
self._authenticate()
def _authenticate(self):
SCOPES = ['https://www.googleapis.com/auth/gmail.modify'] # Use modify to replace emails
if os.path.exists('token.pickle'):
with open('token.pickle', 'rb') as token:
self.creds = pickle.load(token)
if not self.creds or not self.creds.legitimate:
if self.creds and self.creds.expired and self.creds.refresh_token:
self.creds.refresh(Request())
else:
stream = InstalledAppFlow.from_client_secrets_file(
'/Customers/admin/Desktop/Blogs/credentials.json', SCOPES)
self.creds = stream.run_local_server(port=0)
with open('token.pickle', 'wb') as token:
pickle.dump(self.creds, token)
def get_unread_emails(self):
service = construct('gmail', 'v1', credentials=self.creds)
outcomes = service.customers().messages().checklist(userId='me', q='is:unread').execute()
messages = outcomes.get('messages', [])
email_list = []
if not messages:
print('No unread emails discovered.')
else:
for message in messages:
msg = service.customers().messages().get(userId='me', id=message['id'], format="full").execute()
email_list.append(msg)
return email_list
Code Clarification
Here’s what the above code does:
- First, the EmailCollector initialises with self.creds = None and calls the _authenticate methodology to deal with authentication.
- Then, the _authenticate methodology checks if a token.pickle file exists. If it exists, it hundreds the saved credentials from the file utilizing pickle.
- If credentials are expired or lacking, it checks if a refresh token is obtainable to refresh them. If not, it initiates an OAuth stream utilizing InstalledAppFlow, prompting the person to log in.
- After acquiring the credentials (new or refreshed), they’re saved to the token pickle for future use.
- The get_unread_emails methodology retrieves a listing of unread emails after connecting to the Gmail API utilizing the authenticated credentials.
- It loops by way of every unread e mail, retrieves full message particulars, and shops them within the email_list.
- Lastly, the checklist of unread emails is returned for additional use.
Create Mail Knowledge Gatherer
Now, we are going to create the mailDataGatherer() operate to assemble the unread emails and save them as a .csv file.
def mailDataGatherer():
# Create the E mail Collector occasion
email_collector_instance = EmailCollector()
# Get unread emails
email_list = email_collector_instance.get_unread_emails()
# Put together knowledge for DataFrame
emails_data = []
for e mail in email_list:
topic = subsequent(header['value'] for header in e mail['payload']['headers'] if header['name'] == 'Topic')
physique = ''
if 'components' in e mail['payload']:
for half in e mail['payload']['parts']:
if half['mimeType'] == 'textual content/plain':
physique = half['body']['data']
break
else:
physique = e mail['payload']['body']['data']
import base64
physique = base64.urlsafe_b64decode(physique).decode('utf-8')
emails_data.append({
'Topic': topic,
'Physique': physique
})
# Create a DataFrame
df = pd.DataFrame(emails_data)
# Save DataFrame to CSV
df.to_csv('unread_emails.csv', index=False)
df.fillna("",inplace=True)
# Print the DataFrame
return df
Code Clarification
Right here is an evidence of the above code:
- First, the operate handles authentication and retrieves unread emails utilizing an occasion of the EmailCollector class.
- Then, we fetch the unread emails utilizing the get_unread_emails() methodology from the EmailCollector occasion.
- After that, we course of the e-mail knowledge contained in the loop, which is
- The operate extracts the topic from the e-mail headers for every e mail within the checklist.
- It checks the e-mail’s physique, decoding it from base64 format to get the precise textual content.
- It collects each the topic and physique of the emails.
- We are going to retailer the processed e mail knowledge (topic and physique) in a Pandas DataFrame.
- The DataFrame is saved as a CSV file referred to as unread_emails.csv, and any lacking values are full of empty strings.
- Lastly, the operate returns the DataFrame for additional use or viewing.
Now, we are going to create the extract_mail_tool for our agent utilizing the Instrument performance inside crewAI. This instrument will use the emaiDataGatherer operate to assemble the topic and physique of the unread e mail.
# Create the Extract Topics instrument
extract_mail_tool = Instrument(
identify="mailDataGatherer",
description="Get all the themes and physique content material of unread emails.",
func=mailDataGatherer
)
Now, we are going to create a operate that takes within the JSON illustration of the unread emails. It then decodes the emails and labels the corresponding emails in our Gmail inbox as per the pre-defined classes – ‘Reply Instantly’, ‘No Reply’, and ‘Irrelevan.t’
def push_mail_label(dfjson):
emails = pd.DataFrame(json.hundreds(dfjson.substitute("```","").substitute("json","")))
SCOPES = ['https://www.googleapis.com/auth/gmail.modify'] # Use modify to replace emails
#Change the identify and path of your file beneath accordingly
stream = InstalledAppFlow.from_client_secrets_file(
'/Customers/admin/Desktop/Blogs/credentials.json', SCOPES)
creds = stream.run_local_server(port=0)
service = construct('gmail', 'v1', credentials=creds)
labels = service.customers().labels().checklist(userId='me').execute().get('labels', [])
label_map = {label['name']: label['id'] for label in labels}
def get_or_create_label(label_name):
if label_name in label_map:
return label_map[label_name]
else:
# Create new label if it does not exist
new_label = {
'labelListVisibility': 'labelShow',
'messageListVisibility': 'present',
'identify': label_name
}
created_label = service.customers().labels().create(userId='me', physique=new_label).execute()
return created_label['id']
# Map the classes to Gmail labels, creating them if they do not exist
category_label_map = {
"Reply Instantly": get_or_create_label("Reply Instantly"),
"No Reply": get_or_create_label("No Reply"),
"Irrelevant": get_or_create_label("Irrelevant")
}
for i in vary(emails.form[0]):
topic = emails.iloc[i]['Subject']
physique = emails.iloc[i]['Body']
class = emails.iloc[i]['Category']
search_query = f'topic:("{topic}")'
outcomes = service.customers().messages().checklist(userId='me', q=search_query).execute()
message_id = outcomes['messages'][0]['id'] # Get the primary matching message ID
label_id = category_label_map.get(class, None)
# Apply label based mostly on class
if label_id:
service.customers().messages().modify(
userId='me',
id=message_id,
physique={
'addLabelIds': [label_id],
'removeLabelIds': [] # Optionally add label removing logic right here
}
).execute()
print(f'Up to date e mail with topic: {topic} to label: {class}')
else:
print(f'Class {class} doesn't match any Gmail labels.')
return
How does this operate work?
The operate works as follows:
- The dfjson string is first cleaned of additional characters. It’s then parsed right into a Pandas DataFrame that accommodates columns like Topic, Physique, and Class for every e mail.
- Then, with Google OAuth 2.0, the operate authenticates with Gmail by way of InstalledAppFlow utilizing the gmail.modify scope. This enables it to change emails (including labels in our case).
- Subsequent, the operate retrieves all present Gmail labels from the person’s account and shops them in a label_map dictionary (label identify to label ID).
- After that, the get_or_create_label() operate checks if a label already exists. If not, it creates a brand new one utilizing the Gmail API.
- Subsequent, it maps the classes within the e mail knowledge to their respective Gmail labels (Reply Instantly, No Reply, Irrelevant), creating them if obligatory.
- For every e mail, the operate searches Gmail for an identical message by the topic, retrieves the message ID and applies the corresponding label based mostly on the class.
- On the finish, the operate prints a message indicating success or failure for every e mail it processes.
Defining the E mail Sorting Agent
This agent will classify the unread emails into three classes we’ve outlined. The agent makes use of the extract_mail_tool to entry the emails, which makes use of the mailDataGatherer() operate to get the emails. Since this agent makes use of LLM, it’s essential to give correct directions on what is predicted to be accomplished. For instance, one specific instruction I’ve added is in regards to the type of mail that should be included in each bit of content material. You possibly can learn the directions additional within the agent’s backstory parameter.
sorter = Agent(
position="E mail Sorter",
aim="Clasify the emails into one of many 3 classes offered",
instruments=[extract_mail_tool], # Add instruments if wanted
verbose=True,
backstory=(
"You might be an knowledgeable private assistant working for a busy company skilled."
"You might be dealing with the private e mail account of the CEO."
"You've been assigned a process to categorise the unread emails into one of many three classes."
"Checklist of the three classes is : ['Reply Immediately', 'No Reply' and 'Irrelevant']"
"'Reply Instantly' ought to embody unread emails from throughout the group and outdoors. This is usually a basic inquiry, question and calender invitations."
"'No Reply' contains unread emails the place somebody is letting me know of some improvement throughout the firm. It additionally contains Newsletters that I've subscribed to."
"'Irrelevant' accommodates unread emails which might be from exterior companies advertising and marketing there merchandise."
"You possibly can apply your personal intelligence whereas sorting the unread emails."
"You get the info of unread mails in a pandas dataframe utilizing the instrument mailDataGatherer."
"You're going to get 'Topic' and 'Physique' columns within the dataframe returned by instrument."
"You need to classify the mails on foundation of topic and physique and name it 'Class'."
"There isn't a instrument which you need to use for classification. Use your personal intelligence to do it."
"Lastly you come back a json with 3 keys for every mail: 'Topic', 'Physique', 'Class'"
)
)
Observe that we’ve set verbose = True, which helps us view how the mannequin thinks.
Outline the Process
Now, we are going to outline the Process that the sorter agent will carry out. Now we have added the precise checklist of duties, which you’ll be able to learn within the code beneath.
# Process for Sorter: Kind Emails
sort_task = Process(
aim="Kind the categorised emails into: ['Reply Immediately', 'No Reply' and 'Irrelevant'] ",
description= "1. At first, get the dataframe of unread emails utilizing the 'mailDataGatherer'."
"2. Don't use the instrument once more after getting acquired the topic and physique"
"3. Classify the emails into one of many above classes: ['Reply Immediately', 'No Reply' and 'Irrelevant']."
"4. There isn't a instrument which you need to use for classification. Use the directions and your personal intelligence to do it."
"5. Lastly, return a json with three keys for every row: ['Subject', 'Body', 'Category']"
"6. All of the emails should strictly be categorised into one in all above three classes solely.",
expected_output = "A json file with all of the unread emails. There needs to be 3 keys for every mail- Topic, Physique and Class ",
agent=sorter,
async_execution=False
)
Allow Collaboration in CrewAI
Subsequent, we are going to use crewAI’s performance to allow collaboration and let the sorter agent carry out the duties outlined within the sort_task operate above.
# Create the Crew
email_sorting_crew = Crew(
brokers=[sorter],
duties=[sort_task],
verbose=True
)
Lastly, we are going to kickoff our crew to let it type the unread emails mechanically and save the output within the end result variable.
# Working the crew
end result = email_sorting_crew.kickoff()
Since verbose = True for all parts in our agent, we get to see how our agent performs.
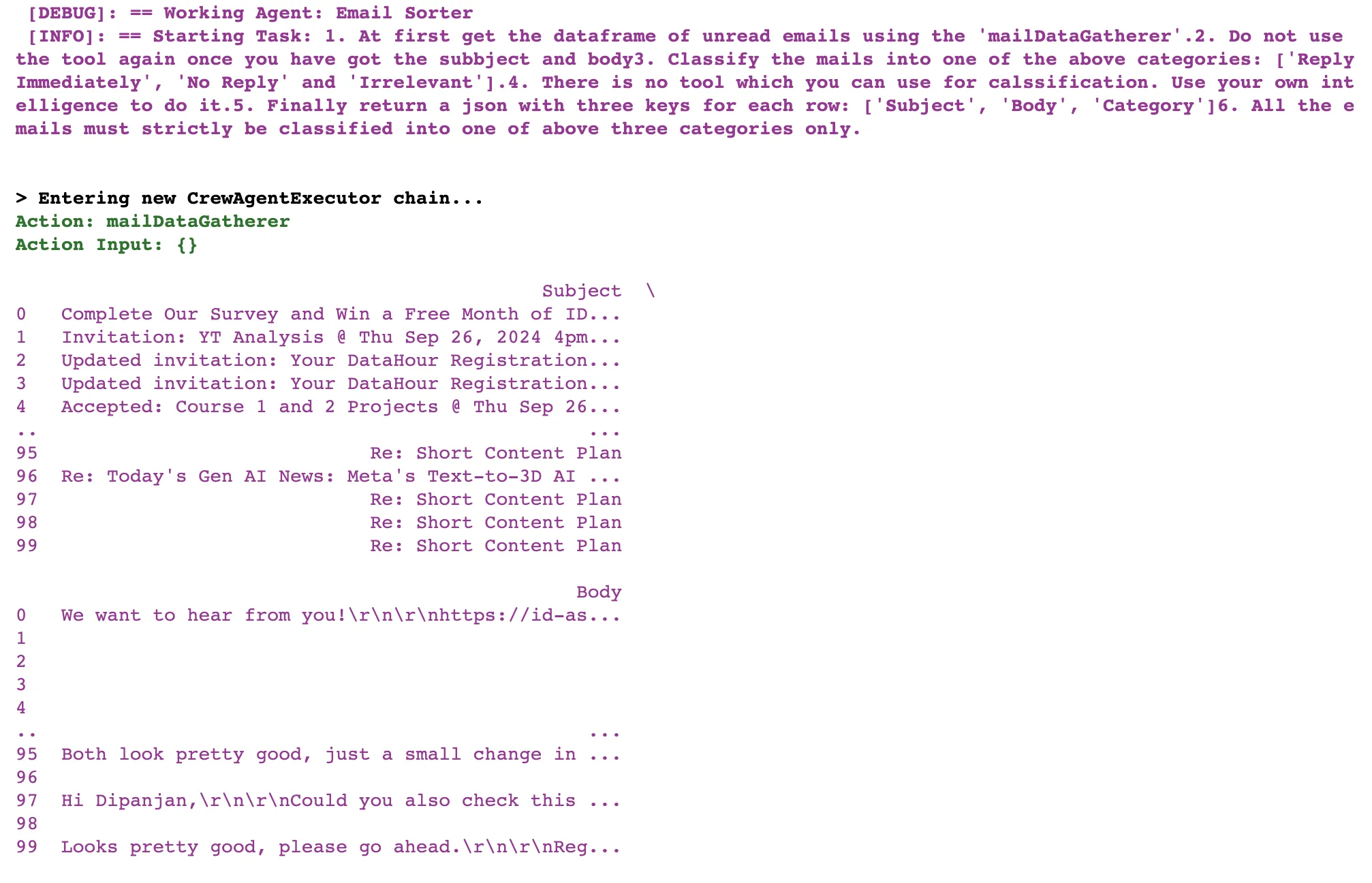
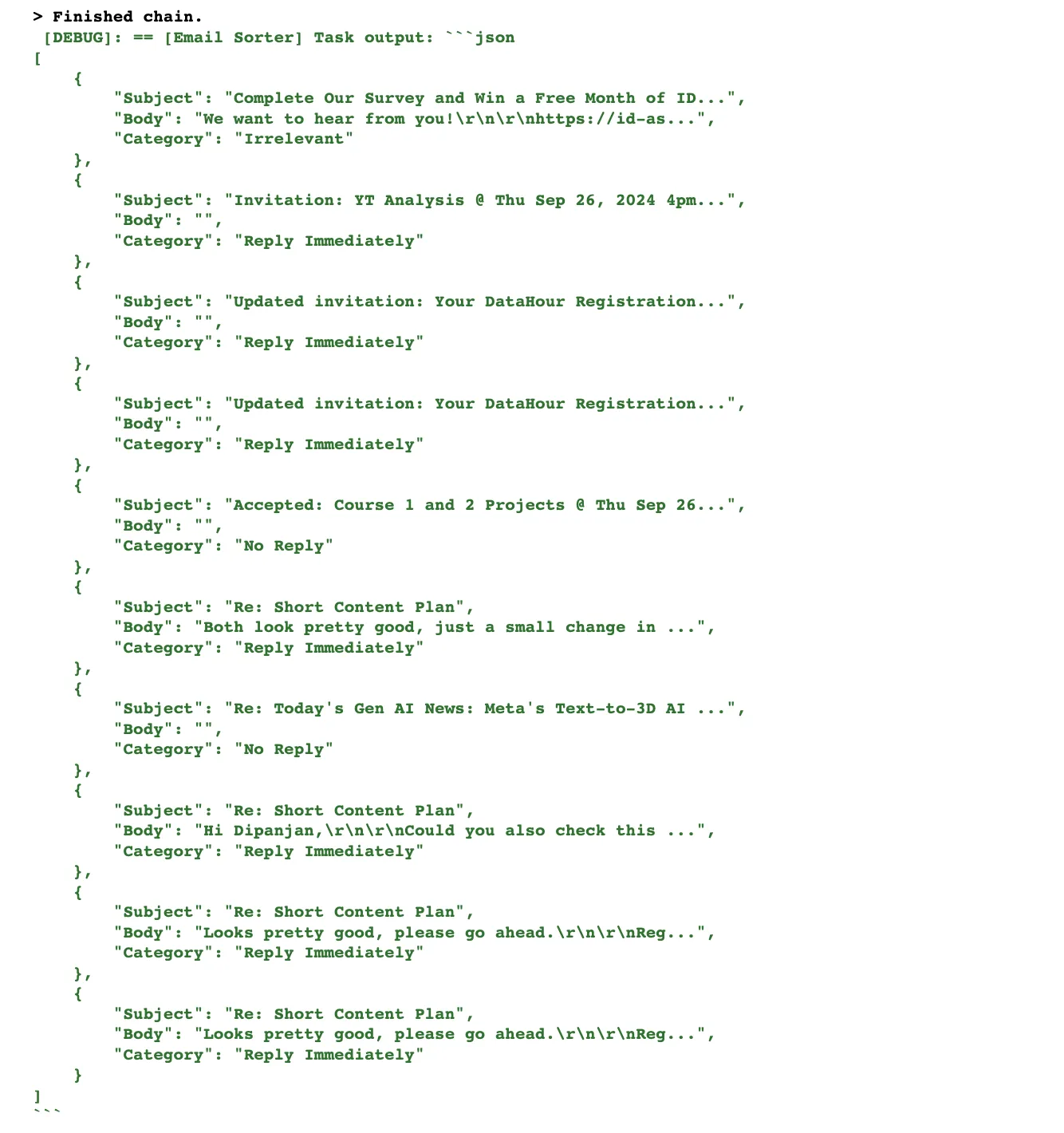
Now, we push this end result into our Gmail utilizing the push_mail_label() operate.
#push the outcomes
push_mail_label(end result)
Observe that all through the execution, you’ll be twice redirected to a special window to offer the required permissions. As soon as the code can reaccess the emails, the unread emails will likely be pushed underneath the related labels.
And Voila! Now we have the emails with labels connected. For privateness, I can’t show my complete inbox.

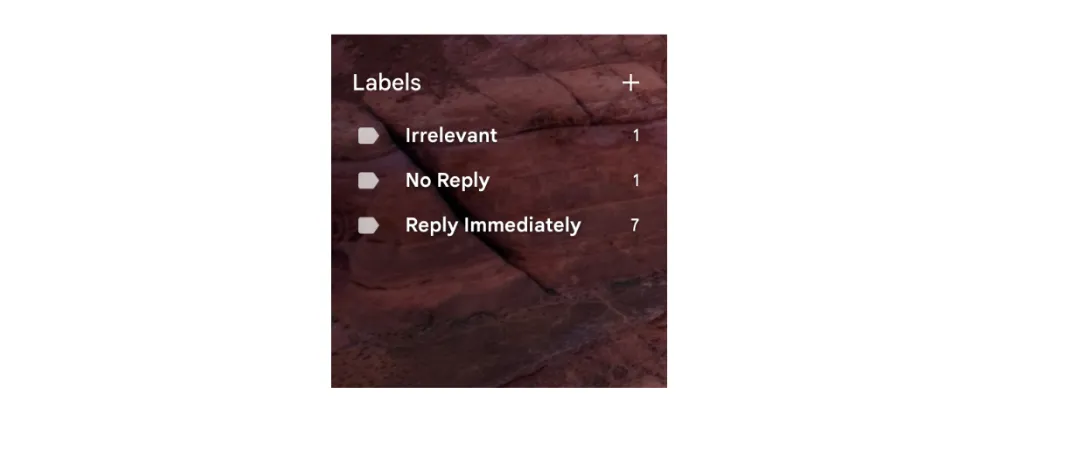
Conclusion
Keep in mind the frustration we mentioned earlier within the weblog? Nicely, crewAI’s e mail sorting and labelling agentic system permits us to avoid wasting a major period of time.
With LLM brokers, the chances of process automation are limitless. It relies on how nicely you suppose and construction your agentic system. As a subsequent step, you may strive constructing an agent that checks whether or not the primary agent’s categorisation is appropriate. Or, you may also strive creating an agent that prepares an e mail for the ‘Reply Instantly’ class and pushes it to the drafts in Gmail. Sounds formidable? So, go on and get your palms soiled. Pleased studying!!
Incessantly Requested Questions
A. crewAI is an open-source Python framework that helps creating and managing multi-agent AI methods. Utilizing crewAI, you may construct LLM-backed AI brokers that may autonomously make selections inside an surroundings based mostly on the variables current.
A. Sure! You should utilize crewAI to construct LLM-backed brokers to automate e mail sorting and labelling duties.
A. Relying in your agentic system construction, you need to use as many brokers as you prefer to type emails in Gmail. Nevertheless, it is suggested that you simply construct one agent per process.
A. CrewAI can carry out varied duties, together with sorting and writing emails, planning initiatives, producing articles, scheduling and posting social media content material, and extra.
A. Since GenAI brokers utilizing crewAI make selections utilizing LLMs, it reduces the requirement to allocate human sources to undergo emails and make selections.
A. To automate e mail sorting utilizing crewAI, it’s essential to outline the brokers with a descriptive backstory throughout the Agent operate, outline duties for every agent utilizing the Process performance, after which create a Crew to allow totally different brokers to collaborate.