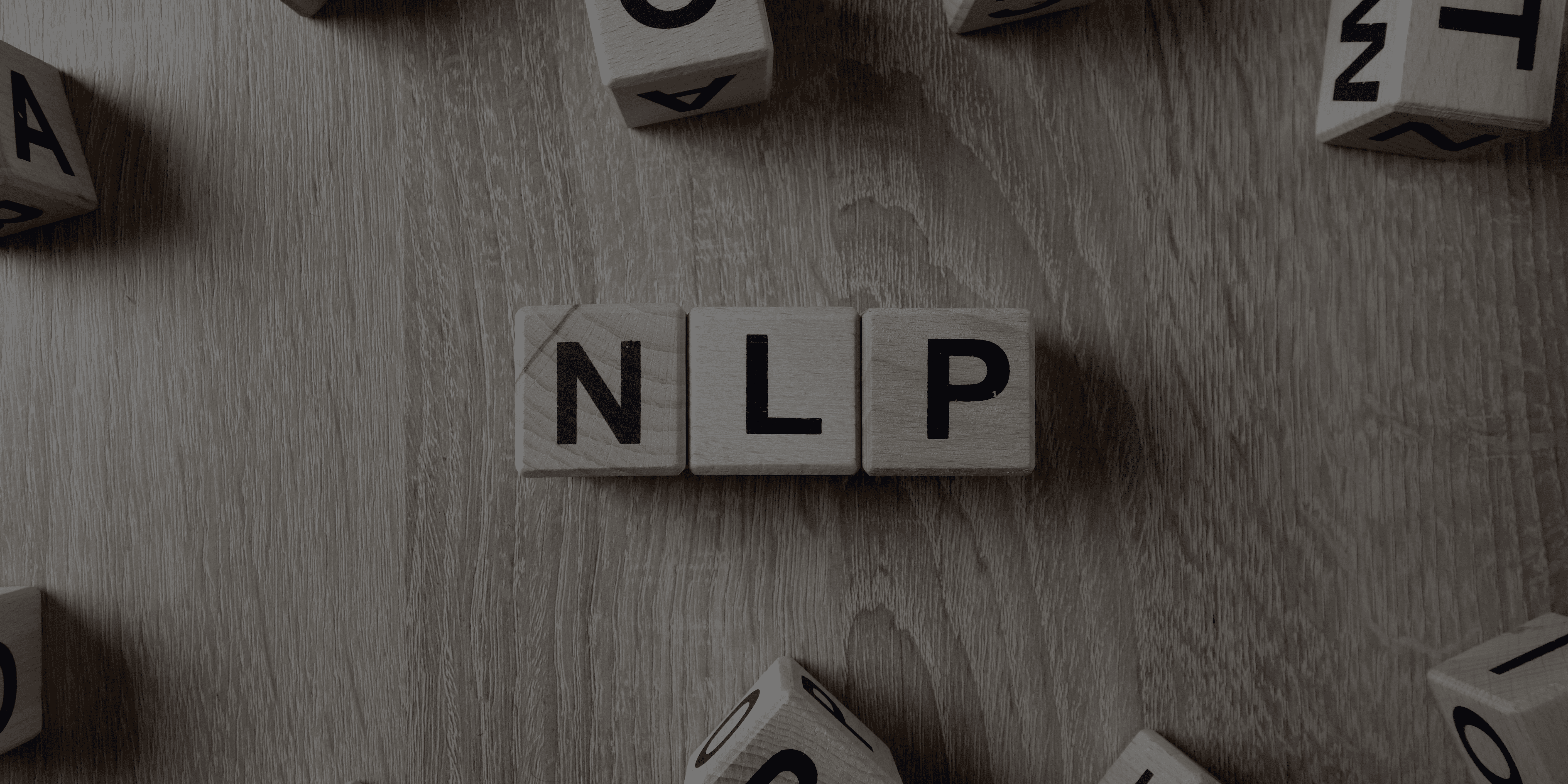
Picture by Creator
Cleansing and preprocessing information is commonly one of the crucial daunting, but essential phases in constructing AI and Machine Studying options fueled by information, and textual content information shouldn’t be the exception.
Our High 5 Free Course Suggestions
1. Google Cybersecurity Certificates – Get on the quick observe to a profession in cybersecurity.
2. Pure Language Processing in TensorFlow – Construct NLP methods
3. Python for All people – Develop packages to assemble, clear, analyze, and visualize information
4. Google IT Assist Skilled Certificates
5. AWS Cloud Options Architect – Skilled Certificates
This tutorial breaks the ice in tackling the problem of getting ready textual content information for NLP duties corresponding to these Language Fashions (LMs) can clear up. By encapsulating your textual content information in pandas DataFrames, the under steps will assist you to get your textual content prepared for being digested by NLP fashions and algorithms.
Load the information right into a Pandas DataFrame
To maintain this tutorial easy and targeted on understanding the required textual content cleansing and preprocessing steps, let’s think about a small pattern of 4 single-attribute textual content information cases that will probably be moved right into a pandas DataFrame occasion. We’ll to any extent further apply each preprocessing step on this DataFrame object.
import pandas as pd
information = {'textual content': ["I love cooking!", "Baking is fun", None, "Japanese cuisine is great!"]}
df = pd.DataFrame(information)
print(df)
Output:
textual content
0 I like cooking!
1 Baking is enjoyable
2 None
3 Japanese delicacies is nice!
Deal with lacking values
Did you discover the ‘None’ worth in one of many instance information cases? This is named a lacking worth. Lacking values are generally collected for varied causes, usually unintended. The underside line: it is advisable to deal with them. The best strategy is to easily detect and take away cases containing lacking values, as performed within the code under:
df.dropna(subset=['text'], inplace=True)
print(df)
Output:
textual content
0 I like cooking!
1 Baking is enjoyable
3 Japanese delicacies is nice!
Normalize the textual content to make it constant
Normalizing textual content implies standardizing or unifying parts which will seem underneath totally different codecs throughout totally different cases, as an illustration, date codecs, full names, or case sensitiveness. The best strategy to normalize our textual content is to transform all of it to lowercase, as follows.
df['text'] = df['text'].str.decrease()
print(df)
Output:
textual content
0 i like cooking!
1 baking is enjoyable
3 japanese delicacies is nice!
Take away noise
Noise is pointless or unexpectedly collected information which will hinder the next modeling or prediction processes if not dealt with adequately. In our instance, we’ll assume that punctuation marks like “!” should not wanted for the next NLP activity to be utilized, therefore we apply some noise elimination on it by detecting punctuation marks within the textual content utilizing a daily expression. The ‘re’ Python bundle is used for working and performing textual content operations based mostly on common expression matching.
import re
df['text'] = df['text'].apply(lambda x: re.sub(r'[^ws]', '', x))
print(df)
Output:
textual content
0 i like cooking
1 baking is enjoyable
3 japanese delicacies is nice
Tokenize the textual content
Tokenization is arguably crucial textual content preprocessing step -along with encoding textual content right into a numerical representation- earlier than utilizing NLP and language fashions. It consists in splitting every textual content enter right into a vector of chunks or tokens. Within the easiest situation, tokens are related to phrases more often than not, however in some circumstances like compound phrases, one phrase may result in a number of tokens. Sure punctuation marks (in the event that they weren’t beforehand eliminated as noise) are additionally generally recognized as standalone tokens.
This code splits every of our three textual content entries into particular person phrases (tokens) and provides them as a brand new column in our DataFrame, then shows the up to date information construction with its two columns. The simplified tokenization strategy utilized is named easy whitespace tokenization: it simply makes use of whitespaces because the criterion to detect and separate tokens.
df['tokens'] = df['text'].str.cut up()
print(df)
Output:
textual content tokens
0 i like cooking [i, love, cooking]
1 baking is enjoyable [baking, is, fun]
3 japanese delicacies is nice [japanese, cuisine, is, great]
Take away stopwords
As soon as the textual content is tokenized, we filter out pointless tokens. That is usually the case of stopwords, like articles “a/an, the”, or conjunctions, which don’t add precise semantics to the textual content and must be eliminated for later environment friendly processing. This course of is language-dependent: the code under makes use of the NLTK library to obtain a dictionary of English stopwords and filter them out from the token vectors.
import nltk
nltk.obtain('stopwords')
stop_words = set(stopwords.phrases('english'))
df['tokens'] = df['tokens'].apply(lambda x: [word for word in x if word not in stop_words])
print(df['tokens'])
Output:
0 [love, cooking]
1 [baking, fun]
3 [japanese, cuisine, great]
Stemming and lemmatization
Nearly there! Stemming and lemmatization are further textual content preprocessing steps which may generally be used relying on the particular activity at hand. Stemming reduces every token (phrase) to its base or root type, while lemmatization additional reduces it to its lemma or base dictionary type relying on the context, e.g. “greatest” -> “good”. For simplicity, we’ll solely apply stemming on this instance, through the use of the PorterStemmer carried out within the NLTK library, aided by the wordnet dataset of word-root associations. The ensuing stemmed phrases are saved in a brand new column within the DataFrame.
from nltk.stem import PorterStemmer
nltk.obtain('wordnet')
stemmer = PorterStemmer()
df['stemmed'] = df['tokens'].apply(lambda x: [stemmer.stem(word) for word in x])
print(df[['tokens','stemmed']])
Output:
tokens stemmed
0 [love, cooking] [love, cook]
1 [baking, fun] [bake, fun]
3 [japanese, cuisine, great] [japanes, cuisin, great]
Convert your textual content into numerical representations
Final however not least, laptop algorithms together with AI/ML fashions don’t perceive human language however numbers, therefore we have to map our phrase vectors into numerical representations, generally often known as embedding vectors, or just embedding. The under instance converts tokenized textual content within the ‘tokens’ column and makes use of a TF-IDF vectorization strategy (one of the crucial widespread approaches within the good outdated days of classical NLP) to remodel the textual content into numerical representations.
from sklearn.feature_extraction.textual content import TfidfVectorizer
df['text'] = df['tokens'].apply(lambda x: ' '.be part of(x))
vectorizer = TfidfVectorizer()
X = vectorizer.fit_transform(df['text'])
print(X.toarray())
Output:
[[0. 0.70710678 0. 0. 0. 0. 0.70710678]
[0.70710678 0. 0. 0.70710678 0. 0. 0. ]
[0. 0. 0.57735027 0. 0.57735027 0.57735027 0. ]]
And that is it! As unintelligible as it might appear to us, this numerical illustration of our preprocessed textual content is what clever methods together with NLP fashions do perceive and may deal with exceptionally properly for difficult language duties like classifying sentiment in textual content, summarizing it, and even translating it to a different language.
The subsequent step can be feeding these numerical representations to our NLP mannequin to let it do its magic.
Iván Palomares Carrascosa is a pacesetter, author, speaker, and adviser in AI, machine studying, deep studying & LLMs. He trains and guides others in harnessing AI in the actual world.