With the rise of AI in finance, traders are more and more leveraging AI-driven insights for higher decision-making. This text explores how we are able to create a hierarchical multi-agent AI system utilizing LangGraph Supervisor to research monetary market traits, carry out sentiment evaluation, and supply funding suggestions. By integrating specialised brokers for market knowledge retrieval, sentiment evaluation, quantitative evaluation, and funding technique formulation, we allow an clever, automated system that mimics the workflow of human monetary analysts.
Studying Goals
- Study hierarchical buildings, supervisor roles, and agent coordination.
- Construct domain-specific, sentiment, and quantitative evaluation brokers.
- Handle agent communication and configure hierarchical workflows.
- Combine AI insights for data-driven suggestions.
- Implement, optimize, and scale AI-driven functions.
- Mitigate biases, guarantee transparency, and improve reliability.
- This module supplies a hands-on method to constructing clever, AI-driven multi-agent techniques utilizing scalable frameworks.
This text was printed as part of the Knowledge Science Blogathon.
Multi-Agent AI System: LangChain Supervisor
Right here’s a easy instance of a supervisor managing two specialised brokers:
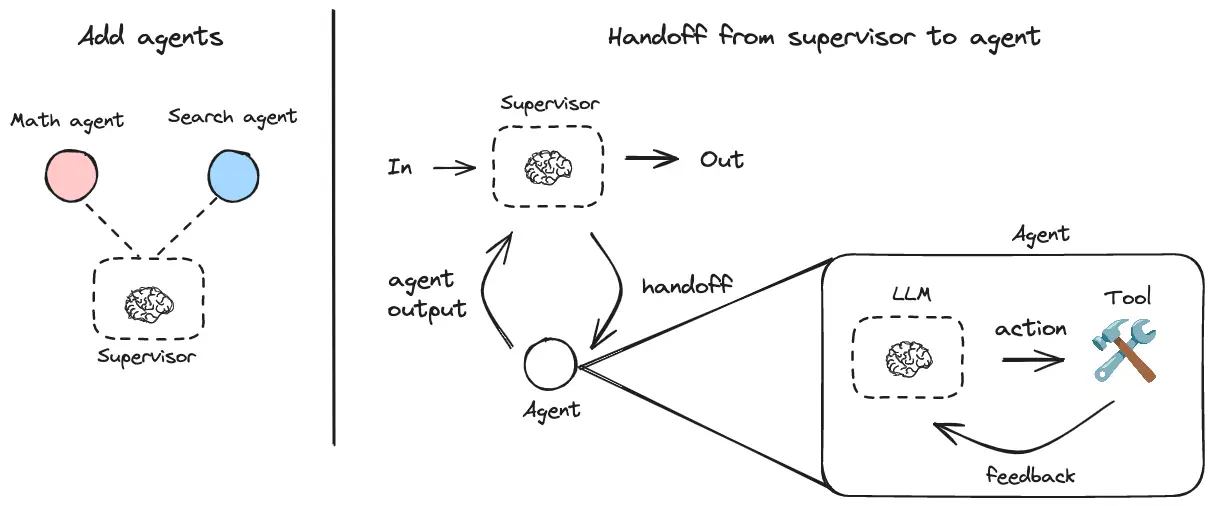
You may management how agent messages are added to the general dialog historical past of the multi-agent system:
Embody full message historical past from an agent:
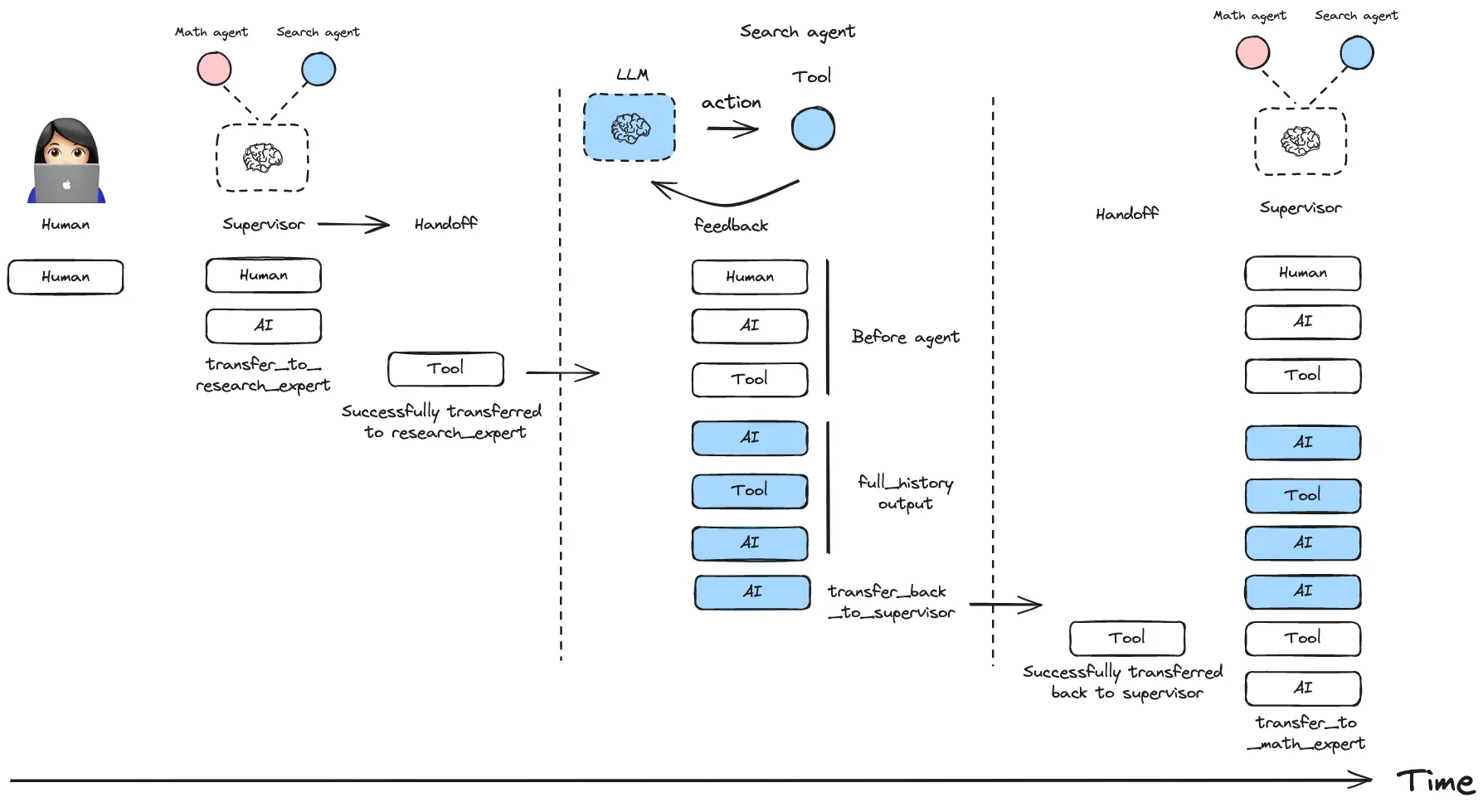
The Multi-Agent Structure
Our system consists of 5 specialised AI brokers working in a coordinated method:
- Market Knowledge Agent (market_data_expert) – Fetches real-time inventory costs, P/E ratios, EPS, and income progress. Answerable for fetching real-time monetary knowledge, together with inventory costs, price-to-earnings (P/E) ratios, earnings per share (EPS), and income progress. Ensures that the system has up-to-date market knowledge for evaluation.
- Sentiment Evaluation Agent (sentiment_expert) – Analyzes information and social media sentiment for shares. Categorizes sentiment as constructive, impartial, or unfavourable to evaluate the market temper towards particular shares.
- Quantitative Analysis Agent (quant_expert) – Computes inventory value traits, shifting averages, and volatility metrics. Helps detect traits, potential breakout factors, and danger ranges primarily based on previous market knowledge.
- Funding Technique Agent (strategy_expert) – Makes use of all obtainable insights to generate a Purchase/Promote/Maintain suggestion. Determines whether or not a inventory needs to be marked as a Purchase, Promote, or Maintain primarily based on calculated dangers and alternatives.
- Supervisor Agent (market_supervisor) – Manages all brokers, making certain easy activity delegation and decision-making. Coordinates multi-agent interactions, screens workflow effectivity and aggregates ultimate suggestions for the person.
Palms-on Multi-Agent AI System for Monetary Market Evaluation
1. Setting Up the Setting
Earlier than implementing the system, set up the required dependencies:
!pip set up langgraph-supervisor langchain-openai
Arrange your OpenAI API key securely:
import os
os.environ["OPENAI_API_KEY"] = "<your_api_key>"
2. Defining Specialised Agent Capabilities
Fetching Market Knowledge
# 1. Fetching Market Knowledge
def fetch_market_data(stock_symbol: str) -> dict:
"""Simulate fetching inventory market knowledge for a given image."""
market_data = {
"AAPL": {"value": 185.22, "pe_ratio": 28.3, "eps": 6.5, "revenue_growth": 8.5},
"GOOG": {"value": 142.11, "pe_ratio": 26.1, "eps": 5.8, "revenue_growth": 7.9},
"TSLA": {"value": 220.34, "pe_ratio": 40.2, "eps": 3.5, "revenue_growth": 6.2},
}
return market_data.get(stock_symbol, {})
Performing Sentiment Evaluation
# 2. Sentiment Evaluation
def analyze_sentiment(stock_symbol: str) -> dict:
"""Carry out sentiment evaluation on monetary information for a inventory."""
sentiment_scores = {
"AAPL": {"news_sentiment": "Constructive", "social_sentiment": "Impartial"},
"GOOG": {"news_sentiment": "Damaging", "social_sentiment": "Constructive"},
"TSLA": {"news_sentiment": "Constructive", "social_sentiment": "Damaging"},
}
return sentiment_scores.get(stock_symbol, {})
Computing Quantitative Evaluation Metrics
# 3. Quantitative Evaluation
def compute_quant_metrics(stock_symbol: str) -> dict:
"""Compute SMA, EMA, and volatility for inventory."""
quant_metrics = {
"AAPL": {"sma_50": 180.5, "ema_50": 182.1, "volatility": 1.9},
"GOOG": {"sma_50": 140.8, "ema_50": 141.3, "volatility": 2.1},
"TSLA": {"sma_50": 215.7, "ema_50": 218.2, "volatility": 3.5},
}
return quant_metrics.get(stock_symbol, {})
Producing Funding Suggestions
# 4. Funding Technique Determination
def investment_strategy(stock_symbol: str, market_data: dict, sentiment: dict, quant: dict) -> str:
"""Analyze knowledge and generate purchase/promote/maintain suggestion."""
if not market_data or not sentiment or not quant:
return "Not sufficient knowledge for suggestion."
choice = "Maintain"
if market_data["pe_ratio"] < 30 and sentiment["news_sentiment"] == "Constructive" and quant["volatility"] < 2:
choice = "Purchase"
elif market_data["pe_ratio"] > 35 or sentiment["news_sentiment"] == "Damaging":
choice = "Promote"
return f"Really useful Motion for {stock_symbol}: {choice}"
3. Creating and Deploying Brokers
import os
from langchain_openai import ChatOpenAI
from langgraph_supervisor import create_supervisor
from langgraph.prebuilt import create_react_agent
# Initialize the Chat mannequin
mannequin = ChatOpenAI(mannequin="gpt-4o")
### --- CREATE AGENTS --- ###
# Market Knowledge Agent
market_data_expert = create_react_agent(
mannequin=mannequin,
instruments=[fetch_market_data],
title="market_data_expert",
immediate="You might be an professional in inventory market knowledge. Fetch inventory knowledge when requested."
)
# Sentiment Evaluation Agent
sentiment_expert = create_react_agent(
mannequin=mannequin,
instruments=[analyze_sentiment],
title="sentiment_expert",
immediate="You analyze monetary information and social media sentiment for inventory symbols."
)
# Quantitative Evaluation Agent
quant_expert = create_react_agent(
mannequin=mannequin,
instruments=[compute_quant_metrics],
title="quant_expert",
immediate="You analyze inventory value traits, shifting averages, and volatility metrics."
)
# Funding Technique Agent
strategy_expert = create_react_agent(
mannequin=mannequin,
instruments=[investment_strategy],
title="strategy_expert",
immediate="You make funding suggestions primarily based on market, sentiment, and quant knowledge."
)
### --- SUPERVISOR AGENT --- ###
market_supervisor = create_supervisor(
brokers=[market_data_expert, sentiment_expert, quant_expert, strategy_expert],
mannequin=mannequin,
immediate=(
"You're a monetary market supervisor managing 4 professional brokers: market knowledge, sentiment, "
"quantitative evaluation, and funding technique. For inventory queries, use market_data_expert. "
"For information/social sentiment, use sentiment_expert. For inventory value evaluation, use quant_expert. "
"For ultimate funding suggestions, use strategy_expert."
)
)
# Compile into an executable workflow
app = market_supervisor.compile()
4. Operating the System
### --- RUN THE SYSTEM --- ###
stock_query = {
"messages": [
{"role": "user", "content": "What is the investment recommendation for AAPL?"}
]
}
# Execute question
end result = app.invoke(stock_query)
print(end result['messages'][-1].content material)

The AI system has analyzed market knowledge, sentiment, and technical indicators to advocate an funding motion
Future Enhancements
- Hook up with Actual APIs (Yahoo Finance, Alpha Vantage) for livestock knowledge.
- Improve Sentiment Evaluation by integrating social media monitoring.
- Develop Portfolio Administration to incorporate danger evaluation and diversification methods.
This multi-agent framework is a scalable AI resolution for monetary evaluation, able to real-time funding decision-making with minimal human intervention!
Key Takeaways
- A multi-agent AI system automates market development evaluation, sentiment analysis, and funding suggestions.
- Specialised brokers deal with market knowledge, sentiment evaluation, quantitative metrics, and funding technique, managed by a supervisor agent.
- The system is constructed utilizing LangGraph Supervisor, defining agent capabilities, deploying them, and working funding queries.
- The multi-agent method enhances modularity, scalability, automation, and accuracy in monetary decision-making.
- Integration of real-time monetary APIs, superior sentiment monitoring, and portfolio administration for a extra complete AI-powered funding system.
The media proven on this article is just not owned by Analytics Vidhya and is used on the Creator’s discretion.
Ceaselessly Requested Questions
Ans. LangGraph Supervisor is a Python library that helps you create hierarchical multi-agent techniques. You may outline specialised brokers and have a central supervisor orchestrate their interactions and duties.
Ans. The supervisor agent makes use of a guiding immediate and the content material of the person’s request to find out which specialised agent can fulfill the question. For instance, if a question requires fetching knowledge, the supervisor will go it to a market knowledge agent. If it includes sentiment evaluation, it delegates to the sentiment evaluation agent, and so forth.
Ans. Sure. You may substitute the simulated capabilities (like fetch_market_data) with precise API calls to providers comparable to Yahoo Finance, Alpha Vantage, or another monetary knowledge supplier.
Ans. A multi-agent structure permits every agent to give attention to a specialised activity (e.g., market knowledge retrieval, sentiment evaluation). This modular method is less complicated to scale and keep, and you may reuse or substitute particular person brokers with out overhauling the whole system.
Ans. LangGraph Supervisor can retailer dialog historical past and agent responses. You may configure it to keep up full agent dialog transcripts or simply ultimate responses. For superior use circumstances, you may combine short-term or long-term reminiscence in order that brokers can discuss with previous interactions.