Creating AI brokers that may work together with the true world is a superb space of analysis and growth. One helpful software is constructing brokers able to looking the online to assemble info and full duties. This weblog put up will information you thru the method of making such an agent utilizing LangChain, a framework for creating LLM-powered purposes, and Llama 3.3, a state-of-the-art giant language mannequin.
Studying Goals
- Perceive construct an AI agent utilizing LangChain and Llama 3.3 for internet looking duties.
- Discover ways to combine exterior data sources like ArXiv and Wikipedia right into a web-searching agent.
- Acquire hands-on expertise establishing the setting and mandatory instruments to develop an AI-powered internet app.
- Discover the function of modularity and error dealing with in creating dependable AI-driven purposes.
- Perceive use Streamlit for constructing consumer interfaces that work together seamlessly with AI brokers.
This text was revealed as part of the Knowledge Science Blogathon.
What’s Llama 3.3?
Llama 3.3 is a 70-billion parameter, instruction-tuned giant language mannequin developed by Meta. It’s optimized to carry out higher on text-based duties, together with following directions, coding, and multilingual processing. Compared, it outperforms its forerunners, together with Llama 3.1 70B and Llama 3.2 90B. It might probably even compete with bigger fashions, resembling Llama 3.1 405B in some areas, whereas saving prices.
Options of Llama 3.3
- Instruction Tuning: Llama 3.3 is fine-tuned to comply with directions precisely, making it extremely efficient for duties that require precision.
- Multilingual Help: The mannequin helps a number of languages, together with English, Spanish, French, German, Hindi, Portuguese, Italian, and Thai, enhancing its versatility in various linguistic contexts.
- Value Effectivity: With aggressive pricing, Llama 3.3 could be an reasonably priced answer for builders who need high-performance language fashions with out prohibitive prices.
- Accessibility: Its optimized structure permits it to be deployed on quite a lot of {hardware} configurations, together with CPUs, making it accessible for a variety of purposes.
What’s LangChain?
LangChain is an open-source framework, helpful for constructing purposes powered by giant language fashions (LLMs). The suite of instruments and abstractions simplifies the combination of LLMs in numerous purposes, enabling builders to create refined AI-driven options with ease.
Key Options of LangChain
- Chainable Parts: LangChain permits builders to create advanced workflows by chaining collectively totally different parts or instruments, facilitating the event of intricate purposes.
- Software Integration: It helps the incorporation of assorted instruments and APIs, enabling the event of brokers able to interacting with exterior techniques simply.
- Reminiscence Administration: LangChain gives mechanisms for managing conversational context, permitting brokers to take care of state throughout interactions.
- Extensibility: The framework accommodates customized parts and integrations as wanted, making it simply extendable.
Core Parts of a Net-Looking Agent
Our web-searching agent will include the next key parts:
- LLM (Llama 3.3): The mind of the agent, accountable for understanding consumer queries, producing search queries, and processing the outcomes.
- Search Software: A instrument that enables the agent to carry out internet searches. We’ll use a search engine API for this function.
- Immediate Template: A template that buildings the enter supplied to the LLM, guaranteeing it receives the required info within the right format.
- Agent Executor: The element that orchestrates the interplay between the LLM and the instruments, executing the agent’s actions.
Circulation Diagram
This workflow outlines the method of integrating a number of instruments and fashions to create an interactive system utilizing Arxiv, Wikipedia, and ChatGroq, powered by Streamlit. It begins by loading mandatory API keys and establishing the required instruments. The consumer is then prompted to enter their question, which is saved within the session state. The ChatGroq mannequin processes this enter whereas search instruments collect related info from Arxiv and Wikipedia. The search agent combines the responses and shows a related reply to the consumer.
The method ensures seamless consumer interplay by initializing the instruments, managing consumer enter, and offering correct responses. In case of errors, the system handles them gracefully, guaranteeing a clean expertise. General, this workflow permits the consumer to effectively obtain responses based mostly on real-time information, leveraging highly effective search and AI fashions for a wealthy and informative dialog.
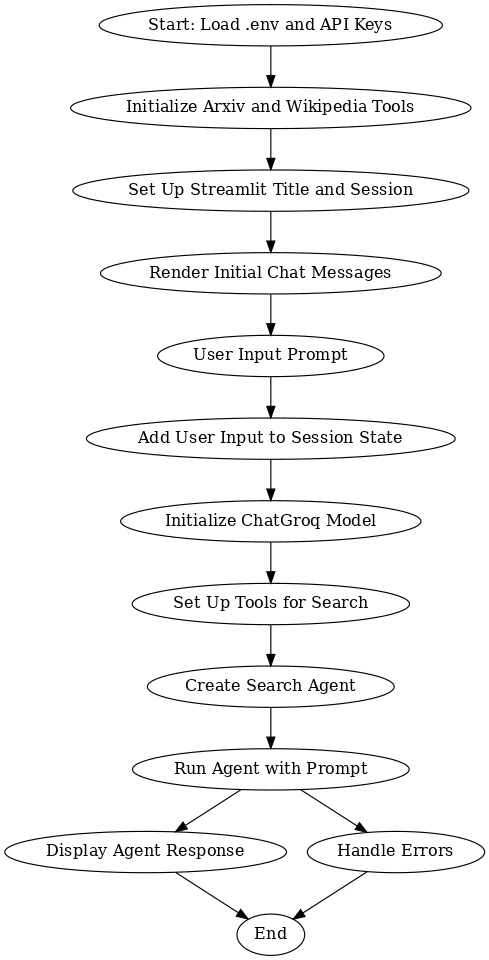
Setting Up the Base
Establishing the bottom is the primary essential step in getting ready your setting for environment friendly processing and interplay with the instruments and fashions required for the duty.
Setting Setup
Setting setup includes configuring the required instruments, API keys, and settings to make sure a clean workflow for the undertaking.
# Create a Setting
python -m venv env
# Activate it on Home windows
.envScriptsactivate
# Activate in MacOS/Linux
supply env/bin/activate
Set up the Necessities.txt
pip set up -r https://uncooked.githubusercontent.com/Gouravlohar/Search-Agent/refs/heads/grasp/necessities.txt
API Key Setup
Make a .env file in your undertaking and go to Groq for API Key.
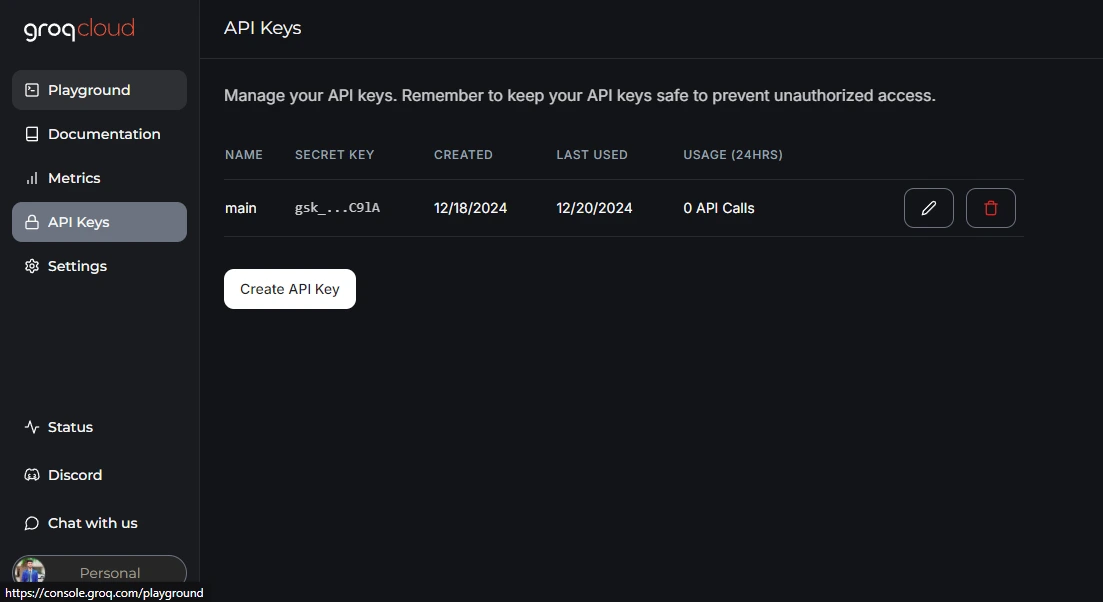
After getting your API Key paste it in your .env file
GROQ_API_KEY="Your API KEY PASTE HERE"
Step1: Importing Essential Libraries
import streamlit as st
from langchain_groq import ChatGroq
from langchain_community.utilities import ArxivAPIWrapper, WikipediaAPIWrapper
from langchain_community.instruments import ArxivQueryRun, WikipediaQueryRun
from langchain.brokers import initialize_agent, AgentType
from langchain_community.callbacks.streamlit import StreamlitCallbackHandler # Up to date import
import os
from dotenv import load_dotenv
Import libraries to construct the Webapp, work together with Llama 3.3, question ArXiv and Wikipedia instruments, initialize brokers, deal with callbacks, and handle setting variables.
Step2: Loading Setting Variables
load_dotenv()
api_key = os.getenv("GROQ_API_KEY")
- load_dotenv(): Fetch setting variables from .env file within the undertaking listing. It is a safe approach to handle delicate information.
- os.getenv(“GROQ_API_KEY”): Retrieves the GROQ_API_KEY from the setting(.env File), which is important to authenticate requests to Groq APIs.
Step3: Setting Up ArXiv and Wikipedia Instruments
arxiv_wrapper = ArxivAPIWrapper(top_k_results=1, doc_content_chars_max=200)
arxiv = ArxivQueryRun(api_wrapper=arxiv_wrapper)
api_wrapper = WikipediaAPIWrapper(top_k_results=1, doc_content_chars_max=200)
wiki = WikipediaQueryRun(api_wrapper=api_wrapper)
- top_k_results=1: Specifies that solely the highest consequence ought to be retrieved.
- doc_content_chars_max=200: It would limits the size of the retrieved doc content material to 200 characters for summaries.
- ArxivQueryRun and WikipediaQueryRun: Join the wrappers to their respective querying mechanisms, enabling the agent to execute searches and retrieve outcomes effectively.
Step4: Setting the App Title
st.title("🔎Search Net with Llama 3.3")
Set a user-friendly title for the online app to point its function.
Step5: Initializing Session State
if "messages" not in st.session_state:
st.session_state["messages"] = [
{"role": "assistant", "content": "Hi, I can search the web. How can I help you?"}
]
Arrange a session state to retailer messages and make sure the chat historical past persists all through the consumer session.
Step6: Displaying Chat Messages
for msg in st.session_state.messages:
st.chat_message(msg["role"]).write(msg['content'])
- st.chat_message(): It shows messages within the chat interface. The msg[“role”] determines whether or not the message is from the “consumer” or the “assistant.”
Step7: Dealing with Consumer Enter
if immediate := st.chat_input(placeholder="Enter Your Query Right here"):
st.session_state.messages.append({"function": "consumer", "content material": immediate})
st.chat_message("consumer").write(immediate)
- st.chat_input(): Creates an enter area for the consumer to enter their query.
- Including Consumer Messages: Appends the consumer’s inquiries to the session state and shows it within the chat interface.
Step8: Initializing the Language Mannequin
llm = ChatGroq(groq_api_key=api_key, model_name="llama-3.3-70b-versatile", streaming=True)
instruments = [arxiv, wiki]
- groq_api_key: Makes use of the API key to authenticate.
- model_name: Specifies the Llama 3.3 mannequin variant to make use of.
- streaming=True: Allows real-time response era within the chat window.
- Instruments: Consists of the ArXiv and Wikipedia querying instruments, making them out there for the agent to make use of.
Step9: Initializing the Search Agent
search_agent = initialize_agent(instruments, llm, agent=AgentType.ZERO_SHOT_REACT_DESCRIPTION, handle_parsing_errors=True)
Mix each the instruments and the language mannequin to create a zero-shot agent able to performing internet searches and offering solutions.
Step10: Producing the Assistant’s Response
with st.chat_message("assistant"):
st_cb = StreamlitCallbackHandler(st.container(), expand_new_thoughts=False)
attempt:
response = search_agent.run([{"role": "user", "content": prompt}], callbacks=[st_cb])
st.session_state.messages.append({'function': 'assistant', "content material": response})
st.write(response)
besides ValueError as e:
st.error(f"An error occurred: {e}")
- st.chat_message(“assistant”): Shows the assistant’s response within the chat interface.
- StreamlitCallbackHandler: Manages how intermediate steps or ideas are displayed in Streamlit.
- search_agent.run(): Executes the agent’s reasoning and instruments to generate a response.
- Enter: A formatted listing containing the consumer’s immediate.
Ensures the app handles points (e.g., invalid responses) gracefully, displaying an error message whennecessary.
Output
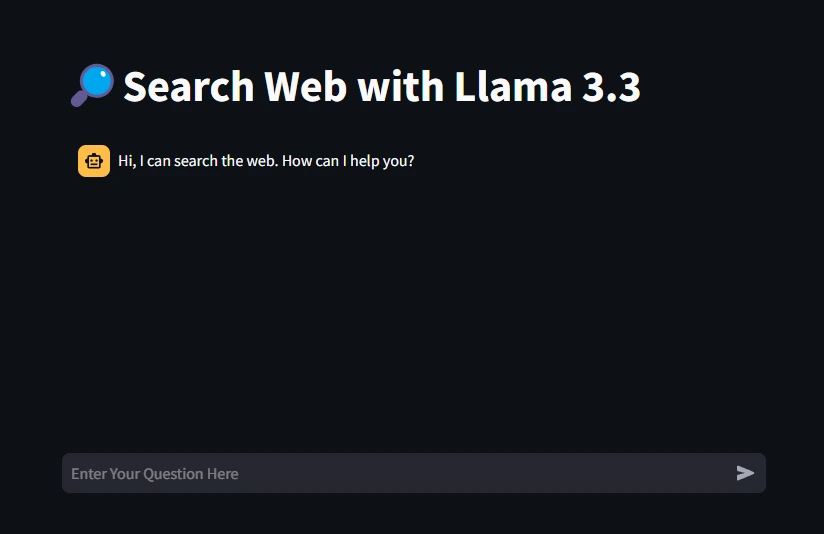
Testing the Webapp
- Enter: What’s Gradient Descent Algorithm
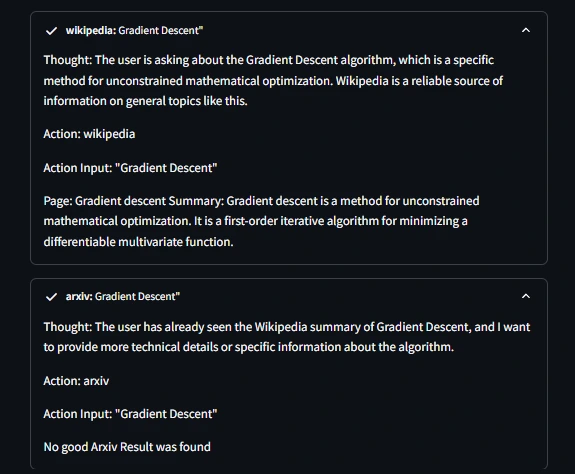
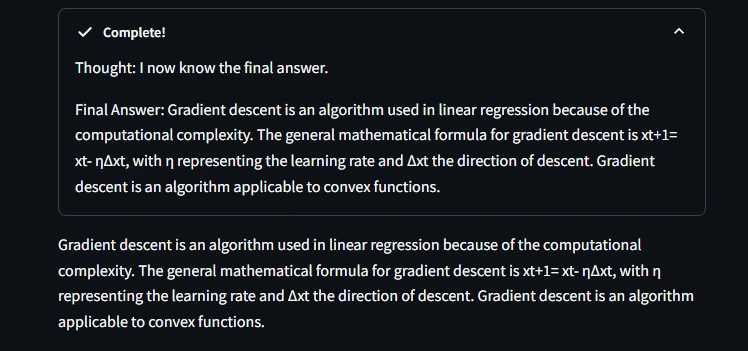
The Agent is offering thought output between each the instruments
Get the Full Code in GitHub Repo Right here
Conclusion
Constructing a web-searching agent with LangChain and Llama 3.3 demonstrates how the mixture of cutting-edge AI with exterior data sources resembling ArXiv and Wikipedia can energy real-world purposes that bridge the hole between conversational AI and real-world purposes. Customers can effortlessly retrieve correct, context-aware info with this method. The undertaking makes use of instruments like Streamlit for consumer interplay and setting variables for safety to offer a seamless and safe expertise for customers.
The modular design permits straightforward scoping, adapting the whole system to domains and even use instances. This modularity, particularly in our AI-driven brokers like this instance, is how we create great steps towards extra clever techniques that increase human capacities for capabilities in analysis and schooling; in actual fact, method additional than that. This analysis serves as the bottom platform for constructing even smarter interactive instruments that seize the excessive potential of AI in searching for data.
Key Takeaways
- This undertaking demonstrates mix a language mannequin like Llama 3.3 with instruments like ArXiv and Wikipedia to create a strong web-searching agent.
- Streamlit gives a easy approach to construct and deploy interactive internet apps, making it excellent for chat-based instruments.
- Setting variables hold delicate credentials, like API keys, safe by stopping publicity within the codebase.
- It would deal with parsing errors in the AI brokers, guaranteeing a greater reliability and consumer expertise within the software.
- This method ensures modularity by wrappers, question instruments, and LangChain brokers, permitting straightforward extension with further instruments or APIs.
Incessantly Requested Questions
A. Llama 3.3 is a flexible language mannequin able to processing and understanding advanced queries. It’s used for its superior reasoning skills and pure language era.
A. These platforms present entry to analysis papers and Knowledge, making them excellent for a knowledge-enhanced web-searching agent.
A. Streamlit gives an intuitive framework to create a chat interface, enabling seamless interplay between the consumer and the AI agent.
A. Due to the modular nature of LangChain, we are able to combine different instruments to develop the agent’s capabilities.
A. We handle errors utilizing a try-except block, guaranteeing the app gives significant suggestions as an alternative of crashing.
The media proven on this article shouldn’t be owned by Analytics Vidhya and is used on the Writer’s discretion.