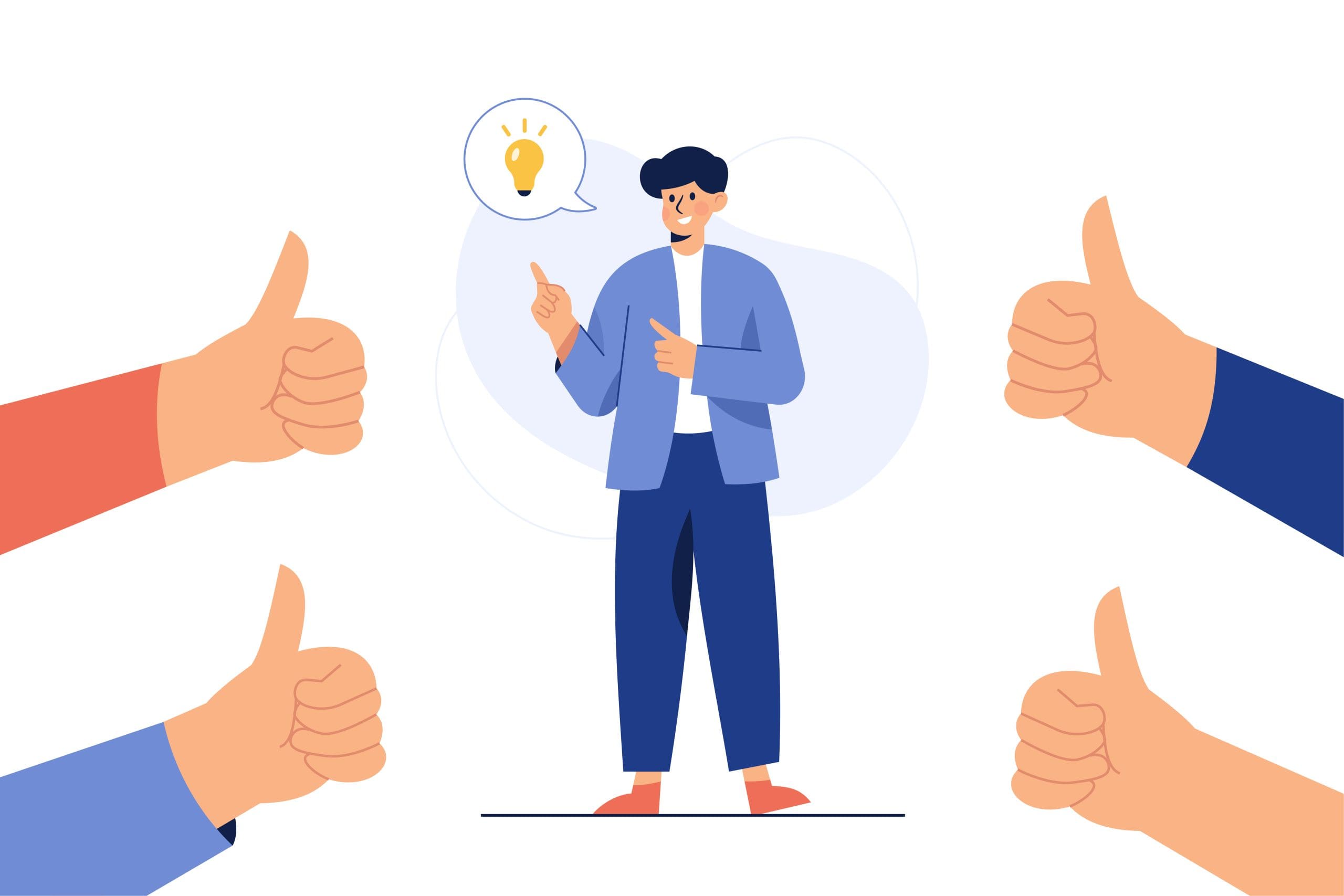
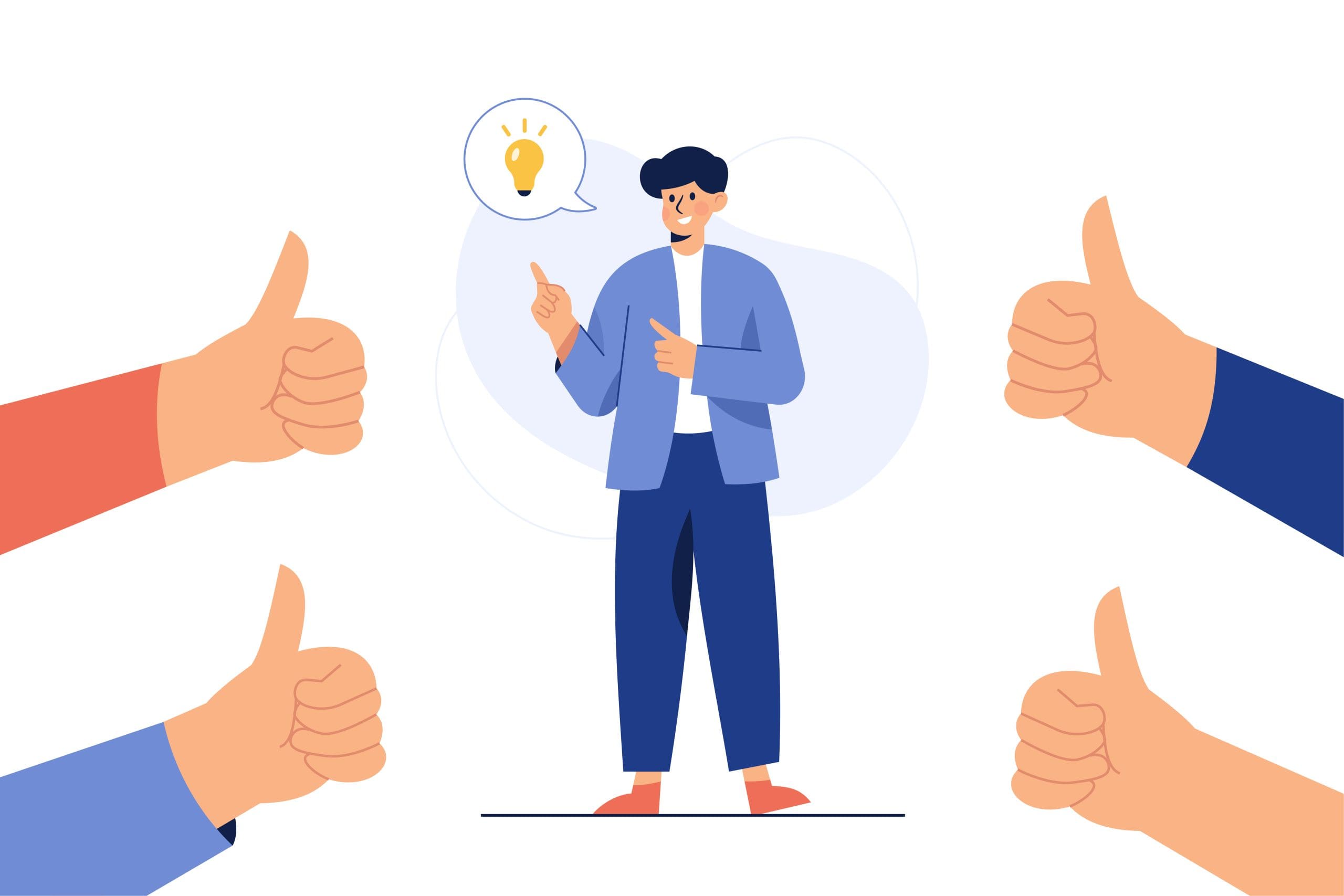
Picture by jcomp on Freepik
We’ve relied on software program in our telephones and computer systems within the trendy period. Many purposes, reminiscent of e-commerce, film streaming, recreation platforms, and others, have modified how we reside, as these purposes make issues simpler. To make issues even higher, the enterprise usually offers options that enable suggestions from the info.
Our High 5 Free Course Suggestions
1. Google Cybersecurity Certificates – Get on the quick monitor to a profession in cybersecurity.
2. Pure Language Processing in TensorFlow – Construct NLP programs
3. Python for All people – Develop applications to collect, clear, analyze, and visualize information
4. Google IT Assist Skilled Certificates
5. AWS Cloud Options Architect – Skilled Certificates
The idea of advice programs is to foretell what the person may fascinated about primarily based on the enter. The system would offer the closest objects primarily based on both the similarity between the objects (content-based filtering) or the habits (collaborative filtering).
With many approaches to the advice system structure, we are able to use the Hugging Face Transformers bundle. In the event you didn’t know, Hugging Face Transformers is an open-source Python bundle that enables APIs to simply entry all of the pre-trained NLP fashions that help duties reminiscent of textual content processing, era, and lots of others.
This text will use the Hugging Face Transformers bundle to develop a easy advice system primarily based on embedding similarity. Let’s get began.
Develop a Suggestion System with Hugging Face Transformers
Earlier than we begin the tutorial, we have to set up the required packages. To try this, you should use the next code:
pip set up transformers torch pandas scikit-learn
You possibly can choose the acceptable model on your atmosphere through their web site for the Torch set up.
As for the dataset instance, we’d use the Anime advice dataset instance from Kaggle.
As soon as the atmosphere and the dataset are prepared, we are going to begin the tutorial. First, we have to learn the dataset and put together them.
import pandas as pd
df = pd.read_csv('anime.csv')
df = df.dropna()
df['description'] = df['name'] +' '+ df['genre'] + ' ' +df['type']+' episodes: '+ df['episodes']
Within the code above, we learn the dataset with Pandas and dropped all of the lacking information. Then, we create a function referred to as “description” that comprises all the knowledge from the accessible information, reminiscent of identify, style, kind, and episode quantity. The brand new column would turn into our foundation for the advice system. It will be higher to have extra full data, such because the anime plot and abstract, however let’s be content material with this one for now.
Subsequent, we’d use Hugging Face Transformers to load an embedding mannequin and rework the textual content right into a numerical vector. Particularly, we’d use sentence embedding to rework the entire sentence.
The advice system could be primarily based on the embedding from all of the anime “description” we are going to carry out quickly. We’d use the cosine similarity technique, which measures the similarity of two vectors. By measuring the similarity between the anime “description” embedding and the person’s question enter embedding, we are able to get exact objects to suggest.
The embedding similarity method sounds easy, however it may be highly effective in comparison with the traditional advice system mannequin, as it could possibly seize the semantic relationship between phrases and supply contextual that means for the advice course of.
We’d use the embedding mannequin sentence transformers from the Hugging Face for this tutorial. To rework the sentence into embedding, we’d use the next code.
from transformers import AutoTokenizer, AutoModel
import torch
import torch.nn.useful as F
def mean_pooling(model_output, attention_mask):
token_embeddings = model_output[0] #First factor of model_output comprises all token embeddings
input_mask_expanded = attention_mask.unsqueeze(-1).develop(token_embeddings.dimension()).float()
return torch.sum(token_embeddings * input_mask_expanded, 1) / torch.clamp(input_mask_expanded.sum(1), min=1e-9)
tokenizer = AutoTokenizer.from_pretrained('sentence-transformers/all-MiniLM-L6-v2')
mannequin = AutoModel.from_pretrained('sentence-transformers/all-MiniLM-L6-v2')
def get_embeddings(sentences):
encoded_input = tokenizer(sentences, padding=True, truncation=True, return_tensors="pt")
with torch.no_grad():
model_output = mannequin(**encoded_input)
sentence_embeddings = mean_pooling(model_output, encoded_input['attention_mask'])
sentence_embeddings = F.normalize(sentence_embeddings, p=2, dim=1)
return sentence_embeddings
Strive the embedding course of and see the vector outcome with the next code. Nevertheless, I might not present the output because it’s fairly lengthy.
sentences = ['Some great movie', 'Another funny movie']
outcome = get_embeddings(sentences)
print("Sentence embeddings:")
print(outcome)
To make issues simpler, Hugging Face maintains a Python bundle for embedding sentence transformers, which might reduce the entire transformation course of in 3 traces of code. Set up the required bundle utilizing the code under.
pip set up -U sentence-transformers
Then, we are able to rework the entire anime “description” with the next code.
from sentence_transformers import SentenceTransformer
mannequin = SentenceTransformer('sentence-transformers/all-MiniLM-L6-v2')
anime_embeddings = mannequin.encode(df['description'].tolist())
With the embedding database is prepared, we’d create a operate to take person enter and carry out cosine similarity as a advice system.
from sklearn.metrics.pairwise import cosine_similarity
def get_recommendations(question, embeddings, df, top_n=5):
query_embedding = mannequin.encode([query])
similarities = cosine_similarity(query_embedding, embeddings)
top_indices = similarities[0].argsort()[-top_n:][::-1]
return df.iloc[top_indices]
Now that every part is prepared, we are able to attempt the advice system. Right here is an instance of buying the highest 5 anime suggestions from the person enter question.
question = "Humorous anime I can watch with pals"
suggestions = get_recommendations(question, anime_embeddings, df)
print(suggestions[['name', 'genre']])
Output>>
identify
7363 Sentou Yousei Shoujo Tasukete! Mave-chan
8140 Anime TV de Hakken! Tamagotchi
4294 SKET Dance: SD Character Flash Anime
1061 Isshuukan Mates.
2850 Oshiete! Galko-chan
style
7363 Comedy, Parody, Sci-Fi, Shounen, Tremendous Energy
8140 Comedy, Fantasy, Children, Slice of Life
4294 Comedy, Faculty, Shounen
1061 Comedy, Faculty, Shounen, Slice of Life
2850 Comedy, Faculty, Slice of Life
The result’s the entire comedy anime, as we wish the humorous anime. Most of them additionally embrace anime, which is appropriate to observe with pals from the style. In fact, the advice could be even higher if we had extra detailed data.
Conclusion
A Suggestion System is a instrument for predicting what customers may be fascinated about primarily based on the enter. Utilizing Hugging Face Transformers, we are able to construct a advice system that makes use of the embedding and cosine similarity method. The embedding method is highly effective as it could possibly account for the textual content’s semantic relationship and contextual that means.
Cornellius Yudha Wijaya is an information science assistant supervisor and information author. Whereas working full-time at Allianz Indonesia, he likes to share Python and information suggestions through social media and writing media. Cornellius writes on a wide range of AI and machine studying matters.