Have you ever ever considered use the facility of synthetic intelligence to make complicated duties simpler? What if you happen to may create AI-driven functions with no need superior technical abilities? With instruments like DeepSeek-V3, a state-of-the-art language mannequin, and Gradio, a easy interface builder, constructing AI options has by no means been extra simple. Whether or not you’re writing YouTube scripts, summarizing authorized paperwork, or planning a visit, this information will present you mix these instruments to create sensible functions.
Studying Aims
- Learn to get hold of your API key and combine it into your Python tasks.
- Create participating and intuitive net interfaces for AI functions, making them accessible to customers with out technical experience utilizing Gradio.
- Crafting prompts that information DeepSeek-V3 to supply desired outputs for various functions.
- Construct hand-on functions that handle sensible issues in content material creation, advertising and marketing, authorized evaluation, sentiment evaluation, and journey planning.
- Perceive how DeepSeek-V3 and Gradio Utilizing Immediate Engineering can streamline AI software growth.
This text was printed as part of the Information Science Blogathon.
Understanding DeepSeek-V3
DeepSeek-V3 is a state-of-the-art language mannequin designed to deal with a variety of complicated pure language processing (NLP) duties. Whether or not it’s good to generate artistic content material, summarize lengthy texts, and even analyze code, DeepSeek-V3 brings a excessive degree of sophistication and nuance to the desk. Its structure leverages superior deep studying methods that permit it to know context, generate coherent responses, and even adapt its tone based mostly on supplied directions. This flexibility makes it excellent for a various set of functions.
Learn extra about Deepseek V3 – right here
Understanding Gradio
Gradio is a strong but easy-to-use python library that enables builders to create interactive net interfaces for machine studying fashions with minimal effort. As an alternative of constructing a person interface from scratch, Gradio allows you to wrap your mannequin in a easy perform and routinely generate a beautiful and user-friendly net app. With its drag-and-drop simplicity, Gradio is the proper companion for DeepSeek-V3, enabling speedy prototyping and seamless deployment of AI functions.
Learn extra about Gradio – right here
All through this information, we’ll break down every software into easy-to-follow steps. This complete method will be sure that even those that are new to AI growth can confidently observe alongside and construct their very own progressive apps.
Stipulations and Setup
Earlier than diving into app growth, guarantee you’ve gotten the next prepared:
Deepseek API Key
- Go to DeepInfra’s official web site, enroll or log in, and navigate to the Dashboard.
- Underneath the “API keys” part, click on on “New API key”, present a reputation, and generate your key.
- Notice: You may view your API key solely as soon as, so retailer it securely.
Set up of Required Dependencies
Open your terminal and run
pip set up gradio openai
It is advisable to set up Gradio and the OpenAI consumer library (which is suitable with DeepSeek-V3) utilizing pip.
Atmosphere Setup
Begin by importing the mandatory libraries and initializing the consumer along with your API key. Additionally, create a brand new Python file, for instance, app.py after which insert the code supplied under.
import openai
import gradio as gr
# Change "YOUR_API_KEY" with the precise API key you obtained from DeepInfra.
API_KEY = "YOUR_API_KEY"
consumer = openai.OpenAI(
api_key=API_KEY,
base_url="https://api.deepinfra.com/v1/openai",
)
YouTube Script Author
The YouTube Script Author app leverages Deepseek-V3’s artistic capabilities to generate detailed video scripts based mostly on a given matter. Good for content material creators in search of inspiration, this app simplifies the script-writing course of by offering a coherent narrative define.
- Initialize the consumer as proven above.
- We outline a perform that calls the DeepSeek-V3 mannequin to generate a YouTube script based mostly on a supplied matter as an skilled YouTube content material creator.
def generate_script(matter: str) -> str:
"""
Generate an in depth YouTube script based mostly on the supplied matter utilizing the DeepSeek-V3 mannequin.
Args:
matter (str): The topic or matter for which the YouTube script is to be generated.
Returns:
str: The generated YouTube script as a string. If an error happens throughout technology, an error message is returned.
"""
strive:
response = consumer.chat.completions.create(
mannequin="deepseek-ai/DeepSeek-V3",
messages=[
{"role": "system", "content": "You are an expert YouTube content creator."},
{"role": "user", "content": f"Write a detailed YouTube script for a video about '{topic}'."}
],
max_tokens=300,
temperature=0.7
)
return response.selections[0].message.content material.strip()
besides Exception as e:
return f"Error: {str(e)}"
- We then create a easy Gradio interface that accepts person enter (a video matter) and shows the generated script.
# Create Gradio interface for the YouTube Script Author
def app_interface(matter: str) -> str:
"""
Generate a YouTube script based mostly on the given matter.
Args:
matter (str): The topic or theme for which the script must be generated.
Returns:
str: The generated YouTube script or an error message if technology fails.
"""
return generate_script(matter)
strive:
interface = gr.Interface(
fn=app_interface,
inputs=gr.Textbox(label="Video Matter"),
outputs=gr.Textbox(label="Generated YouTube Script"),
title="YouTube Script Author"
)
interface.launch()
besides Exception as e:
print(f"Did not launch Gradio interface: {e}")
- When you run your entire code (python app.py) in terminal, it is possible for you to to view the under display screen.
- Give any matter you want and hit Submit. Growth! get a response in a single click on. You may hit flag to save lots of the response in a file regionally.
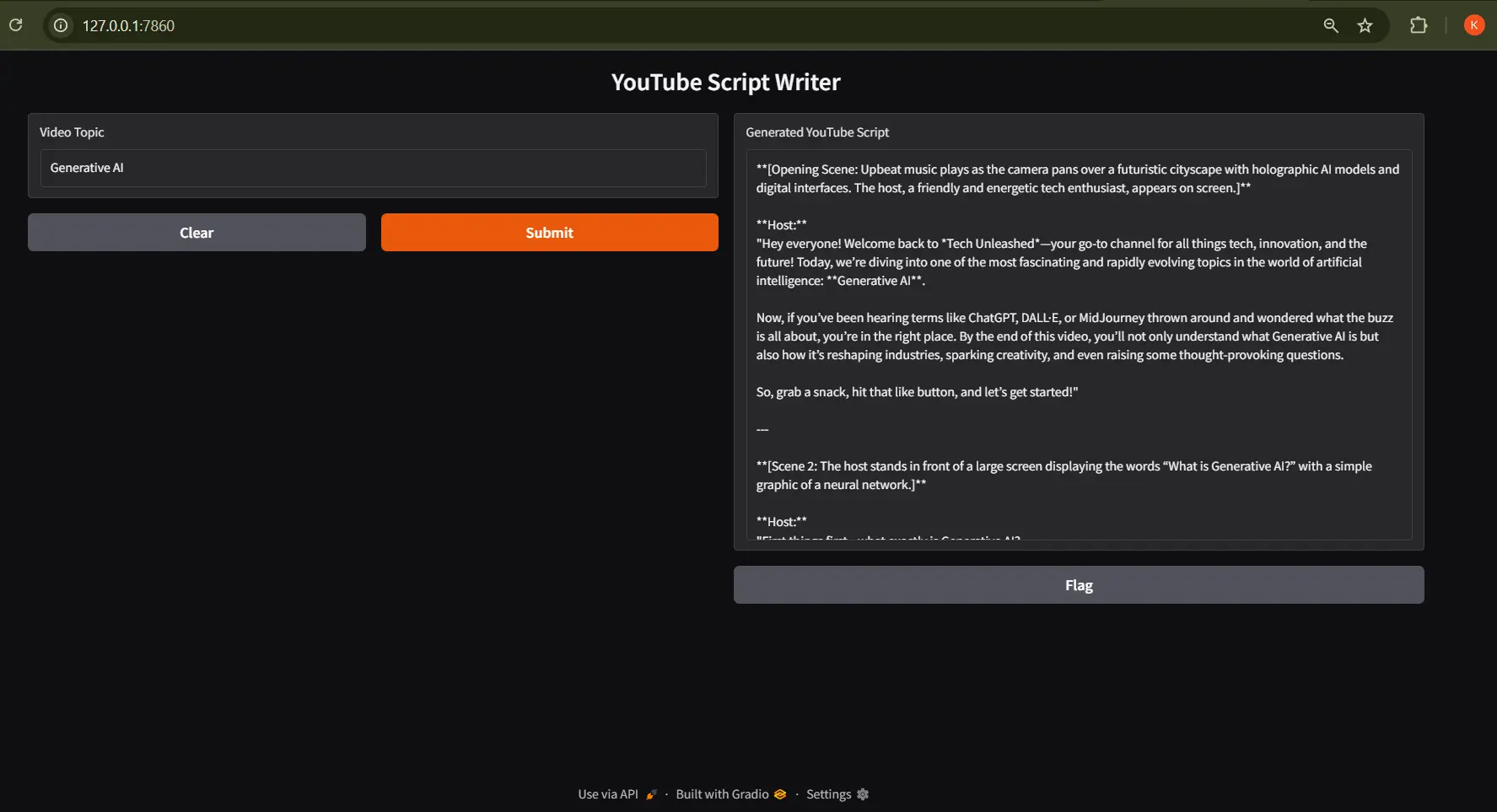
Advertising and marketing AI Assistant
This app is designed to assist advertising and marketing professionals by producing persuasive product descriptions, social media posts, and marketing campaign concepts. Deepseek-V3 is prompted to behave as an skilled advertising and marketing assistant, guaranteeing the generated content material is participating and on level.
- Reuse the beforehand outlined initialization code.
- We now create a perform that sends a immediate to DeepSeek-V3 for producing advertising and marketing copy. We entered an in depth immediate that instructs the mannequin to generate a complete advertising and marketing description for the required product.
def generate_marketing_copy(product: str) -> str:
"""
Generate a advertising and marketing description for a given product.
This perform leverages the DeepSeek-V3 mannequin to create a advertising and marketing copy that features particulars on the target market,
key options, emotional attraction, and a call-to-action for the product.
Args:
product (str): The product for which the advertising and marketing copy must be generated.
Returns:
str: The generated advertising and marketing copy or an error message if an exception happens.
"""
immediate = f"""
Write a advertising and marketing description for {product}. Embody:
- Target market (age, pursuits)
- Key options
- Emotional attraction
- Name-to-action
"""
strive:
response = consumer.chat.completions.create(
mannequin="deepseek-ai/DeepSeek-V3",
messages=[
{"role": "system", "content": "You are a marketing strategist."},
{"role": "user", "content": prompt}
],
max_tokens=300,
)
return response.selections[0].message.content material
besides Exception as e:
return f"Error: {str(e)}"
- We now design the Gradio interface to work together with the advertising and marketing copy perform.
def app_interface(product_name: str) -> str:
"""
Generates a compelling advertising and marketing description for the required product.
Args:
product_name (str): The title of the product for which to generate an outline.
Returns:
str: The generated advertising and marketing description or an error message if technology fails.
"""
immediate = f"Write a compelling advertising and marketing description for the product '{product_name}'."
strive:
return generate_text(immediate)
besides Exception as e:
return f"Error: {str(e)}"
strive:
interface = gr.Interface(
fn=app_interface,
inputs=gr.Textbox(label="Product Title"),
outputs=gr.Textbox(label="Generated Description"),
title="Advertising and marketing AI Assistant"
)
interface.launch()
besides Exception as e:
print(f"Did not launch Gradio interface: {e}")
- When you run your entire code (python app.py) in terminal, it is possible for you to to view the under display screen.
- Enter the Product title and click on undergo get the response.
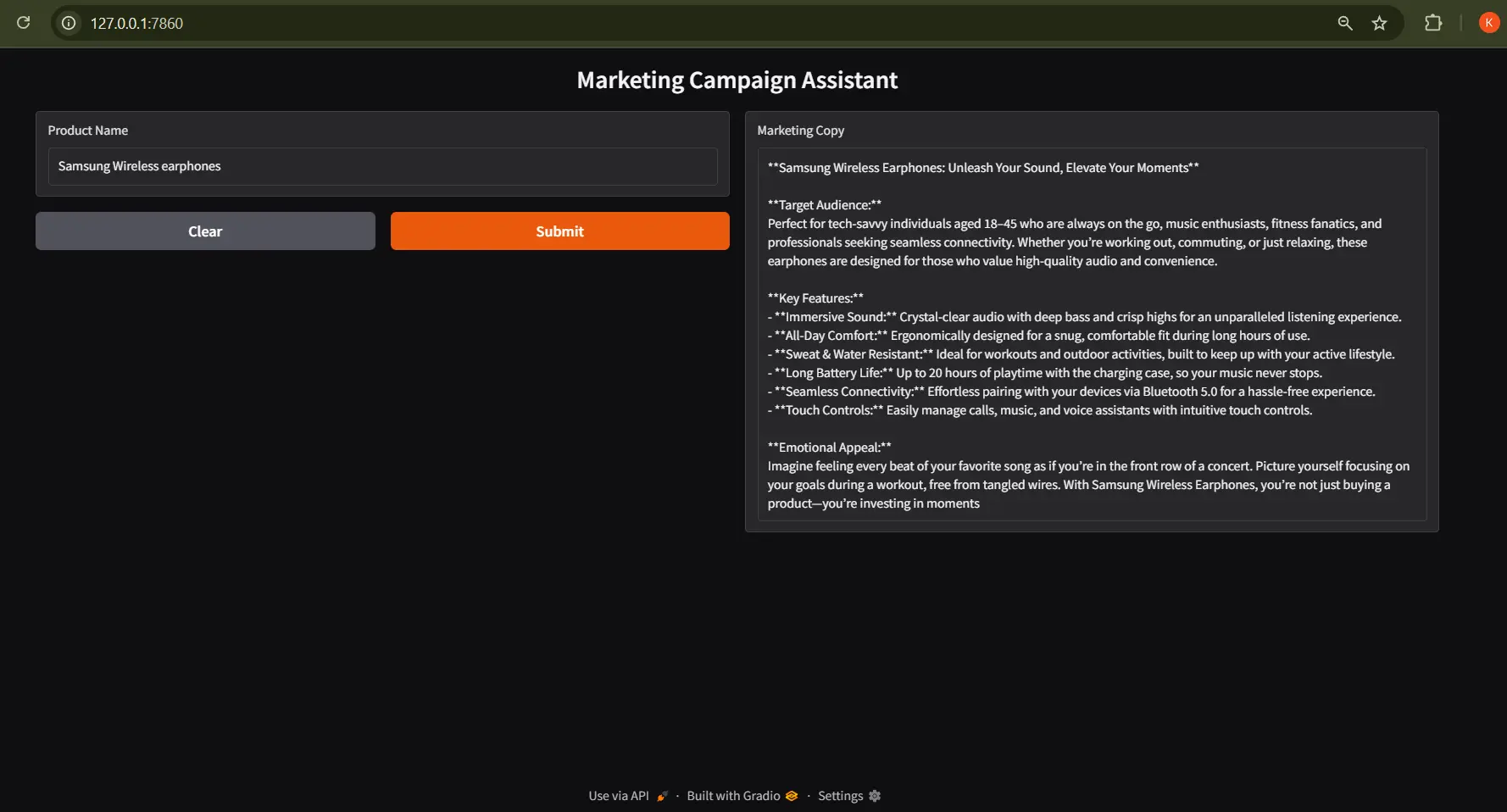
Authorized Doc Summarizer
Authorized paperwork are sometimes dense and stuffed with complicated language. The Authorized Doc Summarizer app simplifies these paperwork by producing a concise abstract, highlighting essential factors similar to obligations, penalties, and termination clauses. This app is especially helpful for authorized professionals and college students who have to rapidly grasp the essence of prolonged paperwork.
- Along with the usual libraries, this app makes use of a PDF studying library similar to PyPDF2. Ensure you set up it if you happen to haven’t already:
pip set up PyPDF2
- Reuse the beforehand outlined initialization code.
- Much like earlier apps, we arrange the API consumer along with your DeepSeek-V3 API key.
- We then create a perform that reads an uploaded PDF, extracts its textual content, after which sends the textual content to DeepSeek-V3 for summarization.
from typing import Any
def summarize_legal_doc(pdf_file: Any) -> str:
"""
Extract and summarize the textual content from a authorized PDF doc.
This perform makes use of PyPDF2 to learn and extract textual content from the supplied PDF file.
The extracted textual content is then despatched to the DeepSeek-V3 mannequin to supply a abstract
within the type of 5 bullet factors, emphasizing obligations, penalties, and termination clauses.
Args:
pdf_file (Any): A file-like object representing the uploaded PDF. It will need to have a 'title' attribute.
Returns:
str: The summarized authorized doc. Returns an error message if no file is supplied or if an error happens.
"""
if pdf_file is None:
return "No file uploaded."
strive:
# Extract textual content from the PDF utilizing PyPDF2
reader = PyPDF2.PdfReader(pdf_file.title)
extracted_text = ""
for web page in reader.pages:
page_text = web page.extract_text()
if page_text:
extracted_text += page_text + "n"
# Put together the messages for the DeepSeek-V3 summarization request
messages = [
{
"role": "system",
"content": (
"Summarize this legal document into 5 bullet points. "
"Highlight obligations, penalties, and termination clauses."
)
},
{
"role": "user",
"content": extracted_text
}
]
# Request the abstract from DeepSeek-V3
response = consumer.chat.completions.create(
mannequin="deepseek-ai/DeepSeek-V3",
messages=messages,
temperature=0.2 # Decrease temperature for factual accuracy
)
return response.selections[0].message.content material.strip()
besides Exception as e:
return f"Error: {str(e)}"
Lastly, we create a Gradio interface that enables customers to add a PDF and examine the generated abstract.
strive:
# Create a Gradio interface
interface = gr.Interface(
fn=summarize_legal_doc,
inputs=gr.File(label="Add PDF Doc"),
outputs=gr.Textbox(label="Abstract"),
title="Authorized Doc Summarizer"
)
# Launch the app
interface.launch()
besides Exception as e:
print(f"Did not launch Gradio interface: {e}")
Run the app.py file within the terminal. This app lets you add the pdf doc and provides the abstract of it.
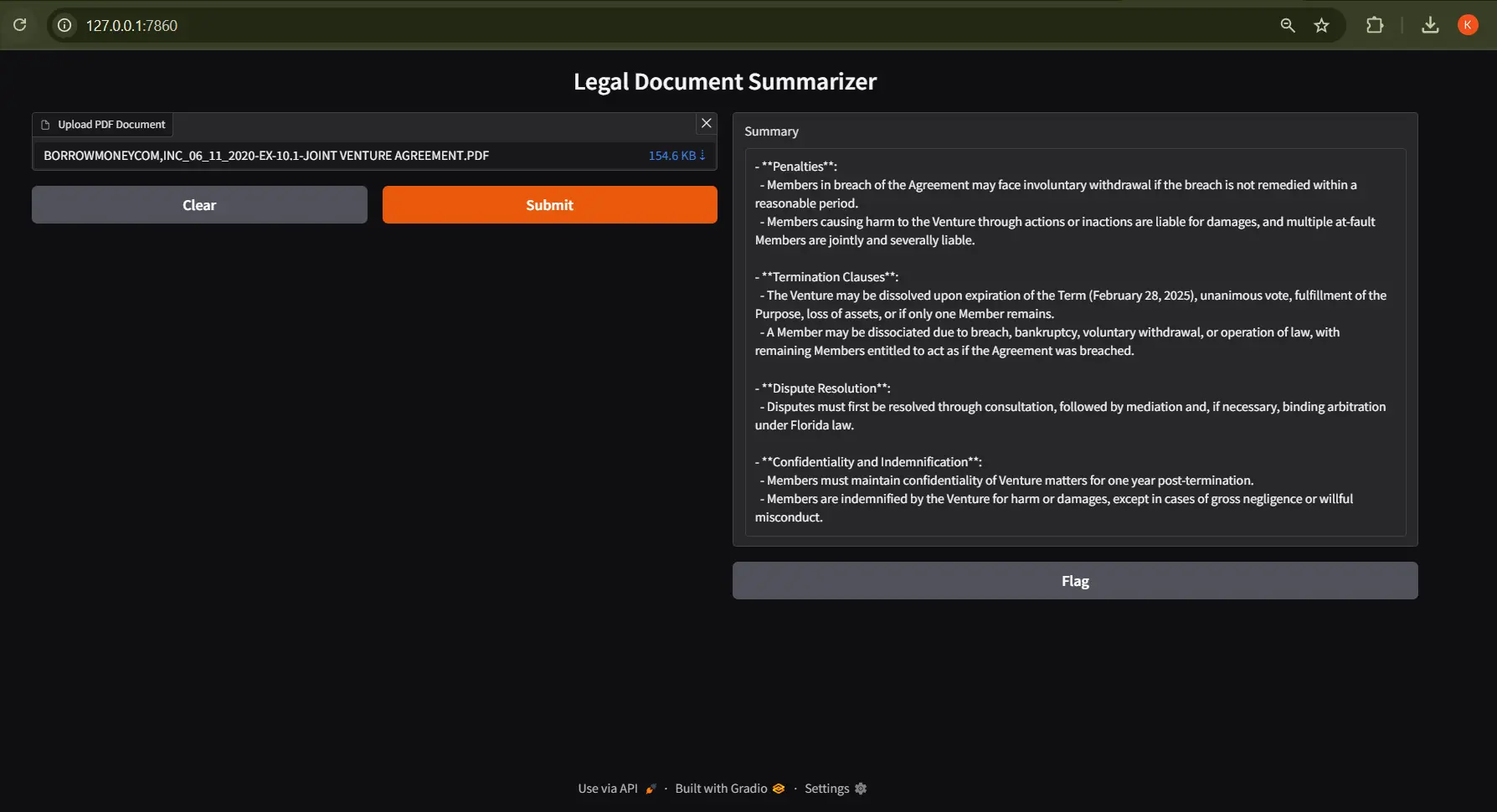
Supply: Used the doc pdf from Kaggle for testing the above app.
Sentiment Evaluation App
Understanding the sentiment behind a chunk of textual content is crucial for gauging public opinion, analyzing buyer suggestions, or monitoring social media tendencies. The Sentiment Evaluation app makes use of DeepSeek-V3 to find out whether or not a given textual content is optimistic, unfavourable, or impartial in a descriptive method.
- Once more, we begin with our customary initialization of the consumer.
- We create a perform that analyzes the sentiment of the enter textual content.
def analyze_sentiment(textual content: str) -> str:
"""
Analyze the sentiment of a given textual content utilizing the DeepSeek-V3 mannequin.
This perform sends the supplied textual content to the DeepSeek-V3 mannequin with a immediate to research sentiment
and returns the evaluation outcome.
Args:
textual content (str): The enter textual content for sentiment evaluation.
Returns:
str: The sentiment evaluation outcome or an error message if an exception is raised.
"""
strive:
response = consumer.chat.completions.create(
mannequin="deepseek-ai/DeepSeek-V3",
messages=[
{"role": "system", "content": "You are an expert in sentiment analysis."},
{"role": "user", "content": f"Analyze the sentiment of this text: {text}"}
],
max_tokens=150,
temperature=0.5
)
return response.selections[0].message.content material.strip()
besides Exception as e:
return f"Error: {str(e)}"
- We construct an interface that enables customers to enter textual content and see the sentiment evaluation. This perform immediately passes the person’s textual content to the sentiment evaluation perform.
def sentiment_interface(textual content: str) -> str:
"""
Function an interface perform to research sentiment of the supplied textual content.
Args:
textual content (str): The enter textual content to be analyzed.
Returns:
str: The results of the sentiment evaluation.
"""
return analyze_sentiment(textual content)
strive:
interface = gr.Interface(
fn=sentiment_interface,
inputs=gr.Textbox(label="Enter Textual content"),
outputs=gr.Textbox(label="Sentiment Evaluation"),
title="Sentiment Evaluation App"
)
interface.launch()
besides Exception as e:
print(f"Did not launch Gradio interface: {e}")
- As soon as the app is launched, you’ll be able to insert the textual content and get the response.
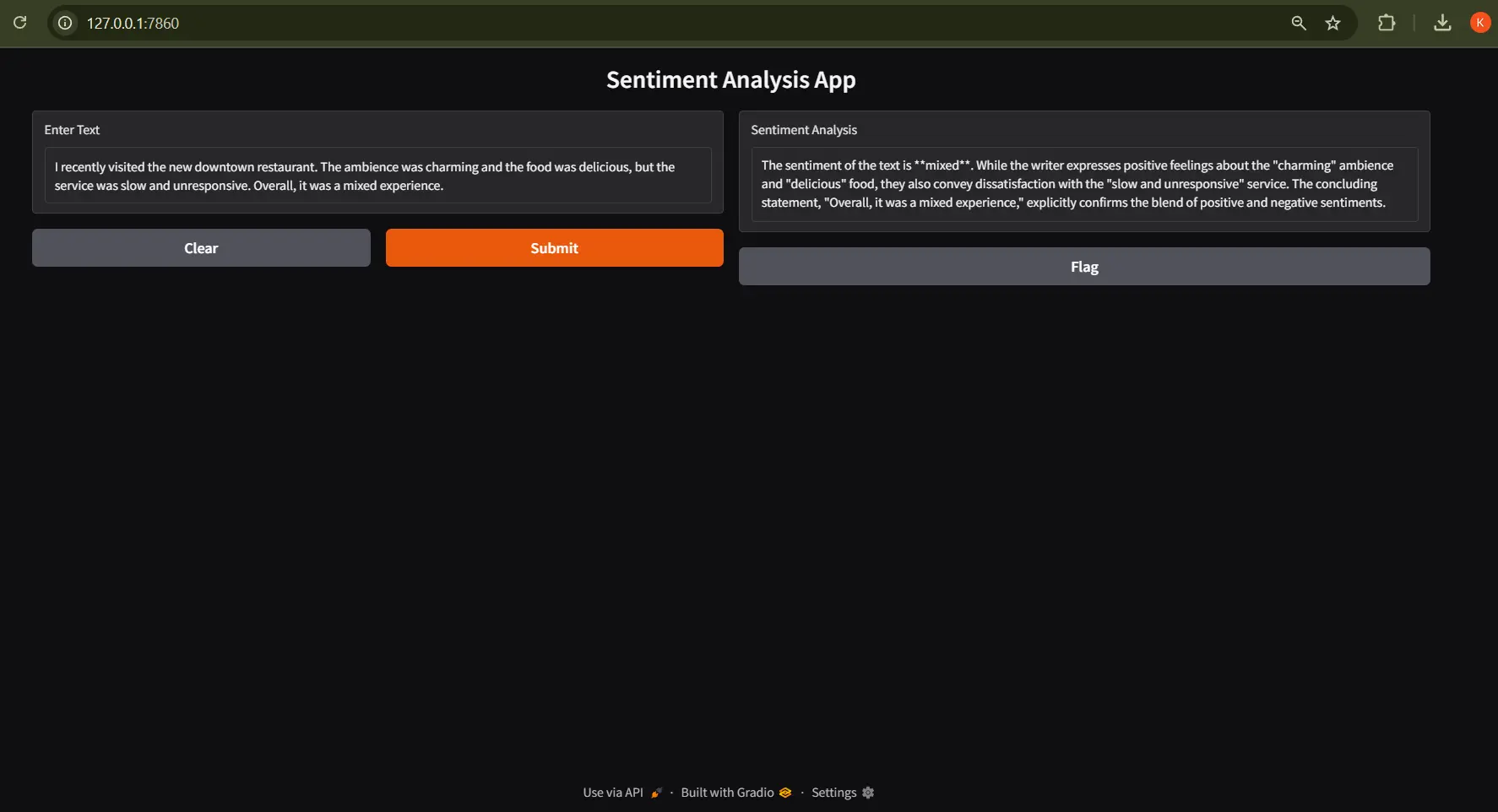
Journey Itinerary Planner
Planning a visit might be overwhelming, however the Journey Itinerary Planner app simplifies the method by producing detailed itineraries based mostly on person enter. By specifying a vacation spot, the variety of days, and pursuits, customers obtain a customized journey plan full with exercise ideas, native delicacies suggestions, and transportation suggestions.
- Use the usual initialization code as soon as extra.
- We create a perform that constructs an in depth journey itinerary based mostly on person inputs. We assemble a immediate that features all mandatory particulars (vacation spot, length, pursuits) and particular directions to incorporate totally different components of the day, delicacies suggestions, and transportation suggestions.
def plan_itinerary(vacation spot: str, days: int, pursuits: str) -> str:
"""
Generate a journey itinerary for the required vacation spot, length, and pursuits.
This perform makes use of the DeepSeek-V3 mannequin to create an in depth journey itinerary that features actions for various occasions of the day,
native delicacies suggestions, and transportation suggestions.
Args:
vacation spot (str): The vacation spot for the journey itinerary.
days (int): The variety of days for the itinerary.
pursuits (str): The journey pursuits (e.g., "Journey", "Cultural", "Rest").
Returns:
str: The generated itinerary textual content, or an error message if an exception happens.
"""
immediate = f"""
Create a {days}-day itinerary for {vacation spot}. Give attention to {pursuits}.
Embody:
- Morning/Afternoon/Night actions
- Native delicacies suggestions
- Transportation suggestions
"""
strive:
response = consumer.chat.completions.create(
mannequin="deepseek-ai/DeepSeek-V3",
messages=[
{"role": "system", "content": "You are a travel expert."},
{"role": "user", "content": prompt}
],
max_tokens=500
)
return response.selections[0].message.content material.strip()
besides Exception as e:
return f"Error: {str(e)}"
- Shows the generated itinerary in a textbox and launches the interface as an internet app. The interface for the itinerary planner is designed to seize a number of inputs from the person.
strive:
interface = gr.Interface(
fn=plan_itinerary,
inputs=[
gr.Textbox(label="Destination"),
gr.Number(label="Number of Days"),
gr.Dropdown(["Adventure", "Cultural", "Relaxation"], label="Pursuits")
],
outputs=gr.Textbox(label="Itinerary"),
title="Journey Itinerary Planner"
)
interface.launch()
besides Exception as e:
print(f"Did not launch Gradio interface: {e}")
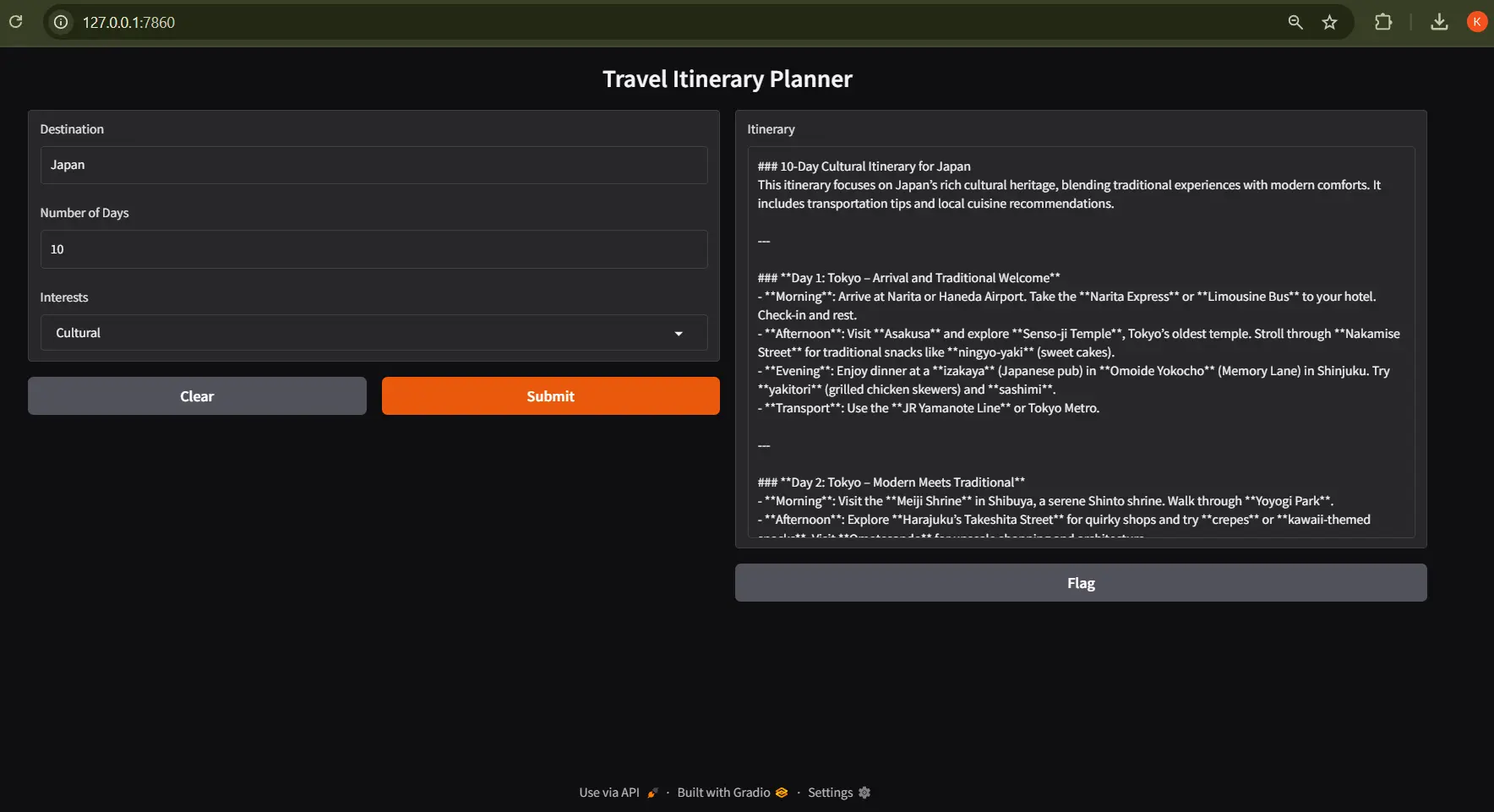
Greatest Practices for Constructing AI Apps
As you’re employed via these tasks, take into account the next finest practices to make sure your apps are sturdy and maintainable:
- Error Dealing with: Use try-except blocks in your features to handle API errors gracefully.
- Tweek parameters: Play with totally different parameters to get the specified output.
- Caching Responses: To reduce repeated API calls and cut back latency, take into account caching responses the place relevant.
- Person Suggestions: Embody clear messages for customers when errors happen or when the app is processing their requests.
- Safety: All the time hold your API keys safe and don’t expose them in public repositories.
Conclusion
By integrating DeepSeek-V3 and Gradio Utilizing Immediate Engineering, builders can unlock a world of potentialities in AI software growth. This information has supplied an intensive walkthrough of constructing 5 distinct AI apps that cater to numerous domains. Whether or not you’re a seasoned developer or simply beginning out, these instruments empower you to experiment, innovate, and produce your concepts to life rapidly. As AI know-how continues to evolve, the instruments and methods mentioned right here will solely develop into extra highly effective. Embracing these improvements at present prepares you for a future the place AI-driven options are integral to all elements of labor and life.
Additionally learn: 7 real-world functions of deepseek-v3
Key Takeaways
- Leveraging DeepSeek-V3 and Gradio Utilizing Immediate Engineering permits builders to construct AI-driven functions with ease, enabling seamless pure language processing and intuitive AI deployment.
- Every app is constructed step-by-step with detailed explanations, making it accessible to rookies whereas nonetheless providing sturdy options for superior customers.
- The information covers a variety of functions from artistic content material technology and advertising and marketing to authorized summarization and journey planning by demonstrating the broad applicability of AI.
- Tailor parameters, system prompts and interface components to swimsuit particular necessities and scale your options in real-world eventualities.
- With modular code and detailed prompts, these apps might be simply personalized and scaled to satisfy particular necessities or built-in into bigger programs.
Ceaselessly Requested Questions
A: DeepSeek-V3 provides a free tier with particular fee limits. For extra superior utilization, please confer with DeepInfra’s pricing web page for particulars.
A: Completely. One of many key strengths of DeepSeek-V3 is its adaptability. You may modify the system and person messages to tailor the output for various functions or industries.
A: Whereas the supplied code serves as a robust basis, additional testing, optimization, and safety enhancements are beneficial earlier than deploying in a manufacturing surroundings.
A: Sure, the modular design of those functions permits for simple integration with different net frameworks like Streamlit and many others, cell apps, or enterprise programs.
The media proven on this article will not be owned by Analytics Vidhya and is used on the Writer’s discretion.
Login to proceed studying and revel in expert-curated content material.