Within the fast-paced digital panorama, companies usually face the problem of promptly responding to buyer emails whereas sustaining accuracy and relevance. Leveraging superior instruments like LangGraph, Llama 3, and Groq, we are able to streamline electronic mail workflows by automating duties equivalent to categorization, contextual analysis, and drafting considerate replies. This information demonstrates the way to construct an automatic system to deal with these duties successfully, together with integration with serps and APIs for seamless operations.
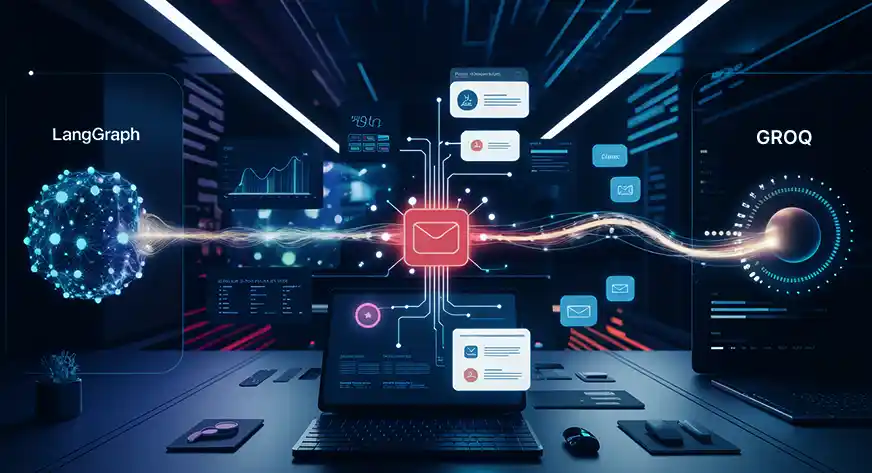
Studying Aims
- Discover ways to outline, handle, and execute multi-step workflows utilizing LangGraph, together with the usage of nodes, edges, and conditional routing.
- Discover the method of incorporating exterior API like GROQ and internet search instruments into LangGraph workflows to reinforce performance and streamline operations.
- Achieve insights into managing shared states throughout workflow steps, together with passing inputs, monitoring intermediate outcomes, and guaranteeing constant outputs.
- Perceive the significance of intermediate evaluation , suggestions loops, and refinement in producing high-quality outputs utilizing giant language fashions(LLMs).
- Discover ways to implement conditional logic for routing duties, dealing with errors, and adapting workflows dynamically primarily based on intermediate outcomes.
This text was printed as part of the Information Science Blogathon.
Getting Began: Setup and Set up
Begin by putting in the mandatory Python libraries for this challenge. These instruments will enable us to create a extremely practical and clever electronic mail reply system.
!pip -q set up langchain-groq duckduckgo-search
!pip -q set up -U langchain_community tiktoken langchainhub
!pip -q set up -U langchain langgraph tavily-python
You possibly can affirm the profitable set up of langgraph by working:
!pip present langgraph
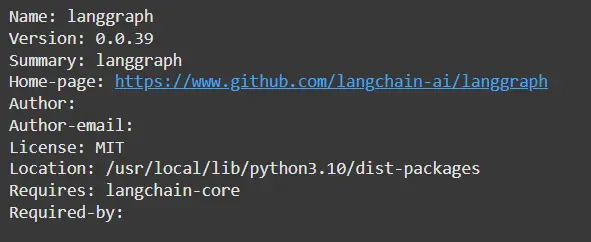
Purpose
The system goals to automate electronic mail replies utilizing a step-by-step course of:
- Retrieve the incoming electronic mail.
- Categorize the e-mail into one of many following sorts:
- Gross sales
- Customized Inquiry
- Off-topic
- Buyer Criticism
- Generate key phrases for related analysis primarily based on the e-mail class and content material.
- Draft a reply utilizing researched info.
- Validate the draft reply.
- Rewrite the reply if obligatory.
This structured strategy ensures the e-mail response is tailor-made, correct, {and professional}.
Setting Up the Atmosphere
To proceed, arrange the atmosphere with the required API keys:
import os
from google.colab import userdata
from pprint import pprint
os.environ["GROQ_API_KEY"] = userdata.get('GROQ_API_KEY')
os.environ["TAVILY_API_KEY"] = userdata.get('TAVILY_API_KEY')
Implementing an Superior E mail Reply System
To energy the e-mail processing pipeline, we use Groq’s Llama3-70b-8192 mannequin. This mannequin is very able to dealing with advanced duties like pure language understanding and technology.
from langchain_groq import ChatGroq
GROQ_LLM = ChatGroq(
mannequin="llama3-70b-8192",
)
The Groq-powered LLM acts because the spine for categorizing emails, producing analysis key phrases, and drafting polished replies.
Constructing Immediate Templates
Utilizing LangChain’s ChatPromptTemplate and output parsers, we outline templates for producing and decoding outcomes. These templates be sure that the mannequin’s output aligns with our system’s necessities.
from langchain_core.prompts import ChatPromptTemplate
from langchain.prompts import PromptTemplate
from langchain_core.output_parsers import StrOutputParser
from langchain_core.output_parsers import JsonOutputParser
These instruments present the pliability wanted to deal with each free-form textual content and structured knowledge, making them best for multi-step processes.
Utilities: Saving Outputs
As a part of the system, it’s helpful to log outputs for debugging or documentation functions. The next utility operate saves content material into markdown information:
def write_markdown_file(content material, filename):
"""Writes the given content material as a markdown file to the native listing.
Args:
content material: The string content material to write down to the file.
filename: The filename to avoid wasting the file as.
"""
with open(f"{filename}.md", "w") as f:
f.write(content material)
This utility helps protect drafts, analysis outcomes, or evaluation reviews for additional evaluate or sharing.
Designing the Fundamental Chains
Our system consists of a collection of logical chains, every addressing a particular facet of the e-mail reply course of. Right here’s a short overview:
- Categorize E mail : Establish the kind of electronic mail(e.g., gross sales,customized inquiry,and so forth.)
- Analysis Router : Direct the e-mail’s context to the suitable search methodology.
- Search Key phrases : Extract related key phrases for gathering further info.
- Write Draft E mail : Use the analysis and electronic mail context to generate a considerate reply.
- Rewrite Router : Decide if the draft requires rewriting or additional enchancment.
- Draft E mail Evaluation : Consider the draft’s coherence, relevance, and tone.
- Rewrite E mail : Finalize the e-mail by refining its tone and content material.
Categorizing Emails
Step one in our pipeline is categorizing the incoming electronic mail. Utilizing a customized immediate template, we information the Llama3 mannequin to research the e-mail and assign it to one of many predefined classes:
- price_enquiry : Questions associated to pricing.
- customer_complaint: Points or grievances.
- product_enquiry: Questions on options, advantages , or companies(excluding pricing).
- customer_feedback : Basic suggestions a couple of services or products.
- off_topic : Emails that don’t it every other class.
Defining the Immediate Template
We create a structured immediate to assist the mannequin deal with the categorization job:
from langchain.prompts import PromptTemplate
immediate = PromptTemplate(
template="""<|begin_of_text|><|start_header_id|>system<|end_header_id|>
You might be an E mail Categorizer Agent. You're a grasp at understanding what a buyer desires once they write an electronic mail and are in a position to categorize it in a helpful means.
<|eot_id|><|start_header_id|>consumer<|end_header_id|>
Conduct a complete evaluation of the e-mail supplied and categorize it into one of many following classes:
price_enquiry - used when somebody is asking for details about pricing
customer_complaint - used when somebody is complaining about one thing
product_enquiry - used when somebody is asking for details about a product function, profit, or service however not about pricing
customer_feedback - used when somebody is giving suggestions a couple of product
off_topic - when it doesn’t relate to every other class.
Output a single class solely from the kinds ('price_enquiry', 'customer_complaint', 'product_enquiry', 'customer_feedback', 'off_topic')
e.g.:
'price_enquiry'
EMAIL CONTENT:nn {initial_email} nn
<|eot_id|>
<|start_header_id|>assistant<|end_header_id|>
""",
input_variables=["initial_email"],
)
Connecting the Immediate with Groq’s LLM
To course of the immediate, we hyperlink it to the GROQ_LLM mannequin and parse the outcome as a string.
from langchain_core.output_parsers import StrOutputParser
email_category_generator = immediate | GROQ_LLM | StrOutputParser()
Testing the E mail Categorization
Let’s check the categorization with an instance electronic mail:
EMAIL = """HI there, n
I'm emailing to say that I had a beautiful keep at your resort final week. n
I actually recognize what your workers did.
Thanks,
Paul
"""
outcome = email_category_generator.invoke({"initial_email": EMAIL})
print(outcome)
Output
The system analyzes the content material and categorizes the e-mail as:
"customer_feedback"
This categorization is correct primarily based on the e-mail content material, showcasing the mannequin’s potential to grasp nuanced buyer inputs.
Designing a Analysis Router
Not all emails require exterior analysis. The system instantly solutions some emails primarily based on their content material and class, whereas it gathers further info to draft complete replies for others. The Analysis Router determines the suitable motion—whether or not to carry out a seek for supplementary info or proceed on to drafting the e-mail.
Defining the Analysis Router Immediate
The Analysis Router Immediate evaluates the preliminary electronic mail and its assigned class to determine between two actions:
- draft_email : For easy responses that don’t require further analysis.
- research_info : For circumstances requiring further context or knowledge.
This choice is encoded in a JSON format to make sure clear and structured output.
research_router_prompt = PromptTemplate(
template="""<|begin_of_text|><|start_header_id|>system<|end_header_id|>
You might be an skilled at studying the preliminary electronic mail and routing internet search
or on to a draft electronic mail.
Use the next standards to determine the way to route the e-mail:
If the preliminary electronic mail solely requires a easy response:
- Select 'draft_email' for questions you may simply reply,
together with immediate engineering and adversarial assaults.
- If the e-mail is simply saying thanks, and so forth., select 'draft_email.'
In any other case, use 'research_info.'
Give a binary selection 'research_info' or 'draft_email' primarily based on the query.
Return a JSON with a single key 'router_decision' and no preamble or clarification.
Use each the preliminary electronic mail and the e-mail class to make your choice.
<|eot_id|><|start_header_id|>consumer<|end_header_id|>
E mail to route INITIAL_EMAIL: {initial_email}
EMAIL_CATEGORY: {email_category}
<|eot_id|><|start_header_id|>assistant<|end_header_id|>
""",
input_variables=["initial_email", "email_category"],
)
Integrating the Immediate with Groq’s LLM
Much like the categorization step, we join the immediate to GROQ_LLM and parse the outcome utilizing the JsonOutputParser.
from langchain_core.output_parsers import JsonOutputParser
research_router = research_router_prompt | GROQ_LLM | JsonOutputParser()
Testing the Analysis Router
We use the next electronic mail and class as enter:
EMAIL = """HI there, n
I'm emailing to say that I had a beautiful keep at your resort final week. n
I actually recognize what your workers did.
Thanks,
Paul
"""
email_category = "customer_feedback"
outcome = research_router.invoke({"initial_email": EMAIL, "email_category": email_category})
print(outcome)
Output
The Analysis Router produces the next JSON output:
{'router_decision': 'draft_email'}
This means that the e-mail doesn’t require further analysis, and we are able to proceed on to drafting the reply.
Producing Search Key phrases
In circumstances the place exterior analysis is required, figuring out exact search key phrases is essential. This step makes use of the e-mail content material and its assigned class to generate the simplest key phrases for retrieving related info.
Defining the Search Key phrases Immediate
The Search Key phrases Immediate helps the mannequin extract as much as three key phrases that may information a targeted and environment friendly internet search. This ensures the analysis part is each correct and related.
search_keyword_prompt = PromptTemplate(
template="""<|begin_of_text|><|start_header_id|>system<|end_header_id|>
You're a grasp at figuring out the very best key phrases for an online search
to seek out probably the most related info for the shopper.
Given the INITIAL_EMAIL and EMAIL_CATEGORY, work out the very best
key phrases that may discover probably the most related info to assist write
the ultimate electronic mail.
Return a JSON with a single key 'key phrases' containing not more than
3 key phrases, and no preamble or clarification.
<|eot_id|><|start_header_id|>consumer<|end_header_id|>
INITIAL_EMAIL: {initial_email}
EMAIL_CATEGORY: {email_category}
<|eot_id|><|start_header_id|>assistant<|end_header_id>
""",
input_variables=["initial_email", "email_category"],
)
Constructing the Search Key phrase Chain
We join the search_keyword_prompt to the GROQ_LLM mannequin and parse the outcomes as JSON for structured output.
search_keyword_chain = search_keyword_prompt | GROQ_LLM | JsonOutputParser()
Testing the Search Key phrases Era
Utilizing the identical electronic mail and class from earlier:
EMAIL = """HI there, n
I'm emailing to say that I had a beautiful keep at your resort final week. n
I actually recognize what your workers did.
Thanks,
Paul
"""
email_category = "customer_feedback"
outcome = search_keyword_chain.invoke({"initial_email": EMAIL, "email_category": email_category})
print(outcome)
Output
The system generates a JSON response with as much as three key phrases:
{'key phrases': ['hotel customer feedback', 'resort appreciation email', 'positive travel review']}
These key phrases replicate the essence of the e-mail and can assist retrieve focused and helpful info for crafting the ultimate response.
By producing exact search key phrases, the system streamlines the analysis part, making it simpler to assemble related knowledge for electronic mail replies. Subsequent, we’ll discover the way to draft the e-mail primarily based on analysis and context. Let me know in the event you’d like to maneuver on!
Writing the Draft E mail
With the analysis full (if wanted) and the e-mail categorized, the following step is to draft a considerate {and professional} response. This step ensures that the response aligns with the shopper’s intent and maintains a pleasant tone.
Defining the Draft Author Immediate
The Draft Author Immediate takes under consideration the preliminary electronic mail, its class, and any supplementary analysis info to craft a customized reply. The template contains particular directions primarily based on the e-mail class to make sure applicable responses:
- off_topic : Ask clarifying questions.
- customer_complaint : Reassure the shopper and handle their issues.
- customer_feedback: Acknowledge the suggestions and categorical gratitude.
- product_enquiry : Present concise and pleasant info primarily based on the analysis.
- price_enquiry : Ship the requested pricing info.
The draft is returned as a JSON object with the important thing email_draft.
draft_writer_prompt = PromptTemplate(
template="""<|begin_of_text|><|start_header_id|>system<|end_header_id|>
You're the E mail Author Agent. Take the INITIAL_EMAIL under from a human that has emailed our firm electronic mail handle, the email_category
that the categorizer agent gave it, and the analysis from the analysis agent, and
write a useful electronic mail in a considerate and pleasant means.
If the shopper electronic mail is 'off_topic' then ask them inquiries to get extra info.
If the shopper electronic mail is 'customer_complaint' then attempt to guarantee we worth them and that we're addressing their points.
If the shopper electronic mail is 'customer_feedback' then thank them and acknowledge their suggestions positively.
If the shopper electronic mail is 'product_enquiry' then attempt to give them the information the researcher supplied in a succinct and pleasant means.
If the shopper electronic mail is 'price_enquiry' then attempt to give the pricing data they requested.
You by no means make up info that hasn't been supplied by the research_info or within the initial_email.
At all times log out the emails in an applicable method and from Sarah, the Resident Supervisor.
Return the e-mail as a JSON with a single key 'email_draft' and no preamble or clarification.
<|eot_id|><|start_header_id|>consumer<|end_header_id|>
INITIAL_EMAIL: {initial_email} n
EMAIL_CATEGORY: {email_category} n
RESEARCH_INFO: {research_info} n
<|eot_id|><|start_header_id|>assistant<|end_header_id>""",
input_variables=["initial_email", "email_category", "research_info"],
)
Constructing the Draft E mail Chain
The immediate is linked to the GROQ_LLM mannequin, and the output is parsed as structured JSON.
draft_writer_chain = draft_writer_prompt | GROQ_LLM | JsonOutputParser()
Testing the Draft E mail Author
We offer the system with the e-mail, class, and analysis info. For this check, no further analysis is required:
email_category = "customer_feedback"
research_info = None
outcome = draft_writer_chain.invoke({
"initial_email": EMAIL,
"email_category": email_category,
"research_info": research_info,
})
print(outcome)
Output:
{
"email_draft": "Expensive Paul,nnThank you a lot on your
sort phrases and suggestions about your latest keep at our
resort. We’re thrilled to listen to that you just had a beautiful
expertise and that our workers made your keep particular.
It actually means quite a bit to us.nnYour satisfaction is our
prime precedence, and we’re at all times right here to make sure each
go to is memorable.nnLooking ahead to welcoming
you again sooner or later!nnWarm regards,nSarahn
Resident Supervisor"
}
Output:
{'email_draft': "Expensive Paul,nnThank you a lot for taking the time to share your great expertise at our resort! We're thrilled to listen to that our workers made a constructive affect on
This response displays the system’s potential to create a considerate and applicable reply whereas adhering to the supplied context.
With the draft electronic mail generated, the pipeline is nearly full. Within the subsequent step, we’ll analyze and, if obligatory, refine the draft for optimum high quality. Let me know in the event you’d wish to proceed!
Rewrite Router
Not all draft emails are excellent on the primary try. The Rewrite Router evaluates the draft electronic mail to find out if it adequately addresses the shopper’s issues or requires rewriting for higher readability, tone, or completeness.
Defining the Rewrite Router Immediate
The Rewrite Router Immediate evaluates the draft electronic mail in opposition to the next standards:
- No Rewrite Required (
- The draft electronic mail supplies a easy response matching the necessities.
- The draft electronic mail addresses all issues from the preliminary electronic mail.
- Rewrite Required (
- The draft electronic mail lacks info wanted to handle the shopper’s issues.
- The draft electronic mail’s tone or content material is inappropriate.
- The response is returned in JSON format with a single key, router_decision.
rewrite_router_prompt = PromptTemplate(
template="""<|begin_of_text|><|start_header_id|>system<|end_header_id|>
You might be an skilled at evaluating emails which can be draft emails for the shopper and deciding in the event that they
should be rewritten to be higher. n
Use the next standards to determine if the DRAFT_EMAIL must be rewritten: nn
If the INITIAL_EMAIL solely requires a easy response which the DRAFT_EMAIL accommodates, then it does not should be rewritten.
If the DRAFT_EMAIL addresses all of the issues of the INITIAL_EMAIL, then it does not should be rewritten.
If the DRAFT_EMAIL is lacking info that the INITIAL_EMAIL requires, then it must be rewritten.
Give a binary selection 'rewrite' (for must be rewritten) or 'no_rewrite' (for does not should be rewritten) primarily based on the DRAFT_EMAIL and the factors.
Return a JSON with a single key 'router_decision' and no preamble or clarification.
<|eot_id|><|start_header_id|>consumer<|end_header_id|>
INITIAL_EMAIL: {initial_email} n
EMAIL_CATEGORY: {email_category} n
DRAFT_EMAIL: {draft_email} n
<|eot_id|><|start_header_id|>assistant<|end_header_id>""",
input_variables=["initial_email", "email_category", "draft_email"],
)
Constructing the Rewrite Router Chain
This chain combines the immediate with the GROQ_LLM mannequin to judge the draft electronic mail and decide if additional refinement is important.
rewrite_router = rewrite_router_prompt | GROQ_LLM | JsonOutputParser()
Testing the Rewrite Router
For testing, let’s consider a draft electronic mail that clearly falls wanting expectations:
email_category = "customer_feedback"
draft_email = "Yo we will not aid you, finest regards Sarah"
outcome = rewrite_router.invoke({
"initial_email": EMAIL,
"email_category": email_category,
"draft_email": draft_email
})
print(outcome)
Output
The system identifies the necessity for rewriting:
{'router_decision': 'rewrite'}
This ensures that inappropriate or incomplete drafts don’t attain the shopper.
By implementing the Rewrite Router, the system ensures that each electronic mail response meets a excessive normal of high quality and relevance.
Draft E mail Evaluation
The Draft E mail Evaluation step evaluates the standard of the draft electronic mail, guaranteeing that it successfully addresses the shopper’s points and supplies actionable suggestions for enchancment.
Defining the Draft Evaluation Immediate
The Draft Evaluation Immediate inspects the draft electronic mail utilizing the next standards:
- Does the draft electronic mail adequately handle the shopper’s issues primarily based on the e-mail class?
- Does it align with the context and tone of the preliminary electronic mail?
- Are there any particular areas the place the draft will be improved (e.g., tone, readability, completeness)?
The output is structured as a JSON object containing a single key, draft_analysis, with suggestions and ideas.
draft_analysis_prompt = PromptTemplate(
template="""<|begin_of_text|><|start_header_id|>system<|end_header_id|>
You're the High quality Management Agent. Learn the INITIAL_EMAIL under from a human that has emailed
our firm electronic mail handle, the email_category that the categorizer agent gave it, and the
analysis from the analysis agent, and write an evaluation of the e-mail.
Test if the DRAFT_EMAIL addresses the shopper's points primarily based on the e-mail class and the
content material of the preliminary electronic mail.n
Give suggestions on how the e-mail will be improved and what particular issues will be added or modified
to make the e-mail simpler at addressing the shopper's points.
You by no means make up or add info that hasn't been supplied by the research_info or within the initial_email.
Return the evaluation as a JSON with a single key 'draft_analysis' and no preamble or clarification.
<|eot_id|><|start_header_id|>consumer<|end_header_id|>
INITIAL_EMAIL: {initial_email} nn
EMAIL_CATEGORY: {email_category} nn
RESEARCH_INFO: {research_info} nn
DRAFT_EMAIL: {draft_email} nn
<|eot_id|><|start_header_id|>assistant<|end_header_id|>""",
input_variables=["initial_email", "email_category", "research_info", "draft_email"],
)
Constructing the Draft Evaluation Chain
This chain pairs the immediate with the GROQ_LLM mannequin and parses the output.
draft_analysis_chain = draft_analysis_prompt | GROQ_LLM | JsonOutputParser()
Testing the Draft Evaluation Chain
We check the chain with a intentionally poor draft electronic mail to look at the system’s suggestions:
email_category = "customer_feedback"
research_info = None
draft_email = "Yo we will not aid you, finest regards Sarah"
email_analysis = draft_analysis_chain.invoke({
"initial_email": EMAIL,
"email_category": email_category,
"research_info": research_info,
"draft_email": draft_email
})
pprint(email_analysis)
Output
The system supplies constructive evaluation:
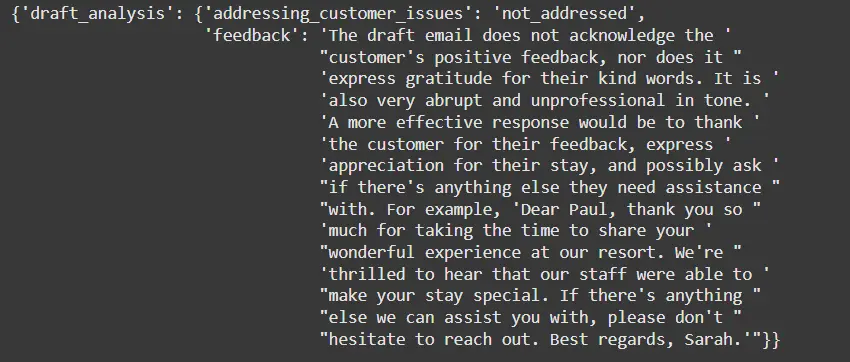
Evaluation
The suggestions emphasizes the necessity for professionalism, alignment with buyer sentiment, and correct acknowledgment of their message. It additionally supplies a concrete instance of how the draft electronic mail will be improved.
With the Draft Evaluation in place, the system ensures steady enchancment and better high quality in buyer communication.
The Rewrite E mail with Evaluation step refines the draft electronic mail utilizing the suggestions supplied within the Draft E mail Evaluation. This remaining step ensures that the e-mail is polished and applicable for sending to the shopper.
Defining the Rewrite E mail Immediate
The Rewrite E mail Immediate combines the draft electronic mail with the suggestions from the Draft E mail Evaluation. The aim is to use the prompt modifications and enhance the tone, readability, and effectiveness of the response with out introducing any new info.
The output is a JSON containing a single key, final_email, which accommodates the refined model of the draft electronic mail.
rewrite_email_prompt = PromptTemplate(
template="""<|begin_of_text|><|start_header_id|>system<|end_header_id|>
You're the Ultimate E mail Agent. Learn the e-mail evaluation under from the QC Agent
and use it to rewrite and enhance the draft_email to create a remaining electronic mail.
You by no means make up or add info that hasn't been supplied by the research_info or within the initial_email.
Return the ultimate electronic mail as JSON with a single key 'final_email' which is a string and no preamble or clarification.
<|eot_id|><|start_header_id|>consumer<|end_header_id|>
EMAIL_CATEGORY: {email_category} nn
RESEARCH_INFO: {research_info} nn
DRAFT_EMAIL: {draft_email} nn
DRAFT_EMAIL_FEEDBACK: {email_analysis} nn
<|eot_id|>"""
input_variables=["initial_email", "email_category", "research_info", "email_analysis", "draft_email"],
)
Constructing the Rewrite Chain
This chain combines the immediate with GROQ_LLM to generate the ultimate model of the e-mail primarily based on the evaluation.
rewrite_chain = rewrite_email_prompt | GROQ_LLM | JsonOutputParser()
Testing the Rewrite E mail Chain
To check, we use the customer_feedback class and a draft electronic mail that requires substantial revision.
email_category = 'customer_feedback'
research_info = None
draft_email = "Yo we will not aid you, finest regards Sarah"
final_email = rewrite_chain.invoke({
"initial_email": EMAIL,
"email_category": email_category,
"research_info": research_info,
"draft_email": draft_email,
"email_analysis": email_analysis
})
print(final_email['final_email'])
Instance Output
'Expensive Paul, thanks a lot for taking the time to share your great expertise at our resort. We're thrilled to listen to that our workers had been in a position to make your keep particular. If ther e's the rest we are able to help you with, please do not hesitate to achieve out. Finest regards, Sarah'
By implementing the Rewrite E mail with Evaluation step, the system delivers a elegant remaining draft that successfully addresses the shopper’s suggestions and maintains knowledgeable tone.
The Instrument Setup part configures the instruments wanted for your entire course of. Right here, the search software is initialized and the state of the graph is outlined. These instruments enable for interplay with exterior knowledge sources and make sure the electronic mail technology course of follows a structured stream.
The TavilySearchResults software is about as much as deal with internet searches and retrieve related outcomes.
from langchain_community.instruments.tavily_search import TavilySearchResults
web_search_tool = TavilySearchResults(ok=1)
The ok=1 parameter ensures that just one result’s fetched per search.
State Setup
The GraphState class, outlined as a TypedDict, represents the state of the method. It tracks the mandatory knowledge throughout the totally different levels of electronic mail processing.
from langchain.schema import Doc
from langgraph.graph import END, StateGraph
from typing_extensions import TypedDict
from typing import Checklist
class GraphState(TypedDict):
"""
Represents the state of our graph.
Attributes:
initial_email: electronic mail
email_category: electronic mail class
draft_email: LLM technology
final_email: LLM technology
research_info: listing of paperwork
info_needed: whether or not so as to add search data
num_steps: variety of steps
"""
initial_email: str
email_category: str
draft_email: str
final_email: str
research_info: Checklist[str]
info_needed: bool
num_steps: int
draft_email_feedback: dict
- initial_email : The content material of the shopper’s electronic mail.
- email_category : The class assigned to the e-mail(e.g., gross sales, criticism, suggestions).
- draft_email : The e-mail generated by the system in response to the shopper’s message.
- final_email : The ultimate model of the e-mail after revisions primarily based on evaluation.
- research_info: A listing of related paperwork gathered in the course of the analysis part.
- info_needed : A boolean flag indicating if further info is required for the e-mail response.
- num_steps : A counter for the variety of steps accomplished.
- draft_email_feedback : Suggestions from the draft evaluation stage.
This state setup helps in monitoring the method, guaranteeing that each one obligatory info is obtainable and up-to-date as the e-mail response evolves.
The Nodes part defines the important thing steps within the electronic mail dealing with course of. These nodes correspond to totally different actions within the pipeline, from categorizing the e-mail to researching and drafting the response. Every operate manipulates the GraphState, performs an motion, after which updates the state.
Categorize E mail
The categorize_email node categorizes the incoming electronic mail primarily based on its content material.
def categorize_email(state):
"""Take the preliminary electronic mail and categorize it"""
print("---CATEGORIZING INITIAL EMAIL---")
initial_email = state['initial_email']
num_steps = int(state['num_steps'])
num_steps += 1
# Categorize the e-mail
email_category = email_category_generator.invoke({"initial_email": initial_email})
print(email_category)
# Save class to native disk
write_markdown_file(email_category, "email_category")
return {"email_category": email_category, "num_steps": num_steps}
- This operate calls the email_category_generator to categorize the e-mail primarily based on its content material.
- The class is saved to a file for record-keeping.
- It returns the up to date state with the email_category and an incremented num_steps.
Analysis Information Search
The research_info_search node performs an online search primarily based on key phrases derived from the preliminary electronic mail and its class.
def research_info_search(state):
print("---RESEARCH INFO SEARCHING---")
initial_email = state["initial_email"]
email_category = state["email_category"]
research_info = state["research_info"]
num_steps = state['num_steps']
num_steps += 1
# Net seek for key phrases
key phrases = search_keyword_chain.invoke({"initial_email": initial_email,
"email_category": email_category })
key phrases = key phrases['keywords']
full_searches = []
for key phrase in key phrases[:1]: # Solely taking the primary key phrase
print(key phrase)
temp_docs = web_search_tool.invoke({"question": key phrase})
web_results = "n".be part of([d["content"] for d in temp_docs])
web_results = Doc(page_content=web_results)
if full_searches shouldn't be None:
full_searches.append(web_results)
else:
full_searches = [web_results]
print(full_searches)
print(kind(full_searches))
return {"research_info": full_searches, "num_steps": num_steps}
- The operate generates a listing of key phrases primarily based on the initial_email and email_category utilizing search_keyword_chain.
- It then performs an online seek for every key phrase utilizing TravilySearchResults.
- The outcomes are gathered into a listing of Doc objects and saved to the state as research_info.
Subsequent Steps within the Course of
To finish the e-mail pipeline, we have to outline further nodes that deal with drafting, analyzing, and rewriting the e-mail. As an example:
- Draft E mail Author: Makes use of the categorized electronic mail, analysis data, and LLMs to generate the primary draft of the e-mail.
- Analyze Draft E mail: Opinions the draft electronic mail and supplies suggestions for enhancements.
- Rewrite E mail: Makes use of the suggestions to rewrite the draft electronic mail right into a remaining model.
- No Rewrite: If no rewrite is important, this node would cross the draft to the following stage with out modifications.
- State Printer: Prints or logs the present state for debugging functions.
Instance of Additional Steps
def draft_email_writer(state):
print("---WRITING DRAFT EMAIL---")
# Implement logic to generate draft electronic mail primarily based on analysis and class.
return {"draft_email": draft_email_content, "num_steps": state['num_steps']}
def analyze_draft_email(state):
print("---ANALYZING DRAFT EMAIL---")
# Implement logic to research draft electronic mail, offering suggestions.
return {"draft_email_feedback": draft_feedback, "num_steps": state['num_steps']}
def rewrite_email(state):
print("---REWRITING EMAIL---")
# Use suggestions to rewrite the e-mail.
return {"final_email": final_email_content, "num_steps": state['num_steps']}
def state_printer(state):
print("---STATE---")
print(state)
return state
These further nodes would guarantee a easy transition by your entire electronic mail processing pipeline.
capabilities draft_email_writer and analyze_draft_email are set as much as generate and consider electronic mail drafts primarily based on the present state, which incorporates the preliminary electronic mail, its class, analysis info, and the present step within the course of. Right here’s a fast evaluation of the 2 capabilities:
Draft E mail Author (draft_email_writer)
This operate creates a draft electronic mail primarily based on the preliminary electronic mail, electronic mail class, and analysis data.
def draft_email_writer(state):
print("---DRAFT EMAIL WRITER---")
## Get the state
initial_email = state["initial_email"]
email_category = state["email_category"]
research_info = state["research_info"]
num_steps = state['num_steps']
num_steps += 1
# Generate draft electronic mail utilizing the draft_writer_chain
draft_email = draft_writer_chain.invoke({"initial_email": initial_email,
"email_category": email_category,
"research_info": research_info})
print(draft_email)
email_draft = draft_email['email_draft'] # Extract the e-mail draft from response
write_markdown_file(email_draft, "draft_email") # Save draft to a markdown file
return {"draft_email": email_draft, "num_steps": num_steps}
- The operate first extracts obligatory particulars from the state (initial_email, email_category, research_info).
- It then calls the draft_writer_chain to generate a draft electronic mail.
- The draft electronic mail is printed and saved to a markdown file for evaluate.
- The up to date state with the generated draft electronic mail and incremented num_steps is returned.
Draft E mail Analyzer (analyze_draft_email)
This operate analyzes the draft electronic mail and supplies suggestions on the way to enhance it.
def analyze_draft_email(state):
print("---DRAFT EMAIL ANALYZER---")
## Get the state
initial_email = state["initial_email"]
email_category = state["email_category"]
draft_email = state["draft_email"]
research_info = state["research_info"]
num_steps = state['num_steps']
num_steps += 1
# Generate draft electronic mail suggestions utilizing the draft_analysis_chain
draft_email_feedback = draft_analysis_chain.invoke({"initial_email": initial_email,
"email_category": email_category,
"research_info": research_info,
"draft_email": draft_email})
# Save suggestions to a markdown file for later evaluate
write_markdown_file(str(draft_email_feedback), "draft_email_feedback")
return {"draft_email_feedback": draft_email_feedback, "num_steps": num_steps}
- The operate takes the state and retrieves obligatory info like draft electronic mail, analysis data, and electronic mail class.
- It then invokes the draft_analysis_chain to research the draft electronic mail, offering suggestions on its high quality and effectiveness.
- The suggestions is saved as a markdown file for reference, and the up to date state (with suggestions and incremented num_steps) is returned.
rewrite_email operate is designed to take the draft electronic mail and its related suggestions, then use that to generate a remaining electronic mail that includes the mandatory modifications and enhancements.
Right here’s a breakdown of the operate:
def rewrite_email(state):
print("---REWRITE EMAIL ---")
# Extract obligatory state variables
initial_email = state["initial_email"]
email_category = state["email_category"]
draft_email = state["draft_email"]
research_info = state["research_info"]
draft_email_feedback = state["draft_email_feedback"]
num_steps = state['num_steps']
# Increment the step depend
num_steps += 1
# Generate the ultimate electronic mail utilizing the rewrite_chain
final_email = rewrite_chain.invoke({
"initial_email": initial_email,
"email_category": email_category,
"research_info": research_info,
"draft_email": draft_email,
"email_analysis": draft_email_feedback
})
# Save the ultimate electronic mail to a markdown file for evaluate
write_markdown_file(str(final_email), "final_email")
# Return up to date state with the ultimate electronic mail and incremented steps
return {"final_email": final_email['final_email'], "num_steps": num_steps}
Key Factors
- Extract State Variables: It begins by extracting the required state variables equivalent to initial_email, email_category, draft_email, research_info, and draft_email_feedback.
- Increment Step Rely: The num_steps counter is incremented to replicate that this can be a new step within the course of (i.e., producing the ultimate electronic mail).
- Generate Ultimate E mail: The rewrite_chain is used to generate the ultimate electronic mail primarily based on the preliminary electronic mail, electronic mail class, analysis data, draft electronic mail, and suggestions (from the earlier step, i.e., the draft_email_feedback).
This step improves the draft electronic mail primarily based on the evaluation suggestions.
- Write Ultimate E mail to Disk: The ultimate electronic mail is saved to a markdown file (for transparency and evaluate).
- Return Up to date State: The operate returns the ultimate electronic mail and up to date num_steps to replace the general state for subsequent steps.
Rationalization of the New Features
- no_rewrite: This operate is used when the draft electronic mail doesn’t require any additional modifications and is able to be despatched as the ultimate electronic mail.
- Inputs: It takes within the state, particularly specializing in the draft electronic mail.
- Course of:
- The draft_email is taken into account remaining, so it’s saved as the ultimate electronic mail.
- The num_steps counter is incremented to trace the progress.
- Output: It returns the state with the final_email set to the draft electronic mail, and an up to date num_steps.
def no_rewrite(state):
print("---NO REWRITE EMAIL ---")
## Get the state
draft_email = state["draft_email"]
num_steps = state['num_steps']
num_steps += 1
# Save the draft electronic mail as remaining electronic mail
write_markdown_file(str(draft_email), "final_email")
return {"final_email": draft_email, "num_steps": num_steps}
- state_printer: This operate prints out the present state of the method, giving an in depth overview of the variables and their values. This may be helpful for debugging or monitoring the progress in your electronic mail technology pipeline.
- Inputs: It takes the total state as enter.
- Course of: It prints the values of the preliminary electronic mail, electronic mail class, draft electronic mail, remaining electronic mail, analysis data, data wanted, and num_steps.
- Output: This operate doesn’t return something however helps in debugging or logging.
def state_printer(state):
"""print the state"""
print("---STATE PRINTER---")
print(f"Preliminary E mail: {state['initial_email']} n" )
print(f"E mail Class: {state['email_category']} n")
print(f"Draft E mail: {state['draft_email']} n" )
print(f"Ultimate E mail: {state['final_email']} n" )
print(f"Analysis Information: {state['research_info']} n")
print(f"Num Steps: {state['num_steps']} n")
# Test if 'info_needed' exists within the state
info_needed = state.get('info_needed', 'N/A')
print(f"Information Wanted: {info_needed} n")
return
How These Match Into the Workflow
- The no_rewrite operate is usually known as if the system determines that the draft electronic mail doesn’t want modifications, that means it’s prepared for remaining supply.
- The state_printer operate is beneficial throughout debugging or to test your entire course of step-by-step.
Instance Movement
- If the rewrite_router or one other course of determines that no modifications are wanted, the no_rewrite operate known as.
- The state_printer will be invoked at any level within the workflow (usually after finishing the e-mail technology) to examine the ultimate state of the system.
Rationalization of route_to_research Perform
This operate determines the following step within the course of stream primarily based on the routing choice made by the research_router. The choice will depend on the content material of the e-mail and its categorization. Right here’s the way it works:
- Inputs: The state dictionary, which accommodates the present state of the e-mail processing workflow, together with the preliminary electronic mail and electronic mail class.
- Course of: The operate invokes the research_router to determine whether or not the e-mail requires further analysis (internet search) or if it may instantly proceed to drafting a response.
The research_router returns a router_decision indicating whether or not to proceed with analysis (‘research_info’) or go straight to drafting the e-mail (‘draft_email’).
- Outputs: The operate returns the title of the following node to name within the workflow: “research_info” (if the e-mail wants additional analysis) or “draft_email” (if the e-mail will be drafted instantly).
- Logging: There are print statements to show the choice made and the reasoning behind it. That is helpful for debugging and guaranteeing that the routing logic works as supposed.
Code Implementation
def route_to_research(state):
"""
Route electronic mail to internet search or not.
Args:
state (dict): The present graph state
Returns:
str: Subsequent node to name
"""
print("---ROUTE TO RESEARCH---")
initial_email = state["initial_email"]
email_category = state["email_category"]
# Route choice primarily based on the e-mail class and content material
router = research_router.invoke({"initial_email": initial_email, "email_category": email_category})
print(router)
# Retrieve the router's choice
print(router['router_decision'])
# Routing logic
if router['router_decision'] == 'research_info':
print("---ROUTE EMAIL TO RESEARCH INFO---")
return "research_info"
elif router['router_decision'] == 'draft_email':
print("---ROUTE EMAIL TO DRAFT EMAIL---")
return "draft_email"
How It Matches into the Graph
- Routing Determination: Based mostly on the outcome from research_router, this operate routes the method to both:
- research_info: If the e-mail requires analysis to reply a buyer question.
- draft_email: If the e-mail will be answered instantly with out additional analysis.
- Conditional Edge: This operate is usually a part of a decision-making step (conditional edge) in your state graph, serving to steer the workflow.
Instance Workflow
- Preliminary E mail is obtained.
- E mail Categorization occurs to categorise the e-mail into one of many outlined classes (e.g., buyer suggestions).
- Route Determination: Based mostly on the e-mail’s class and content material, the route_to_research operate decides if additional analysis is required or if a draft electronic mail will be created.
- If analysis is required, it routes to research_info. In any other case, it proceeds to draft_email.
Rationalization of route_to_rewrite Perform
This operate determines the following step within the course of stream primarily based on the analysis of the draft electronic mail. Particularly, it decides whether or not the draft electronic mail must be rewritten or if it may be despatched as is. Right here’s the way it works:
Inputs
The state dictionary, which accommodates:
- initial_email: The unique electronic mail from the shopper.
- email_category: The class assigned to the e-mail (e.g., buyer suggestions, product inquiry, and so forth.).
- draft_email: The e-mail draft generated earlier.
- research_info: Any related info retrieved from the analysis step, though it isn’t used instantly right here.
Course of
The operate invokes the rewrite_router to evaluate whether or not the draft electronic mail wants rewriting primarily based on the initial_email, email_category, and draft_email.
The rewrite_router returns a router_decision, which will be both:
- rewrite: Signifies the draft electronic mail must be improved and despatched for evaluation.
- no_rewrite: Signifies the draft electronic mail is adequate and may proceed to the ultimate stage.
Outputs
- If the choice is rewrite, the operate routes to the rewrite step to revise the draft.
- If the choice is no_rewrite, the operate proceeds to finalize the e-mail.
Logging
There are print statements to log the choice and observe the workflow progress.
Code Implementation
def route_to_rewrite(state):
"""
Route electronic mail to rewrite or not, primarily based on the draft electronic mail high quality.
Args:
state (dict): The present graph state
Returns:
str: Subsequent node to name (rewrite or no_rewrite)
"""
print("---ROUTE TO REWRITE---")
initial_email = state["initial_email"]
email_category = state["email_category"]
draft_email = state["draft_email"]
research_info = state["research_info"]
# Invoke the rewrite router to judge the draft electronic mail
router = rewrite_router.invoke({"initial_email": initial_email,
"email_category": email_category,
"draft_email": draft_email})
print(router)
# Retrieve the router's choice
print(router['router_decision'])
# Routing logic primarily based on the analysis
if router['router_decision'] == 'rewrite':
print("---ROUTE TO ANALYSIS - REWRITE---")
return "rewrite"
elif router['router_decision'] == 'no_rewrite':
print("---ROUTE EMAIL TO FINAL EMAIL---")
return "no_rewrite"
How It Matches into the Graph
- This operate is usually invoked after the draft electronic mail has been generated.
- It evaluates the standard of the draft electronic mail and decides if it wants additional revision or if it may be finalized instantly.
- Based mostly on the choice, it both routes to the rewrite step (the place the e-mail is revised) or the no_rewrite step (the place the draft is finalized).
Instance Workflow
- Preliminary E mail: The client sends an electronic mail.
- E mail Categorization: The e-mail is categorized primarily based on its content material.
- Draft E mail Creation: A draft electronic mail is generated.
- Rewrite Determination: The route_to_rewrite operate evaluates if the draft must be rewritten:
- If rewrite is required, the draft goes to the rewrite step.
- If no_rewrite is chosen, the draft strikes to the ultimate electronic mail stage.
This code defines the construction of the workflow for processing buyer emails utilizing a state graph, the place every step of the method is dealt with by totally different nodes. Let’s break down the workflow and clarify the way it works:
Workflow Overview
- StateGraph: This can be a directed graph the place nodes characterize duties (like categorizing emails, drafting replies, and so forth.), and edges outline the stream of the method from one job to the following.
- Nodes: Every node corresponds to a operate or motion that shall be carried out in the course of the workflow (e.g., categorizing the e-mail, producing a draft reply, analyzing the draft).
- Edges: These outline the transitions between nodes. Conditional edges enable routing primarily based on selections (e.g., whether or not to seek for analysis info or draft the e-mail).
Key Elements of the Graph
- Entry Level: The method begins on the categorize_email node.
- Categorizing the E mail:
- The primary job is to categorize the incoming electronic mail right into a predefined class (like “buyer suggestions”, “product inquiry”, and so forth.). That is carried out within the categorize_email node.
- As soon as categorized, a choice is made whether or not to seek for analysis data or instantly draft a response primarily based on the class.
- Conditional Routing
From categorize_email:
If analysis data is required (primarily based on the e-mail class), the workflow strikes to research_info_search. In any other case, it goes to draft_email_writer to instantly generate a response draft.
From draft_email_writer: After the draft is created:
- The system evaluates the draft and decides whether or not it requires rewriting.
- If rewriting is important, it sends the draft to analyze_draft_email for evaluate and enchancment.
- If no rewrite is required, the system forwards the draft on to state_printer for remaining output.
Finalization
After analyzing and rewriting the draft electronic mail or accepting it as-is, the system sends the e-mail to state_printer, which prints the ultimate state of the e-mail together with all related info.
Finish Level: The method concludes when state_printer has completed printing the ultimate electronic mail and state info.
# Outline the workflow (state graph)
workflow = StateGraph(GraphState)
# Add nodes to the workflow graph
workflow.add_node("categorize_email", categorize_email) # Categorize the e-mail
workflow.add_node("research_info_search", research_info_search) # Carry out internet seek for data
workflow.add_node("state_printer", state_printer) # Print the ultimate state
workflow.add_node("draft_email_writer", draft_email_writer) # Generate draft electronic mail
workflow.add_node("analyze_draft_email", analyze_draft_email) # Analyze the draft
workflow.add_node("rewrite_email", rewrite_email) # Rewrite the e-mail if obligatory
workflow.add_node("no_rewrite", no_rewrite) # No rewrite wanted, simply finalize
# Set the entry level to the "categorize_email" node
workflow.set_entry_point("categorize_email")
# Add conditional edges primarily based on the end result of the categorization
workflow.add_conditional_edges(
"categorize_email",
route_to_research,
{
"research_info": "research_info_search", # If analysis data wanted, go to analysis
"draft_email": "draft_email_writer", # If no analysis wanted, go to draft electronic mail technology
},
)
# Add edges between nodes
workflow.add_edge("research_info_search", "draft_email_writer") # After analysis, go to drafting
# Add conditional edges primarily based on whether or not the draft electronic mail wants rewriting or not
workflow.add_conditional_edges(
"draft_email_writer",
route_to_rewrite,
{
"rewrite": "analyze_draft_email", # If rewrite wanted, go to research draft
"no_rewrite": "no_rewrite", # If no rewrite wanted, go to remaining electronic mail
},
)
# Add edges to finalize the e-mail or ship for rewriting
workflow.add_edge("analyze_draft_email", "rewrite_email") # After analyzing, rewrite the e-mail
workflow.add_edge("no_rewrite", "state_printer") # No rewrite, finalize the e-mail
workflow.add_edge("rewrite_email", "state_printer") # After rewriting, finalize the e-mail
# Lastly, add the top node
workflow.add_edge("state_printer", END)
# Compile the workflow into an executable software
app = workflow.compile()
Workflow Execution
- The method begins with categorizing the e-mail.
- Relying on the e-mail class, it both searches for related info or strikes on to drafting a response.
- As soon as the draft is prepared, it’s evaluated for any obligatory revisions.
- The method ends when the ultimate electronic mail (both rewritten or not) is printed.
This setup creates a versatile and automatic course of for dealing with buyer emails, permitting for customized responses primarily based on their wants.
EMAIL = """HI there, n
I'm emailing to say that I had a beautiful keep at your resort final week. n
I actually appreaciate what your workers did
Thanks,
Paul
"""
EMAIL = """HI there, n
I'm emailing to say that the resort climate was strategy to cloudy and overcast. n
I needed to write down a music known as 'Right here comes the solar but it surely by no means got here'
What must be the climate in Arizona in April?
I actually hope you repair this subsequent time.
Thanks,
George
"""
# run the agent
inputs = {
"initial_email": EMAIL,
"research_info": None,
"num_steps": 0,
"info_needed": False # Guarantee this key's added
}
for output in app.stream(inputs):
for key, worth in output.gadgets():
pprint(f"Completed working: {key}:")
Output:
---CATEGORIZING INITIAL EMAIL---
'customer_complaint'
---ROUTE TO RESEARCH---
{'router_decision': 'research_info'} research_info
---ROUTE EMAIL TO RESEARCH INFO---
'Completed working: categorize_email:'
---RESEARCH INFO SEARCHING--- Arizona climate April
[Document(metadata={}, page_content="{'location': {'name': 'Arizona', 'region': 'Atlantida', 'country': 'Honduras', 'lat: 15.6333, 'lon': -87.3167, 'tz_id': 'America/Tegucigalpa', 'localtime_epoch: 1731842866, 'localtime': '2824-11-17 05:27'), 'current': {'last_updated_epoch: 1731842100, 'last_updated': '2824-11-17 85:15', 'temp_c": 23.4, 'temp_f': 74.1, 'is_day': 0, 'cond:
<class 'list'>
'Finished running: research_info_search:
---DRAFT EMAIL WRITER---
{"email_draft": "Hi George, nnThank you for reaching out to us about the weather conditions during your recent resort stay. Sorry to hear that it was cloudy and overcast, and I can understand why you'd want to write a song about it!nnRegarding your question about the weather in Arizona in April, I can provide you with some information. Typically, Arizona's weather in April ---ROUTE TO REWRITE--- {'router_decision': 'no_rewrite"}
no_rewrite
---ROUTE EMAIL TO FINAL EMAIL---
'Finished running: draft_email_writer:"
---NO REWRITE EMAIL ---
'Finished running: no_rewrite:'
---STATE PRINTER---
Initial Email: HI there,
I am emailing to say that the resort weather was way to cloudy and overcast.
I wanted to write a song called 'Here comes the sun but it never came'
What should be the weather in Arizona in April?
I really hope you fix this next time.
Thanks, George
Email Category: 'customer_complaint'
Draft Email: Hi George,
Thank you for reaching out to us about the weather conditions during your recent resort stay. Sorry to hear that it was cloudy and overcast, and I can understand why you'd want to write a song about it!
Regarding your question about the weather in Arizona in April, I can provide you with some information. Typically, Arizona's weather in April is quite comfortable, with low temperatures around 64°F and highs up to 84°F. You can expect a few rainy days, but overall, the weather is usually pleasant during this time.
I want to assure you that we value your feedback and would like to make things right. Unfortunately, we can't control the weather, but we'll do our best to ensure that your next stay with us is more to your liking.
Thank you for your feedback, and I hope you get to write that song someday!
Best regards,
Sarah, Resident Manager
Final Email: Hi George,
Thank you for reaching out to us about the weather conditions during your recent resort stay. Sorry to hear that it was cloudy and overcast, and I can understand why you'd want to write a song about it!
Regarding your question about the weather in Arizona in April, I can provide you with some information. Typically, Arizona's weather in April is quite comfortable, with low temperatures around 64°F and highs up to 84°F. You can expect a few rainy days, but overall, the weather is usually pleasant during this time.
output = app.invoke(inputs)
print(output['final_email'])
Output:
---CATEGORIZING INITIAL EMAIL---
'customer_complaint'
---ROUTE TO RESEARCH---
{'router_decision': 'research_info"} analysis data
---ROUTE EMAIL TO RESEARCH INFO---
---RESEARCH INFO SEARCHING---
Arizona climate April
[Doc(metadata={}, page_content="{'location': {'title': 'Arizona', 'area': 'Atlantida', 'nation': 'Honduras', 'lat: 15.6333, 'lon': -87.3167, 'tz_id': 'America/Tegucigalpa', 'localtime_epoch: 1731842866, 'localtime': '2824-11-17 85:27"}, 'present': {'last_updated_epoch': 1731842188, 'last_updated': '2824-11-17 85:15', 'temp_c": 23.4, 'temp_f': 74.1, 'is_day': 0, 'cone <class 'listing'>
---DRAFT EMAIL WRITER---
---ROUTE TO REWRITE---
{"email_draft": "Hello George, nnI'm so sorry to listen to that the climate did not fairly dwell as much as your expectations throughout your latest keep at our resort. I can perceive how irritating it have to be to expertise cloudy and overcast climate, and I recognize your humorousness in wanting to write down a music titled 'Right here comes the solar but it surely by no means got here'!nnRegarding your query ab {'router_decision': 'no_rewrite"}
no_rewrite
---ROUTE EMAIL TO FINAL EMAIL---
---NO REWRITE EMAIL ---
---STATE PRINTER---
Preliminary E mail: HI there,
I'm emailing to say that the resort climate was strategy to cloudy and overcast.
I needed to write down a music known as 'Right here comes the solar but it surely by no means got here'
What must be the climate in Arizona in April?
I actually hope you repair this subsequent time.
Thanks, George
E mail Class: 'customer_complaint"
Draft E mail: Hello George,
I am so sorry to listen to that the climate did not fairly dwell as much as your expectations throughout your latest keep at our resort. I can perceive how irritating it have to be to expertise cloudy and overcast climate, and I recognize your humorousness in wanting to write down a music titled 'Right here comes the solar but it surely by no means got here'!
Relating to your query in regards to the climate in Arizona in April, I might be completely happy to assist. In line with our analysis, April is a good time to go to Arizona, with comfy temperatures starting from 64°F to 84°F. Whereas it is not unusual to expertise some wet days in the course of the month, the climate is usually nice and comfy. When you're planning a visit to Arizona in April, As soon as once more, I apologize for any inconvenience the climate could have prompted throughout your keep, and I hope you will give us one other likelihood to offer you a extra fulfilling expertise sooner or later.
Thanks for reaching out to us, and I want you all the very best.
Finest regards,
Sarah
Resident Supervisor
Ultimate E mail: Hello George,
I am so sorry to listen to that the climate did not fairly dwell as much as your expectations throughout your latest keep at our resort. I can perceive how irritating it have to be to expertise cloudy and overcast climate, and I recognize your humorousness in wanting to write down a music titled 'Right here comes the solar but it surely by no means got here'!
Relating to your query in regards to the climate in Arizona in April, I might be completely happy to assist. In line with our analysis, April is a good time to go to Arizona, with comfy temperatures starting from 64°F to 84°F. Whereas it is not unusual to expertise some wet days in the course of the month, the climate is usually nice and comfy. When you're planning a visit to Arizona in April, As soon as once more, I apologize for any inconvenience the climate could have prompted throughout your keep, and I hope you will give us one other likelihood to offer you a extra fulfilling expertise sooner or later.
Thanks for reaching out to us, and I want you all the very best.
Finest regards,
Sarah
Resident Supervisor
Analysis Information: [Doc(metadata={}, page_content="{"location": {'title': 'Arizona', 'area': 'Atlantida', 'nation': 'Honduras', 'lat': 15.6333, 'lon': -87.3167, 'tz_id": 'America/Tegucigalpa', 'localtime_epoch": 1731842866, 'localtime': '2824-11-17 85:27'), 'present': {'last_updated_epoch": 1731842180, "last_updated': '2824-11-17 05:15', 'temp_c": 23.4, 'temp_f': 74.1, 'is Information Wanted: False
Num Steps: 4
Hello George,
I am so sorry to listen to that the climate did not fairly dwell as much as your expectations throughout your latest keep at our resort. I can perceive how irritating it have to be to expertise cloudy and overcast climate, and I recognize your humorousness in wanting to write down a music titled 'Right here comes the solar but it surely by no means got here'!
Relating to your query in regards to the climate in Arizona in April, I might be completely happy to assist. In line with our analysis, April is a good time to go to Arizona, with comfy temperatures starting from 64°F to 84°F. Whereas it is not unusual to expertise some wet days in the course of the month, the climate is usually nice and comfy. When you're planning a visit to Arizona in April, As soon as once more, I apologize for any inconvenience the climate could have prompted throughout your keep, and I hope you will give us one other likelihood to offer you a extra fulfilling expertise sooner or later.
Thanks for reaching out to us, and I want you all the very best.
Finest regards,
Sarah
Resident Supervisor
The system effectively categorized the e-mail as a ‘customer_complaint’ and routed it to analysis the climate info for Arizona in April. The analysis module gathered detailed knowledge about anticipated climate patterns, together with common temperatures and rainfall. Utilizing this info, a well mannered and informative draft electronic mail was generated, instantly addressing the issues raised by the shopper.
Because the draft met high quality expectations, it bypassed the rewrite course of, finalizing with a well-crafted response that supplied related climate insights and reassured the shopper. The method concluded in simply 4 steps, showcasing the system’s potential to ship contextually correct, customer-focused communication with minimal intervention.
Conclusion
Incorporating LangGraph and GROQ’s LLM into your electronic mail workflow supplies a sturdy, scalable, and environment friendly answer for dealing with buyer communications. By leveraging LangGraph’s versatile state administration and GROQ’s superior pure language processing capabilities, this workflow automates duties like electronic mail categorization, analysis integration, and response drafting whereas guaranteeing high quality management and buyer satisfaction.
This strategy not solely saves time and sources but in addition enhances the accuracy and professionalism of your responses, fostering higher relationships together with your prospects. Whether or not you’re dealing with easy inquiries or advanced complaints, this workflow is adaptable, dependable, and future-proof, making it a useful software for contemporary customer support operations.
As companies proceed to prioritize effectivity and buyer expertise, implementing such clever workflows is a step towards sustaining a aggressive edge in at this time’s dynamic atmosphere.
Key Takeaways
- LangGraph automates advanced processes with modular, adaptable workflows.
- Steps like evaluation and rewriting enhance LLM output high quality.
- Correct state monitoring ensures easy transitions and constant outcomes.
- Conditional routing tailors processes to enter wants.
- Integrating analysis augments LLM outputs with related, knowledgeable content material.
Often Requested Questions
A. LangGraph is a state administration library designed for orchestrating and structuring workflows involving giant language fashions (LLMs). It supplies instruments for constructing and managing advanced workflows whereas preserving observe of the state of every course of step.
A. GROQ API supplies entry to GROQ-powered LLMs, providing high-performance pure language processing capabilities. It’s used for duties like textual content technology, summarization, classification, and extra, making it a necessary software for AI-driven purposes.
A. LangGraph supplies the construction and management stream for workflows, whereas GROQ API delivers the LLM capabilities for processing duties inside these workflows. For instance, LangGraph can outline a workflow the place GROQ’s LLM is used to categorize emails, draft responses, or carry out evaluation.
A. Sure, LangGraph lets you outline workflows with conditional paths primarily based on intermediate outputs. That is helpful for purposes like routing buyer inquiries primarily based on their content material.
A. GROQ API helps text-based inputs for duties like textual content classification, technology, and summarization. It will possibly additionally deal with structured knowledge when appropriately formatted.
The media proven on this article shouldn’t be owned by Analytics Vidhya and is used on the Creator’s discretion.