Introduction
Suppose as an example that you’re cooking a meal that can have a sure style that you simply need if solely the sequence of processes is adopted as anticipated. Likewise, in arithmetic and programming, getting factorial definition of a quantity requires a novel sequence of multiplication of a collection of decrement optimistic integers. Factorials’ operation is understood and used as a base attribute in a number of branches together with combinatorics, algebra, and pc sciences.
Wth the assistance of this text, the reader will learn to clear up a factorial in Python, reveal the that means of such a program, and perceive what approaches can be utilized to attain this aim.
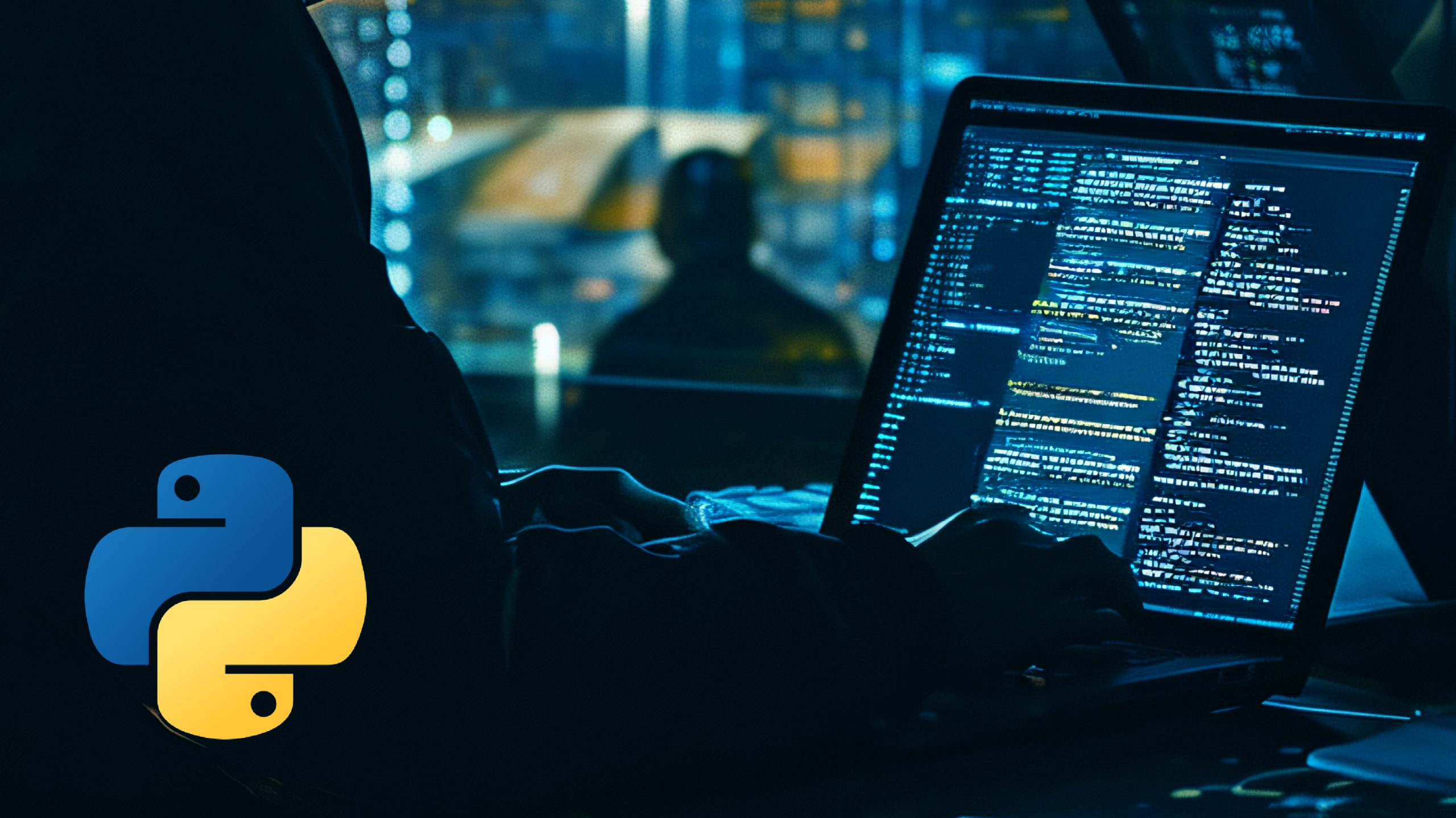
Studying Outcomes
- Study what a factorial is and it’s significance in arithmetic.
- Discover ways to write a factorial program in Python utilizing iterative and recursive methodologies to carry out operations.
- For particular questions concerning the calculations of factorials in Python you’ve got come to the suitable place.
What’s a Factorial?
A factorial of a non-negative integer ( n ) is the product of all optimistic integers lower than or equal to ( n ). It’s denoted by ( n! ).
For instance:
Particular Case:
Why Factorial is beneficial?
Factorials are broadly utilized in:
- Permutations and Combos: Determining what number of methods there are to decide on or arrange issues.
- Likelihood Idea: Recognizing the chance of happenings.
- Algebra and Calculus: Finishing collection expansions and equations.
- Laptop Algorithms: Implementing varied mathematical algorithms.
Writing a Factorial Program in Python
There are a number of methods to calculate the factorial of a quantity in Python. We’ll cowl the most typical strategies: iterative and recursive.
Iterative Methodology
Within the iterative technique, we use a loop to multiply the numbers in descending order.
def factorial_iterative(n):
outcome = 1
for i in vary(1, n + 1):
outcome *= i
return outcome
# Instance utilization
quantity = 5
print(f"The factorial of {quantity} is {factorial_iterative(quantity)}")
Output:
The factorial of 5 is 120
Recursive Methodology
Within the recursive method, a operate solves smaller circumstances of the identical drawback by invoking itself till it reaches the bottom case.
def factorial_recursive(n):
if n == 0 or n == 1:
return 1
else:
return n * factorial_recursive(n - 1)
# Instance utilization
quantity = 5
print(f"The factorial of {quantity} is {factorial_recursive(quantity)}")
Output:
The factorial of 5 is 120
Utilizing Python’s Constructed-in Operate
Python supplies a built-in operate within the math
module to calculate the factorial.
import math
quantity = 5
print(f"The factorial of {quantity} is {math.factorial(quantity)}")
Output:
The factorial of 5 is 120
Effectivity and Complexity
- Iterative Methodology: Since, the iterative strategy includes three operations and has a time complexity of O_(n) traversing via giant enter values and an area complexity of O of 1 with out requiring further house.
- Recursive Methodology: This recursive operate additionally has a time complexity of O(n) nevertheless it additionally served an area complexity of O(n) due to the decision stack, which will be problematic when dealing with very giant inputs.
- Constructed-in Methodology: Want utilizing the built-in operate for its effectivity, simplicity, and efficiency.
Conclusion
Computing the factorial of the given quantity is a straightforward operate in arithmetic and pc science. Python gives many approaches, starting from cycles to recursion and features with a built-in map. This data identifies the benefits and downsides of every technique to make sure the suitable technique is utilized in the suitable context. Whether or not you’re engaged on a combinatorial drawback or discovering an answer for a sure algorithm, with the ability to calculate factorials is all the time useful.
Steadily Requested Questions
A. A factorial of a non-negative integer ( n ) is the product of all optimistic integers lower than or equal to ( n ), denoted by ( n! ).
A. You may calculate the factorial utilizing iterative loops, recursion, or Python’s built-in math.factorial
operate.
A. Utilizing the built-in math.factorial
operate is usually essentially the most environment friendly and easiest technique.
A.Python’s recursion depth restrict and name stack measurement can restrict the recursive technique, making it much less appropriate for very giant inputs in comparison with the iterative technique.
A. Permutations, mixtures, chance concept, algebra, calculus, and quite a lot of pc methods all make use of factorials.