Introduction
Chatbots have remodeled the best way we have interaction with know-how, enabling automated, clever conversations throughout numerous domains. Constructing these chat methods will be difficult, particularly when aiming for flexibility and scalability. AutoGen simplifies this course of by leveraging AI brokers, which deal with complicated dialogues and duties autonomously. On this article, we’ll discover how you can construct agentic chatbots utilizing AutoGen. We’ll discover its highly effective agent-based framework that makes creating adaptive, clever conversational bots simpler than ever.
Overview
- Study what the AutoGen framework is all about and what it will possibly do.
- See how one can create chatbots that may maintain discussions with one another, reply to human queries, search the online, and do much more.
- Know the setup necessities and conditions wanted for constructing agentic chatbots utilizing AutoGen.
- Learn to improve chatbots by integrating instruments like Tavily for internet searches.
What’s AutoGen?
In AutoGen, all interactions are modelled as conversations between brokers. This agent-to-agent, chat-based communication streamlines the workflow, making it intuitive to begin constructing chatbots. The framework additionally provides flexibility by supporting numerous dialog patterns equivalent to sequential chats, group chats, and extra.
Let’s discover the AutoGen chatbot capabilities as we construct various kinds of chatbots:
- Dialectic between brokers: Two specialists in a subject focus on a subject and attempt to resolve their contradictions.
- Interview preparation chatbot: We’ll use an agent to organize for the interview by asking questions and evaluating the solutions.
- Chat with Internet search device: We will chat with a search device to get any data from the online.
Study Extra: Autogen: Exploring the Fundamentals of a Multi-Agent Framework
Conditions
Earlier than constructing AutoGen brokers, guarantee you could have the mandatory API keys for LLMs. We will even use Tavily to look the online.
Accessing by way of API
On this article, we’re utilizing OpenAI and Groq API keys. Groq provides entry to many open-source LLMs free of charge as much as some charge limits.
We will use any LLM we choose. Begin by producing an API key for the LLM and Tavily search device.
Create a .env file to securely retailer this key, protecting it personal whereas making it simply accessible inside your venture.

Libraries Required
autogen-agentchat – 0.2.36
tavily-python – 0.5.0
groq – 0.7.0
openai – 1.46.0
Dialectic Between Brokers
Dialectic is a technique of argumentation or reasoning that seeks to discover and resolve contradictions or opposing viewpoints. We let the 2 LLMs take part within the dialectic utilizing AutoGen brokers.
Let’s create our first agent:
from autogen import ConversableAgent
agent_1 = ConversableAgent(
identify="expert_1",
system_message="""You might be collaborating in a Dialectic about considerations of Generative AI with one other knowledgeable.
Make your factors on the thesis concisely.""",
llm_config={"config_list": [{"model": "gpt-4o-mini", "temperature": 0.5}]},
code_execution_config=False,
human_input_mode="NEVER",
)
Code Clarification
- ConversableAgent: That is the bottom class for constructing customizable brokers that may speak and work together with different brokers, individuals, and instruments to unravel duties.
- System Message: The system_message parameter defines the agent’s function and objective within the dialog. On this case, agent_1 is instructed to have interaction in a dialectic about generative AI, making concise factors on the thesis.
- llm_config: This configuration specifies the language mannequin for use, right here “gpt-4o-mini”. Further parameters like temperature=0.5 are set to manage the mannequin’s response creativity and variability.
- code_execution_config=False: This means that no code execution capabilities are enabled for the agent.
- human_input_mode=”NEVER”: This setting ensures the agent doesn’t depend on human enter, working solely autonomously.
Now the second agent
agent_2 = ConversableAgent(
"expert_2",
system_message="""You might be collaborating in a Dialectic about considerations of Generative AI with one other knowledgeable. Make your factors on the anti-thesis concisely.""",
llm_config={"config_list": [{"api_type": "groq", "model": "llama-3.1-70b-versatile", "temperature": 0.3}]},
code_execution_config=False,
human_input_mode="NEVER",
)
Right here, we are going to use the Llama 3.1 mannequin from Groq. To know how you can set completely different LLMs, we are able to refer right here.
Allow us to provoke the chat:
consequence = agent_1.initiate_chat(agent_2, message="""The character of knowledge assortment for coaching AI fashions pose inherent privateness dangers""",
max_turns=3, silent=False, summary_method="reflection_with_llm")
Code Clarification
On this code, agent_1 initiates a dialog with agent_2 utilizing the supplied message.
- max_turns=3: This limits the dialog to 3 exchanges between the brokers earlier than it mechanically ends.
- silent=False: This may show the dialog in real-time.
- summary_method=’reflection_with_llm’: This employs a big language mannequin (LLM) to summarize your complete dialogue between the brokers after the dialog concludes, offering a reflective abstract of their interplay.
You may undergo your complete dialectic utilizing the chat_history methodology.
Right here’s the consequence:
len(consequence.chat_history)
>>> 6
# every agent has 3 replies.
# we are able to additionally verify the associated fee incurred
print(consequence.price)
# get chathistory
print(consequence.chat_history)
# lastly abstract of the chat
print(consequence.abstract['content'])
Interview Preparation Chatbot
Along with making two brokers chat amongst themselves, we are able to additionally chat with an AI agent. Let’s do this by constructing an agent that can be utilized for interview preparation.
interviewer = ConversableAgent(
"interviewer",
system_message="""You might be interviewing to pick out for the Generative AI intern place.
Ask appropriate questions and consider the candidate.""",
llm_config={"config_list": [{"api_type": "groq", "model": "llama-3.1-70b-versatile", "temperature": 0.0}]},
code_execution_config=False,
human_input_mode="NEVER",
# max_consecutive_auto_reply=2,
is_termination_msg=lambda msg: "goodbye" in msg["content"].decrease()
)
Code Clarification
Use the system_message to outline the function of the agent.
To terminate the dialog we are able to use both of the under two parameters:
- max_consecutive_auto_reply: This parameter limits the variety of consecutive replies an agent can ship. As soon as the agent reaches this restrict, the dialog mechanically ends, stopping it from persevering with indefinitely.
- is_termination_msg: This parameter checks if a message accommodates a particular pre-defined key phrase. When this key phrase is detected, the dialog is mechanically terminated.
candidate = ConversableAgent(
"candidate",
system_message="""You might be attending an interview for the Generative AI intern place.
Reply the questions accordingly""",
llm_config=False,
code_execution_config=False,
human_input_mode="ALWAYS",
)
For the reason that consumer goes to offer the reply, we are going to use human_input_mode=”ALWAYS” and llm_config=False
Now, we are able to initialize the mock interview:
consequence = candidate.initiate_chat(interviewer, message="Hello, thanks for calling me.", summary_method="reflection_with_llm")
# we are able to get the abstract of the dialog too
print(consequence.abstract)
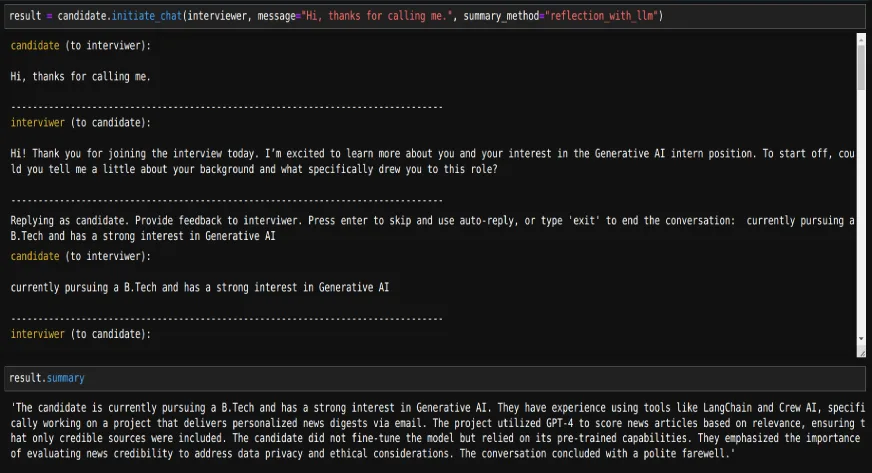
Chat with Internet Search
Now, let’s construct a chatbot that may use the web to seek for the queries requested.
For this, first, outline a operate that searches the online utilizing Tavily.
from tavily import TavilyClient
from autogen import register_function
def web_search(question: str):
tavily_client = TavilyClient()
response = tavily_client.search(question, max_results=3)
return response['results']
An assistant agent which decides to name the device or terminate
assistant = ConversableAgent(
identify="Assistant",
system_message="""You're a useful AI assistant. You may search internet to get the outcomes.
Return 'TERMINATE' when the duty is finished.""",
llm_config={"config_list": [{"model": "gpt-4o-mini"}]},
silent=True,
)
The consumer proxy agent is used for interacting with the assistant agent and executes device calls.
user_proxy = ConversableAgent(
identify="Person",
llm_config=False,
is_termination_msg=lambda msg: msg.get("content material") isn't None and "TERMINATE" in msg["content"],
human_input_mode="TERMINATE",
)
When the termination situation is met, it should ask for human enter. We will both proceed to question or finish the chat.
Register the operate for the 2 brokers:
register_function(
web_search,
caller=assistant, # The assistant agent can recommend calls to the calculator.
executor=user_proxy, # The consumer proxy agent can execute the calculator calls.
identify="web_search", # By default, the operate identify is used because the device identify.
description="Searches web to get the outcomes a for given question", # An outline of the device.
)
Now we are able to question:
chat_result = user_proxy.initiate_chat(assistant, message="Who gained the Nobel prizes in 2024")
# Relying on the size of the chat historical past we are able to entry the mandatory content material
print(chat_result.chat_history[5]['content'])
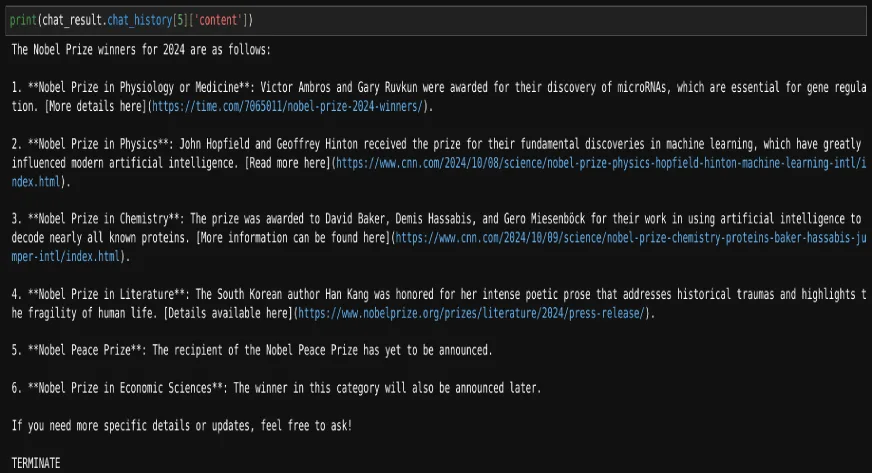
On this method, we are able to construct various kinds of agentic chatbots utilizing AutoGen.
Additionally Learn: Strategic Staff Constructing with AutoGen AI
Conclusion
On this article, we realized how you can construct agentic chatbots utilizing AutoGen and explored their numerous capabilities. With its agent-based structure, builders can construct versatile and scalable bots able to complicated interactions, equivalent to dialectics and internet searches. AutoGen’s simple setup and gear integration empower customers to craft custom-made conversational brokers for numerous functions. As AI-driven communication evolves, AutoGen serves as a precious framework for simplifying and enhancing chatbot growth, enabling partaking consumer interactions.
To grasp AI brokers, checkout our Agentic AI Pioneer Program.
Regularly Requested Questions
A. AutoGen is a framework that simplifies the event of chatbots through the use of an agent-based structure, permitting for versatile and scalable conversational interactions.
A. Sure, AutoGen helps numerous dialog patterns, together with sequential and group chats, permitting builders to tailor interactions primarily based on their wants.
A. AutoGen makes use of agent-to-agent communication, enabling a number of brokers to have interaction in structured dialogues, equivalent to dialectics, making it simpler to handle complicated conversational situations.
A. You may terminate a chat in AutoGen through the use of parameters like `max_consecutive_auto_reply`, which limits the variety of consecutive replies, or `is_termination_msg`, which checks for particular key phrases within the dialog to set off an automated finish. We will additionally use max_turns to restrict the dialog.
A. Auogen permits brokers to make use of exterior instruments, like Tavily for internet searches, by registering features that the brokers can name throughout conversations, enhancing the chatbot’s capabilities with real-time knowledge and extra performance.