CUDA for Machine Studying: Sensible Purposes
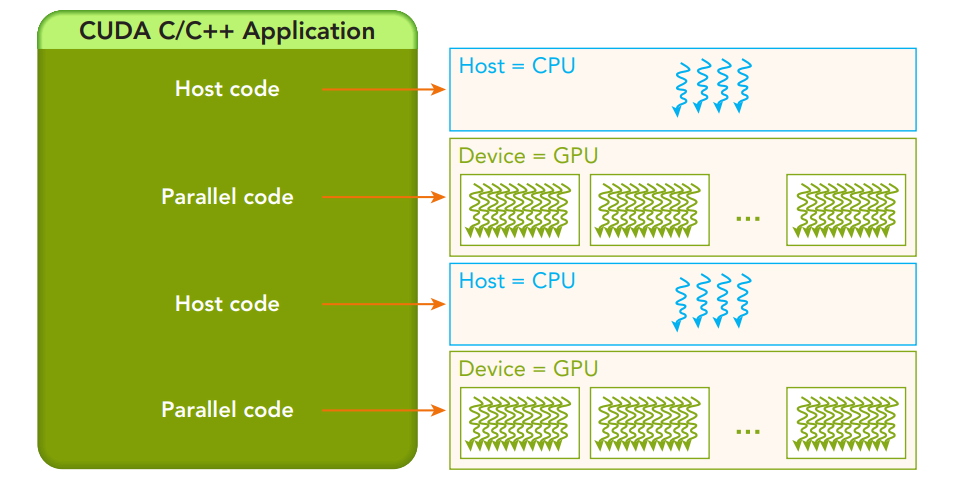
Construction of a CUDA C/C++ utility, the place the host (CPU) code manages the execution of parallel code on the machine (GPU).
Now that we have coated the fundamentals, let’s discover how CUDA may be utilized to frequent machine studying duties.
-
Matrix Multiplication
Matrix multiplication is a basic operation in lots of machine studying algorithms, notably in neural networks. CUDA can considerably speed up this operation. This is a easy implementation:
__global__ void matrixMulKernel(float *A, float *B, float *C, int N) { int row = blockIdx.y * blockDim.y + threadIdx.y; int col = blockIdx.x * blockDim.x + threadIdx.x; float sum = 0.0f; if (row < N && col < N) { for (int i = 0; i < N; i++) { sum += A[row * N + i] * B[i * N + col]; } C[row * N + col] = sum; } } // Host perform to arrange and launch the kernel void matrixMul(float *A, float *B, float *C, int N) { dim3 threadsPerBlock(16, 16); dim3 numBlocks((N + threadsPerBlock.x - 1) / threadsPerBlock.x, (N + threadsPerBlock.y - 1) / threadsPerBlock.y); matrixMulKernelnumBlocks, threadsPerBlock(A, B, C, N); }
This implementation divides the output matrix into blocks, with every thread computing one component of the end result. Whereas this primary model is already sooner than a CPU implementation for giant matrices, there’s room for optimization utilizing shared reminiscence and different strategies.
-
Convolution Operations
Convolutional Neural Networks (CNNs) rely closely on convolution operations. CUDA can dramatically pace up these computations. This is a simplified 2D convolution kernel:
__global__ void convolution2DKernel(float *enter, float *kernel, float *output, int inputWidth, int inputHeight, int kernelWidth, int kernelHeight) { int x = blockIdx.x * blockDim.x + threadIdx.x; int y = blockIdx.y * blockDim.y + threadIdx.y; if (x < inputWidth && y < inputHeight) { float sum = 0.0f; for (int ky = 0; ky < kernelHeight; ky++) { for (int kx = 0; kx < kernelWidth; kx++) { int inputX = x + kx - kernelWidth / 2; int inputY = y + ky - kernelHeight / 2; if (inputX >= 0 && inputX < inputWidth && inputY >= 0 && inputY < inputHeight) { sum += enter[inputY * inputWidth + inputX] * kernel[ky * kernelWidth + kx]; } } } output[y * inputWidth + x] = sum; } }
This kernel performs a 2D convolution, with every thread computing one output pixel. In observe, extra subtle implementations would use shared reminiscence to cut back world reminiscence accesses and optimize for varied kernel sizes.
-
Stochastic Gradient Descent (SGD)
SGD is a cornerstone optimization algorithm in machine studying. CUDA can parallelize the computation of gradients throughout a number of knowledge factors. This is a simplified instance for linear regression:
__global__ void sgdKernel(float *X, float *y, float *weights, float learningRate, int n, int d) { int i = blockIdx.x * blockDim.x + threadIdx.x; if (i < n) { float prediction = 0.0f; for (int j = 0; j < d; j++) { prediction += X[i * d + j] * weights[j]; } float error = prediction - y[i]; for (int j = 0; j < d; j++) { atomicAdd(&weights[j], -learningRate * error * X[i * d + j]); } } } void sgd(float *X, float *y, float *weights, float learningRate, int n, int d, int iterations) { int threadsPerBlock = 256; int numBlocks = (n + threadsPerBlock - 1) / threadsPerBlock; for (int iter = 0; iter < iterations; iter++) { sgdKernel<<<numBlocks, threadsPerBlock>>>(X, y, weights, learningRate, n, d); } }
This implementation updates the weights in parallel for every knowledge level. The atomicAdd
perform is used to deal with concurrent updates to the weights safely.
Optimizing CUDA for Machine Studying
Whereas the above examples display the fundamentals of utilizing CUDA for machine studying duties, there are a number of optimization strategies that may additional improve efficiency:
-
Coalesced Reminiscence Entry
GPUs obtain peak efficiency when threads in a warp entry contiguous reminiscence places. Guarantee your knowledge constructions and entry patterns promote coalesced reminiscence entry.
-
Shared Reminiscence Utilization
Shared reminiscence is way sooner than world reminiscence. Use it to cache continuously accessed knowledge inside a thread block.
This diagram illustrates the structure of a multi-processor system with shared reminiscence. Every processor has its personal cache, permitting for quick entry to continuously used knowledge. The processors talk by way of a shared bus, which connects them to a bigger shared reminiscence area.
For instance, in matrix multiplication:
__global__ void matrixMulSharedKernel(float *A, float *B, float *C, int N) { __shared__ float sharedA[TILE_SIZE][TILE_SIZE]; __shared__ float sharedB[TILE_SIZE][TILE_SIZE]; int bx = blockIdx.x; int by = blockIdx.y; int tx = threadIdx.x; int ty = threadIdx.y; int row = by * TILE_SIZE + ty; int col = bx * TILE_SIZE + tx; float sum = 0.0f; for (int tile = 0; tile < (N + TILE_SIZE - 1) / TILE_SIZE; tile++) { if (row < N && tile * TILE_SIZE + tx < N) sharedA[ty][tx] = A[row * N + tile * TILE_SIZE + tx]; else sharedA[ty][tx] = 0.0f; if (col < N && tile * TILE_SIZE + ty < N) sharedB[ty][tx] = B[(tile * TILE_SIZE + ty) * N + col]; else sharedB[ty][tx] = 0.0f; __syncthreads(); for (int okay = 0; okay < TILE_SIZE; okay++) sum += sharedA[ty][k] * sharedB[k][tx]; __syncthreads(); } if (row < N && col < N) C[row * N + col] = sum; }
This optimized model makes use of shared reminiscence to cut back world reminiscence accesses, considerably bettering efficiency for giant matrices.
-
Asynchronous Operations
CUDA helps asynchronous operations, permitting you to overlap computation with knowledge switch. That is notably helpful in machine studying pipelines the place you possibly can put together the following batch of knowledge whereas the present batch is being processed.
cudaStream_t stream1, stream2; cudaStreamCreate(&stream1); cudaStreamCreate(&stream2); // Asynchronous reminiscence transfers and kernel launches cudaMemcpyAsync(d_data1, h_data1, dimension, cudaMemcpyHostToDevice, stream1); myKernel<<<grid, block, 0, stream1>>>(d_data1, ...); cudaMemcpyAsync(d_data2, h_data2, dimension, cudaMemcpyHostToDevice, stream2); myKernel<<<grid, block, 0, stream2>>>(d_data2, ...); cudaStreamSynchronize(stream1); cudaStreamSynchronize(stream2);
-
Tensor Cores
For machine studying workloads, NVIDIA’s Tensor Cores (out there in newer GPU architectures) can present vital speedups for matrix multiply and convolution operations. Libraries like cuDNN and cuBLAS robotically leverage Tensor Cores when out there.
Challenges and Concerns
Whereas CUDA affords great advantages for machine studying, it is essential to pay attention to potential challenges:
- Reminiscence Administration: GPU reminiscence is proscribed in comparison with system reminiscence. Environment friendly reminiscence administration is essential, particularly when working with giant datasets or fashions.
- Information Switch Overhead: Transferring knowledge between CPU and GPU is usually a bottleneck. Decrease transfers and use asynchronous operations when potential.
- Precision: GPUs historically excel at single-precision (FP32) computations. Whereas assist for double-precision (FP64) has improved, it is typically slower. Many machine studying duties can work nicely with decrease precision (e.g., FP16), which trendy GPUs deal with very effectively.
- Code Complexity: Writing environment friendly CUDA code may be extra advanced than CPU code. Leveraging libraries like cuDNN, cuBLAS, and frameworks like TensorFlow or PyTorch can assist summary away a few of this complexity.
As machine studying fashions develop in dimension and complexity, a single GPU might not be adequate to deal with the workload. CUDA makes it potential to scale your utility throughout a number of GPUs, both inside a single node or throughout a cluster.
CUDA Programming Construction
To successfully make the most of CUDA, it is important to grasp its programming construction, which entails writing kernels (capabilities that run on the GPU) and managing reminiscence between the host (CPU) and machine (GPU).
Host vs. Machine Reminiscence
In CUDA, reminiscence is managed individually for the host and machine. The next are the first capabilities used for reminiscence administration:
- cudaMalloc: Allocates reminiscence on the machine.
- cudaMemcpy: Copies knowledge between host and machine.
- cudaFree: Frees reminiscence on the machine.
Instance: Summing Two Arrays
Let’s have a look at an instance that sums two arrays utilizing CUDA:
__global__ void sumArraysOnGPU(float *A, float *B, float *C, int N) { int idx = threadIdx.x + blockIdx.x * blockDim.x; if (idx < N) C[idx] = A[idx] + B[idx]; } int essential() { int N = 1024; size_t bytes = N * sizeof(float); float *h_A, *h_B, *h_C; h_A = (float*)malloc(bytes); h_B = (float*)malloc(bytes); h_C = (float*)malloc(bytes); float *d_A, *d_B, *d_C; cudaMalloc(&d_A, bytes); cudaMalloc(&d_B, bytes); cudaMalloc(&d_C, bytes); cudaMemcpy(d_A, h_A, bytes, cudaMemcpyHostToDevice); cudaMemcpy(d_B, h_B, bytes, cudaMemcpyHostToDevice); int blockSize = 256; int gridSize = (N + blockSize - 1) / blockSize; sumArraysOnGPU<<<gridSize, blockSize>>>(d_A, d_B, d_C, N); cudaMemcpy(h_C, d_C, bytes, cudaMemcpyDeviceToHost); cudaFree(d_A); cudaFree(d_B); cudaFree(d_C); free(h_A); free(h_B); free(h_C); return 0; }
On this instance, reminiscence is allotted on each the host and machine, knowledge is transferred to the machine, and the kernel is launched to carry out the computation.
Conclusion
CUDA is a robust software for machine studying engineers seeking to speed up their fashions and deal with bigger datasets. By understanding the CUDA reminiscence mannequin, optimizing reminiscence entry, and leveraging a number of GPUs, you possibly can considerably improve the efficiency of your machine studying purposes.