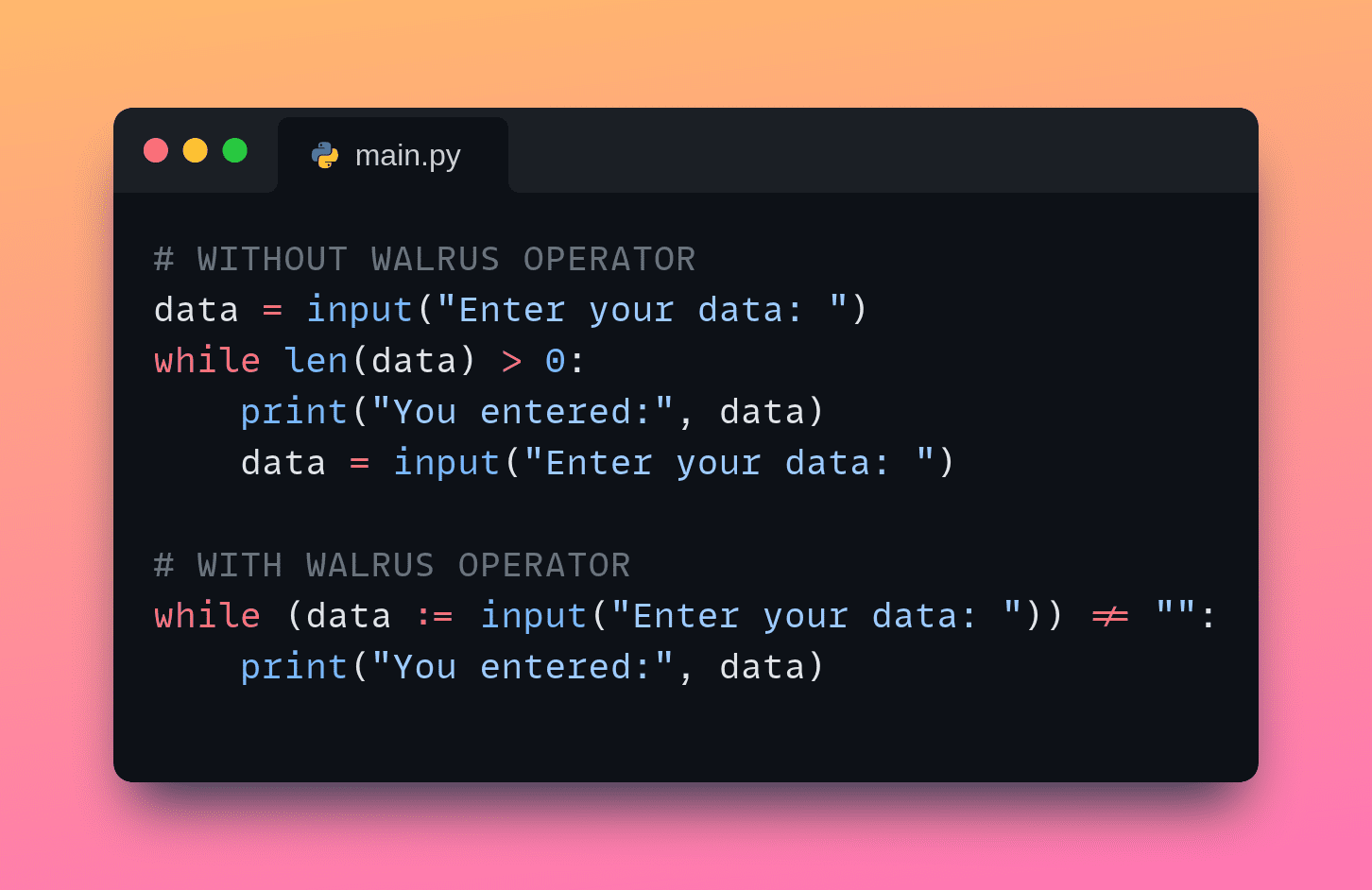
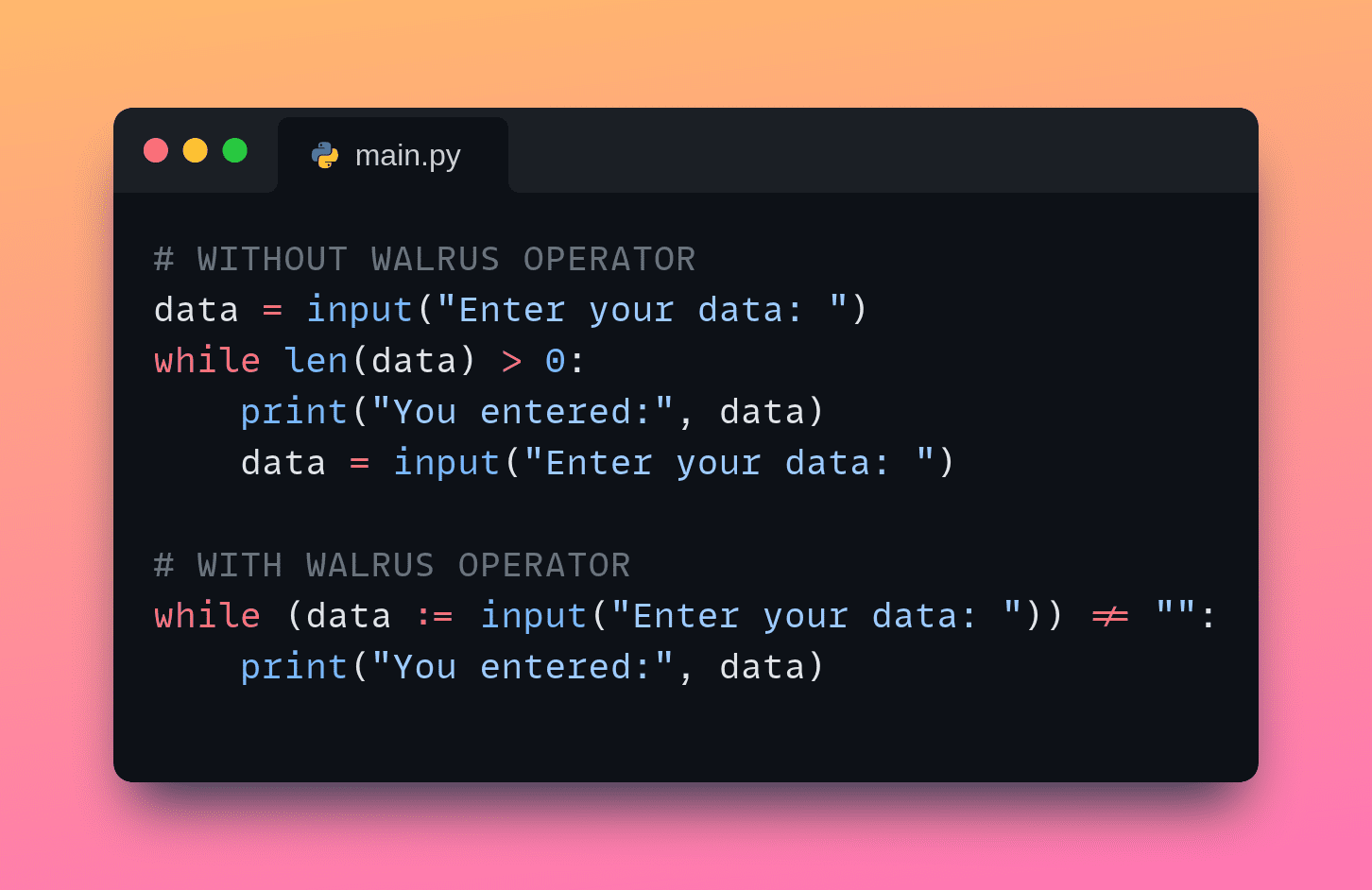
Picture by Creator | Created on Snappify
In Python, if you wish to assign values to variables inside an expression, you should use the Walrus operator :=. Whereas x = 5
is a straightforward variable task, x := 5
is how you will use the Walrus operator.
This operator was launched in Python 3.8 and will help you write extra concise and probably extra readable code (in some circumstances). Nonetheless, utilizing it when not essential or greater than essential may also make code more durable to know.
On this tutorial, we’ll discover each the efficient and the not-so-effective makes use of of the Walrus operator with easy code examples. Let’s get began!
How and When Python’s Walrus Operator is Useful
Let’s begin by taking a look at examples the place the walrus operator could make your code higher.
1. Extra Concise Loops
It is fairly widespread to have loop constructs the place you learn in an enter to course of inside the loop and the looping situation relies on the enter. In such circumstances, utilizing the walrus operator helps you retain your loops cleaner.
With out Walrus Operator
Contemplate the next instance:
information = enter("Enter your information: ")
whereas len(information) > 0:
print("You entered:", information)
information = enter("Enter your information: ")
Once you run the above code, you’ll be repeatedly prompted to enter a worth as long as you enter a non-empty string.
Be aware that there’s redundancy. You initially learn within the enter to the information
variable. Inside the loop, you print out the entered worth and immediate the consumer for enter once more. The looping situation checks if the string is non-empty.
With Walrus Operator
You possibly can take away the redundancy and rewrite the above model utilizing the walrus operator. To take action, you possibly can learn within the enter and examine if it’s a non-empty string—all inside the looping situation—utilizing the walrus operator like so:
whereas (information := enter("Enter your information: ")) != "":
print("You entered:", information)
Now that is extra concise than the primary model.
2. Higher Checklist Comprehensions
You’ll typically have perform calls inside checklist comprehensions. This may be inefficient if there are a number of costly perform calls. In these circumstances, rewriting utilizing the walrus operator will be useful.
With out Walrus Operator
Take the next instance the place there are two calls to the `compute_profit` perform within the checklist comprehension expression:
- To populate the brand new checklist with the revenue worth and
- To examine if the revenue worth is above a specified threshold.
# Perform to compute revenue
def compute_profit(gross sales, value):
return gross sales - value
# With out Walrus Operator
sales_data = [(100, 70), (200, 150), (150, 100), (300, 200)]
earnings = [compute_profit(sales, cost) for sales, cost in sales_data if compute_profit(sales, cost) > 50]
With Walrus Operator
You possibly can assign the return values from the perform name to the `revenue` variable and use that the populate the checklist like so:
# Perform to compute revenue
def compute_profit(gross sales, value):
return gross sales - value
# With Walrus Operator
sales_data = [(100, 70), (200, 150), (150, 100), (300, 200)]
earnings = [profit for sales, cost in sales_data if (profit := compute_profit(sales, cost)) > 50]
This model is healthier if the filtering situation includes an costly perform name.
How To not Use Python’s Walrus Operator
Now that we’ve seen a number of examples of how and when you should use Python’s walrus operator, let’s see a number of anti-patterns.
1. Advanced Checklist Comprehensions
We used the walrus operator inside an inventory comprehension to keep away from repeated perform calls in a earlier instance. However overusing the walrus operator will be simply as dangerous.
The next checklist comprehension is tough to learn because of a number of nested situations and assignments.
# Perform to compute revenue
def compute_profit(gross sales, value):
return gross sales - value
# Messy checklist comprehension with nested walrus operator
sales_data = [(100, 70), (200, 150), (150, 100), (300, 200)]
outcomes = [
(sales, cost, profit, sales_ratio)
for sales, cost in sales_data
if (profit := compute_profit(sales, cost)) > 50
if (sales_ratio := sales / cost) > 1.5
if (profit_margin := (profit / sales)) > 0.2
]
As an train, you possibly can attempt breaking down the logic into separate steps—inside an everyday loop and if conditional statements. This may make the code a lot simpler to learn and keep.
2. Nested Walrus Operators
Utilizing nested walrus operators can result in code that’s troublesome to each learn and keep. That is notably problematic when the logic includes a number of assignments and situations inside a single expression.
# Instance of nested walrus operators
values = [5, 15, 25, 35, 45]
threshold = 20
outcomes = []
for worth in values:
if (above_threshold := worth > threshold) and (incremented := (new_value := worth + 10) > 30):
outcomes.append(new_value)
print(outcomes)
On this instance, the nested walrus operators make it obscure—requiring the reader to unpack a number of layers of logic inside a single line, decreasing readability.
Wrapping Up
On this fast tutorial, we went over how and when to and when to not use Python’s walrus operator. You will discover the code examples on GitHub.
For those who’re searching for widespread gotchas to keep away from when programming with Python, learn 5 Frequent Python Gotchas and The way to Keep away from Them.
Hold coding!
Bala Priya C is a developer and technical author from India. She likes working on the intersection of math, programming, information science, and content material creation. Her areas of curiosity and experience embrace DevOps, information science, and pure language processing. She enjoys studying, writing, coding, and occasional! At present, she’s engaged on studying and sharing her information with the developer neighborhood by authoring tutorials, how-to guides, opinion items, and extra. Bala additionally creates partaking useful resource overviews and coding tutorials.