Evaluating giant language fashions (LLMs) is crucial. You want to perceive how properly they carry out and guarantee they meet your requirements. The Hugging Face Consider library affords a useful set of instruments for this job. This information exhibits you find out how to use the Consider library to evaluate LLMs with sensible code examples.
Understanding the Hugging Face Consider Library
The Hugging Face Consider library offers instruments for various analysis wants. These instruments fall into three fundamental classes:
- Metrics: These measure a mannequin’s efficiency by evaluating its predictions to floor reality labels. Examples embody accuracy, F1-score, BLEU, and ROUGE.
- Comparisons: These assist examine two fashions, usually by analyzing how their predictions align with one another or with reference labels.
- Measurements: These instruments examine the properties of datasets themselves, like calculating textual content complexity or label distributions.
You may entry all these analysis modules utilizing a single operate: consider.load().
Getting Began
Set up
First, you should set up the library. Open your terminal or command immediate and run:
pip set up consider
pip set up rouge_score # Wanted for textual content era metrics
pip set up consider[visualization] # For plotting capabilities
These instructions set up the core consider library, the rouge_score bundle (required for the ROUGE metric usually utilized in summarization), and non-compulsory dependencies for visualization like radar plots.
Loading an Analysis Module
To make use of a particular analysis instrument, you load it by identify. As an example, to load the accuracy metric:
import consider
accuracy_metric = consider.load("accuracy")
print("Accuracy metric loaded.")
Output:

This code imports the consider library and masses the accuracy metric object. You’ll use this object to compute accuracy scores.
Fundamental Analysis Examples
Let’s stroll by some widespread analysis eventualities.
Computing Accuracy Immediately
You may compute a metric by offering all references (floor reality) and predictions directly.
import consider
# Load the accuracy metric
accuracy_metric = consider.load("accuracy")
# Pattern floor reality and predictions
references = [0, 1, 0, 1]
predictions = [1, 0, 0, 1]
# Compute accuracy
outcome = accuracy_metric.compute(references=references, predictions=predictions)
print(f"Direct computation outcome: {outcome}")
# Instance with exact_match metric
exact_match_metric = consider.load('exact_match')
match_result = exact_match_metric.compute(references=['hello world'], predictions=['hello world'])
no_match_result = exact_match_metric.compute(references=['hello'], predictions=['hell'])
print(f"Actual match outcome (match): {match_result}")
print(f"Actual match outcome (no match): {no_match_result}")
Output:

Clarification:
- We outline two lists: references holds the proper labels, and predictions holds the mannequin’s outputs.
- The compute methodology takes these lists and calculates the accuracy, returning the outcome as a dictionary.
- We additionally present the exact_match metric, which checks if the prediction completely matches the reference.
Incremental Analysis (Utilizing add_batch)
For giant datasets, processing predictions in batches may be extra memory-efficient. You may add batches incrementally and compute the ultimate rating on the finish.
import consider
# Load the accuracy metric
accuracy_metric = consider.load("accuracy")
# Pattern batches of refrences and predictions
references_batch1 = [0, 1]
predictions_batch1 = [1, 0]
references_batch2 = [0, 1]
predictions_batch2 = [0, 1]
# Add batches incrementally
accuracy_metric.add_batch(references=references_batch1, predictions=predictions_batch1)
accuracy_metric.add_batch(references=references_batch2, predictions=predictions_batch2)
# Compute remaining accuracy
final_result = accuracy_metric.compute()
print(f"Incremental computation outcome: {final_result}")
Output:

Clarification:
- We simulate processing information in two batches.
- add_batch updates the metric’s inner state with every batch.
- Calling compute() with out arguments calculates the metric over all added batches.
Combining A number of Metrics
You usually need to calculate a number of metrics concurrently (e.g., accuracy, F1, precision, recall for classification). The consider.mix operate simplifies this.
import consider
# Mix a number of classification metrics
clf_metrics = consider.mix(["accuracy", "f1", "precision", "recall"])
# Pattern information
predictions = [0, 1, 0]
references = [0, 1, 1] # Observe: The final prediction is wrong
# Compute all metrics directly
outcomes = clf_metrics.compute(predictions=predictions, references=references)
print(f"Mixed metrics outcome: {outcomes}")
Output:

Clarification:
- consider.mix takes a listing of metric names and returns a mixed analysis object.
- Calling compute on this object calculates all the required metrics utilizing the identical enter information.
Utilizing Measurements
Measurements can be utilized to investigate datasets. Right here’s find out how to use the word_length measurement:
import consider
# Load the word_length measurement
# Observe: Could require NLTK information obtain on first run
attempt:
word_length = consider.load("word_length", module_type="measurement")
information = ["hello world", "this is another sentence"]
outcomes = word_length.compute(information=information)
print(f"Phrase size measurement outcome: {outcomes}")
besides Exception as e:
print(f"Couldn't run word_length measurement, presumably NLTK information lacking: {e}")
print("Making an attempt NLTK obtain...")
import nltk
nltk.obtain('punkt') # Uncomment and run if wanted
Output:

Clarification:
- We load word_length and specify module_type=”measurement”.
- The compute methodology takes the dataset (a listing of strings right here) as enter.
- It returns statistics in regards to the phrase lengths within the offered information. (Observe: Requires nltk and its ‘punkt’ tokenizer information).
Evaluating Particular NLP Duties
Completely different NLP duties require particular metrics. Hugging Face Consider consists of many normal ones.
Machine Translation (BLEU)
BLEU (Bilingual Analysis Understudy) is widespread for translation high quality. It measures n-gram overlap between the mannequin’s translation (speculation) and reference translations.
import consider
def evaluate_machine_translation(hypotheses, references):
"""Calculates BLEU rating for machine translation."""
bleu_metric = consider.load("bleu")
outcomes = bleu_metric.compute(predictions=hypotheses, references=references)
# Extract the primary BLEU rating
bleu_score = outcomes["bleu"]
return bleu_score
# Instance hypotheses (mannequin translations)
hypotheses = ["the cat sat on mat.", "the dog played in garden."]
# Instance references (right translations, can have a number of per speculation)
references = [["the cat sat on the mat."], ["the dog played in the garden."]]
bleu_score = evaluate_machine_translation(hypotheses, references)
print(f"BLEU Rating: {bleu_score:.4f}") # Format for readability
Output:

Clarification:
- The operate masses the BLEU metric.
- It computes the rating evaluating predicted translations (hypotheses) towards a number of right references.
- A better BLEU rating (nearer to 1.0) typically signifies higher translation high quality, suggesting extra overlap with reference translations. A rating round 0.51 suggests reasonable overlap.
Named Entity Recognition (NER – utilizing seqeval)
For sequence labeling duties like NER, metrics like precision, recall, and F1-score per entity kind are helpful. The seqeval metric handles this format (e.g., B-PER, I-PER, O tags).
To run the next code, seqeval library can be required. It may very well be put in by working the next command:
pip set up seqeval
Code:
import consider
# Load the seqeval metric
attempt:
seqeval_metric = consider.load("seqeval")
# Instance labels (utilizing IOB format)
true_labels = [['O', 'B-PER', 'I-PER', 'O'], ['B-LOC', 'I-LOC', 'O']]
predicted_labels = [['O', 'B-PER', 'I-PER', 'O'], ['B-LOC', 'I-LOC', 'O']] # Instance: Excellent prediction right here
outcomes = seqeval_metric.compute(predictions=predicted_labels, references=true_labels)
print("Seqeval Outcomes (per entity kind):")
# Print outcomes properly
for key, worth in outcomes.gadgets():
if isinstance(worth, dict):
print(f" {key}: Precision={worth['precision']:.2f}, Recall={worth['recall']:.2f}, F1={worth['f1']:.2f}, Quantity={worth['number']}")
else:
print(f" {key}: {worth:.4f}")
besides ModuleNotFoundError:
print("Seqeval metric not put in. Run: pip set up seqeval")
Output:
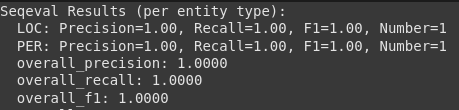
Clarification:
- We load the seqeval metric.
- It takes lists of lists, the place every internal listing represents the tags for a sentence.
- The compute methodology returns detailed precision, recall, and F1 scores for every entity kind recognized (like PER for Individual, LOC for Location) and general scores.
Textual content Summarization (ROUGE)
ROUGE (Recall-Oriented Understudy for Gisting Analysis) compares a generated abstract towards reference summaries, specializing in overlapping n-grams and longest widespread subsequences.
import consider
def simple_summarizer(textual content):
"""A really fundamental summarizer - simply takes the primary sentence."""
attempt:
sentences = textual content.cut up(".")
return sentences[0].strip() + "." if sentences[0].strip() else ""
besides:
return "" # Deal with empty or malformed textual content
# Load ROUGE metric
rouge_metric = consider.load("rouge")
# Instance textual content and reference abstract
textual content = "At present is a phenomenal day. The solar is shining and the birds are singing. I'm going for a stroll within the park."
reference = "The climate is nice at this time."
# Generate abstract utilizing the straightforward operate
prediction = simple_summarizer(textual content)
print(f"Generated Abstract: {prediction}")
print(f"Reference Abstract: {reference}")
# Compute ROUGE scores
rouge_results = rouge_metric.compute(predictions=[prediction], references=[reference])
print(f"ROUGE Scores: {rouge_results}")
Output:
Generated Abstract: At present is a phenomenal day.Reference Abstract: The climate is nice at this time.
ROUGE Scores: {'rouge1': np.float64(0.4000000000000001), 'rouge2':
np.float64(0.0), 'rougeL': np.float64(0.20000000000000004), 'rougeLsum':
np.float64(0.20000000000000004)}
Clarification:
- We load the rouge metric.
- We outline a simplistic summarizer for demonstration.
- compute calculates completely different ROUGE scores:
- Scores nearer to 1.0 point out increased similarity to the reference abstract. The low scores right here mirror the essential nature of our simple_summarizer.
Query Answering (SQuAD)
The SQuAD metric is used for extractive query answering benchmarks. It calculates Actual Match (EM) and F1-score.
import consider
# Load the SQuAD metric
squad_metric = consider.load("squad")
# Instance predictions and references format for SQuAD
predictions = [{'prediction_text': '1976', 'id': '56e10a3be3433e1400422b22'}]
references = [{'answers': {'answer_start': [97], 'textual content': ['1976']}, 'id': '56e10a3be3433e1400422b22'}]
outcomes = squad_metric.compute(predictions=predictions, references=references)
print(f"SQuAD Outcomes: {outcomes}")
Output:

Clarification:
- Hundreds the squad metric.
- Takes predictions and references in a particular dictionary format, together with the anticipated textual content and the bottom reality solutions with their begin positions.
- exact_match: Share of predictions that precisely match one of many floor reality solutions.
- f1: Common F1 rating over all questions, contemplating partial matches on the token degree.
Superior Analysis with the Evaluator Class
The Evaluator class streamlines the method by integrating mannequin loading, inference, and metric calculation. It’s notably helpful for traditional duties like textual content classification.
# Observe: Requires transformers and datasets libraries
# pip set up transformers datasets torch # or tensorflow/jax
import consider
from consider import evaluator
from transformers import pipeline
from datasets import load_dataset
# Load a pre-trained textual content classification pipeline
# Utilizing a smaller mannequin for probably quicker execution
attempt:
pipe = pipeline("text-classification", mannequin="distilbert-base-uncased-finetuned-sst-2-english", machine=-1) # Use CPU
besides Exception as e:
print(f"Couldn't load pipeline: {e}")
pipe = None
if pipe:
# Load a small subset of the IMDB dataset
attempt:
information = load_dataset("imdb", cut up="check").shuffle(seed=42).choose(vary(100)) # Smaller subset for pace
besides Exception as e:
print(f"Couldn't load dataset: {e}")
information = None
if information:
# Load the accuracy metric
accuracy_metric = consider.load("accuracy")
# Create an evaluator for the duty
task_evaluator = evaluator("text-classification")
# Right label_mapping for IMDB dataset
label_mapping = {
'NEGATIVE': 0, # Map NEGATIVE to 0
'POSITIVE': 1 # Map POSITIVE to 1
}
# Compute outcomes
eval_results = task_evaluator.compute(
model_or_pipeline=pipe,
information=information,
metric=accuracy_metric,
input_column="textual content", # Specify the textual content column
label_column="label", # Specify the label column
label_mapping=label_mapping # Move the corrected label mapping
)
print("nEvaluator Outcomes:")
print(eval_results)
# Compute with bootstrapping for confidence intervals
bootstrap_results = task_evaluator.compute(
model_or_pipeline=pipe,
information=information,
metric=accuracy_metric,
input_column="textual content",
label_column="label",
label_mapping=label_mapping, # Move the corrected label mapping
technique="bootstrap",
n_resamples=10 # Use fewer resamples for quicker demo
)
print("nEvaluator Outcomes with Bootstrapping:")
print(bootstrap_results)
Output:
Gadget set to make use of cpuEvaluator Outcomes:
{'accuracy': 0.9, 'total_time_in_seconds': 24.277618517999997,
'samples_per_second': 4.119020155368932, 'latency_in_seconds':
0.24277618517999996}Evaluator Outcomes with Bootstrapping:
{'accuracy': {'confidence_interval': (np.float64(0.8703044820750653),
np.float64(0.9335706530476571)), 'standard_error':
np.float64(0.02412928142780514), 'rating': 0.9}, 'total_time_in_seconds':
23.871316319000016, 'samples_per_second': 4.189128017226537,
'latency_in_seconds': 0.23871316319000013}
Clarification:
- We load a transformers pipeline for textual content classification and a pattern of the IMDb dataset.
- We create an evaluator particularly for “text-classification”.
- The compute methodology handles feeding information (textual content column) to the pipeline, getting predictions, evaluating them to the true labels (label column) utilizing the required metric, and making use of the label_mapping.
- It returns the metric rating together with efficiency stats like complete time and samples per second.
- Utilizing technique=”bootstrap” performs resampling to estimate confidence intervals and normal error for the metric, giving a way of the rating’s stability.
Utilizing Analysis Suites
Analysis Suites bundle a number of evaluations, usually focusing on particular benchmarks like GLUE. This enables working a mannequin towards an ordinary set of duties.
# Observe: Operating a full suite may be computationally intensive and time-consuming.
# This instance demonstrates the idea however may take a very long time or require important assets.
# It additionally installs a number of datasets and will require particular mannequin configurations.
import consider
attempt:
print("nLoading GLUE analysis suite (this may obtain datasets)...")
# Load the GLUE job instantly
# Utilizing "mrpc" for instance job, however you'll be able to select from the legitimate ones listed above
job = consider.load("glue", "mrpc") # Specify the duty like "mrpc", "sst2", and so forth.
print("Job loaded.")
# Now you can run the duty on a mannequin (for instance: "distilbert-base-uncased")
# WARNING: This may take time for inference or fine-tuning.
# outcomes = job.compute(model_or_pipeline="distilbert-base-uncased")
# print("nEvaluation Outcomes (MRPC Job):")
# print(outcomes)
print("Skipping mannequin inference for brevity on this instance.")
print("Discuss with Hugging Face documentation for full EvaluationSuite utilization.")
besides Exception as e:
print(f"Couldn't load or run analysis suite: {e}")
Output:
Loading GLUE analysis suite (this may obtain datasets)...Job loaded.
Skipping mannequin inference for brevity on this instance.
Discuss with Hugging Face documentation for full EvaluationSuite utilization.
Clarification:
- EvaluationSuite.load masses a predefined set of analysis duties (right here, simply the MRPC job from the GLUE benchmark for demonstration).
- The suite.run(“model_name”) command would sometimes execute the mannequin on every dataset inside the suite and compute the related metrics.
- The output is often a listing of dictionaries, every containing the outcomes for one job within the suite. (Observe: Operating this usually requires particular atmosphere setups and substantial compute time).
Visualizing Analysis Outcomes
Visualizations assist examine a number of fashions throughout completely different metrics. Radar plots are efficient for this.
import consider
import matplotlib.pyplot as plt # Guarantee matplotlib is put in
from consider.visualization import radar_plot
# Pattern information for a number of fashions throughout a number of metrics
# Decrease latency is best, so we would invert it or think about it individually.
information = [
{"accuracy": 0.99, "precision": 0.80, "f1": 0.95, "latency_inv": 1/33.6},
{"accuracy": 0.98, "precision": 0.87, "f1": 0.91, "latency_inv": 1/11.2},
{"accuracy": 0.98, "precision": 0.78, "f1": 0.88, "latency_inv": 1/87.6},
{"accuracy": 0.88, "precision": 0.78, "f1": 0.81, "latency_inv": 1/101.6}
]
model_names = ["Model A", "Model B", "Model C", "Model D"]
# Generate the radar plot
# Increased values are typically higher on a radar plot
attempt:
# Generate radar plot (make sure you go an accurate format and that information is legitimate)
plot = radar_plot(information=information, model_names=model_names)
# Show the plot
plt.present() # Explicitly present the plot, is likely to be needed in some environments
# To save lots of the plot to a file (uncomment to make use of)
# plot.savefig("model_comparison_radar.png")
plt.shut() # Shut the plot window after exhibiting/saving
besides ImportError:
print("Visualization requires matplotlib. Run: pip set up matplotlib")
besides Exception as e:
print(f"Couldn't generate plot: {e}")
Output:
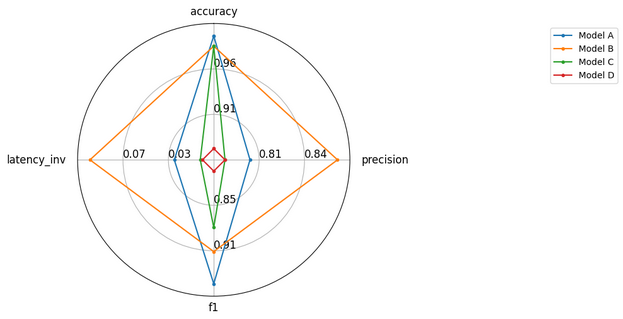
Clarification:
- We put together pattern outcomes for 4 fashions throughout accuracy, precision, F1, and inverted latency (so increased is best).
- radar_plot creates a plot the place every axis represents a metric, exhibiting how fashions examine visually.
Saving Analysis Outcomes
It can save you your analysis outcomes to a file, usually in JSON format, for record-keeping or later evaluation.
import consider
from pathlib import Path
# Carry out an analysis
accuracy_metric = consider.load("accuracy")
outcome = accuracy_metric.compute(references=[0, 1, 0, 1], predictions=[1, 0, 0, 1])
print(f"End result to save lots of: {outcome}")
# Outline hyperparameters or different metadata
hyperparams = {"model_name": "my_custom_model", "learning_rate": 0.001}
run_details = {"experiment_id": "run_42"}
# Mix outcomes and metadata
save_data = {**outcome, **hyperparams, **run_details}
# Outline save listing and filename
save_dir = Path("./evaluation_results")
save_dir.mkdir(exist_ok=True) # Create listing if it would not exist
# Use consider.save to retailer the outcomes
attempt:
saved_path = consider.save(save_directory=save_dir, **save_data)
print(f"Outcomes saved to: {saved_path}")
# You can too manually save as JSON
import json
manual_save_path = save_dir / "manual_results.json"
with open(manual_save_path, 'w') as f:
json.dump(save_data, f, indent=4)
print(f"Outcomes manually saved to: {manual_save_path}")
besides Exception as e:
# Catch potential git-related errors if run outdoors a repo
print(f"consider.save encountered a difficulty (presumably git associated): {e}")
print("Making an attempt guide JSON save as a substitute.")
import json
manual_save_path = save_dir / "manual_results_fallback.json"
with open(manual_save_path, 'w') as f:
json.dump(save_data, f, indent=4)
print(f"Outcomes manually saved to: {manual_save_path}")
Output:
End result to save lots of: {'accuracy': 0.5}consider.save encountered a difficulty (presumably git associated): save() lacking 1
required positional argument: 'path_or_file'Making an attempt guide JSON save as a substitute.
Outcomes manually saved to: evaluation_results/manual_results_fallback.json
Clarification:
- We mix the computed outcome dictionary with different metadata like hyperparams.
- consider.save makes an attempt to save lots of this information to a JSON file within the specified listing. It would attempt to add git commit data if run inside a repository, which may trigger errors in any other case (as seen within the authentic log).
- We embody a fallback to manually save the dictionary as a JSON file, which is commonly adequate.
Selecting the Proper Metric
Choosing the suitable metric is essential. Take into account these factors:
- Job Sort: Is it classification, translation, summarization, NER, QA? Use metrics normal for that job (Accuracy/F1 for classification, BLEU/ROUGE for era, Seqeval for NER, SQuAD for QA).
- Dataset: Some benchmarks (like GLUE, SQuAD) have particular related metrics. Leaderboards (e.g., on Papers With Code) usually present generally used metrics for particular datasets.
- Objective: What facet of efficiency issues most?
- Accuracy: General correctness (good for balanced courses).
- Precision/Recall/F1: Vital for imbalanced courses or when false positives/negatives have completely different prices.
- BLEU/ROUGE: Fluency and content material overlap in textual content era.
- Perplexity: How properly a language mannequin predicts a pattern (decrease is best, usually used for generative fashions).
- Metric Playing cards: Learn the Hugging Face metric playing cards (documentation) for detailed explanations, limitations, and acceptable use instances (e.g., BLEU card, SQuAD card).
Conclusion
The Hugging Face Consider library affords a flexible and user-friendly option to assess giant language fashions and datasets. It offers normal metrics, dataset measurements, and instruments just like the Evaluator and EvaluationSuite to streamline the method. By utilizing these instruments and selecting metrics acceptable on your job, you’ll be able to acquire clear insights into your mannequin’s strengths and weaknesses.
For extra particulars and superior utilization, seek the advice of the official assets:
Login to proceed studying and revel in expert-curated content material.