Boolean values are the first crucial of creating selections in Python as statements permit applications to make selections after which pursue particular actions. Actually, regardless of the place you might be in your code and whether or not you might be testing for the existence of a file, confirming consumer enter, or constructing your logic inside functions, attending to know booleans is essential. Right here inside this information, we are going to study concerning the booleans in python, from their definition to how we are able to use them, to sensible examples that may be utilized in actual situations in order that you’ll not want another information.
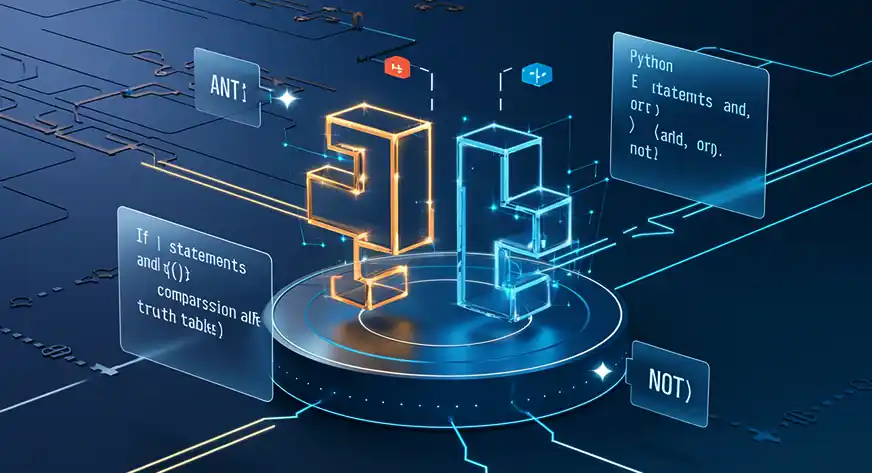
Studying Outcomes
- Perceive what booleans are in Python and their significance.
- Use boolean values successfully in conditional statements.
- Leverage Python’s built-in boolean operators.
- Discover truthy and falsy values and their influence on code logic.
- Implement booleans in real-world examples.
What Are Booleans in Python?
In Python, you utilize boolean as a basic information kind to characterize two values: True and False. These values are logical the place boolean kind the idea of resolution making within the programming of the software program. Coders took Boole’s work on Boolean algebra and named booleans after him as a result of he’s the founding father of algebra of logic.
Booleans are sometimes utilized in management constructions resembling if-structures, whereas constructions and for constructions to determine whether or not or not given strains of code ought to be executed based mostly upon some specific circumstance.
In Python, a boolean is a knowledge kind with two potential values: True
and False
. These values characterize logical states:
True
: Represents the logical fact.False
: Represents the logical falsehood.
Boolean Knowledge Kind in Python
Python defines True
and False
as constants of the bool
class:
print(kind(True)) # <class 'bool'>
print(kind(False)) # <class 'bool'>
Boolean Operators in Python
Boolean operators are particular key phrases in Python used to carry out logical operations on boolean values (True
or False
). These operators consider circumstances and return a boolean consequence (True
or False
). They’re important for decision-making, conditional logic, and controlling program move.
Python gives three major boolean operators:
and Operator
The and
operator returns True
provided that each operands are True
. If both operand is False
, the result’s False
. This operator evaluates circumstances sequentially from left to proper, and stops analysis (short-circuits) as quickly because it encounters a False
worth.
Syntax:
condition1 and condition2
Fact Desk:
Situation 1 | Situation 2 | Outcome |
---|---|---|
True | True | True |
True | False | False |
False | True | False |
False | False | False |
Instance:
x = 5
y = 10
# Each circumstances have to be True
consequence = (x > 0) and (y < 20) # True
print(consequence) # Output: True
Rationalization: Since each x > 0
and y < 20
are True
, the results of the and
operation is True
.
or Operator
The or
operator returns True
if no less than one operand is True
. If each operands are False
, the result’s False
. Just like the and
operator, or
additionally evaluates circumstances sequentially and stops analysis (short-circuits) as quickly because it encounters a True
worth.
Syntax:
condition1 or condition2
Fact Desk:
Situation 1 | Situation 2 | Outcome |
---|---|---|
True | True | True |
True | False | True |
False | True | True |
False | False | False |
Instance:
x = 5
y = 10
# At the very least one situation have to be True
consequence = (x > 0) or (y > 20) # True
print(consequence) # Output: True
Rationalization: Since x > 0
is True
, the or
operation short-circuits and returns True
with out evaluating y > 20
.
not Operator
The not
operator is a unary operator that negates the worth of its operand. It returns True
if the operand is False
and False
if the operand is True
.
Syntax:
not situation
Fact Desk:
Situation | Outcome |
---|---|
True | False |
False | True |
Instance:
x = False
# Negates the boolean worth
consequence = not x # True
print(consequence) # Output: True
Rationalization: Since x
is False
, the not
operator negates it and returns True
.
Operator Priority
Python evaluates boolean operators within the following order of priority:
not
(highest priority)and
or
(lowest priority)
Combining Boolean Operators
Boolean operators will be mixed to kind advanced logical expressions. Parentheses can be utilized to group circumstances and management the order of analysis.
Instance:
x = 5
y = 10
z = 15
# Complicated situation
consequence = (x > 0 and y < 20) or (z == 15 and never (y > 30))
print(consequence) # Output: True
Rationalization:
(x > 0 and y < 20)
evaluates toTrue
.(z == 15 and never (y > 30))
evaluates toTrue
.- The
or
operator combines these, returningTrue
since no less than one situation isTrue
.
Frequent Use Circumstances for Booleans in Python
Booleans are a cornerstone of programming logic, enabling Python applications to make selections, validate circumstances, and management the move of execution. Under are a few of the most typical use instances for booleans in Python:
Management Circulation and Choice-Making
Booleans are central to controlling program conduct utilizing conditional statements like if
, elif
, and else
.
Use Case: Making selections based mostly on circumstances.
Instance:
is_raining = True
if is_raining:
print("Take an umbrella.")
else:
print("No want for an umbrella.")
Output:
Take an umbrella.
Loop Management
Booleans are used to manage loop execution, enabling iterations to proceed or break based mostly on circumstances.
Use Case 1: Whereas loop management.
Instance:
keep_running = True
whereas keep_running:
user_input = enter("Proceed? (sure/no): ").decrease()
if user_input == "no":
keep_running = False
Use Case 2: Breaking out of a loop.
Instance:
for quantity in vary(10):
if quantity == 5:
print("Discovered 5, exiting loop.")
break
Validation and Enter Checking
Booleans are used to validate consumer inputs or guarantee sure circumstances are met earlier than continuing.
Use Case: Validating a password.
Instance:
password = "secure123"
is_valid = len(password) >= 8 and password.isalnum()
if is_valid:
print("Password is legitimate.")
else:
print("Password is invalid.")
Flags for State Administration
Booleans are sometimes used as flags to trace the state of a program or an operation.
Use Case: Monitoring the success of an operation.
Instance:
operation_successful = False
strive:
# Simulate some operation
consequence = 10 / 2
operation_successful = True
besides ZeroDivisionError:
operation_successful = False
if operation_successful:
print("Operation accomplished efficiently.")
else:
print("Operation failed.")
Brief-Circuiting Logic
Brief-circuit analysis optimizes boolean expressions by stopping the analysis as quickly as Python determines the result.
Use Case: Conditional execution of high-priced operations.
Instance:
is_valid = True
is_authenticated = False
if is_valid and is_authenticated:
print("Entry granted.")
else:
print("Entry denied.")
If is_valid
is False
, Python doesn’t consider is_authenticated
, saving computation time.
Conditional Assignments (Ternary Operator)
Booleans are used for concise conditional assignments.
Use Case: Setting a worth based mostly on a situation.
Instance:
age = 20
is_adult = True if age >= 18 else False
print(f"Is grownup: {is_adult}")
Error Dealing with and Security Checks
Booleans assist be sure that code doesn’t proceed in unsafe circumstances.
Use Case: Stopping division by zero.
Instance:
denominator = 0
is_safe_to_divide = denominator != 0
if is_safe_to_divide:
print(10 / denominator)
else:
print("Can not divide by zero.")
Conclusion
Boolean values are paramount to logical operations and management move within the language of Python. It implies that by understanding how booleans, you achieve the information on write smarter and more practical applications. From fundamental or if/else conditional checks to functions resembling authentication, booleans assist to behave as the basic constructing blocks in your decisions in code.
Key Takeaways
- Booleans in Python are True and False on the idea of which you make selections in your code.
- The usage of circumstances is essential in search processes with the assistance of Boolean operators and, or, not.
- Numbers totally different from zero in addition to strings totally different from the empty one are thought of as True whereas, quite the opposite, None, [], () are thought of as False.
- Comparability operators like
==
,!=
,>
,<
play a vital function in logical expressions. - Boolean values management the move of loops and conditional statements in Python.
Incessantly Requested Questions
A. Booleans are information varieties that characterize both True
or False
and are used for logical operations and decision-making.
A. The operators and
, or
, and not
management logical circumstances: and
requires each circumstances to be True
, or
requires no less than one situation to be True
, and not
negates a boolean worth.
A. Truthy values like non-zero numbers, non-empty strings, and lists are thought of True
, whereas falsy values like None
, 0
, empty strings, and lists are thought of False
.
A. Comparability operators like ==
, !=
, >
, <
consider whether or not two values are equal, not equal, better than, or lower than one another.
if
statements?
A. Booleans management the execution move in if
statements, permitting you to make selections based mostly on circumstances in your code.