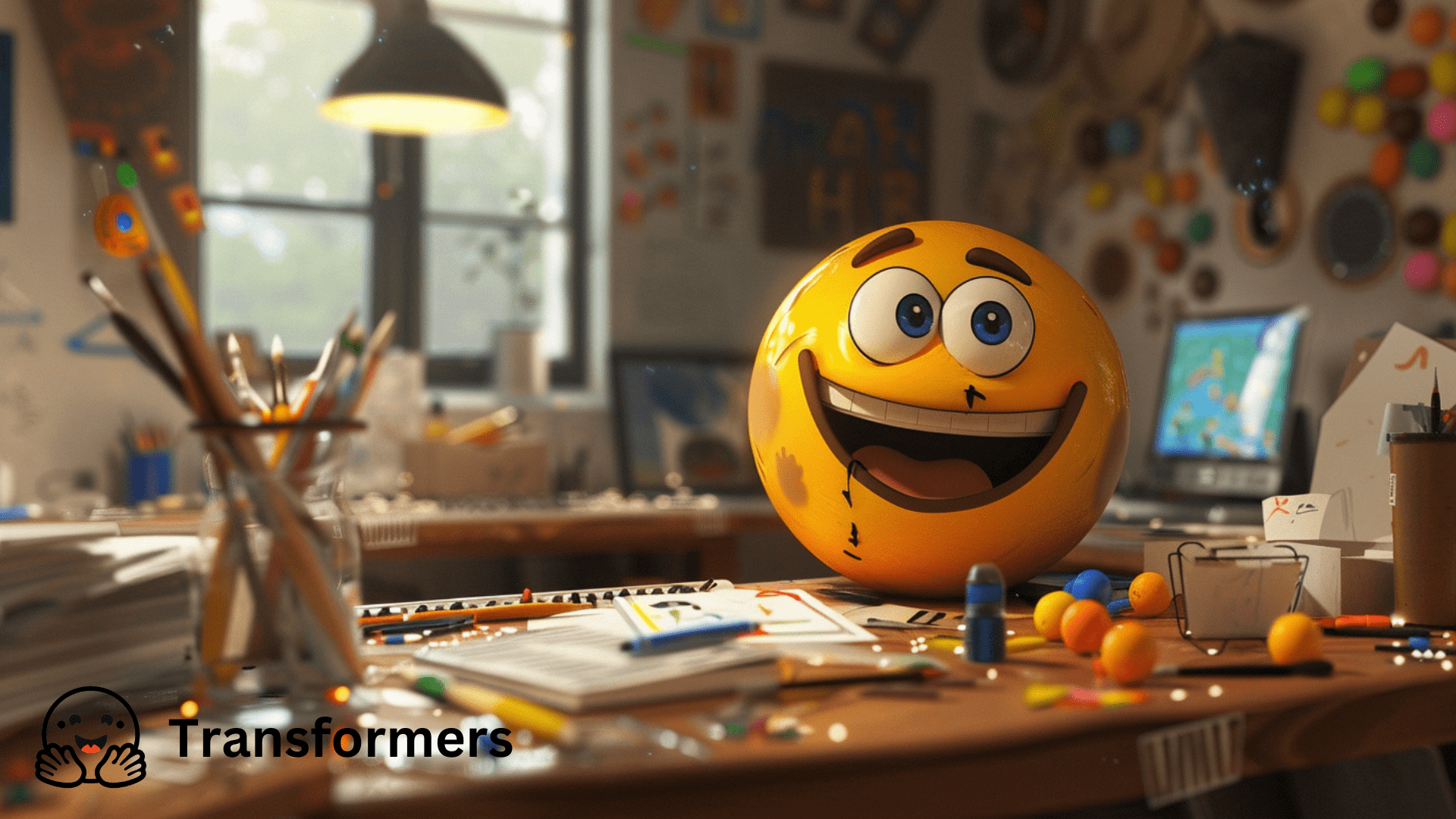
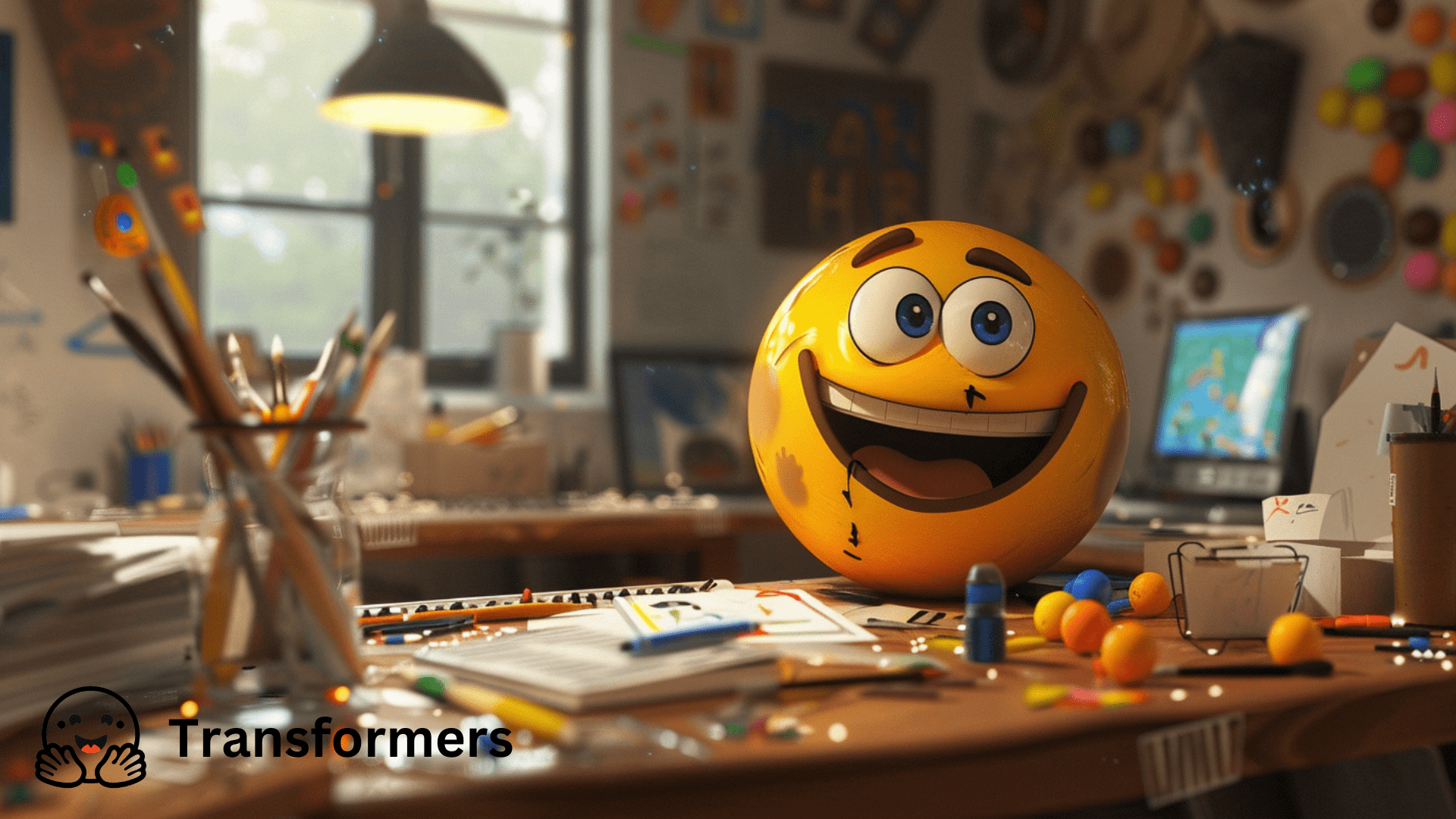
Picture by Editor | Midjourney
Hugging Face Transformers library offers instruments for simply loading and utilizing pre-trained Language Fashions (LMs) primarily based on the transformer structure. However, do you know this library additionally means that you can implement and practice your transformer mannequin from scratch? This tutorial illustrates how by means of a step-by-step sentiment classification instance.
Necessary be aware: Coaching a transformer mannequin from scratch is computationally costly, with a coaching loop sometimes requiring hours to say the least. To run the code on this tutorial, it’s extremely advisable to have entry to high-performance computing assets, be it on-premises or by way of a cloud supplier.
Step-by-Step Course of
Preliminary Setup and Dataset Loading
Relying on the kind of Python improvement surroundings you’re engaged on, you might want to put in Hugging Face’s transformers and datasets libraries, in addition to the speed up library to coach your transformer mannequin in a distributed computing setting.
!pip set up transformers datasets
!pip set up speed up -U
As soon as the required libraries are put in, let’s load the feelings dataset for sentiment classification of Twitter messages from Hugging Face hub:
from datasets import load_dataset
dataset = load_dataset('jeffnyman/feelings')
Utilizing the info for coaching a transformer-based LM requires tokenizing the textual content. The next code initializes a BERT tokenizer (BERT is a household of transformer fashions appropriate for textual content classification duties), defines a perform to tokenize textual content information with padding and truncation, and applies it to the dataset in batches.
from transformers import AutoTokenizer
def tokenize_function(examples):
return tokenizer(examples['text'], padding="max_length", truncation=True)
tokenizer = AutoTokenizer.from_pretrained('bert-base-uncased')
tokenized_datasets = dataset.map(tokenize_function, batched=True)
Earlier than shifting on to initialize the transformer mannequin, let’s confirm the distinctive labels within the dataset. Having a verified set of current class labels helps stop GPU-related errors throughout coaching by verifying label consistency and correctness. We’ll use this label set afterward.
unique_labels = set(tokenized_datasets['train']['label'])
print(f"Distinctive labels within the coaching set: {unique_labels}")
def check_labels(dataset):
for label in dataset['train']['label']:
if label not in unique_labels:
print(f"Discovered invalid label: {label}")
check_labels(tokenized_datasets)
Subsequent, we create and outline a mannequin configuration, after which instantiate the transformer mannequin with this configuration. That is the place we specify hyperparameters in regards to the transformer structure like embedding measurement, variety of consideration heads, and the beforehand calculated set of distinctive labels, key in constructing the ultimate output layer for sentiment classification.
from transformers import BertConfig
from transformers import BertForSequenceClassification
config = BertConfig(
vocab_size=tokenizer.vocab_size,
hidden_size=512,
num_hidden_layers=6,
num_attention_heads=8,
intermediate_size=2048,
max_position_embeddings=512,
num_labels=len(unique_labels)
)
mannequin = BertForSequenceClassification(config)
We’re virtually prepared to coach our transformer mannequin. It simply stays to instantiate two mandatory situations: TrainingArguments, with specs in regards to the coaching loop such because the variety of epochs, and Coach, which glues collectively the mannequin occasion, the coaching arguments, and the info utilized for coaching and validation.
from transformers import TrainingArguments, Coach
training_args = TrainingArguments(
output_dir="./outcomes",
evaluation_strategy="epoch",
learning_rate=2e-5,
per_device_train_batch_size=16,
per_device_eval_batch_size=16,
num_train_epochs=3,
weight_decay=0.01,
)
coach = Coach(
mannequin=mannequin,
args=training_args,
train_dataset=tokenized_datasets["train"],
eval_dataset=tokenized_datasets["test"],
)
Time to coach the mannequin, sit again, and loosen up. Keep in mind this instruction will take a big period of time to finish:
As soon as educated, your transformer mannequin ought to be prepared for passing in enter examples for sentiment prediction.
Troubleshooting
If issues seem or persist when executing the coaching loop or throughout its setup, you might want to examine the configuration of the GPU/CPU assets getting used. As an illustration, if utilizing a CUDA GPU, including these directions initially of your code might help stop errors within the coaching loop:
import os
os.environ["CUDA_LAUNCH_BLOCKING"] = "1"
These traces disable the GPU and make CUDA operations synchronous, offering extra speedy and correct error messages for debugging.
Alternatively, if you’re making an attempt this code in a Google Colab occasion, chances are high this error message exhibits up throughout execution, even if in case you have beforehand put in the speed up library:
ImportError: Utilizing the `Coach` with `PyTorch` requires `speed up>=0.21.0`: Please run `pip set up transformers[torch]` or `pip set up speed up -U`
To handle this situation, attempt restarting your session within the ‘Runtime’ menu: the speed up library sometimes requires resetting the run surroundings after being put in.
Abstract and Wrap-Up
This tutorial showcased the important thing steps to construct your transformer-based LM from scratch utilizing Hugging Face libraries. The principle steps and parts concerned may be summarized as:
- Loading the dataset and tokenizing the textual content information.
- Initializing your mannequin through the use of a mannequin configuration occasion for the kind of mannequin (language job) it’s meant for, e.g. BertConfig.
- Establishing a Coach and TrainingArguments situations and operating the coaching loop.
As a subsequent studying step, we encourage you to discover easy methods to make predictions and inferences along with your newly educated mannequin.
Iván Palomares Carrascosa is a frontrunner, author, speaker, and adviser in AI, machine studying, deep studying & LLMs. He trains and guides others in harnessing AI in the true world.