Introduction
The OpenAI o1 mannequin household considerably advances reasoning energy and financial efficiency, particularly in science, coding, and problem-solving. OpenAI’s objective is to create ever-more-advanced AI, and o1 fashions are an development over GPT-4 when it comes to efficiency and security. This text will clarify tips on how to construct video games with OpenAI o1, akin to Brick Breaker and Snake video games.
Key Options of OpenAI o1 Fashions
As a result of the o1 fashions are tailor-made explicitly for classy problem-solving in domains like coding, arithmetic, and scientific analysis, they’re significantly well-suited for actions requiring superior reasoning. Their precision, velocity, and flexibility are noticeably higher than these of GPT-4.
Improved Reasoning Capabilities
The o1 household is exclusive in that it might cause in varied conditions. In distinction to traditional language fashions that will have problem with difficult logical reasoning, the o1 fashions are wonderful at deriving complicated solutions, which makes them good for tackling issues in technical {and professional} domains. For instance, they’ll deal with points involving a number of ranges of data and grasp multi-step directions in an organized means.
Effectivity and Price-Effectiveness
The o1 fashions are distinguished by their excessive computational effectivity. Specifically, the o1-mini mannequin launch permits lowered bills with out sacrificing efficiency high quality. With o1-mini, builders can entry sturdy instruments for a tenth of the same old computing value for debugging and code assist actions. For cost-sensitive functions, akin to educational instruments or early-stage enterprises with restricted sources, o1-mini turns into invaluable.
Security Enhancements
The o1 fashions have higher security options, akin to elevated resistance to jailbreaks and extra exact obedience to consumer directions. This makes the fashions reliable in tutorial {and professional} contexts the place utilizing AI safely and ethically is a prime concern. These fashions are made to make sure that they operate inside the tight parameters of accountable AI deployment whereas additionally minimizing damaging outputs.
Additionally Learn: GPT-4o vs OpenAI o1: Is the New OpenAI Mannequin Definitely worth the Hype?
Learn how to Construct Video games utilizing OpenAI o1-preview?
On this part, I’ll be utilizing o1-preview to construct video games. It was an extremely enjoyable expertise, as I centered primarily on establishing the surroundings (which isn’t an issue) and easily copy-pasting code. Past that, o1-preview dealt with every part else, making the method seamless and environment friendly. Okay, let’s get into this part.
Additionally Learn: Learn how to Entry OpenAI o1?
Prompting o1-preview
Immediate – “I wish to construct a small/primary sport. It must be only for illustration.”
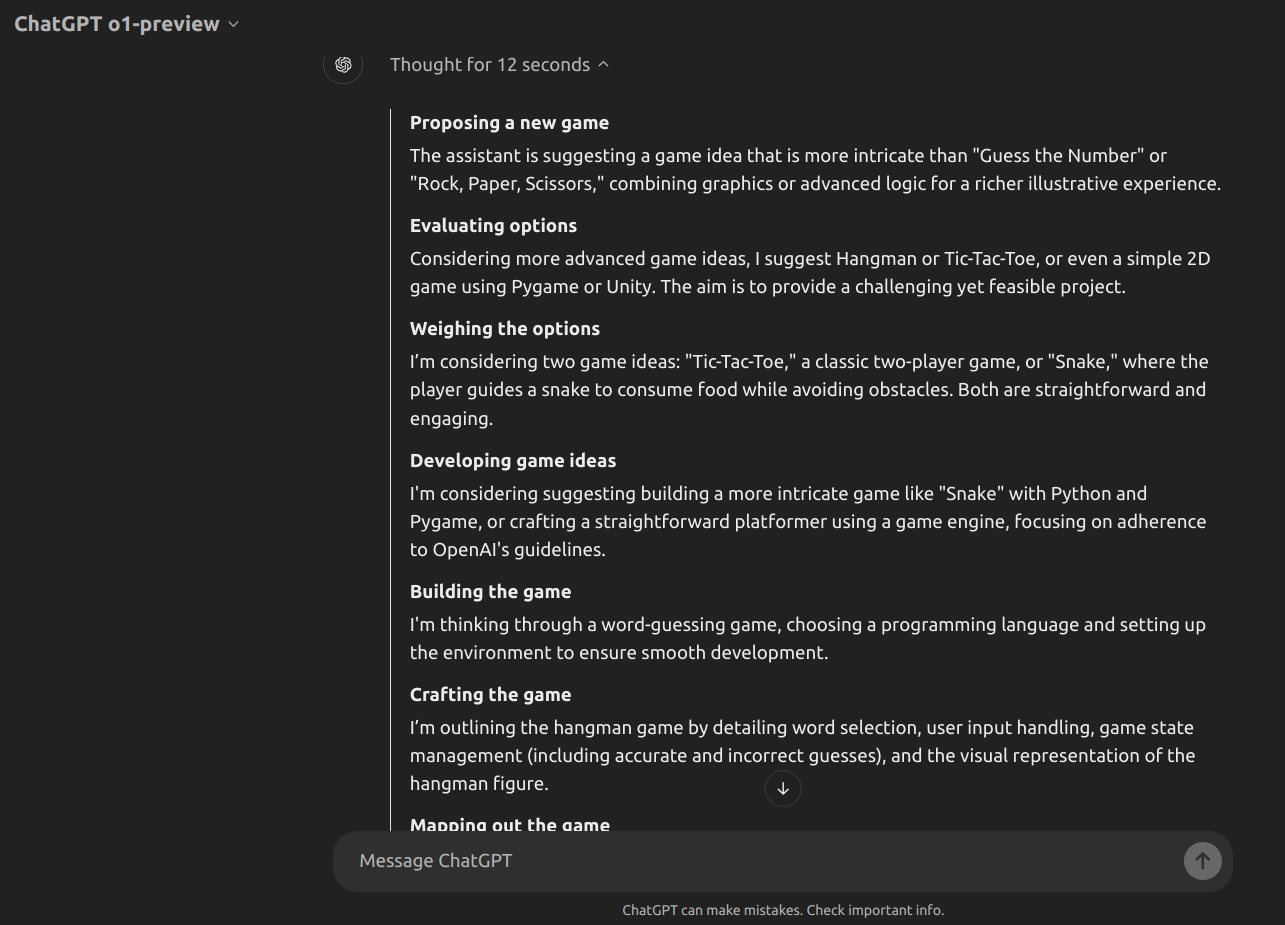
Within the above picture, we are able to see the o1-preview chain of ideas. This reveals how o1-preview approaches the issue or given immediate. We are able to additionally infer that it takes 12 seconds to reply. This additionally goes increased than 40 seconds, generally based mostly on the immediate and the quantity of pondering required. The picture beneath reveals the OpenAI o1’s ideas on constructing a brand new sport after constructing the primary one.
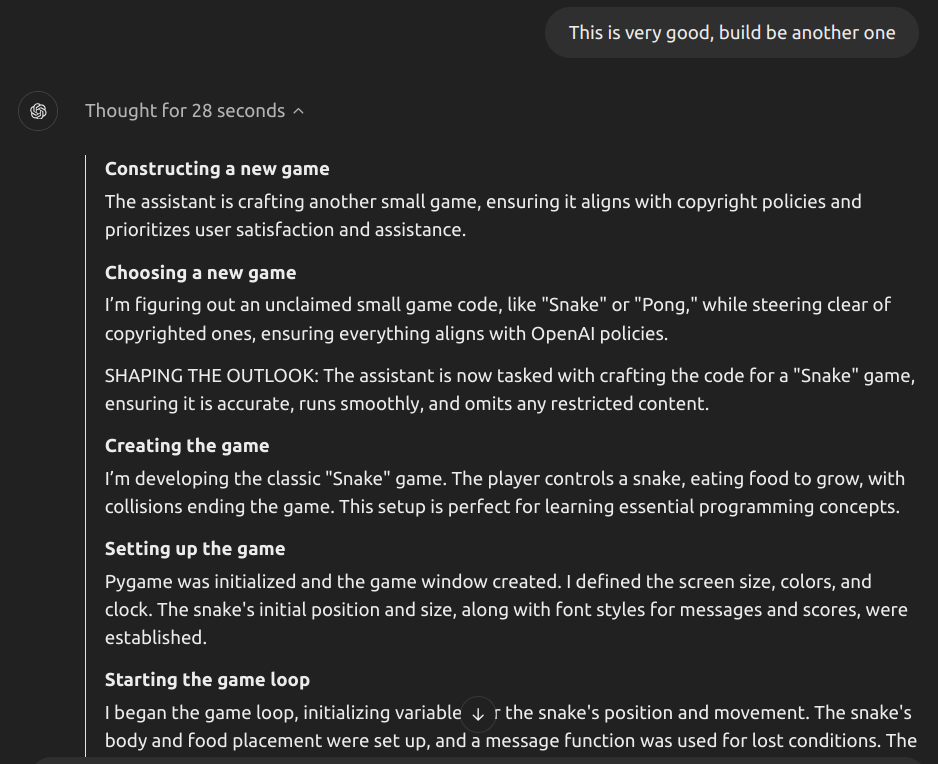
Recreation 1: Brick Breaker
Description:
- The participant controls a paddle on the backside of the display screen.
- A ball bounces across the display screen, breaking bricks when it hits them.
- The target is to interrupt all of the bricks with out letting the ball move the paddle.
- Incorporates primary physics, collision detection, and sport loop ideas.
Essential Parts:
- Recreation Window
- Paddle
- Ball
- Bricks
- Recreation Loop
- Occasion Dealing with
Putting in dependencies
pip set up pygame
Code for Brick Breaker
You possibly can copy and paste this code right into a file named brick_breaker.py
# brick_breaker.py
import pygame
import sys
# Initialize Pygame
pygame.init()
# Arrange the sport window
SCREEN_WIDTH = 800
SCREEN_HEIGHT = 600
display screen = pygame.show.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
pygame.show.set_caption('Brick Breaker')
# Outline Colours
WHITE = (255, 255, 255)
BLACK = (0, 0, 0)
RED = (200, 0, 0)
# Outline Paddle Class
class Paddle:
def __init__(self):
self.width = 100
self.top = 10
self.x = (SCREEN_WIDTH - self.width) / 2
self.y = SCREEN_HEIGHT - 30
self.velocity = 7
self.rect = pygame.Rect(self.x, self.y, self.width, self.top)
def transfer(self, dx):
self.rect.x += dx * self.velocity
# Forestall paddle from transferring off-screen
if self.rect.left < 0:
self.rect.left = 0
if self.rect.proper > SCREEN_WIDTH:
self.rect.proper = SCREEN_WIDTH
def draw(self, floor):
pygame.draw.rect(floor, WHITE, self.rect)
# Outline Ball Class
class Ball:
def __init__(self):
self.radius = 8
self.x = SCREEN_WIDTH / 2
self.y = SCREEN_HEIGHT / 2
self.speed_x = 4
self.speed_y = -4
self.rect = pygame.Rect(self.x - self.radius, self.y - self.radius,
self.radius * 2, self.radius * 2)
def transfer(self):
self.rect.x += self.speed_x
self.rect.y += self.speed_y
# Bounce off partitions
if self.rect.left <= 0 or self.rect.proper >= SCREEN_WIDTH:
self.speed_x *= -1
if self.rect.prime <= 0:
self.speed_y *= -1
def draw(self, floor):
pygame.draw.circle(floor, WHITE,
(self.rect.x + self.radius, self.rect.y + self.radius),
self.radius)
# Outline Brick Class
class Brick:
def __init__(self, x, y):
self.width = 60
self.top = 20
self.rect = pygame.Rect(x, y, self.width, self.top)
self.shade = RED # Pink shade
def draw(self, floor):
pygame.draw.rect(floor, self.shade, self.rect)
# Perform to Create Bricks
def create_bricks(rows, cols):
bricks = []
padding = 5
offset_x = 35
offset_y = 50
for row in vary(rows):
for col in vary(cols):
x = offset_x + col * (60 + padding)
y = offset_y + row * (20 + padding)
bricks.append(Brick(x, y))
return bricks
# Essential Recreation Loop
def essential():
clock = pygame.time.Clock()
paddle = Paddle()
ball = Ball()
bricks = create_bricks(5, 11) # 5 rows, 11 columns
operating = True
whereas operating:
clock.tick(60) # Restrict to 60 frames per second
display screen.fill(BLACK) # Clear display screen with black shade
# Occasion Dealing with
for occasion in pygame.occasion.get():
if occasion.sort == pygame.QUIT:
operating = False
# Paddle Motion
keys = pygame.key.get_pressed()
if keys[pygame.K_LEFT]:
paddle.transfer(-1)
if keys[pygame.K_RIGHT]:
paddle.transfer(1)
# Transfer Ball
ball.transfer()
# Collision Detection
if ball.rect.colliderect(paddle.rect):
ball.speed_y *= -1 # Bounce off paddle
# Verify for collision with bricks
for brick in bricks[:]:
if ball.rect.colliderect(brick.rect):
ball.speed_y *= -1
bricks.take away(brick)
break # Forestall a number of collisions in a single body
# Verify if ball is out of bounds
if ball.rect.backside >= SCREEN_HEIGHT:
print("Recreation Over")
operating = False
# Draw Recreation Objects
paddle.draw(display screen)
ball.draw(display screen)
for brick in bricks:
brick.draw(display screen)
# Replace Show
pygame.show.flip()
pygame.give up()
sys.exit()
if __name__ == "__main__":
essential()
Run the Recreation
python brick_breaker.py
Add brick_breaker video
Study Extra: Machine Studying and AI in Recreation Growth in 2024
Recreation 2: Snake Recreation
Description:
- You management a snake that strikes across the display screen.
- The snake grows longer every time it eats meals.
- The sport ends if the snake collides with the partitions or itself.
- The target is to eat as a lot meals as doable to realize a excessive rating.
Recreation Controls
Recreation Goal
- Eat Meals
- Develop
- Keep away from Collisions
- Rating
Code for Snake Recreation
# snake_game.py
import pygame
import sys
import random
# Initialize Pygame
pygame.init()
# Arrange the sport window
SCREEN_WIDTH = 600
SCREEN_HEIGHT = 400
display screen = pygame.show.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
pygame.show.set_caption('Snake Recreation')
# Outline Colours
WHITE = (255, 255, 255)
BLACK = (0, 0, 0)
GREEN = (0, 255, 0)
RED = (213, 50, 80)
# Arrange the clock for an honest framerate
clock = pygame.time.Clock()
# Outline the snake's preliminary place and measurement
snake_block = 10
snake_speed = 15
# Fonts for displaying rating and messages
font_style = pygame.font.SysFont(None, 30)
score_font = pygame.font.SysFont(None, 25)
def display_score(rating):
worth = score_font.render("Your Rating: " + str(rating), True, WHITE)
display screen.blit(worth, [0, 0])
def draw_snake(snake_block, snake_list):
for x in snake_list:
pygame.draw.rect(display screen, GREEN, [x[0], x[1], snake_block, snake_block])
def message(msg, shade):
mesg = font_style.render(msg, True, shade)
display screen.blit(mesg, [SCREEN_WIDTH / 6, SCREEN_HEIGHT / 3])
def game_loop():
game_over = False
game_close = False
# Beginning place of the snake
x1 = SCREEN_WIDTH / 2
y1 = SCREEN_HEIGHT / 2
# Change in place
x1_change = 0
y1_change = 0
# Snake physique record
snake_list = []
length_of_snake = 1
# Place meals randomly
foodx = spherical(random.randrange(0, SCREEN_WIDTH - snake_block) / 10.0) * 10.0
foody = spherical(random.randrange(0, SCREEN_HEIGHT - snake_block) / 10.0) * 10.0
whereas not game_over:
whereas game_close:
display screen.fill(BLACK)
message("You Misplaced! Press C-Play Once more or Q-Give up", RED)
pygame.show.replace()
# Occasion dealing with for sport over display screen
for occasion in pygame.occasion.get():
if occasion.sort == pygame.KEYDOWN:
if occasion.key == pygame.K_q:
game_over = True
game_close = False
if occasion.key == pygame.K_c:
game_loop()
if occasion.sort == pygame.QUIT:
game_over = True
game_close = False
# Occasion dealing with for sport play
for occasion in pygame.occasion.get():
if occasion.sort == pygame.QUIT:
game_over = True
if occasion.sort == pygame.KEYDOWN:
if occasion.key == pygame.K_LEFT and x1_change != snake_block:
x1_change = -snake_block
y1_change = 0
elif occasion.key == pygame.K_RIGHT and x1_change != -snake_block:
x1_change = snake_block
y1_change = 0
elif occasion.key == pygame.K_UP and y1_change != snake_block:
y1_change = -snake_block
x1_change = 0
elif occasion.key == pygame.K_DOWN and y1_change != -snake_block:
y1_change = snake_block
x1_change = 0
# Verify for boundaries
if x1 >= SCREEN_WIDTH or x1 < 0 or y1 >= SCREEN_HEIGHT or y1 < 0:
game_close = True
# Replace snake place
x1 += x1_change
y1 += y1_change
display screen.fill(BLACK)
pygame.draw.rect(display screen, RED, [foodx, foody, snake_block, snake_block])
snake_head = [x1, y1]
snake_list.append(snake_head)
if len(snake_list) > length_of_snake:
del snake_list[0]
# Verify if snake collides with itself
for x in snake_list[:-1]:
if x == snake_head:
game_close = True
draw_snake(snake_block, snake_list)
display_score(length_of_snake - 1)
pygame.show.replace()
# Verify if snake has eaten the meals
if x1 == foodx and y1 == foody:
foodx = spherical(random.randrange(0, SCREEN_WIDTH - snake_block) / 10.0) * 10.0
foody = spherical(random.randrange(0, SCREEN_HEIGHT - snake_block) / 10.0) * 10.0
length_of_snake += 1
clock.tick(snake_speed)
pygame.give up()
sys.exit()
if __name__ == "__main__":
game_loop()
Run the Recreation
python snake_game.pyAdd Snake sport video
Additionally Learn: 3 Arms-On Experiments with OpenAI’s o1 You Must See
Recreation 3: Ping Pong Recreation
Description:
- Two gamers management paddles on reverse sides of the display screen.
- A ball bounces between the paddles.
- Every participant tries to forestall the ball from getting previous their paddle.
- The sport ends when one participant reaches a set rating.
Recreation Controls
Recreation Goal
- Forestall the ball from passing your paddle.
- Rating some extent every time the ball will get previous the opponent’s paddle.
- The sport continues indefinitely; you possibly can add a scoring restrict to finish the sport.
Code for Ping Pong Recreation
# pong_game.py
import pygame
import sys
# Initialize Pygame
pygame.init()
# Arrange the sport window
SCREEN_WIDTH = 800
SCREEN_HEIGHT = 600
display screen = pygame.show.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
pygame.show.set_caption('Pong')
# Outline Colours
WHITE = (255, 255, 255)
BLACK = (0, 0, 0)
# Paddle and Ball Settings
PADDLE_WIDTH = 10
PADDLE_HEIGHT = 100
BALL_SIZE = 10
PADDLE_SPEED = 6
BALL_SPEED_X = 4
BALL_SPEED_Y = 4
# Fonts for displaying rating
score_font = pygame.font.SysFont(None, 35)
# Outline Paddle Class
class Paddle:
def __init__(self, x, y):
self.rect = pygame.Rect(x, y, PADDLE_WIDTH, PADDLE_HEIGHT)
self.velocity = PADDLE_SPEED
def transfer(self, up, down):
keys = pygame.key.get_pressed()
if keys[up] and self.rect.prime > 0:
self.rect.y -= self.velocity
if keys[down] and self.rect.backside < SCREEN_HEIGHT:
self.rect.y += self.velocity
def draw(self, floor):
pygame.draw.rect(floor, WHITE, self.rect)
# Outline Ball Class
class Ball:
def __init__(self):
self.rect = pygame.Rect(SCREEN_WIDTH // 2, SCREEN_HEIGHT // 2, BALL_SIZE, BALL_SIZE)
self.speed_x = BALL_SPEED_X
self.speed_y = BALL_SPEED_Y
def transfer(self):
self.rect.x += self.speed_x
self.rect.y += self.speed_y
# Bounce off prime and backside partitions
if self.rect.prime <= 0 or self.rect.backside >= SCREEN_HEIGHT:
self.speed_y *= -1
def draw(self, floor):
pygame.draw.ellipse(floor, WHITE, self.rect)
# Perform to show the rating
def display_score(score1, score2):
score_text = score_font.render(f"Participant 1: {score1} Participant 2: {score2}", True, WHITE)
display screen.blit(score_text, (SCREEN_WIDTH // 2 - score_text.get_width() // 2, 20))
# Essential Recreation Loop
def essential():
clock = pygame.time.Clock()
# Create Paddles and Ball
paddle1 = Paddle(30, SCREEN_HEIGHT // 2 - PADDLE_HEIGHT // 2)
paddle2 = Paddle(SCREEN_WIDTH - 30 - PADDLE_WIDTH, SCREEN_HEIGHT // 2 - PADDLE_HEIGHT // 2)
ball = Ball()
# Initialize scores
score1 = 0
score2 = 0
operating = True
whereas operating:
clock.tick(60) # Restrict to 60 frames per second
display screen.fill(BLACK) # Clear display screen with black shade
# Occasion Dealing with
for occasion in pygame.occasion.get():
if occasion.sort == pygame.QUIT:
operating = False
# Transfer Paddles
paddle1.transfer(pygame.K_w, pygame.K_s)
paddle2.transfer(pygame.K_UP, pygame.K_DOWN)
# Transfer Ball
ball.transfer()
# Collision Detection with Paddles
if ball.rect.colliderect(paddle1.rect) or ball.rect.colliderect(paddle2.rect):
ball.speed_x *= -1 # Bounce off paddles
# Verify for Scoring
if ball.rect.left <= 0:
score2 += 1
ball.rect.middle = (SCREEN_WIDTH // 2, SCREEN_HEIGHT // 2) # Reset ball
ball.speed_x *= -1
if ball.rect.proper >= SCREEN_WIDTH:
score1 += 1
ball.rect.middle = (SCREEN_WIDTH // 2, SCREEN_HEIGHT // 2) # Reset ball
ball.speed_x *= -1
# Draw Recreation Objects
paddle1.draw(display screen)
paddle2.draw(display screen)
ball.draw(display screen)
display_score(score1, score2)
# Replace Show
pygame.show.flip()
pygame.give up()
sys.exit()
if __name__ == "__main__":
essential()
Run the Recreation
python pong_game.pyAdd ping pong sport video
Recreation 4: Tic Tac Toe
Description:
- A 3×3 grid the place two gamers take turns inserting their marks (X or O).
- The target is to be the primary participant to get three marks in a row (horizontally, vertically, or diagonally).
- The sport ends in a win or a draw if all cells are crammed with no winner.
Recreation Controls
Recreation Goal
- Be the primary participant to get three of your marks (X or O) in a row, column, or diagonal.
- The sport ends in a win or a draw if all cells are crammed with no winner.
Code for Tic Tac Toe
# tic_tac_toe.py
import pygame
import sys
# Initialize Pygame
pygame.init()
# Arrange the sport window
SCREEN_WIDTH = 600
SCREEN_HEIGHT = 600
display screen = pygame.show.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
pygame.show.set_caption('Tic-Tac-Toe')
# Outline Colours
WHITE = (255, 255, 255)
BLACK = (0, 0, 0)
RED = (255, 0, 0)
BLUE = (0, 0, 255)
# Outline Board Settings
BOARD_ROWS = 3
BOARD_COLS = 3
SQUARE_SIZE = SCREEN_WIDTH // BOARD_COLS
LINE_WIDTH = 15
# Initialize the board
board = [[None for _ in range(BOARD_COLS)] for _ in vary(BOARD_ROWS)]
current_player="X" # Begin with participant X
def draw_board():
display screen.fill(WHITE)
# Draw grid traces
for row in vary(1, BOARD_ROWS):
pygame.draw.line(display screen, BLACK, (0, row * SQUARE_SIZE), (SCREEN_WIDTH, row * SQUARE_SIZE), LINE_WIDTH)
for col in vary(1, BOARD_COLS):
pygame.draw.line(display screen, BLACK, (col * SQUARE_SIZE, 0), (col * SQUARE_SIZE, SCREEN_HEIGHT), LINE_WIDTH)
def draw_markers():
for row in vary(BOARD_ROWS):
for col in vary(BOARD_COLS):
marker = board[row][col]
if marker == 'X':
pygame.draw.line(display screen, RED,
(col * SQUARE_SIZE + 20, row * SQUARE_SIZE + 20),
((col + 1) * SQUARE_SIZE - 20, (row + 1) * SQUARE_SIZE - 20), LINE_WIDTH)
pygame.draw.line(display screen, RED,
(col * SQUARE_SIZE + 20, (row + 1) * SQUARE_SIZE - 20),
((col + 1) * SQUARE_SIZE - 20, row * SQUARE_SIZE + 20), LINE_WIDTH)
elif marker == 'O':
pygame.draw.circle(display screen, BLUE,
(col * SQUARE_SIZE + SQUARE_SIZE // 2, row * SQUARE_SIZE + SQUARE_SIZE // 2),
SQUARE_SIZE // 2 - 20, LINE_WIDTH)
def check_winner():
# Verify rows and columns for a win
for row in vary(BOARD_ROWS):
if board[row][0] == board[row][1] == board[row][2] and board[row][0] shouldn't be None:
return board[row][0]
for col in vary(BOARD_COLS):
if board[0][col] == board[1][col] == board[2][col] and board[0][col] shouldn't be None:
return board[0][col]
# Verify diagonals for a win
if board[0][0] == board[1][1] == board[2][2] and board[0][0] shouldn't be None:
return board[0][0]
if board[0][2] == board[1][1] == board[2][0] and board[0][2] shouldn't be None:
return board[0][2]
# Verify for a draw
if all(all(cell shouldn't be None for cell in row) for row in board):
return 'Draw'
return None
def game_over_message(winner):
font = pygame.font.SysFont(None, 55)
if winner == 'Draw':
textual content = font.render('Draw! Press R to Restart', True, BLACK)
else:
textual content = font.render(f'{winner} Wins! Press R to Restart', True, BLACK)
display screen.blit(textual content, (SCREEN_WIDTH // 2 - textual content.get_width() // 2, SCREEN_HEIGHT // 2 - textual content.get_height() // 2))
def reset_game():
international board, current_player
board = [[None for _ in range(BOARD_COLS)] for _ in vary(BOARD_ROWS)]
current_player="X"
def essential():
international current_player
operating = True
winner = None
whereas operating:
for occasion in pygame.occasion.get():
if occasion.sort == pygame.QUIT:
operating = False
if occasion.sort == pygame.MOUSEBUTTONDOWN and winner is None:
mouse_x, mouse_y = occasion.pos
clicked_row = mouse_y // SQUARE_SIZE
clicked_col = mouse_x // SQUARE_SIZE
if board[clicked_row][clicked_col] is None:
board[clicked_row][clicked_col] = current_player
current_player="O" if current_player == 'X' else 'X'
if occasion.sort == pygame.KEYDOWN:
if occasion.key == pygame.K_r:
reset_game()
winner = None
draw_board()
draw_markers()
winner = check_winner()
if winner:
game_over_message(winner)
pygame.show.flip()
pygame.give up()
sys.exit()
if __name__ == "__main__":
essential()
Run the Recreation
python tic_tac_toe.pyAdd tic tac toe video
Study Extra: Prime 10 AI Instruments Reworking Recreation Growth
Recreation 5: Recreation 2048
Description:
- The sport consists of a 4×4 grid of tiles.
- Gamers mix tiles with the identical quantity to create bigger numbers, aiming to achieve the 2048 tile.
- Gamers can transfer all tiles up, down, left, or proper.
- When two tiles with the identical quantity contact, they merge into one.
Why “2048”?
- Logical Pondering
- Interactive and Partaking
- Expandable
Recreation Controls
Recreation Goal
- Mix tiles with the identical quantity to create higher-numbered tiles.
- Goal to achieve the
Code for Recreation 2048
# game_2048.py
import pygame
import sys
import random
# Initialize Pygame
pygame.init()
# Arrange the sport window
SIZE = WIDTH, HEIGHT = 400, 400
display screen = pygame.show.set_mode(SIZE)
pygame.show.set_caption('2048')
# Outline Colours
BACKGROUND_COLOR = (187, 173, 160)
EMPTY_TILE_COLOR = (205, 193, 180)
TILE_COLORS = {
2: (238, 228, 218),
4: (237, 224, 200),
8: (242, 177, 121),
16: (245, 149, 99),
32: (246, 124, 95),
64: (246, 94, 59),
128: (237, 207, 114),
256: (237, 204, 97),
512: (237, 200, 80),
1024: (237, 197, 63),
2048: (237, 194, 46),
}
FONT_COLOR = (119, 110, 101)
FONT = pygame.font.SysFont('Arial', 24, daring=True)
# Initialize sport variables
GRID_SIZE = 4
TILE_SIZE = WIDTH // GRID_SIZE
GRID = [[0] * GRID_SIZE for _ in vary(GRID_SIZE)]
def add_new_tile():
empty_tiles = [(i, j) for i in range(GRID_SIZE) for j in range(GRID_SIZE) if GRID[i][j] == 0]
if empty_tiles:
i, j = random.selection(empty_tiles)
GRID[i][j] = random.selection([2, 4])
def draw_grid():
display screen.fill(BACKGROUND_COLOR)
for i in vary(GRID_SIZE):
for j in vary(GRID_SIZE):
worth = GRID[i][j]
rect = pygame.Rect(j * TILE_SIZE, i * TILE_SIZE, TILE_SIZE, TILE_SIZE)
pygame.draw.rect(display screen, TILE_COLORS.get(worth, EMPTY_TILE_COLOR), rect)
if worth != 0:
text_surface = FONT.render(str(worth), True, FONT_COLOR)
text_rect = text_surface.get_rect(middle=rect.middle)
display screen.blit(text_surface, text_rect)
def move_left():
moved = False
for i in vary(GRID_SIZE):
tiles = [value for value in GRID[i] if worth != 0]
new_row = []
skip = False
for j in vary(len(tiles)):
if skip:
skip = False
proceed
if j + 1 < len(tiles) and tiles[j] == tiles[j + 1]:
new_row.append(tiles[j] * 2)
skip = True
moved = True
else:
new_row.append(tiles[j])
new_row += [0] * (GRID_SIZE - len(new_row))
if GRID[i] != new_row:
GRID[i] = new_row
moved = True
return moved
def move_right():
moved = False
for i in vary(GRID_SIZE):
tiles = [value for value in GRID[i] if worth != 0]
new_row = []
skip = False
for j in vary(len(tiles) - 1, -1, -1):
if skip:
skip = False
proceed
if j - 1 >= 0 and tiles[j] == tiles[j - 1]:
new_row.insert(0, tiles[j] * 2)
skip = True
moved = True
else:
new_row.insert(0, tiles[j])
new_row = [0] * (GRID_SIZE - len(new_row)) + new_row
if GRID[i] != new_row:
GRID[i] = new_row
moved = True
return moved
def move_up():
moved = False
for j in vary(GRID_SIZE):
tiles = [GRID[i][j] for i in vary(GRID_SIZE) if GRID[i][j] != 0]
new_column = []
skip = False
for i in vary(len(tiles)):
if skip:
skip = False
proceed
if i + 1 < len(tiles) and tiles[i] == tiles[i + 1]:
new_column.append(tiles[i] * 2)
skip = True
moved = True
else:
new_column.append(tiles[i])
new_column += [0] * (GRID_SIZE - len(new_column))
for i in vary(GRID_SIZE):
if GRID[i][j] != new_column[i]:
GRID[i][j] = new_column[i]
moved = True
return moved
def move_down():
moved = False
for j in vary(GRID_SIZE):
tiles = [GRID[i][j] for i in vary(GRID_SIZE) if GRID[i][j] != 0]
new_column = []
skip = False
for i in vary(len(tiles) - 1, -1, -1):
if skip:
skip = False
proceed
if i - 1 >= 0 and tiles[i] == tiles[i - 1]:
new_column.insert(0, tiles[i] * 2)
skip = True
moved = True
else:
new_column.insert(0, tiles[i])
new_column = [0] * (GRID_SIZE - len(new_column)) + new_column
for i in vary(GRID_SIZE):
if GRID[i][j] != new_column[i]:
GRID[i][j] = new_column[i]
moved = True
return moved
def is_game_over():
for i in vary(GRID_SIZE):
for j in vary(GRID_SIZE):
if GRID[i][j] == 0:
return False
if j + 1 < GRID_SIZE and GRID[i][j] == GRID[i][j + 1]:
return False
if i + 1 < GRID_SIZE and GRID[i][j] == GRID[i + 1][j]:
return False
return True
def essential():
add_new_tile()
add_new_tile()
operating = True
whereas operating:
draw_grid()
pygame.show.flip()
if is_game_over():
print("Recreation Over!")
operating = False
proceed
for occasion in pygame.occasion.get():
if occasion.sort == pygame.QUIT:
operating = False
elif occasion.sort == pygame.KEYDOWN:
moved = False
if occasion.key == pygame.K_LEFT:
moved = move_left()
elif occasion.key == pygame.K_RIGHT:
moved = move_right()
elif occasion.key == pygame.K_UP:
moved = move_up()
elif occasion.key == pygame.K_DOWN:
moved = move_down()
if moved:
add_new_tile()
pygame.give up()
sys.exit()
if __name__ == "__main__":
essential()
Run the Recreation
python game_2048.pyAdd the video for Recreation 2048
Additionally Learn: Learn how to Entry the OpenAI o1 API?
Conclusion
With its particular design to handle difficult reasoning issues in science, arithmetic, and coding, the OpenAI o1 mannequin household demonstrates a powerful leap ahead in AI know-how. It’s helpful for tutorial, analysis, {and professional} settings due to its cost-effectiveness, elevated security options, and expanded reasoning capabilities. I investigated the OpenAI o1 mannequin’s potential for constructing video games. I may see its effectiveness in producing interactive video games akin to Brick Breaker, Snake Recreation, Ping Pong, Tic Tac Toe, and 2048. Fashions like o1 will turn out to be more and more necessary as AI develops, facilitating artistic and efficient problem-solving in varied industries.
Keep tuned to Analytics Vidhya weblog to know extra concerning the makes use of of o1!
Incessantly Requested Questions
A. The o1 fashions supply enhanced reasoning capabilities and improved security options, making them very best for coding, arithmetic, and scientific analysis.
A. o1-mini is optimized for prime computational effectivity, permitting builders to run duties like debugging at a fraction of the fee whereas sustaining sturdy efficiency.
A. o1 fashions characteristic stronger resistance to jailbreak makes an attempt and extra correct adherence to consumer directions, making certain protected and moral AI use in skilled settings.
A. The o1 fashions excel at complicated, multi-step problem-solving duties, significantly in coding, logic, scientific evaluation, and technical problem-solving.
A. Sure, o1-preview was used to construct a number of video games, together with Brick Breaker, Snake Recreation, Ping Pong, Tic Tac Toe, and 2048, showcasing its versatility in coding tasks.