QR codes may be seen all over the place . Particularly on eating places , petrol pumps , retailers primarily for making funds. There are a number of different purposes additionally. The primary purpose is it’s a simple option to retailer info within the type of a small, easy-to-scan picture . On this information, I’ll assist you to mastering QR codes and the way QR codes work and stroll you thru creating them utilizing python.
Studying Outcomes
- Perceive the basics of QR codes and their performance.
- Mastering QR Codes allows you to effectively create, customise, and apply QR codes in varied real-world eventualities utilizing Python.
- Discover ways to generate and customise QR codes utilizing Python.
- Discover the historical past and benefits of QR codes in varied purposes.
- Acquire hands-on expertise by making a Wi-Fi QR code for straightforward community entry.
- Uncover real-world makes use of for QR codes, together with in public areas and companies.
This text was printed as part of the Knowledge Science Blogathon.
What are QR Codes?
Fast Response(QR) codes are literally two-dimensional matrix barcode that may retailer varied forms of information, equivalent to URLs, textual content, contact info, or Wi-Fi credentials .Fast Response indicating that The code contents ought to be decoded in a short time at excessive velocity .Normally the code consists of black modules organized in a sq. sample on a white background. In contrast to conventional barcodes, which solely maintain info in a single route (horizontally). QR codes can retailer information each horizontally and vertically. Permitting for a lot higher storage capability.
Historical past of QR Codes
It was invented by Denso Wave in 1994 for Toyota Group .The aim was to trace automotive components throughout manufacturing. Their capacity was to retailer a big quantity of information and may be scanned shortly made them common in varied industries over time.
Why Use QR Codes?
QR codes have a number of benefits:
- Simple to Use : It may be scanned with a smartphone digicam or a devoted QR scanner.
- Versatile : can retailer various kinds of information, together with URLs, textual content, and extra.
- Quick Entry : shortly offers info with only a scan.
- Contactless : helpful for contactless transactions and sharing.
QR Codes with Python
On this part, I’ll clarify the best way to generate QR codes utilizing python. We’ll cowl totally different examples to show the method.Ranging from making a easy QR code to customizing it additional. We’ll use the qrcode library, which offers an easy option to create QR codes. To start, be sure you have the library put in:
pip set up qrcode[pil]
Example1 : Producing a Easy QR Code
On this first instance, create a primary QR code with default settings. Right here’s the code:
import qrcode
from PIL import Picture
#Knowledge to be encoded
information = "Welcome to QR Code Tutorial"
#Create a QR code object
qr = qrcode.QRCode(
model=1, # Measurement of the QR code
box_size=10, # Measurement of every field within the QR code grid
border=4 # Border thickness
)
qr.add_data(information)
qr.make(match=True)
#Generate QR code picture
img = qr.make_image(fill="black", back_color="white")
img.present()
img.save('simple_qr_code.png')
Right here’s a clarification for the parameters used within the code:
- model: It is going to controls the code’s complexity and measurement.
- box_size: measurement of every field within the QR code.
- border: the thickness of the outer white house.
The above code will generate a easy QR code encoding the textual content “Welcome to QR Code Tutorial.” The next would be the consequence ,merely scan the code to view the output.
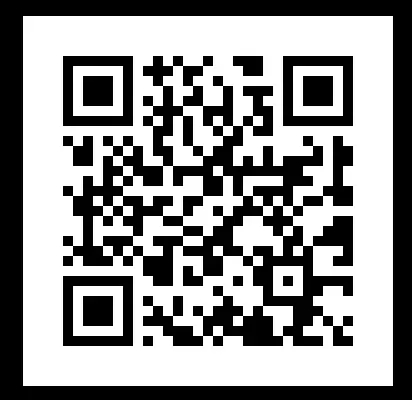
Example2: Producing a QR Code with Customized Colours
Right here what we’re doing is make the QR code extra visually interesting.Inorder to realize this we now have to both change foreground colour or background colour or change each.On this instance i hvae modified each foreground and background colours.You possibly can tryout the code with totally different colour combos.
import qrcode
from PIL import Picture
#Knowledge to be encoded
information = "Welcome to QR Code Tutorial"
#Create a QR code object
qr = qrcode.QRCode(
model=1, # Measurement of the QR code
box_size=10, # Measurement of every field within the QR code grid
border=4 # Border thickness
)
qr.add_data(information)
qr.make(match=True)
#Create a QR code with customized colours
img_colored = qr.make_image(fill_color="darkgreen", back_color="lightyellow")
img_colored.present()
img_colored.save('custom_color_qr_code.png')
The above code will generate a QR code with a darkish inexperienced foreground and light-weight yellow background. Scan the code to view the output with these customized colours.
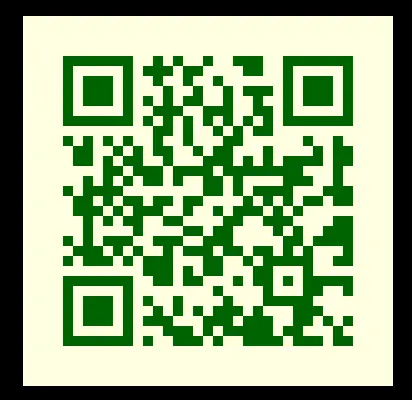
Example3: QR Code Technology from Analytics Vidhya URL
On this instance, making a QR code that encodes the URL of Analytics Vidhya. This enables anybody who scans the code to straight go to the web site. Right here’s the code:
import qrcode
from PIL import Picture
#Create the QR code as ordinary
qr = qrcode.QRCode(
model=5, # Model adjusted to incorporate the brand
box_size=10,
border=4
)
qr.add_data("https://www.analyticsvidhya.com/")
qr.make(match=True)
#Generate the QR code picture
img = qr.make_image(fill="black", back_color="white")
#Save and present the consequence
img.save('QR_code_AnalyticsVidhya.png')
img.present()
Simply scan it. The QR will direct you in direction of official web page of Analytics Vidhya
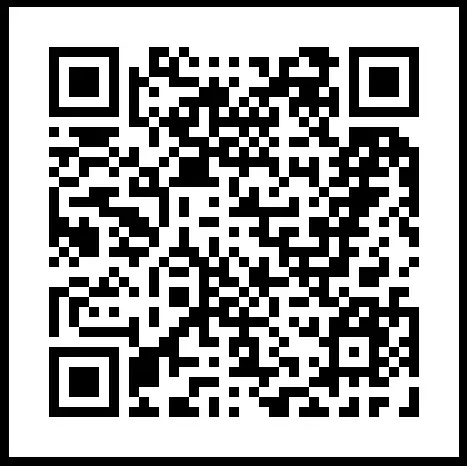
Example4: QR Code with Embedded Brand and URL Mixed
Right here we’ll tryout a personalised QR code that not solely hyperlinks to the Analytics Vidhya web site but additionally contains a customized brand embedded proper within the middle.It can provide a particular and branded look. Let’s dive into the code instance
import qrcode
from PIL import Picture
# Create the QR code as ordinary
qr = qrcode.QRCode(
model=5, # Model adjusted to incorporate a brand
box_size=10,
border=4
)
qr.add_data("https://www.analyticsvidhya.com/")
qr.make(match=True)
# Generate the QR code picture
img = qr.make_image(fill="black", back_color="white")
# Open the brand picture
brand = Picture.open('AV_logo.png')
# Calculate the scale for the brand
logo_size = 100 # Set this in accordance with your wants
brand = brand.resize((logo_size, logo_size), Picture.Resampling.LANCZOS) # Use LANCZOS for high-quality downsampling
# Paste the brand onto the QR code
pos = ((img.measurement[0] - logo_size) // 2, (img.measurement[1] - logo_size) // 2)
img.paste(brand, pos, masks=brand)
# Save and present the consequence
img.save('QR_code_with_AnalyticsVidhya_Logo.png')
img.present()
The next cropped picture is an instance you could strive along with your code:
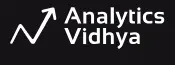
This model of code provides a contact of pleasure and emphasizes the distinctive, branded side of the QR code.
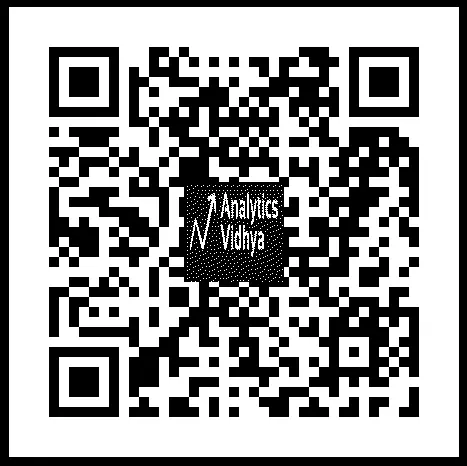
Example5: Studying QR Codes from an Picture
Right here, I’ll present the best way to learn and decode a QR code from a picture utilizing OpenCV. That is helpful when you must extract information from a QR code that’s already been created or shared in a picture file. Right here’s the code:Simply take the earlier output QR Code picture as new enter,then check out the next code
# !pip set up opencv-python
import cv2
# Learn the picture file
picture = cv2.imread('/residence/Tutorials/Analytics Vidya/QR_code_with_AnalyticsVidhya_Logo.png')
# Initialize the QR code detector
detector = cv2.QRCodeDetector()
# Detect and decode the QR code
information, vertices_array, _ = detector.detectAndDecode(picture)
if vertices_array is just not None:
print(f"Decoded Knowledge: {information}")
else:
print("QR code not detected.")
The output shall be:
" Decoded Knowledge: https://www.analyticsvidhya.com/ "
Mini-Challenge : Making a Wi-Fi QR Code
QR code is a straightforward option to permit customers to attach with a community. By simply scanning the code. Utilizing this we will keep away from manually typing wifi identify or password . We are able to implement a qr code that incorporates all the required info.
On this mini challenge ,I’ll information you to construct a QR code to retailer wifi particulars.It may be a lot helpful in public locations, workplaces in addition to for private use.The generated qr code will retailer wifi credentials, such because the community identify (SSID), password, and safety sort (in my case WPA2) ,permitting gadgets to attach with ease.
Inorder to tryout this mini challenge,1st you need to findout your Wi-Fi SSID,Wi-Fi Safety Kind and Password utilizing following linux instructions within the terminal.Bear in mind , strive one after one other
#1 Discover the Wi-Fi SSID (Community Identify)
nmcli -t -f energetic,ssid dev wifi | grep '^sure'
#2 Get Wi-Fi Safety Kind
# right here my SSID Identify is RAGAI_4G
nmcli -f ssid,safety dev wifi | grep "RAGAI_4G"
#3Get Password
sudo grep -r "psk=" /and many others/NetworkManager/system-connections/
Code Implementation
Allow us to now implement the code under:
# Import the required library
import qrcode
# Outline Wi-Fi credentials
wifi_ssid = "RAGAI_4G"#exchange along with your SSID Identify
wifi_password = "PASSWORD" #Ur PASSWORD
wifi_security = "WPA2" # Might be 'WEP', 'WPA', or 'nopass' (no password)
# Create the QR code information within the format that encodes Wi-Fi info
wifi_data = f"WIFI:T:{wifi_security};S:{wifi_ssid};P:{wifi_password};;"
# Create a QR code object with desired settings
qr = qrcode.QRCode(
model=1,
error_correction=qrcode.constants.ERROR_CORRECT_L,
box_size=10,
border=4
)
# Add the Wi-Fi information to the QR code object
qr.add_data(wifi_data)
qr.make(match=True)
# Generate the QR code picture
img = qr.make_image(fill="black", back_color="white")
# Save the QR code picture to a file
img.save('wifi_qr_code.png')
# Show the QR code picture (for testing)
img.present()
print("Wi-Fi QR code saved efficiently")
Scanning the QR Code
Anticipating you will have efficiently tried out the code i’ve shared.Now you will have the output QR code picture.Simply can scan it with a smartphone or scanner to connect with the wifi community. Comply with the steps one after the other:
- Open the digicam app or QR scanner in your telephone.
- Level the digicam on the QR code.
- Android telephones will show the Wi-Fi community identify and immediate to attach mechanically.
Bear in mind ,in case your telephone doesnot have inbuilt purposes,you possibly can obtain any scanner purposes from playstore.
Sensible Use Circumstances for QR Codes
This Wi-Fi QR code may be particularly useful in:
- Public Areas: In cafes, libraries,pumps or lodges the place guests can merely scan the code to attach while not having to ask for the credentials.
- Residence Networks: Giving family and friends a simple method to connect with your community with out sharing your password.
- Enterprise Settings: Sharing community entry with company or workers with out compromising safety.
Conclusion
The examples on this article may help you for varied functions and each in your interest tasks and actual time tasks.If any features happen in your house you possibly can invite your mates by saring the placement info with the assistance of a qr code.In case you are at workplace or faculty the identical may be utilized for occasion scheduling and kind filling.
Right here is the Github hyperlink.
Key Takeaways
- QR codes are versatile instruments that retailer varied information sorts, from URLs to Wi-Fi credentials.
- Python’s
qrcode
library simplifies the method of making and customizing QR codes. - QR codes improve consumer expertise by enabling quick, contactless info sharing.
- Customizing QR codes with colours or logos can enhance branding and visible enchantment.
- Wi-Fi QR codes present a seamless option to join gadgets to a community with out guide entry.
- Mastering QR Codes unlocks the potential to streamline information sharing, improve consumer experiences, and enhance entry to info shortly and successfully.
Steadily Requested Questions
A. A QR code is a two-dimensional barcode that shops info equivalent to URLs, textual content, or Wi-Fi credentials, and may be scanned shortly by a digicam or scanner.
A. You should utilize the qrcode
library in Python to generate QR codes by putting in it with pip set up qrcode[pil]
and making a QR code object along with your information.
A. Sure, you possibly can change the foreground and background colours, and even embed logos inside the QR code for a extra customized look.
A. A Wi-Fi QR code shops community credentials (SSID, password, and safety sort) so customers can hook up with a community by merely scanning the code.
A. Sure, QR codes serve varied purposes, equivalent to sharing URLs, contact info, occasion particulars, and even Wi-Fi credentials.
A. Mastering QR codes means that you can simply create and customise codes for varied functions, equivalent to sharing URLs, Wi-Fi credentials, and extra, enhancing comfort and accessibility in digital interactions.
The media proven on this article is just not owned by Analytics Vidhya and is used on the Creator’s discretion.