Introduction
Contemplate writing a code that entails features which can be linked, one to a different, in a method that doesn’t break the stream of a sentence. That’s methodology chaining in Python—an environment friendly method that makes it doable to invoke a number of strategies inside an object utilizing a single line of code. It makes code shorter, extra simply to learn, and straightforward to know, along with giving a fairly pure method of coding successive operations on information or objects. On this article we’ll cowl what methodology chaining is, its advantages and how one can put it to use in Python.
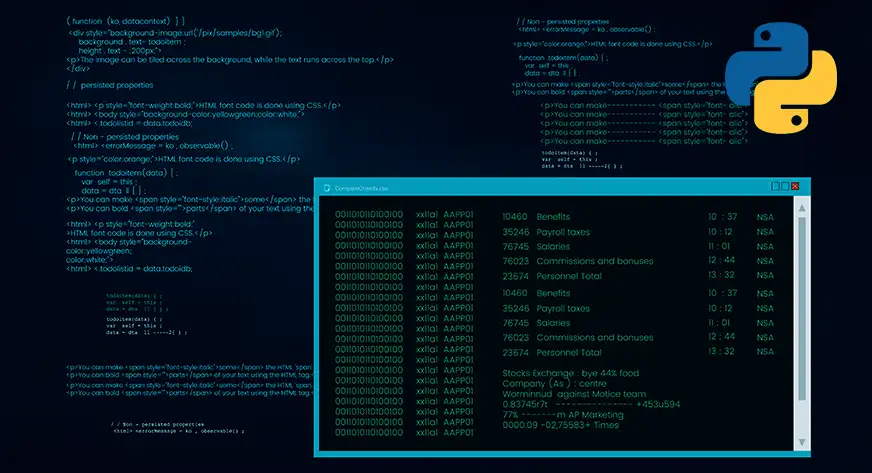
Studying Outcomes
- Perceive the idea of methodology chaining in Python.
- Implement methodology chaining in customized Python lessons.
- Acknowledge the benefits and drawbacks of methodology chaining.
- Enhance code readability and conciseness utilizing methodology chaining.
- Apply methodology chaining in real-world Python tasks.
What’s Methodology Chaining?
Methodology chaining is referring to the situation whereby one or many strategies are invoked successively in the identical line of code, on a given object. The naming conference of this chain of methodology calls is thus doable as a result of every of the strategies themselves return the item in query, or a derived model of it, as a parameter on which additional strategies might then be invoked. This makes the code extra fluent and streamlined, kind the syntactical viewpoint thereby making the code extra elegant.
In Python, methodology chaining is primarily made doable by strategies returning self
(or the present occasion of the item) after performing an motion. This implies the identical object is handed alongside the chain, enabling successive operations on that object with no need to retailer intermediate ends in variables.
Instance of Methodology Chaining
Allow us to now discover the instance of methodology chaining under:
class TextProcessor:
def __init__(self, textual content):
self.textual content = textual content
def remove_whitespace(self):
self.textual content = self.textual content.strip() # Removes main and trailing areas
return self
def to_upper(self):
self.textual content = self.textual content.higher() # Converts the textual content to uppercase
return self
def replace_word(self, old_word, new_word):
self.textual content = self.textual content.substitute(old_word, new_word) # Replaces old_word with new_word
return self
def get_text(self):
return self.textual content
# Utilizing methodology chaining
textual content = TextProcessor(" Howdy World ")
outcome = textual content.remove_whitespace().to_upper().replace_word('WORLD', 'EVERYONE').get_text()
print(outcome) # Output: "HELLO EVERYONE"
Right here, a number of strategies (remove_whitespace()
, to_upper()
, and replace_word()
) are known as in a series on the identical TextProcessor
object. Every methodology modifies the inner state of the item and returns self
, permitting the chain to proceed with the subsequent methodology.
Benefits of Methodology Chaining
Allow us to find out about benefits of methodology chaining.
- Decreased Boilerplate: Removes the necessity for intermediate variables, making the code cleaner.
- Improved Stream: Strategies may be mixed right into a single line of execution, making the code appear like a sequence of pure operations.
- Elegant Design: Provides the API a fluid and intuitive interface that’s simple to make use of for builders.
Disadvantages of Methodology Chaining
Allow us to find out about disadvantages of methodology chaining.
- Tough Debugging: If a bug happens, it’s tougher to pinpoint the precise methodology inflicting the issue since a number of strategies are known as in a single line.
- Advanced Chains: Lengthy chains can change into troublesome to learn and preserve, particularly if every methodology’s function isn’t clear.
- Coupling: Methodology chaining can tightly couple strategies, making it tougher to alter the category implementation with out affecting the chain.
How Methodology Chaining Works
Right here’s a deeper have a look at how methodology chaining works in Python, significantly with Pandas, utilizing a step-by-step breakdown.
Step 1: Preliminary Object Creation
You begin with an object. For instance, in Pandas, you usually create a DataFrame.
import pandas as pd
information = {'Identify': ['Alice', 'Bob', 'Charlie'], 'Age': [25, 30, 35]}
df = pd.DataFrame(information)
The df
object now holds a DataFrame with the next construction:
Identify Age
0 Alice 25
1 Bob 30
2 Charlie 35
Step 2: Methodology Name and Return Worth
You’ll be able to name a way on this DataFrame object. For instance:
renamed_df = df.rename(columns={'Identify': 'Full Identify'})
On this case, the rename
methodology returns a brand new DataFrame with the column Identify
modified to Full Identify
. The unique df
stays unchanged.
Step 3: Chaining Further Strategies
With methodology chaining, you’ll be able to instantly name one other methodology on the results of the earlier methodology name:
sorted_df = renamed_df.sort_values(by='Age')
This kinds the DataFrame based mostly on the Age
column. Nonetheless, as a substitute of storing the intermediate end in a brand new variable, you’ll be able to mix these steps:
outcome = df.rename(columns={'Identify': 'Full Identify'}).sort_values(by='Age')
Right here, outcome
now incorporates the sorted DataFrame with the renamed column.
Step 4: Persevering with the Chain
You’ll be able to proceed to chain extra strategies. As an illustration, you would possibly need to reset the index after sorting:
final_result = df.rename(columns={'Identify': 'Full Identify'}).sort_values(by='Age').reset_index(drop=True)
When to Use Methodology Chaining
Methodology chaining is especially helpful when coping with:
- Knowledge transformations: When you want to apply a sequence of transformations to an object (e.g., processing textual content, information cleansing, mathematical operations).
- Fluent APIs: Many libraries, akin to pandas or jQuery, implement methodology chaining to supply a extra user-friendly and readable interface.
In pandas, for instance, you’ll be able to chain a number of operations on a DataFrame:
import pandas as pd
information = {'Identify': ['Alice', 'Bob', 'Charlie'], 'Age': [25, 30, 35]}
df = pd.DataFrame(information)
# Chaining strategies
outcome = df.rename(columns={'Identify': 'Full Identify'}).sort_values(by='Age').reset_index(drop=True)
print(outcome)
Methodology Chaining with .strip(), .decrease(), and .substitute() in Python
Let’s dive deeper into how the string strategies .strip()
, .decrease()
, and .substitute()
work in Python. These are highly effective built-in string strategies generally used for manipulating and cleansing string information. I’ll clarify every methodology intimately, beginning with their function, use circumstances, and syntax, adopted by some examples.
.strip()
Methodology
The .strip() methodology is a string methodology that’s used to trim the string eliminating main and trailing areas. Whitespace is areas, tabs typically with the t notation, and newline characters typically with n notation. When known as with no arguments, .strip() methodology will trim the string eradicating all kinds of main and trailing areas.
The way it Works:
.strip()
is usually used when cleansing consumer enter, eradicating pointless areas from a string for additional processing or comparisons.- It doesn’t take away whitespace or characters from the center of the string, solely from the start and the tip.
Instance:
# Instance 1: Eradicating main and trailing areas
textual content = " Howdy, World! "
cleaned_text = textual content.strip()
print(cleaned_text) # Output: "Howdy, World!"
# Instance 2: Eradicating particular characters
textual content = "!!!Howdy, World!!!"
cleaned_text = textual content.strip("!")
print(cleaned_text) # Output: "Howdy, World"
.decrease()
Methodology
The.decrease() methodology makes all of the letters of a string decrease case that’s if there are higher case letters within the string it can change them. That is significantly useful to make use of when evaluating textual content in a method that’s case-insentitive or for different functions of equalization.
The way it Works:
- .decrease() methodology takes all of the uppercase characters in a string and places in consequence, their counterparts, the lowercase characters. Any image or numerals too are retained as it’s and don’t endure any modification.
- Usually used for textual content preprocessing the place the enter have to be transformed into an ordinary format extra particularly for case insensitive search or comparability.
Instance:
# Instance 1: Changing to lowercase
textual content = "HELLO WORLD"
lowercase_text = textual content.decrease()
print(lowercase_text) # Output: "whats up world"
# Instance 2: Case-insensitive comparability
name1 = "John"
name2 = "john"
if name1.decrease() == name2.decrease():
print("Names match!")
else:
print("Names don't match!")
# Output: "Names match!"
.substitute()
Methodology
The .substitute()
methodology is used to exchange occurrences of a substring inside a string with one other substring. It may be used to switch or clear strings by changing sure characters or sequences with new values.
The way it Works:
.substitute()
searches the string for all occurrences of theprevious
substring and replaces them with thenew
substring. By default, it replaces all occurrences until a selectedrely
is given.- This methodology is especially helpful for duties like cleansing or standardizing information, or for formatting textual content.
Instance:
# Instance 1: Fundamental substitute
textual content = "Howdy World"
new_text = textual content.substitute("World", "Everybody")
print(new_text) # Output: "Howdy Everybody"
# Instance 2: Exchange solely a sure variety of occurrences
textual content = "apple apple apple"
new_text = textual content.substitute("apple", "banana", 2)
print(new_text) # Output: "banana banana apple"
Greatest Practices for Methodology Chaining
- Return
self
Fastidiously: Be sure that the item returned from every methodology is similar one being manipulated. Keep away from returning new objects until it’s a part of the specified conduct. - Readable Chains: Whereas methodology chaining enhances readability, keep away from overly lengthy chains that may be troublesome to debug.
- Error Dealing with: Implement acceptable error dealing with in your strategies to make sure that invalid operations in a series don’t trigger sudden failures.
- Design for Chaining: Methodology chaining is most helpful in lessons designed to carry out a sequence of transformations or operations. Guarantee your strategies function logically in sequence.
Actual-World Use Circumstances of Methodology Chaining
- Pandas DataFrame Operations: Pandas extensively makes use of methodology chaining to permit successive operations on a DataFrame.
import pandas as pd
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
outcome = df.dropna().sort_values('A').reset_index(drop=True)
- Flask Net Framework: The Flask framework for constructing internet functions makes use of methodology chaining for routing and response era.
from flask import Flask, jsonify
app = Flask(__name__)
@app.route("https://www.analyticsvidhya.com/")
def index():
return jsonify(message="Howdy, World!").status_code(200)
Pitfalls of Methodology Chaining
Though methodology chaining has many benefits, there are some potential pitfalls to pay attention to:
- Complexity: Whereas concise, lengthy chains can change into obscure and debug. If a way in the course of a series fails, it may be tough to isolate the issue.
- Error Dealing with: Since methodology chaining depends upon every methodology returning the right object, if one methodology doesn’t return
self
or raises an error, your complete chain can break down. - Readability Points: If not used fastidiously, methodology chaining can cut back readability. Chains which can be too lengthy or contain too many steps can change into tougher to observe than breaking the chain into separate steps.
- Tight Coupling: Methodology chaining might tightly couple strategies, making it troublesome to switch the category’s conduct with out affecting current chains of calls.
Conclusion
It’s essential to notice that methodology chaining in Python truly supplies a method that’s efficient and exquisite. In case you return the item from every of those strategies, they’re offered as a fluent interface, the code seems to be far more pure. Methodology chaining is definitely an excellent function, however one must be very cautious with its utilization as overcomplicated or too lengthy chains are hardly comprehensible and will trigger difficulties in debugging. Making use of greatest practices when utilizing methodology chaining in your programmed-in Python provides your work effectivity and readability.
Regularly Requested Questions
A. No, solely lessons designed to return the occasion (self
) from every methodology can help methodology chaining. It’s good to implement this sample manually in customized lessons.
A. Methodology chaining itself doesn’t enhance efficiency; it primarily improves code readability and reduces the necessity for intermediate variables.
A. Sure, debugging may be tougher when utilizing methodology chaining as a result of a number of operations happen in a single line, making it troublesome to hint errors. Nonetheless, this may be mitigated by protecting chains brief and utilizing correct logging.
A. Sure, many built-in sorts like strings and lists in Python help methodology chaining as a result of their strategies return new objects or modified variations of the unique object.