Introduction
Suppose you might be planning a really huge occasion and notice that you must decide essentially the most environment friendly approach of distributing the workload among the many crew members. You try a few approaches however end up getting caught and are unable to maneuver ahead. That is the place native search algorithms are available in. Hill climbing and simulated annealing are a few of these methods that can help you escape these repetitive issues and develop improved options.
On this article, We’ll focus on concerning the LS algorithms, the place they’re utilized in AI, and the way it could make you higher downside solver regardless of you might be in job scheduling or operate optimization.
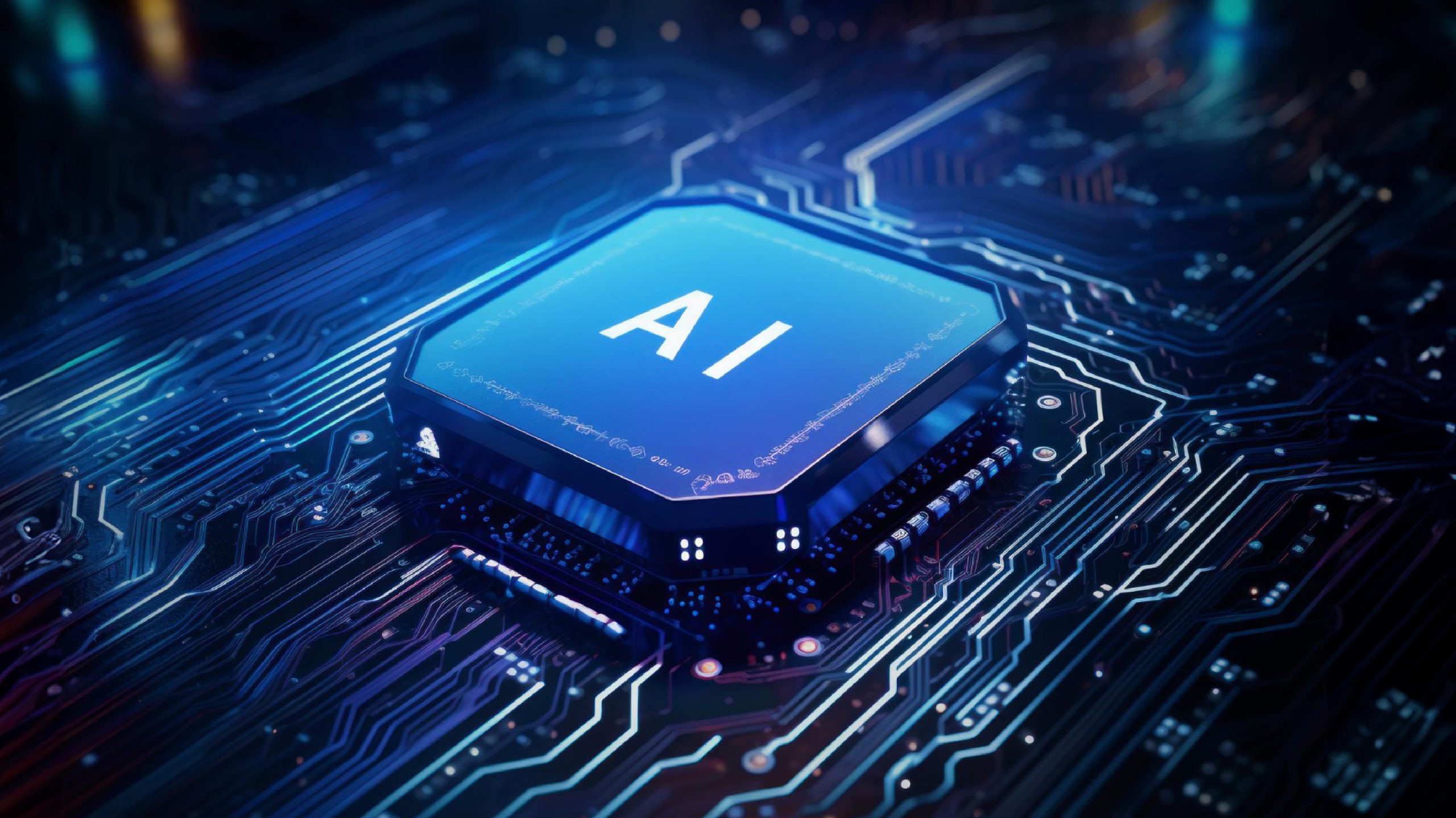
Studying Outcomes
- Perceive the core rules of native search algorithms.
- Determine widespread sorts of native search algorithms and their use circumstances.
- Discover ways to implement and apply these algorithms in sensible situations.
- Achieve insights into optimizing native search processes and dealing with potential challenges.
Core Rules of Native Search Algorithms
Native search algorithms are meant to unravel optimization issues by shifting from one resolution to the opposite within the neighborhood. In easy phrases, it consists of taking an preliminary resolution and making incremental adjustments to it to optimize it.
- Preliminary Resolution: Begin with an preliminary guess or resolution.
- Neighbor Technology: Generate neighboring options by making small modifications to the present resolution.
- Analysis: Assess the standard of the neighboring options utilizing a predefined goal operate.
- Choice: Select the most effective neighbor as the brand new present resolution.
- Termination: Repeat the method till a stopping criterion is met (e.g., a most variety of iterations or no enchancment).
Widespread Kinds of Native Search Algorithms
- Hill Climbing: A easy algorithm that constantly strikes to the neighboring resolution with the very best worth. It’s intuitive however can get caught in native optima.
- Simulated Annealing: An extension of hill climbing that permits occasional strikes to worse options to flee native optima. It makes use of a temperature parameter that steadily decreases over time.
- Genetic Algorithms: Though many researchers categorize GA as belonging to the place of the evolutionary algorithms class, these algorithms additionally use options of native search by means of processes like mutation and crossover to go looking the answer area.
- Tabu Search: Tabu search is a extra subtle methodology than the essential Hill Climbing algorithm as a result of it consists of particular reminiscence constructions that stop the options’ return to earlier states, thus escaping native optima.
- Particle-Swarm Optimization (PSO): One other method, Particle-Swarm Optimization (PSO), tries to discover a resolution within the area of a operate; throughout this particles examine their positions and modify them based on their greatest particular person place and the most effective place of all the swarm. This methodology helps give you the most effective options by means of the optimization of multi-variable features in a particular approach.
Sensible Implementation
To successfully implement native search algorithms, observe these steps:
- Outline the Downside: Clearly articulate the optimization downside, together with the target operate and constraints.
- Select an Algorithm: Choose a neighborhood search algorithm suited to the issue traits.
- Implement the Algorithm: Write code to initialize the answer, generate neighbors, consider them, and deal with termination.
- Tune Parameters: Regulate algorithm parameters (e.g., temperature in simulated annealing) to steadiness exploration and exploitation.
- Validate Outcomes: Check the algorithm on varied cases of the issue to make sure it performs nicely.
Examples of Native Search Algorithms
Allow us to now look into some native search algorithms beneath intimately.
Hill Climbing
Hill Climbing is an easy method that strikes to the neighboring resolution with the very best worth. Though intuitive, it may well get caught in native optima.
Instance
def hill_climbing(initial_solution, objective_function):
current_solution = initial_solution
current_score = objective_function(current_solution)
whereas True:
neighbors = generate_neighbors(current_solution)
best_neighbor = None
best_neighbor_score = current_score
for neighbor in neighbors:
rating = objective_function(neighbor)
if rating > best_neighbor_score:
best_neighbor = neighbor
best_neighbor_score = rating
if best_neighbor is None:
break
current_solution = best_neighbor
current_score = best_neighbor_score
return current_solution, current_score
def generate_neighbors(resolution):
# Instance neighbor technology for a easy case
return [solution + 1, solution - 1]
def objective_function(x):
return -x**2 # Instance: maximization downside
initial_solution = 0
best_solution, best_score = hill_climbing(initial_solution, objective_function)
print(f"Greatest resolution: {best_solution} with rating: {best_score}")
Output:
Greatest resolution: 0 with rating: 0
Simulated Annealing
The premise of the Simulated Annealing algorithm is the annealing course of referring to metallurgy the place the steel is steadily cooled with a view to eradicate the presence of defects in its construction. It initializes the temperature to be excessive, such that the algorithm can traverse extra space of resolution after which comes down with low temperatures to cut back the time of accepting resolution which is worse.
Instance
Let give attention to the formal downside, corresponding to a touring salesman downside through which a salesman has to journey by means of a number of cities and get again to the start line within the minimal period of time. One method to shortly discover a constraint-optimal route is to make use of simulated annealing. This methodology generally accepts an extended route in hopes of discovering a greater general route.
import random
import math
def objective_function(route):
# Instance operate: the whole distance of the route
return sum(math.sqrt((route[i] - route[i-1])**2) for i in vary(len(route)))
def simulated_annealing(initial_route, temperature, cooling_rate):
current_route = initial_route
current_score = objective_function(current_route)
best_route = current_route
best_score = current_score
whereas temperature > 0.1:
new_route = current_route[:]
i, j = random.pattern(vary(len(route)), 2)
new_route[i], new_route[j] = new_route[j], new_route[i]
new_score = objective_function(new_route)
if new_score < current_score or random.random() < math.exp((current_score - new_score) / temperature):
current_route = new_route
current_score = new_score
if new_score < best_score:
best_route = new_route
best_score = new_score
temperature *= cooling_rate
return best_route, best_score
# Instance utilization
route = [0, 1, 2, 3, 4]
best_route, best_score = simulated_annealing(route, 1000, 0.995)
print(f"Greatest route: {best_route} with rating: {best_score}")
Output:
Greatest route: [0, 1, 2, 3, 4] with rating: 8.0
Tabu Search
Tabu Search makes use of reminiscence constructions to maintain monitor of lately visited options, stopping the algorithm from revisiting them. This helps in avoiding cycles and encourages exploration of latest areas of the answer area.
Instance
You’ll be able to make use of tabu search in job scheduling issues to allocate jobs to totally different machines and reduce complete completion time by avoiding lately tried job allocations.
import random
def objective_function(schedule):
# Instance operate: complete completion time
return sum(job['duration'] for job in schedule)
def tabu_search(initial_schedule, iterations, tabu_tenure):
current_schedule = initial_schedule
best_schedule = current_schedule
best_score = objective_function(current_schedule)
tabu_list = []
for _ in vary(iterations):
neighbors = generate_neighbors(current_schedule)
best_neighbor = None
best_neighbor_score = float('inf')
for neighbor in neighbors:
if neighbor not in tabu_list:
rating = objective_function(neighbor)
if rating < best_neighbor_score:
best_neighbor = neighbor
best_neighbor_score = rating
if best_neighbor:
current_schedule = best_neighbor
tabu_list.append(current_schedule)
if len(tabu_list) > tabu_tenure:
tabu_list.pop(0)
if best_neighbor_score < best_score:
best_schedule = best_neighbor
best_score = best_neighbor_score
return best_schedule, best_score
def generate_neighbors(schedule):
# Generate neighbors by swapping job allocations
neighbors = []
for i in vary(len(schedule)):
for j in vary(i + 1, len(schedule)):
neighbor = schedule[:]
neighbor[i], neighbor[j] = neighbor[j], neighbor[i]
neighbors.append(neighbor)
return neighbors
# Instance utilization
schedule = [{'job': 'A', 'duration': 3}, {'job': 'B', 'duration': 2}, {'job': 'C', 'duration': 1}]
best_schedule, best_score = tabu_search(schedule, 100, 5)
print(f"Greatest schedule: {best_schedule} with rating: {best_score}")
Output:
Greatest schedule: [{'job': 'A', 'duration': 3}, {'job': 'B', 'duration': 2}, {'job': 'C', 'duration': 1}] with rating: 6
Grasping Algorithms
Many organizations use GA construct up resolution piece by piece and it’s typically selecting the piece that brings essentially the most advantages within the brief run. Whereas will not be the most effective options at all times, they may very well be highly effective in sorts of issues.
Instance
Within the knapsack downside, if you could seize as a lot worth as doable inside the allowed weight of the bag, you’ll be able to deal with it by adopting a grasping algorithm. This method types gadgets based mostly on their value-to-weight ratio.
def knapsack_greedy(gadgets, capability):
gadgets = sorted(gadgets, key=lambda x: x['value'] / x['weight'], reverse=True)
total_value = 0
total_weight = 0
for merchandise in gadgets:
if total_weight + merchandise['weight'] <= capability:
total_weight += merchandise['weight']
total_value += merchandise['value']
else:
break
return total_value
# Instance utilization
gadgets = [{'value': 60, 'weight': 10}, {'value': 100, 'weight': 20}, {'value': 120, 'weight': 30}]
capability = 50
best_value = knapsack_greedy(gadgets, capability)
print(f"Most worth in knapsack: {best_value}")
Output:
Most worth in knapsack: 160
Particle Swarm Optimization
PSO is predicated on the imitation of birds’ and fishes’ exercise. Brokers (or particles) roam within the search area of the issues whereas modifying their positions based on their very own studying experiences in addition to the training experiences of their neighbors.
Instance
You’ll be able to apply PSO to operate optimization issues, the place particles discover the operate’s area and replace their positions based mostly on their particular person and collective greatest options.
import numpy as np
def objective_function(x):
return sum(x**2)
def particle_swarm_optimization(num_particles, dimensions, iterations):
particles = np.random.rand(num_particles, dimensions)
velocities = np.random.rand(num_particles, dimensions)
personal_best = particles.copy()
global_best = particles[np.argmin([objective_function(p) for p in particles])]
for _ in vary(iterations):
for i in vary(num_particles):
r1, r2 = np.random.rand(dimensions), np.random.rand(dimensions)
velocities[i] = 0.5 * velocities[i] + 2 * r1 * (personal_best[i] - particles[i]) + 2 * r2 * (global_best - particles[i])
particles[i] += velocities[i]
if objective_function(particles[i]) < objective_function(personal_best[i]):
personal_best[i] = particles[i]
if objective_function(personal_best[i]) < objective_function(global_best):
global_best = personal_best[i]
return global_best, objective_function(global_best)
# Instance utilization
best_position, best_value = particle_swarm_optimization(30, 5, 100)
print(f"Greatest place: {best_position} with worth: {best_value}")
Output:
Greatest place: [ 3.35110987e-07 6.94381793e-07 -1.03625781e-06 2.22941746e-06
-9.73259302e-07] with worth: 7.585831600413816e-12
Conclusion
The native search algorithms are environment friendly instruments for the decision-making to unravel the optimization points, contemplating the advance of the sure neighborhood options. That’s the reason introduction to indices even from the side of native search is instrumental upon the accomplishment of cognitive Preliminary theorems whatever the duties you might be more likely to encounter – schedule willpower, routing or forms of design issues. For those who make a sensible choice of the algorithm, tune the parameters accurately and test the outcomes, can address advanced resolution of the area and procure or nearly the most effective resolution to unravel the issue into account.
Steadily Requested Questions
A. Native search algorithms are efficient at discovering good options to optimization issues by means of iterative enchancment, making them appropriate for issues the place actual options are troublesome to acquire.
A. You’ll be able to enhance native search algorithms by incorporating methods like simulated annealing, tabu search, or hybrid approaches to flee native optima and improve resolution high quality.
A. Hill climbing can get caught in native optima and will not discover all the resolution area, which limits its skill to seek out the worldwide optimum.
A. Simulated annealing permits occasional strikes to worse options to flee native optima, whereas hill climbing solely strikes to raised options.
A. The tabu record in tabu search helps keep away from revisiting lately explored options, thereby enhancing the search’s skill to discover new areas of the answer area.