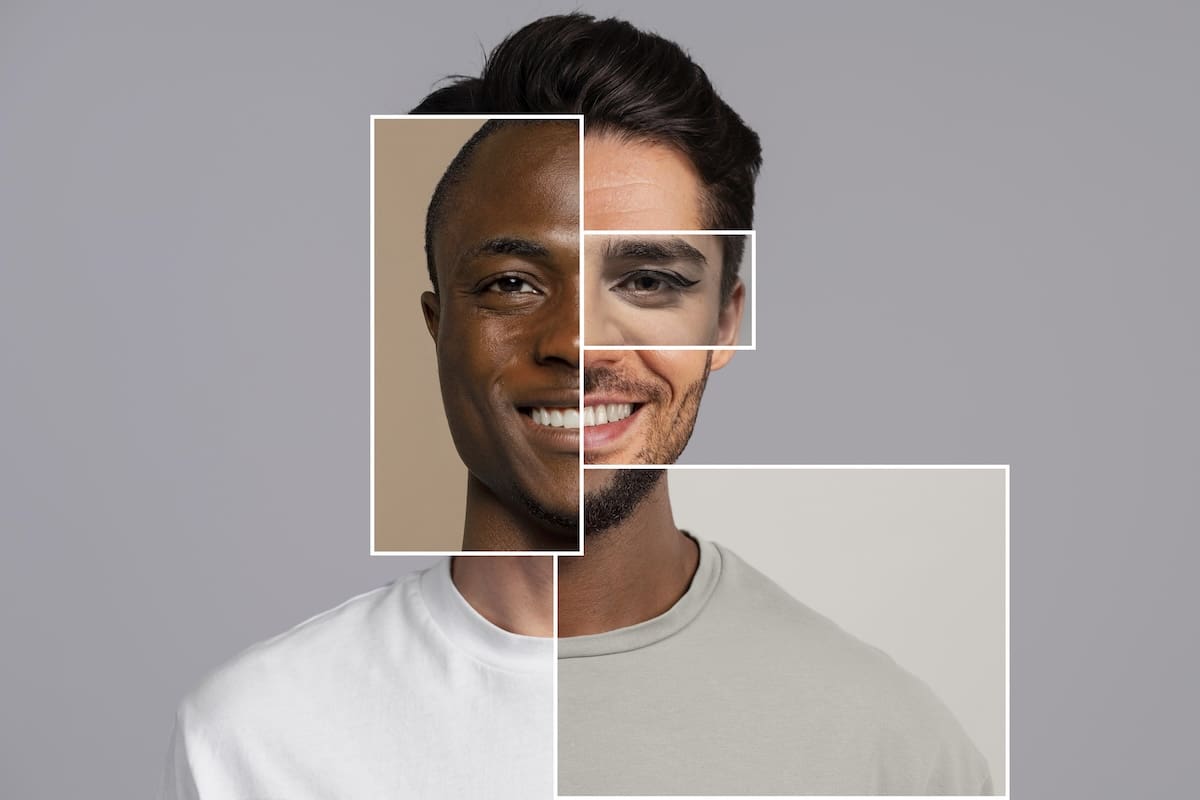
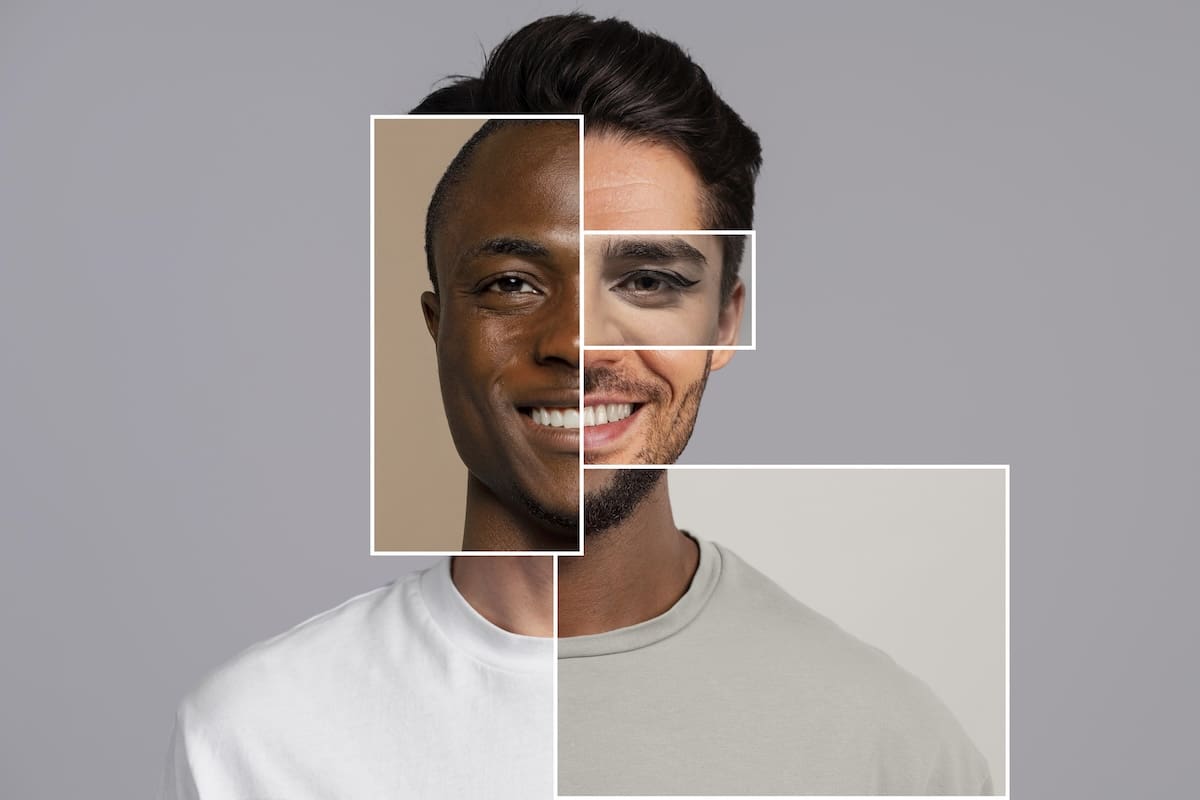
Picture by freepik
NumPy is a sturdy device for picture processing in Python. It allows you to manipulate photos utilizing array operations. This text explores a number of picture processing strategies utilizing NumPy.
Importing Libraries
We should import the required libraries: PIL, NumPy, and Matplotlib. PIL is used for opening photos. NumPy permits for environment friendly array operations and picture processing. Matplotlib is used for visualizing photos
import numpy as np
from PIL import Picture
import matplotlib.pyplot as plt
Crop Picture
We outline coordinates to mark the world we wish to crop from the picture. The brand new picture accommodates solely the chosen half and discards the remainder.
# Load the picture utilizing PIL (Python Imaging Library)
img = Picture.open('cat.jpg')
# Convert the picture to a NumPy array
img_array = np.array(img)
# Outline the cropping coordinates
y1, x1 = 1000, 1000 # Prime-left nook of ROI
y2, x2 = 2500, 2000 # Backside-right nook of ROI
cropped_img = img_array[y1:y2, x1:x2]
# Show the unique picture and the cropped picture
plt.determine(figsize=(10, 5))
# Show the unique picture
plt.subplot(1, 2, 1)
plt.imshow(img_array)
plt.title('Unique Picture')
plt.axis('off')
# Show the cropped picture
plt.subplot(1, 2, 2)
plt.imshow(cropped_img)
plt.title('Cropped Picture')
plt.axis('off')
plt.tight_layout()
plt.present()
Rotate Picture
We rotate the picture array 90 levels counterclockwise utilizing NumPy’s ‘rot90’ perform.
# Load the picture utilizing PIL (Python Imaging Library)
img = Picture.open('cat.jpg')
# Convert the picture to a NumPy array
img_array = np.array(img)
# Rotate the picture by 90 levels counterclockwise
rotated_img = np.rot90(img_array)
# Show the unique picture and the rotated picture
plt.determine(figsize=(10, 5))
# Show the unique picture
plt.subplot(1, 2, 1)
plt.imshow(img_array)
plt.title('Unique Picture')
plt.axis('off')
# Show the rotated picture
plt.subplot(1, 2, 2)
plt.imshow(rotated_img)
plt.title('Rotated Picture (90 levels)')
plt.axis('off')
plt.tight_layout()
plt.present()
Flip Picture
We use NumPy’s ‘fliplr’ perform to flip the picture array horizontally.
# Load the picture utilizing PIL (Python Imaging Library)
img = Picture.open('cat.jpg')
# Convert the picture to a NumPy array
img_array = np.array(img)
# Flip the picture horizontally
flipped_img = np.fliplr(img_array)
# Show the unique picture and the flipped picture
plt.determine(figsize=(10, 5))
# Show the unique picture
plt.subplot(1, 2, 1)
plt.imshow(img_array)
plt.title('Unique Picture')
plt.axis('off')
# Show the flipped picture
plt.subplot(1, 2, 2)
plt.imshow(flipped_img)
plt.title('Flipped Picture')
plt.axis('off')
plt.tight_layout()
plt.present()
Destructive of an Picture
The adverse of a picture is made by reversing its pixel values. In grayscale photos, every pixel’s worth is subtracted from the utmost (255 for 8-bit photos). In colour photos, that is finished individually for every colour channel.
# Load the picture utilizing PIL (Python Imaging Library)
img = Picture.open('cat.jpg')
# Convert the picture to a NumPy array
img_array = np.array(img)
# Verify if the picture is grayscale or RGB
is_grayscale = len(img_array.form)
Binarize Picture
Binarizing a picture converts it to black and white. Every pixel is marked black or white primarily based on a threshold worth. Pixels which might be lower than the brink turn out to be 0 (black) and above these above it turn out to be 255 (white).
# Load the picture utilizing PIL (Python Imaging Library)
img = Picture.open('cat.jpg')
# Convert the picture to grayscale
img_gray = img.convert('L')
# Convert the grayscale picture to a NumPy array
img_array = np.array(img_gray)
# Binarize the picture utilizing a threshold
threshold = 128
binary_img = np.the place(img_array
Colour Area Conversion
Colour house conversion modifications a picture from one colour mannequin to a different. That is finished by altering the array of pixel values. We use a weighted sum of the RGB channels to transform a colour picture to a grayscale.
# Load the picture utilizing PIL (Python Imaging Library)
img = Picture.open('cat.jpg')
# Convert the picture to a NumPy array
img_array = np.array(img)
# Grayscale conversion components: Y = 0.299*R + 0.587*G + 0.114*B
gray_img = np.dot (img_array[..., :3], [0.299, 0.587, 0.114])
# Show the unique RGB picture
plt.determine(figsize=(10, 5))
plt.subplot(1, 2, 1)
plt.imshow(img_array)
plt.title('Unique RGB Picture')
plt.axis('off')
# Show the transformed grayscale picture
plt.subplot(1, 2, 2)
plt.imshow(gray_img, cmap='grey')
plt.title('Grayscale Picture')
plt.axis('off')
plt.tight_layout()
plt.present()
Pixel Depth Histogram
The histogram exhibits the distribution of pixel values in a picture. The picture is flattened right into a one-dimensional array to compute the histogram.
# Load the picture utilizing PIL (Python Imaging Library)
img = Picture.open('cat.jpg')
# Convert the picture to a NumPy array
img_array = np.array(img)
# Compute the histogram of the picture
hist, bins = np.histogram(img_array.flatten(), bins=256, vary= (0, 256))
# Plot the histogram
plt.determine(figsize=(10, 5))
plt.hist(img_array.flatten(), bins=256, vary= (0, 256), density=True, colour="grey")
plt.xlabel('Pixel Depth')
plt.ylabel('Normalized Frequency')
plt.title('Histogram of Grayscale Picture')
plt.grid(True)
plt.present()
Masking Picture
Masking a picture means exhibiting or hiding elements primarily based on guidelines. Pixels marked as 1 are saved whereas pixels marked as 0 are hidden.
# Load the picture utilizing PIL (Python Imaging Library)
img = Picture.open('cat.jpg')
# Convert the picture to a NumPy array
img_array = np.array(img)
# Create a binary masks
masks = np.zeros_like(img_array[:, :, 0], dtype=np.uint8)
middle = (img_array.form[0] // 2, img_array.form[1] // 2)
radius = min(img_array.form[0], img_array.form[1]) // 2 # Enhance radius for an even bigger circle
rr, cc = np.meshgrid(np.arange(img_array.form[0]), np.arange(img_array.form[1]), indexing='ij')
circle_mask = (rr - middle [0]) ** 2 + (cc - middle [1]) ** 2
Wrapping Up
This text confirmed other ways to course of photos utilizing NumPy. We used PIL, NumPy and Matplotlib to crop, rotate, flip, and binarize photos. Moreover, we discovered about creating picture negatives, altering colour areas, making histograms, and making use of masks.
Jayita Gulati is a machine studying fanatic and technical author pushed by her ardour for constructing machine studying fashions. She holds a Grasp’s diploma in Laptop Science from the College of Liverpool.