Dealing with lacking knowledge is likely one of the commonest challenges in knowledge evaluation and machine studying. Lacking values can come up for varied causes, similar to errors in knowledge assortment, guide omissions, and even the pure absence of data. Whatever the trigger, these gaps can considerably affect your evaluation’s or predictive fashions’ high quality and accuracy.
Pandas, one of the vital standard Python libraries for knowledge manipulation, offers strong instruments to cope with lacking values successfully. Amongst these, the fillna() methodology stands out as a flexible and environment friendly strategy to deal with lacking knowledge by way of imputation. This methodology means that you can substitute lacking values with a selected worth, the imply, median, mode, and even forward- and backward-fill strategies, making certain that your dataset is full and analysis-ready.
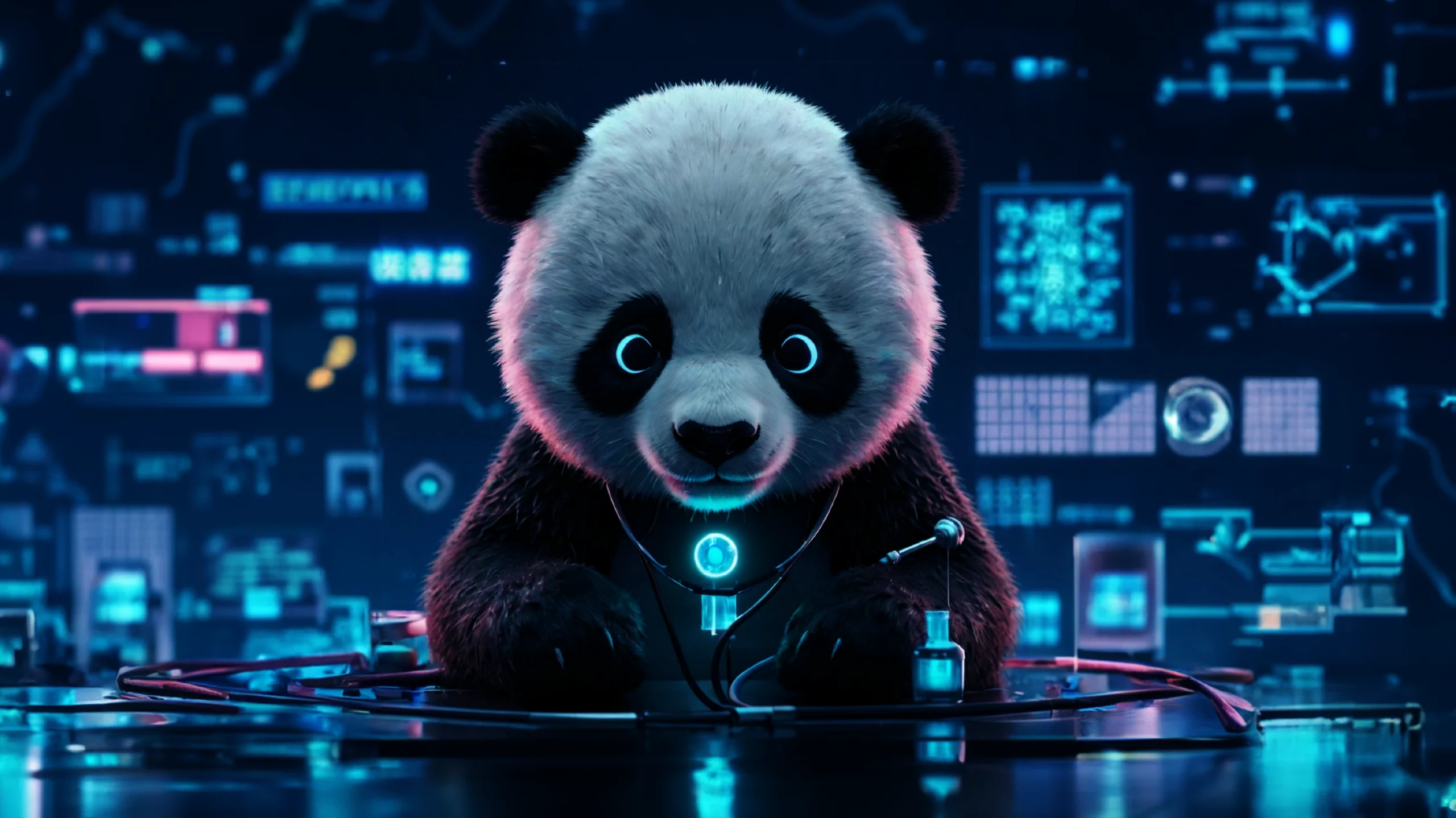
What’s Information Imputation?
Information imputation is the method of filling in lacking or incomplete knowledge in a dataset. When knowledge is lacking, it could possibly create issues in evaluation, as many algorithms and statistical strategies require an entire dataset to operate correctly. Information imputation addresses this challenge by estimating and changing the lacking values with believable ones, primarily based on the present knowledge within the dataset.
Why is Information Imputation Vital?
Right here’s why:
Distorted Dataset
- Lacking knowledge can skew the distribution of variables, altering the dataset’s integrity. This distortion might result in anomalies, change the relative significance of classes, and produce deceptive outcomes.
- For instance, a excessive variety of lacking values in a selected demographic group may trigger incorrect weighting in a survey evaluation.
Limitations with Machine Studying Libraries
- Most machine studying libraries, similar to Scikit-learn, assume that datasets are full. Lacking values could cause errors or forestall the profitable execution of algorithms, as these instruments usually lack built-in mechanisms for dealing with such points.
- Builders should preprocess the info to deal with lacking values earlier than feeding it into these fashions.
Influence on Mannequin Efficiency
- Lacking knowledge introduces bias, resulting in inaccurate predictions and unreliable insights. A mannequin skilled on incomplete or improperly dealt with knowledge would possibly fail to generalize successfully.
- As an example, if earnings knowledge is lacking predominantly for a selected group, the mannequin might fail to seize key tendencies associated to that group.
Want to Restore Dataset Completeness
- In circumstances the place knowledge is important or datasets are small, shedding even a small portion can considerably affect the evaluation. Imputation turns into important to retain all obtainable info whereas mitigating the consequences of lacking knowledge.
- For instance, a small medical research dataset would possibly lose statistical significance if rows with lacking values are eliminated.
Additionally learn: Pandas Capabilities for Information Evaluation and Manipulation
Understanding fillna() in Pandas
The fillna() methodology replaces lacking values (NaN) in a DataFrame or Sequence with specified values or computed ones. Lacking values can come up on account of varied causes, similar to incomplete knowledge entry or knowledge extraction errors. Addressing these lacking values ensures the integrity and reliability of your evaluation or mannequin.
Syntax of fillna() in Pandas
There are some vital parameters obtainable in fillna():
- worth: Scalar, dictionary, Sequence, or DataFrame to fill the lacking values.
- methodology: Imputation methodology. Might be:
- ‘ffill’ (ahead fill): Replaces NaN with the final legitimate worth alongside the axis.
- ‘bfill’ (backward fill): Replaces NaN with the subsequent legitimate worth.
- axis: Axis alongside which to use the tactic (0 for rows, 1 for columns).
- inplace: If True, modifies the unique object.
- restrict: Most variety of consecutive NaNs to fill.
- downcast: Makes an attempt to downcast the ensuing knowledge to a smaller knowledge sort.
Utilizing fillna() for Completely different Information Imputation Strategies
There are a number of knowledge Imputation strategies which goals to protect the dataset’s construction and statistical properties whereas minimizing bias. These strategies vary from easy statistical approaches to superior machine learning-based methods, every suited to particular kinds of knowledge and missingness patterns.
We’ll see a few of these strategies which will be applied with fillna():
1. Subsequent or Earlier Worth
For time-series or ordered knowledge, imputation strategies usually leverage the pure order of the dataset, assuming that close by values are extra comparable than distant ones. A typical strategy replaces lacking values with both the subsequent or earlier worth within the sequence. This system works properly for each nominal and numerical knowledge.
import pandas as pd
knowledge = {'Time': [1, 2, 3, 4, 5], 'Worth': [10, None, None, 25, 30]}
df = pd.DataFrame(knowledge)
# Ahead fill
df_ffill = df.fillna(methodology='ffill')
# Backward fill
df_bfill = df.fillna(methodology='bfill')
print(df_ffill)
print(df_bfill)
Additionally learn: Efficient Methods for Dealing with Lacking Values in Information Evaluation
2. Most or Minimal Worth
When the info is understood to fall inside a selected vary, lacking values will be imputed utilizing both the utmost or minimal boundary of that vary. This methodology is especially helpful when knowledge assortment devices saturate at a restrict. For instance, if a value cap is reached in a monetary market, the lacking value will be changed with the utmost allowable worth.
import pandas as pd
knowledge = {'Time': [1, 2, 3, 4, 5], 'Worth': [10, None, None, 25, 30]}
df = pd.DataFrame(knowledge)
# Impute lacking values with the minimal worth of the column
df_min = df.fillna(df.min())
# Impute lacking values with the utmost worth of the column
df_max = df.fillna(df.max())
print(df_min)
print(df_max)
3. Imply Imputation
Imply Imputation includes changing lacking values with the imply (common) worth of the obtainable knowledge within the column. This can be a easy strategy that works properly when the info is comparatively symmetrical and freed from outliers. The imply represents the central tendency of the info, making it an affordable selection for imputation when the dataset has a standard distribution. Nevertheless, the foremost downside of utilizing the imply is that it’s delicate to outliers. Excessive values can skew the imply, resulting in an imputation that will not replicate the true distribution of the info. Due to this fact, it’s not best for datasets with important outliers or skewed distributions.
import pandas as pd
import numpy as np
# Pattern dataset with lacking values
knowledge = {'A': [1, 2, np.nan, 4, 5, np.nan, 7],
'B': [10, np.nan, 30, 40, np.nan, 60, 70]}
df = pd.DataFrame(knowledge)
# Imply Imputation
df['A_mean'] = df['A'].fillna(df['A'].imply())
print("Dataset after Imputation:")
print(df)
4. Median Imputation
Median Imputation replaces lacking values with the median worth, which is the center worth when the info is ordered. This methodology is particularly helpful when the info comprises outliers or is skewed. In contrast to the imply, the median is not affected by excessive values, making it a extra strong selection in such circumstances. When the info has a excessive variance or comprises outliers that would distort the imply, the median offers a greater measure of central tendency. Nevertheless, one draw back is that it could not seize the complete variability within the knowledge, particularly in datasets that observe a regular distribution. Thus, in such circumstances, the imply would usually present a extra correct illustration of the info’s true central worth.
import pandas as pd
import numpy as np
# Pattern dataset with lacking values
knowledge = {'A': [1, 2, np.nan, 4, 5, np.nan, 7],
'B': [10, np.nan, 30, 40, np.nan, 60, 70]}
df = pd.DataFrame(knowledge)
# Median Imputation
df['A_median'] = df['A'].fillna(df['A'].median())
print("Dataset after Imputation:")
print(df)
5. Shifting Common Imputation
The Shifting Common Imputation methodology calculates the common of a specified variety of surrounding values, referred to as a “window,” and makes use of this common to impute lacking knowledge. This methodology is especially beneficial for time-series knowledge or datasets the place observations are associated to earlier or subsequent ones. The transferring common helps clean out fluctuations, offering a extra contextual estimate for lacking values. It’s generally used to deal with gaps in time-series knowledge, the place the idea is that close by values are prone to be extra comparable. The most important drawback is that it could possibly introduce bias if the info has massive gaps or irregular patterns, and it can be computationally intensive for big datasets or complicated transferring averages. Nevertheless, it’s extremely efficient in capturing temporal relationships throughout the knowledge.
import pandas as pd
import numpy as np
# Pattern dataset with lacking values
knowledge = {'A': [1, 2, np.nan, 4, 5, np.nan, 7],
'B': [10, np.nan, 30, 40, np.nan, 60, 70]}
df = pd.DataFrame(knowledge)
# Shifting Common Imputation (utilizing a window of two)
df['A_moving_avg'] = df['A'].fillna(df['A'].rolling(window=2, min_periods=1).imply())
print("Dataset after Imputation:")
print(df)
6. Rounded Imply Imputation
The Rounded Imply Imputation approach includes changing lacking values with the rounded imply worth. This methodology is commonly utilized when the info has a selected precision or scale requirement, similar to when coping with discrete values or knowledge that ought to be rounded to a sure decimal place. As an example, if a dataset comprises values with two decimal locations, rounding the imply to 2 decimal locations ensures that the imputed values are in keeping with the remainder of the info. This strategy makes the info extra interpretable and aligns the imputation with the precision stage of the dataset. Nevertheless, a draw back is that rounding can result in a lack of precision, particularly in datasets the place fine-grained values are essential for evaluation.
import pandas as pd
import numpy as np
# Pattern dataset with lacking values
knowledge = {'A': [1, 2, np.nan, 4, 5, np.nan, 7],
'B': [10, np.nan, 30, 40, np.nan, 60, 70]}
df = pd.DataFrame(knowledge)
# Rounded Imply Imputation
df['A_rounded_mean'] = df['A'].fillna(spherical(df['A'].imply()))
print("Dataset after Imputation:")
print(df)
7. Fastened Worth Imputation
Fastened worth imputation is a straightforward and versatile approach for dealing with lacking knowledge by changing lacking values with a predetermined worth, chosen primarily based on the context of the dataset. For categorical knowledge, this would possibly contain substituting lacking responses with placeholders like “not answered” or “unknown,” whereas numerical knowledge would possibly use 0 or one other fastened worth that’s logically significant. This strategy ensures consistency and is straightforward to implement, making it appropriate for fast preprocessing. Nevertheless, it could introduce bias if the fastened worth doesn’t replicate the info’s distribution, probably lowering variability and impacting mannequin efficiency. To mitigate these points, it is very important select contextually significant values, doc the imputed values clearly, and analyze the extent of missingness to evaluate the imputation’s affect.
import pandas as pd
# Pattern dataset with lacking values
knowledge = {
'Age': [25, None, 30, None],
'Survey_Response': ['Yes', None, 'No', None]
}
df = pd.DataFrame(knowledge)
# Fastened worth imputation
# For numerical knowledge (e.g., Age), substitute lacking values with a hard and fast quantity, similar to 0
df['Age'] = df['Age'].fillna(0)
# For categorical knowledge (e.g., Survey_Response), substitute lacking values with "Not Answered"
df['Survey_Response'] = df['Survey_Response'].fillna('Not Answered')
print("nDataFrame after Fastened Worth Imputation:")
print(df)
Additionally learn: An Correct Method to Information Imputation
Conclusion
Dealing with lacking knowledge successfully is essential for sustaining the integrity of datasets and making certain the accuracy of analyses and machine studying fashions. Pandas fillna() methodology provides a versatile and environment friendly strategy to knowledge imputation, accommodating a wide range of strategies tailor-made to totally different knowledge sorts and contexts.
From easy strategies like changing lacking values with fastened values or statistical measures (imply, median, mode) to extra subtle strategies like ahead/backward filling and transferring averages, every technique has its strengths and is suited to particular eventualities. By selecting the suitable imputation approach, practitioners can mitigate the affect of lacking knowledge, decrease bias, and protect the dataset’s statistical properties.
Finally, deciding on the correct imputation methodology requires understanding the character of the dataset, the sample of missingness, and the targets of the evaluation. With instruments like fillna(), knowledge scientists and analysts are outfitted to deal with lacking knowledge effectively, enabling strong and dependable ends in their workflows.
If you’re on the lookout for an AI/ML course on-line, then, discover: Licensed AI & ML BlackBelt PlusProgram
Often Requested Questions
Ans. The fillna() methodology in Pandas is used to exchange lacking values (NaN) in a DataFrame or Sequence with a specified worth, methodology, or computation. It permits filling with a hard and fast worth, propagating the earlier or subsequent legitimate worth utilizing strategies like ffill (ahead fill) or bfill (backward fill), or making use of totally different methods column-wise with dictionaries. This operate is important for dealing with lacking knowledge and making certain datasets are full for evaluation.
Ans. The first distinction between dropna() and fillna() in Pandas lies in how they deal with lacking values (NaN). dropna() removes rows or columns containing lacking values, successfully lowering the scale of the DataFrame or Sequence. In distinction, fillna() replaces lacking values with specified knowledge, similar to a hard and fast worth, a computed worth, or by propagating close by values, with out altering the DataFrame’s dimensions. Use dropna() whenever you need to exclude incomplete knowledge and fillna() whenever you need to retain the dataset’s construction by filling gaps.
Ans. In Pandas, each fillna()
and interpolate()
deal with lacking values however differ in strategy. fillna()
replaces NaNs with specified values (e.g., constants, imply, median) or propagates present values (e.g., ffill
, bfill
). In distinction, interpolate()
estimates lacking values utilizing surrounding knowledge, making it best for numerical knowledge with logical tendencies. Basically, fillna()
applies express replacements, whereas interpolate()
infers values primarily based on knowledge patterns.