Introduction
Think about you’re in the midst of an intense dialog, and the proper response slips your thoughts simply once you want it most. Now, think about should you had a device that might adapt to each twist and switch of the dialogue, providing simply the fitting phrases on the proper time. That’s the facility of adaptive prompting, and it’s not only a dream—it’s a cutting-edge method remodeling how we work together with AI. On this article, we’ll discover how one can harness the capabilities of adaptive prompting utilizing DSPy, diving into real-world functions like sentiment evaluation. Whether or not you’re a knowledge scientist trying to refine your fashions or simply inquisitive about the way forward for AI, this information will present you why adaptive prompting is the following huge factor it’s essential to find out about.
Studying Goals
- Perceive the idea of adaptive prompting and its advantages in creating more practical and context-sensitive interactions.
- Get acquainted with dynamic programming ideas and the way DSPy simplifies their software.
- Observe a sensible information to utilizing DSPY to construct adaptive prompting methods.
- See adaptive prompting in motion by means of a case examine, showcasing its impression on immediate effectiveness.
This text was printed as part of the Knowledge Science Blogathon.
What’s Adaptive Prompting?
Adaptive prompting is a dynamic method to interacting with fashions that contain adjusting prompts primarily based on the responses acquired or the context of the interplay. In contrast to conventional static prompting, the place the immediate stays fastened whatever the mannequin’s output or the dialog’s progress, adaptive prompting evolves in actual time to optimize the interplay.
In adaptive prompting, prompts are designed to be versatile and responsive. They modify primarily based on the suggestions from the mannequin or person, aiming to elicit extra correct, related, or detailed responses. This dynamic adjustment can improve the effectiveness of interactions by tailoring prompts to raised match the present context or the precise wants of the duty.
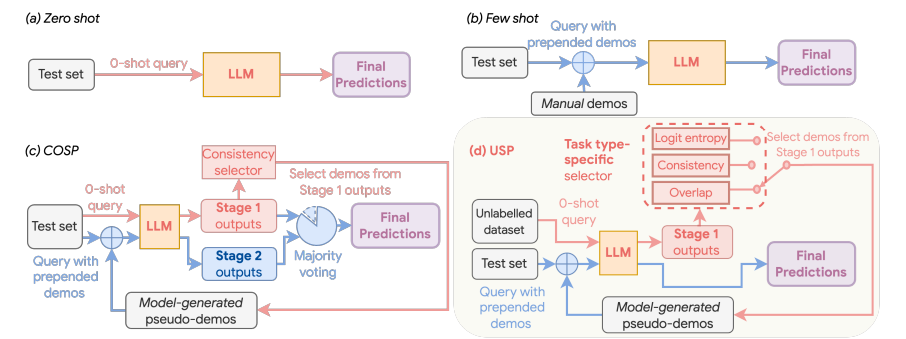
Advantages of Adaptive Prompting
- Enhanced Relevance: By adapting prompts primarily based on mannequin responses, you possibly can improve the relevance and precision of the output.
- Improved Consumer Engagement: Dynamic prompts could make interactions extra participating and customized, resulting in a greater person expertise.
- Higher Dealing with of Ambiguity: Adaptive prompting will help make clear ambiguous responses by refining the prompts to solicit extra particular info.
Fundamental Adaptive Prompting Utilizing Language Mannequin
Beneath is a Python code snippet demonstrating a primary adaptive prompting system utilizing a language mannequin. The instance reveals how one can regulate prompts primarily based on a mannequin’s response:
from transformers import GPT3Tokenizer, GPT3Model
# Initialize the mannequin and tokenizer
model_name = "gpt-3.5-turbo"
tokenizer = GPT3Tokenizer.from_pretrained(model_name)
mannequin = GPT3Model.from_pretrained(model_name)
def generate_response(immediate):
inputs = tokenizer(immediate, return_tensors="pt")
outputs = mannequin(**inputs)
return tokenizer.decode(outputs.logits.argmax(dim=-1))
def adaptive_prompting(initial_prompt, model_response):
# Modify the immediate primarily based on the mannequin's response
if "I do not know" in model_response:
new_prompt = f"{initial_prompt} Are you able to present extra particulars?"
else:
new_prompt = f"{initial_prompt} That is attention-grabbing. Are you able to develop on
that?"
return new_prompt
# Instance interplay
initial_prompt = "Inform me concerning the significance of adaptive prompting."
response = generate_response(initial_prompt)
print("Mannequin Response:", response)
# Adaptive prompting
new_prompt = adaptive_prompting(initial_prompt, response)
print("New Immediate:", new_prompt)
new_response = generate_response(new_prompt)
print("New Mannequin Response:", new_response)
Within the above code snippet, we use a language mannequin (GPT-3.5-turbo) to reveal how prompts may be dynamically adjusted primarily based on the mannequin’s responses. The code initializes the mannequin and tokenizer, then defines a operate, generate_response, that takes a immediate, processes it with the mannequin, and returns the generated textual content. One other operate, adaptive_prompting, modifies the preliminary immediate relying on the mannequin’s response. If the response incorporates phrases indicating uncertainty, akin to “I don’t know,” the immediate is refined to request extra particulars. In any other case, the immediate is adjusted to encourage additional elaboration.
For instance, if the preliminary immediate is “Inform me concerning the significance of adaptive prompting,” and the mannequin responds with an unsure reply, the adaptive immediate may be adjusted to “Are you able to present extra particulars?” The mannequin would then generate a brand new response primarily based on this refined immediate. The anticipated output can be an up to date immediate that goals to elicit a extra informative and particular reply, adopted by a extra detailed response from the mannequin.
Use Instances of Adaptive Prompting
Adaptive prompting may be notably helpful in varied situations, together with:
- Dialogue Techniques: Adaptive prompting in dialogue methods helps tailor the dialog stream primarily based on person responses. This may be achieved utilizing dynamic programming to handle state transitions and immediate changes.
- Query-Answering: Adaptive prompting can refine queries primarily based on preliminary responses to acquire extra detailed solutions.
- Interactive Storytelling: Adaptive prompting adjusts the narrative primarily based on person decisions, enhancing the interactive storytelling expertise.
- Knowledge Assortment and Annotation: Adaptive prompting can refine knowledge assortment queries primarily based on preliminary responses to assemble extra exact info.
By leveraging adaptive prompting, functions can change into more practical at participating customers, dealing with advanced interactions, and offering helpful insights. Adaptive prompting’s flexibility and responsiveness make it a strong device for bettering the standard and relevance of mannequin interactions throughout varied domains.
Constructing Adaptive Prompting Methods with DSPy
Creating adaptive prompting methods includes leveraging dynamic programming (DP) ideas to regulate prompts primarily based on mannequin interactions and suggestions. The DSPy library simplifies this course of by offering a structured method to managing states, actions, and transitions. Beneath is a step-by-step information on establishing an adaptive prompting technique utilizing DSPy.
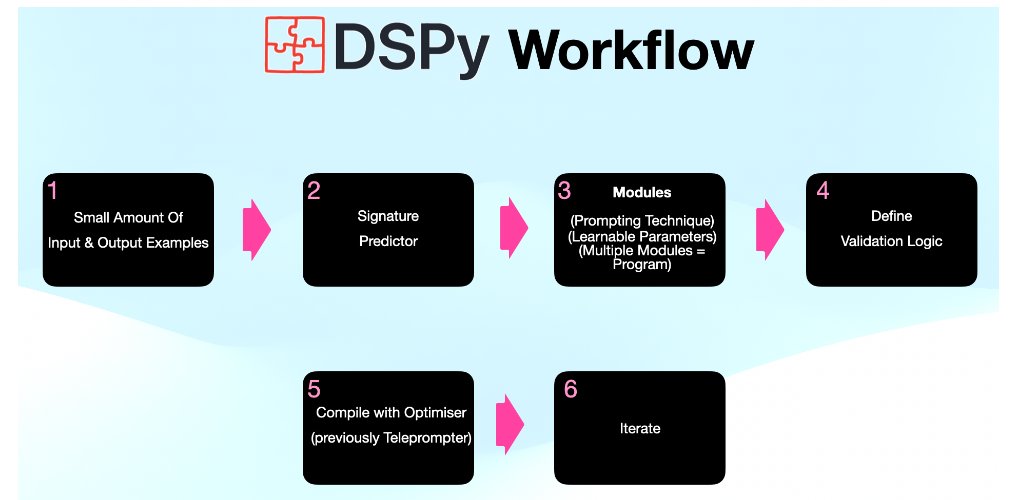
Step-by-Step Information to Constructing Adaptive Prompting Methods
Allow us to now look into the step-by-step information to constructing Adaptive prompting methods.
- Outline the Downside Scope: Decide the precise adaptive prompting situation you might be addressing. For instance, you may be designing a system that adjusts prompts in a dialogue system primarily based on person responses.
- Establish States and Actions: Outline the states representing completely different situations or situations in your prompting system. Establish actions that modify these states primarily based on person suggestions or mannequin responses.
- Create Recurrence Relations: Set up recurrence relations that dictate how the states transition from one to a different primarily based on the actions taken. These relations information how prompts are adjusted adaptively.
- Implement the Technique Utilizing DSPy: Make the most of the DSPy library to mannequin the outlined states, actions, and recurrence relations and implement the adaptive prompting technique.
Defining States and Actions
In adaptive prompting, states usually embrace the present immediate and person suggestions, whereas actions contain modifying the immediate primarily based on the suggestions.
Instance:
- States:
- State_Prompt: Represents the present immediate.
- State_Feedback: Represents person suggestions or mannequin responses.
- Actions:
- Action_Adjust_Prompt: Adjusts the immediate primarily based on suggestions.
Code Instance: Defining States and Actions
from dspy import State, Motion
class AdaptivePromptingDP:
def __init__(self):
# Outline states
self.states = {
'preliminary': State('initial_prompt'),
'suggestions': State('suggestions')
}
# Outline actions
self.actions = {
'adjust_prompt': Motion(self.adjust_prompt)
}
def adjust_prompt(self, state, suggestions):
# Logic to regulate the immediate primarily based on suggestions
if "unclear" in suggestions:
return "Are you able to make clear your response?"
else:
return "Thanks on your suggestions."
# Initialize adaptive prompting
adaptive_dp = AdaptivePromptingDP()
Making a Recurrence Relation
Recurrence relations information how states transition primarily based on actions. Adaptive prompting includes defining how prompts change primarily based on person suggestions.
Instance: The recurrence relation would possibly specify that if the person supplies unclear suggestions, the system ought to transition to a state the place it asks for clarification.
Code Instance: Making a Recurrence Relation
from dspy import Transition
class AdaptivePromptingDP:
def __init__(self):
# Outline states
self.states = {
'preliminary': State('initial_prompt'),
'clarification': State('clarification_prompt')
}
# Outline actions
self.actions = {
'adjust_prompt': Motion(self.adjust_prompt)
}
# Outline transitions
self.transitions = [
Transition(self.states['initial'], self.states['clarification'],
self.actions['adjust_prompt'])
]
def adjust_prompt(self, state, suggestions):
if "unclear" in suggestions:
return self.states['clarification']
else:
return self.states['initial']
Implementing with DSPy
The ultimate step is to implement the outlined technique utilizing DSPy. This includes establishing the states, actions, and transitions inside DSPy’s framework and working the algorithm to regulate prompts adaptively.
Code Instance: Full Implementation
from dspy import State, Motion, Transition, DPAlgorithm
class AdaptivePromptingDP(DPAlgorithm):
def __init__(self):
tremendous().__init__()
# Outline states
self.states = {
'preliminary': State('initial_prompt'),
'clarification': State('clarification_prompt')
}
# Outline actions
self.actions = {
'adjust_prompt': Motion(self.adjust_prompt)
}
# Outline transitions
self.transitions = [
Transition(self.states['initial'], self.states['clarification'],
self.actions['adjust_prompt'])
]
def adjust_prompt(self, state, suggestions):
if "unclear" in suggestions:
return self.states['clarification']
else:
return self.states['initial']
def compute(self, initial_state, suggestions):
# Compute the tailored immediate primarily based on suggestions
return self.run(initial_state, suggestions)
# Instance utilization
adaptive_dp = AdaptivePromptingDP()
initial_state = adaptive_dp.states['initial']
suggestions = "I do not perceive this."
adapted_prompt = adaptive_dp.compute(initial_state, suggestions)
print("Tailored Immediate:", adapted_prompt)
Code Rationalization:
- State and Motion Definitions: States characterize the present immediate and any adjustments. Actions outline how one can regulate the immediate primarily based on suggestions.
- Transitions: Transitions dictate how the state adjustments primarily based on the actions.
- compute Technique: This technique processes suggestions and computes the tailored immediate utilizing the DP algorithm outlined with dspy.
Anticipated Output:
Given the preliminary state and suggestions like “I don’t perceive this,” the system would transition to the ‘clarification_prompt’ state and output a immediate asking for extra particulars, akin to “Are you able to make clear your response?”
Case Examine: Adaptive Prompting in Sentiment Evaluation
Understanding the nuances of person opinions may be difficult in sentiment evaluation, particularly when coping with ambiguous or obscure suggestions. Adaptive prompting can considerably improve this course of by dynamically adjusting the prompts primarily based on person responses to elicit extra detailed and exact opinions.
Situation
Think about a sentiment evaluation system designed to gauge person opinions a couple of new product. Initially, the system asks a common query like, “What do you concentrate on our new product?” If the person’s response is unclear or lacks element, the system ought to adaptively refine the immediate to assemble extra particular suggestions, akin to “Are you able to present extra particulars about what you appreciated or disliked?
This adaptive method ensures that the suggestions collected is extra informative and actionable, bettering sentiment evaluation’s general accuracy and usefulness.
Implementation
To implement adaptive prompting in sentiment evaluation utilizing DSPy, observe these steps:
- Outline States and Actions:
- States: Characterize completely different levels of the prompting course of, akin to preliminary immediate, clarification wanted, and detailed suggestions.
- Actions: Outline how one can regulate the immediate primarily based on the suggestions acquired.
- Create Recurrence Relations: Arrange transitions between states primarily based on person responses to information the prompting course of adaptively.
- Implement with DSPy: Use DSPy to outline the states, actions and transitions after which run the dynamic programming algorithm to adaptively regulate the prompts.
Code Instance: Setting Up the Dynamic Program
Allow us to now look into the steps under for establishing dynamic program.
Step1: Importing Required Libraries
Step one includes importing the required libraries. The dspy
library is used for managing states, actions, and transitions, whereas matplotlib.pyplot
is utilized for visualizing the outcomes.
from dspy import State, Motion, Transition, DPAlgorithm
import matplotlib.pyplot as plt
Step2: Defining the SentimentAnalysisPrompting Class
The SentimentAnalysisPrompting
class inherits from DPAlgorithm
, establishing the dynamic programming construction. It initializes states, actions, and transitions, which characterize completely different levels of the adaptive prompting course of.
class SentimentAnalysisPrompting(DPAlgorithm):
def __init__(self):
tremendous().__init__()
# Outline states
self.states = {
'preliminary': State('initial_prompt'),
'clarification': State('clarification_prompt'),
'detailed_feedback': State('detailed_feedback_prompt')
}
# Outline actions
self.actions = {
'request_clarification': Motion(self.request_clarification),
'request_detailed_feedback': Motion(self.request_detailed_feedback)
}
# Outline transitions
self.transitions = [
Transition(self.states['initial'], self.states['clarification'],
self.actions['request_clarification']),
Transition(self.states['clarification'], self.states
['detailed_feedback'], self.actions['request_detailed_feedback'])
]
Step3: Request Clarification Motion
This technique defines what occurs when suggestions is unclear or too temporary. If the suggestions is obscure, the system transitions to a clarification immediate, asking for extra info.
def request_clarification(self, state, suggestions):
# Transition to clarification immediate if suggestions is unclear or brief
if "not clear" in suggestions or len(suggestions.break up()) < 5:
return self.states['clarification']
return self.states['initial']
Step4: Request Detailed Suggestions Motion
On this technique, if the suggestions suggests the necessity for extra particulars, the system transitions to a immediate particularly asking for detailed suggestions.
def request_detailed_feedback(self, state, suggestions):
# Transition to detailed suggestions immediate if suggestions signifies a necessity
# for extra particulars
if "particulars" in suggestions:
return self.states['detailed_feedback']
return self.states['initial']
Step5: Compute Technique
The compute
technique is answerable for working the dynamic programming algorithm. It determines the following state and immediate primarily based on the preliminary state and the given suggestions.
def compute(self, initial_state, suggestions):
# Compute the following immediate primarily based on the present state and suggestions
return self.run(initial_state, suggestions)
Step6: Initializing and Processing Suggestions
Right here, the SentimentAnalysisPrompting
class is initialized, and a set of pattern suggestions is processed. The system computes the tailored immediate primarily based on every suggestions entry.
# Initialize sentiment evaluation prompting
sa_prompting = SentimentAnalysisPrompting()
initial_state = sa_prompting.states['initial']
# Pattern feedbacks for testing
feedbacks = [
"I don't like it.",
"The product is okay but not great.",
"Can you tell me more about the features?",
"I need more information to provide a detailed review."
]
# Course of feedbacks and acquire outcomes
outcomes = []
for suggestions in feedbacks:
adapted_prompt = sa_prompting.compute(initial_state, suggestions)
outcomes.append({
'Suggestions': suggestions,
'Tailored Immediate': adapted_prompt.identify
})
Step7: Visualizing the Outcomes
Lastly, the outcomes are visualized utilizing a bar chart. The chart shows the variety of responses categorized by the kind of immediate: Preliminary, Clarification, and Detailed Suggestions.
# Print outcomes
for end in outcomes:
print(f"Suggestions: {consequence['Feedback']}nAdapted Immediate: {consequence['Adapted Prompt']}n")
# Instance knowledge for visualization
# Rely of responses at every immediate stage
prompt_names = ['Initial', 'Clarification', 'Detailed Feedback']
counts = [sum(1 for r in results if r['Adapted Prompt'] == identify) for identify in prompt_names]
# Plotting
plt.bar(prompt_names, counts, colour=['blue', 'orange', 'green'])
plt.xlabel('Immediate Sort')
plt.ylabel('Variety of Responses')
plt.title('Variety of Responses per Immediate Sort')
plt.present()
Anticipated Output
- Suggestions and Tailored Immediate: The outcomes for every suggestions merchandise displaying which immediate kind was chosen.
- Visualization: A bar chart (under) illustrating what number of responses fell into every immediate class.
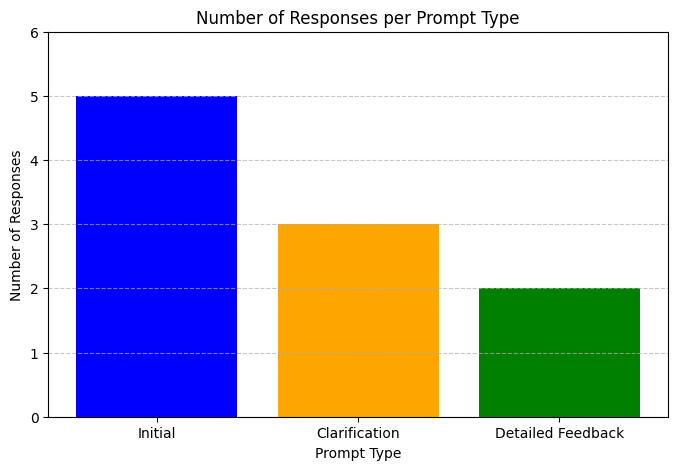
The bar chart reveals that the ‘Preliminary’ immediate kind dominates in utilization and effectiveness, garnering the best variety of responses. The system occasionally requires clarification prompts, and requests for ‘Detailed Suggestions’ are even much less widespread. This implies that preliminary prompts are essential for person engagement, whereas detailed suggestions is much less crucial. Adjusting focus and optimization primarily based on these insights can improve prompting methods.
Advantages of Utilizing DSPy for Adaptive Prompting
DSPy provides a number of compelling advantages for implementing adaptive prompting methods. By leveraging DSPy’s capabilities, you possibly can considerably improve your adaptive prompting options’ effectivity, flexibility, and scalability.
- Effectivity: DSPy streamlines the event of adaptive methods by offering high-level abstractions. This simplifies the method, reduces implementation time, and minimizes the chance of errors, permitting you to focus extra on technique design moderately than low-level particulars.
- Flexibility: With DSPy, you possibly can shortly experiment with and regulate completely different prompting methods. Its versatile framework helps speedy iteration, enabling you to refine prompts primarily based on real-time suggestions and evolving necessities.
- Scalability: DSPy’s modular design is constructed to deal with large-scale and complicated NLP duties. As your knowledge and complexity develop, DSPy scales along with your wants, making certain that adaptive prompting stays efficient and strong throughout varied situations.
Challenges in Implementing Adaptive Prompting
Regardless of its benefits, utilizing DSPy for adaptive prompting comes with its challenges. It’s vital to concentrate on these potential points and handle them to optimize your implementation.
- Complexity Administration: Managing quite a few states and transitions may be difficult resulting from their elevated complexity. Efficient complexity administration requires retaining your state mannequin easy and making certain thorough documentation to facilitate debugging and upkeep.
- Efficiency Overhead: Dynamic programming introduces computational overhead that will impression efficiency. To mitigate this, optimize your state and transition definitions and conduct efficiency profiling to establish and resolve bottlenecks.
- Consumer Expertise: Overly adaptive prompting can negatively have an effect on person expertise if prompts change into too frequent or intrusive. Hanging a stability between adaptiveness and stability is essential to make sure that prompts are useful and don’t disrupt the person expertise.
Conclusion
We have now explored the mixing of adaptive prompting with the DSPy library to boost NLP functions. We mentioned how adaptive prompting improves interactions by dynamically adjusting prompts primarily based on person suggestions or mannequin outputs. By leveraging DSPy’s dynamic programming framework, we demonstrated how one can implement these methods effectively and flexibly.
Sensible examples, akin to sentiment evaluation, highlighted how DSPy simplifies advanced state administration and transitions. Whereas DSPy provides advantages like elevated effectivity and scalability, it presents challenges like complexity administration and potential efficiency overhead. Embracing DSPy in your initiatives can result in more practical and responsive NLP methods.
Key Takeaways
- Adaptive prompting dynamically adjusts prompts primarily based on person suggestions to enhance interactions.
- DSPy simplifies the implementation of adaptive prompting with dynamic programming abstractions.
- Advantages of utilizing DSPy embrace environment friendly growth, flexibility in experimentation, and scalability.
- Challenges embrace managing complexity and addressing potential efficiency overhead.
Steadily Requested Questions
A. Adaptive prompting includes dynamically adjusting prompts primarily based on suggestions or mannequin outputs to enhance person interactions and accuracy. It is vital as a result of it permits for extra customized and efficient responses, enhancing person engagement and satisfaction in NLP functions.
A. DSPy supplies a dynamic programming framework that simplifies the administration of states, actions, and transitions in adaptive prompting. It provides high-level abstractions to streamline the implementation course of, making experimenting with and refining prompting methods simpler.
A. The primary advantages embrace elevated growth effectivity, flexibility for speedy experimentation with completely different methods, and scalability to deal with advanced NLP duties. DSPy helps streamline the adaptive prompting course of and improves general system efficiency.
A. Challenges embrace managing the complexity of quite a few states and transitions, potential efficiency overhead, and balancing adaptiveness with person expertise. Efficient complexity administration and efficiency optimization are important to handle these challenges.
A. To get began with DSPy, discover its documentation and tutorials to know its options and capabilities. Implement primary dynamic programming ideas with DSPy, and progressively combine it into your adaptive prompting methods. Experiment with completely different situations and use circumstances to refine your method and obtain the specified outcomes.
The media proven on this article will not be owned by Analytics Vidhya and is used on the Creator’s discretion.