Creating publication-ready figures and tables is crucial for educational analysis and knowledge presentation. Python, with its strong ecosystem of libraries, gives a variety of instruments that can assist you generate high-quality, aesthetically pleasing, and customizable visuals to your analysis papers.
On this article, we’ll discover learn how to use Python to generate publication-ready figures and tables. We’ll cowl well-liked libraries resembling Matplotlib, Seaborn, Plotly, and Pandas for creating figures, and learn how to use Pandas and Matplotlib for formatting tables. We’ll additionally focus on necessary design rules and suggestions for optimizing these parts for publication.
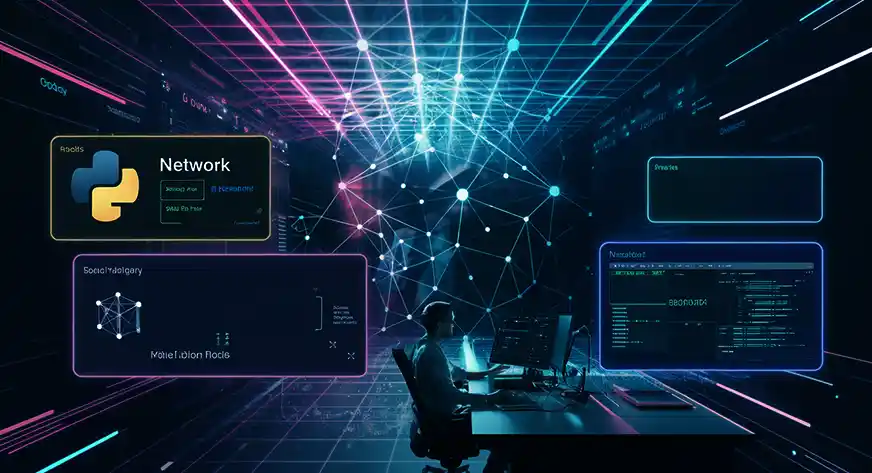
Overview of the publication-ready Figures and Tables Libraries
1. Matplotlib
Matplotlib is certainly one of Python’s most generally used knowledge visualisation libraries. It gives in depth management over each side of a determine, from its dimension and structure to its colours and fonts. Researchers can customise their plots to go well with the necessities of their publication, guaranteeing that the visible parts are constant and clear.
- Key Options:
- Effective-grained management over plot parts.
- Intensive help for 2D plotting (line, scatter, bar charts, and so on.).
- Excessive flexibility for styling plots (titles, labels, axis ticks).
- Can export figures in publication-quality codecs (e.g., PDF, PNG, SVG).
2. Seaborn
Seaborn builds on prime of Matplotlib and gives a higher-level interface for creating visually engaging and informative statistical graphics. It simplifies the method of making complicated visualizations like heatmaps, violin plots, and regression plots, whereas additionally mechanically dealing with aesthetic parts like shade palettes and axis labels.
- Key Options:
- Predefined themes and shade palettes that are perfect for publication-ready plots.
- Excessive-level capabilities for statistical plots (e.g., boxplots, pair plots, and categorical plots).
- Seamless integration with Pandas knowledge constructions.
3. Plotly
Plotly is an interactive visualization library that excels in creating extremely interactive, web-based plots. Though it’s mostly used for dashboards and internet apps, Plotly’s export choices enable for high-quality, static visualizations appropriate for publications. Plotly helps all kinds of chart varieties, together with scatter plots, choropleth maps, and 3D plots.
- Key Options:
- Interactive visualizations (hover, zoom, and click on functionalities).
- Publication-quality static exports (e.g., PNG, SVG, PDF).
- Big selection of chart varieties (e.g., choropleth maps, community graphs).
- Simple customization of plot parts.
4. Pandas
Whereas Pandas is primarily recognized for its knowledge manipulation capabilities, it additionally gives strong performance for creating easy tables and plots. Pandas integrates seamlessly with Matplotlib and Seaborn, enabling straightforward conversion of DataFrames into graphical plots and styled tables. You’ll be able to export Pandas tables to HTML, LaTeX, or Excel codecs, which is especially helpful when making ready tables for educational papers.
- Key Options:
- Constructed-in plotting capabilities for fast visualizations from DataFrames.
- Potential to format tables for show (e.g., setting column widths, textual content alignment, and borders).
- Export choices for numerous codecs (HTML, LaTeX, Excel).
Creating Publication-Prepared Figures
Listed below are the rules and libraries we’ll use:
Key Libraries
- Matplotlib: A flexible library for static, animated, and interactive plots. It permits fine-grained management over nearly each side of the determine.
- Seaborn: Constructed on prime of Matplotlib, Seaborn gives a high-level interface for drawing engaging statistical graphics.
- Plotly: For interactive visualizations, although additionally helps static exports that can be utilized in publications.
Common Pointers for Figures:
- Decision: Guarantee your figures are saved in a excessive decision (at the very least 300 DPI for print high quality).
- Coloration: Use shade palettes which can be printer-friendly and appropriate for color-blind viewers.
- Legibility: Use giant fonts for axis labels, titles, and legends. Figures needs to be simply readable even at diminished sizes.
- Consistency: Hold kinds constant throughout all figures within the paper (identical font, gridlines, shade schemes, and so on.).
- Clear Labels: Use significant axis labels and legends, guaranteeing that every determine is self-explanatory.
Let’s Create a Determine with Matplotlib
import matplotlib.pyplot as plt
import numpy as np
# Create knowledge
x = np.linspace(0, 10, 100)
y = np.sin(x)
# Create a determine with publication-quality aesthetics
plt.determine(figsize=(6, 4), dpi=300) # Set determine dimension and backbone
plt.plot(x, y, label="Sine Wave", shade="b", linewidth=2)
# Including labels and title
plt.xlabel("X-axis label", fontsize=14)
plt.ylabel("Y-axis label", fontsize=14)
plt.title("Sine Wave Instance", fontsize=16)
# Including grid and legend
plt.grid(True, which="each", linestyle="--", linewidth=0.5)
plt.legend(fontsize=12)
# Saving the determine as a high-resolution PNG
plt.savefig("sine_wave_figure.png", dpi=300, bbox_inches="tight")
plt.present()
Output
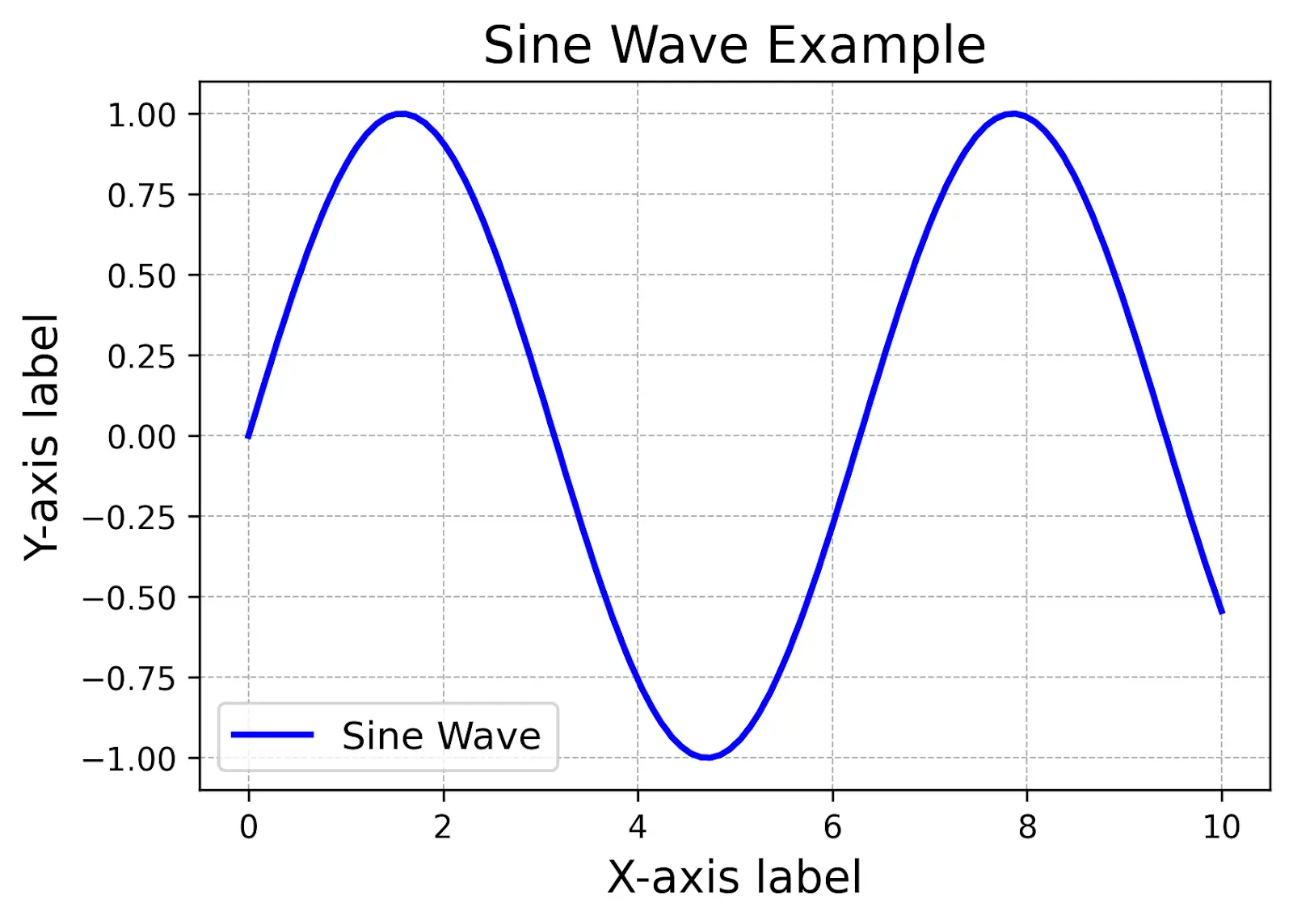
Clarification
- The figsize parameter units the determine dimensions (6×4 inches right here).
- The dpi=300 ensures the determine is excessive decision.
- bbox_inches=’tight’ removes further whitespace when saving the determine.
Additionally learn: Introduction to Matplotlib utilizing Python for Rookies
Superior Customization with Seaborn
Seaborn simplifies the creation of complicated statistical plots. It integrates with Matplotlib and might shortly generate high-quality plots with engaging default kinds.
Instance: A Publication-Prepared Heatmap with Seaborn
import seaborn as sns
import numpy as np
# Create a random correlation matrix
knowledge = np.random.rand(10, 10)
# Create a heatmap with Seaborn
plt.determine(figsize=(8, 6))
sns.heatmap(knowledge, annot=True, cmap="coolwarm", fmt=".2f", linewidths=0.5)
# Including labels and title
plt.title("Correlation Heatmap", fontsize=16)
plt.xlabel("X-axis label", fontsize=14)
plt.ylabel("Y-axis label", fontsize=14)
# Save determine
plt.savefig("heatmap.png", dpi=300, bbox_inches="tight")
plt.present()
Output
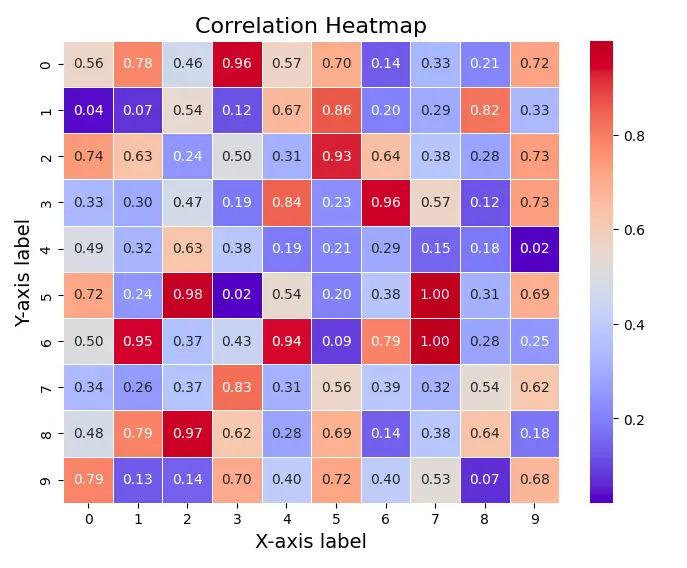
Right here, Seaborn handles a lot of the styling mechanically (like the color map and annotations), permitting you to give attention to the info.
Additionally learn: The Final Information to Pandas For Knowledge Science!
Creating Interactive Figures with Plotly
In case your publication permits for interactive elements (e.g., supplementary supplies), Plotly is a robust device. It lets you generate interactive plots that may be embedded in internet pages or exported as static photos.
Instance: Interactive Scatter Plot with Plotly
!pip set up --upgrade kaleido
import plotly.categorical as px
import pandas as pd
import numpy as np
# Pattern knowledge
df = pd.DataFrame({
"X": np.random.randn(100),
"Y": np.random.randn(100),
"Class": np.random.selection(['A', 'B', 'C'], dimension=100)
})
# Create an interactive scatter plot
fig = px.scatter(df, x="X", y="Y", shade="Class", title="Interactive Scatter Plot")
# Save as HTML file (for interactive use) or PNG (for publication)
fig.write_html("scatter_plot.html")
fig.write_image("scatter_plot.png", width=800, peak=600, scale=2)
Output
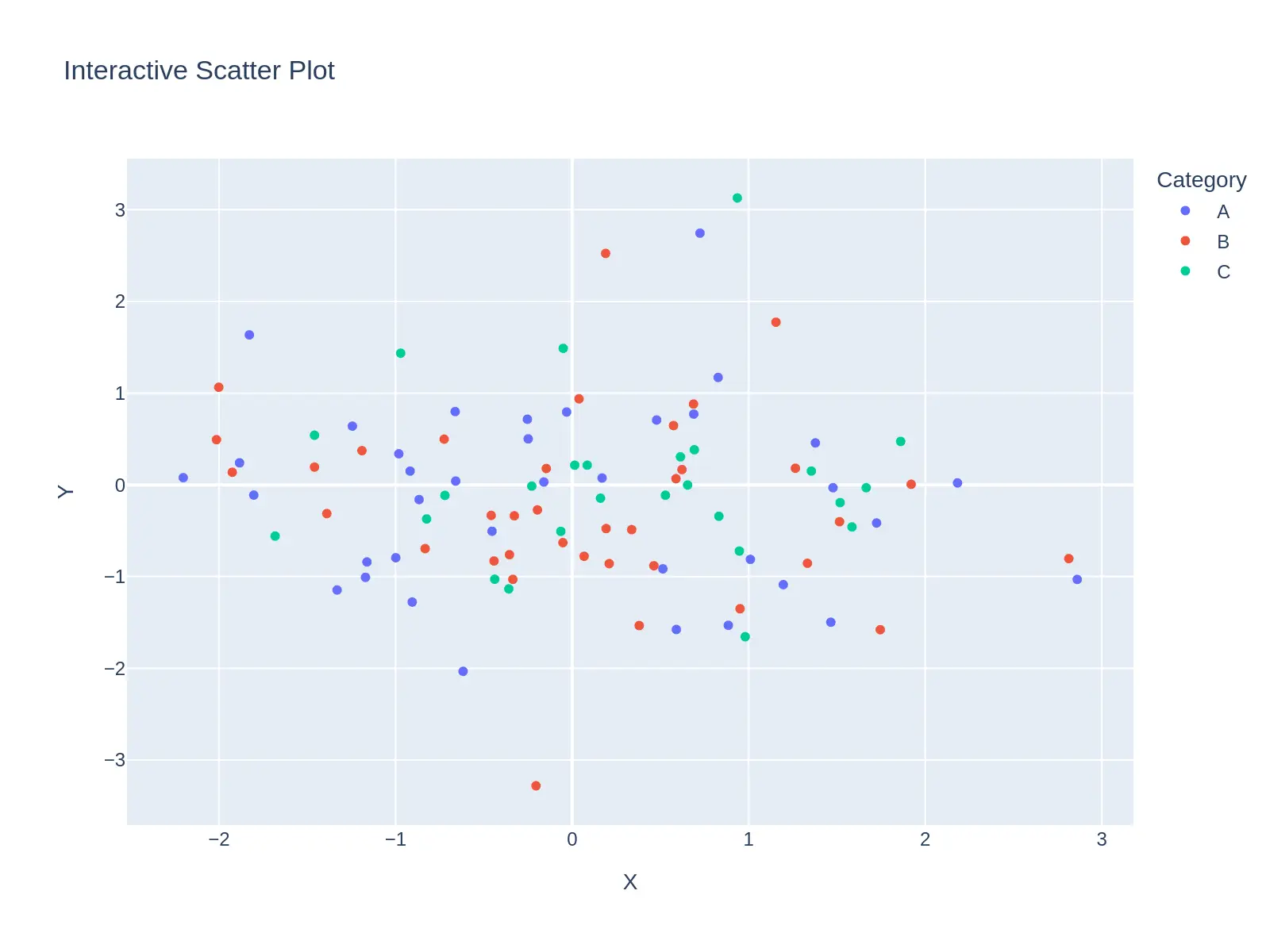
Additionally learn: Information to Create Interactive Plots with Plotly Python
Tables for Publications
Tables are one other essential a part of scientific papers. Python’s Pandas library is broadly used for creating and formatting knowledge tables. For publication, tables needs to be clear, well-organized, and straightforward to learn.
Instance: Making a Desk with Pandas
import pandas as pd
import plotly.categorical as px
from IPython.core.show import HTML
# Create a DataFrame with inhabitants, continent, and nation flags
knowledge = {
'Nation': ['China', 'India', 'USA', 'Indonesia', 'Pakistan', 'Brazil', 'Nigeria', 'Bangladesh', 'Russia', 'Mexico'],
'Inhabitants (thousands and thousands)': [1444216, 1393409, 332915, 276361, 225199, 213993, 211400, 166303, 145912, 130262],
'Continent': ['Asia', 'Asia', 'North America', 'Asia', 'Asia', 'South America', 'Africa', 'Asia', 'Europe', 'North America'],
'Flag': [
'https://upload.wikimedia.org/wikipedia/commons/0/0d/Flag_of_China.svg',
'https://upload.wikimedia.org/wikipedia/commons/4/41/Flag_of_India.svg',
'https://upload.wikimedia.org/wikipedia/commons/a/a4/Flag_of_the_United_States.svg',
'https://upload.wikimedia.org/wikipedia/commons/9/9d/Flag_of_Indonesia.svg',
'https://upload.wikimedia.org/wikipedia/commons/3/3f/Flag_of_Pakistan.svg',
'https://upload.wikimedia.org/wikipedia/commons/0/05/Flag_of_Brazil.svg',
'https://upload.wikimedia.org/wikipedia/commons/7/79/Flag_of_Nigeria.svg',
'https://upload.wikimedia.org/wikipedia/commons/f/f9/Flag_of_Bangladesh.svg',
'https://upload.wikimedia.org/wikipedia/commons/f/f3/Flag_of_Russia.svg',
'https://upload.wikimedia.org/wikipedia/commons/f/fc/Flag_of_Mexico.svg'
]
}
# Create DataFrame
df = pd.DataFrame(knowledge)
# Manually add flags as HTML img tags (with customized width and peak)
df['Flag'] = df['Flag'].apply(lambda x: f'<img src="https://www.analyticsvidhya.com/weblog/2024/12/figures-and-tables-with-python/{x}" width="30" peak="20">')
# Show the DataFrame utilizing HTML rendering (to point out flags accurately)
html_table = df.to_html(escape=False) # Escape is False to permit HTML rendering
# Show the desk with flags within the pocket book
show(HTML(html_table))
# Map visualization utilizing Plotly
fig = px.choropleth(df,
places="Nation",
locationmode="nation names",
shade="Inhabitants (thousands and thousands)",
hover_name="Nation",
hover_data=["Continent", "Population (millions)"],
color_continuous_scale=px.colours.sequential.Plasma,
title="Prime 10 International locations by Inhabitants")
# Non-obligatory: To obtain the HTML desk
from google.colab import information
df.to_html("population_table_with_flags.html", escape=False)
information.obtain("population_table_with_flags.html")
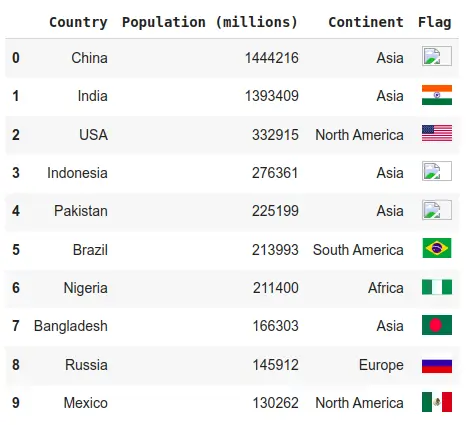
Finest Practices for Tables in Publications
- Use Clear Headers: Column headers needs to be descriptive, and keep away from overly technical jargon.
- Consistency: Be certain that all numbers are introduced in a constant format (e.g., decimal locations).
- Alignment: Align numbers by decimal factors and textual content by the left.
- Footnotes: Use footnotes for extra explanations as a substitute of cluttering the desk.
Conclusion
Python gives a robust suite of instruments for producing publication-ready figures and tables. Whether or not you’re creating static plots with Matplotlib, statistical plots with Seaborn, or interactive visualizations with Plotly, Python has you coated. For tables, Pandas lets you simply format and export knowledge in numerous codecs for publication.
Key Takeaways
- Select applicable libraries for the duty (Matplotlib/Seaborn for static, Plotly for interactive).
- Prioritize readability and consistency in your designs.
- Export at excessive decision (300 DPI) and use readable font sizes.
- For tables, guarantee clear headers, constant formatting, and clear design.
By following the following tips and using Python’s in depth libraries, you may create professional-quality figures and tables that can improve the readability and influence of your analysis paper.
Incessantly Requested Questions
Ans. Publication-ready figures and tables are graphics and knowledge tables formatted to fulfill the requirements of educational journals and publications. They have to be clear, high-quality, and visually interesting whereas adhering to model pointers resembling font dimension, decision, and structure.
Ans. Python gives highly effective libraries like Matplotlib, Seaborn, and Plotly to create customizable, high-quality visualizations. These instruments enable for exact management over determine design, together with shade schemes, labels, and axis formatting, making it simpler to provide publication-ready figures and tables with Python.
Ans. Sure, Python permits for the creation of high-resolution figures by specifying the DPI (dots per inch) when saving photos. Utilizing libraries like Matplotlib, you may export figures in numerous codecs (e.g., PNG, SVG, PDF) whereas guaranteeing they meet publication requirements.
These FAQs present a fast overview of how Python can be utilized to create “Publication-Prepared Figures and Tables with Python,” which is crucial for researchers and knowledge scientists aiming to publish high-quality, visually interesting analysis outputs.
Ans. A determine is taken into account publication-ready when it’s visually clear, aesthetically pleasing, and adheres to particular journal pointers. This consists of selecting applicable shade schemes, guaranteeing excessive decision (300 DPI or larger), correct axis labeling, and together with legends, titles, and annotations.