Whereas engaged on Agentic AI, builders typically discover themselves navigating the trade-offs between velocity, flexibility, and useful resource effectivity. I’ve been exploring the Agentic AI framework and got here throughout Agno (earlier it was Phi-data). Agno is a light-weight framework for constructing multi-modal Brokers. They’re claiming to be ~10,000x sooner than LangGraph and ~50x much less reminiscence than LangGraph. Sound intriguing proper?
Agno and LangGraph—provide very totally different experiences. After going hands-on with Agno and evaluating its efficiency and structure to LangGraph, right here’s a breakdown of how they differ, the place every one shines, and what Agno brings to the desk.
TL;DR
- Constructing TriSage and Advertising and marketing Analyst Agent
- Use Agno if you’d like velocity, low reminiscence use, multimodal capabilities, and adaptability with fashions/instruments.
- Use LangGraph for those who desire flow-based logic, or structured execution paths, or are already tied into LangChain’s ecosystem.
The Agno: What it Affords?
Agno is designed with a laser deal with efficiency and minimalism. At its core, Agno is an open-source, model-agnostic agent framework constructed for multimodal duties—that means it handles textual content, photos, audio, and video natively. What makes it distinctive is how gentle and quick it’s below the hood, even when orchestrating massive numbers of brokers with added complexity like reminiscence, instruments, and vector shops.
Key strengths that stand out:
- Blazing Instantiation Pace: Agent creation in Agno clocks in at about 2μs per agent, which is ~10,000x sooner than LangGraph.
- Featherlight Reminiscence Footprint: Agno brokers use simply ~3.75 KiB of reminiscence on common—~50x lower than LangGraph brokers.
- Multimodal Native Assist: No hacks or plugins—Agno is constructed from the bottom as much as work seamlessly with numerous media varieties.
- Mannequin Agnostic: Agno doesn’t care for those who’re utilizing OpenAI, Claude, Gemini, or open-source LLMs. You’re not locked into a selected supplier or runtime.
- Actual-Time Monitoring: Agent periods and efficiency will be noticed stay by way of Agno, which makes debugging and optimization a lot smoother.
Fingers-On with Agno: Constructing TriSage Agent
Utilizing Agno feels refreshingly environment friendly. You possibly can spin up whole fleets of brokers that not solely function in parallel but additionally share reminiscence, instruments, and data bases. These brokers will be specialised and grouped into multi-agent groups, and the reminiscence layer helps storing periods and states in a persistent database.
What’s actually spectacular is how Agno manages complexity with out sacrificing efficiency. It handles real-world agent orchestration—like device chaining, RAG-based retrieval, or structured output era—with out changing into a efficiency bottleneck.
In the event you’ve labored with LangGraph or related frameworks, you’ll instantly discover the startup lag and useful resource consumption that Agno avoids. This turns into a essential differentiator at scale. Let’s construct the TriSage Agent.
Putting in Required Libraries
!pip set up -U agno
!pip set up duckduckgo-search
!pip set up openai
!pip set up pycountry
These are shell instructions to put in the required Python packages:
- agno: Core framework used to outline and run AI brokers.
- duckduckgo-search: Lets brokers use DuckDuckGo to look the net.
- openai: For interfacing with OpenAI’s fashions like GPT-4 or GPT-3.5.
- pycountry: (In all probability not used right here, however put in) helps deal with nation information.
Required Imports
from agno.agent import Agent
from agno.fashions.openai import OpenAIChat
from agno.instruments.duckduckgo import DuckDuckGoTools
from agno.instruments.googlesearch import GoogleSearchTools
from agno.instruments.dalle import DalleTools
from agno.staff import Group
from textwrap import dedent
API Key Setup
from getpass import getpass
OPENAI_KEY = getpass('Enter Open AI API Key: ')
import os
os.environ['OPENAI_API_KEY'] = OPENAI_KEY
- getpass(): Safe strategy to enter your API key (so it’s not seen).
- The secret is then saved within the setting in order that the
agno
framework can decide it up when calling OpenAI’s API.
web_agent – Searches the Internet, writer_agent – Writes the Article, image_agent – Creates Visuals
web_agent = Agent(
title="Internet Agent",
function="Search the net for data on Eiffel tower",
mannequin=OpenAIChat(id="o3-mini"),
instruments=[DuckDuckGoTools()],
directions="Give historic data",
show_tool_calls=True,
markdown=True,
)
writer_agent = Agent(
title="Author Agent",
function="Write complete article on the offered subject",
mannequin=OpenAIChat(id="o3-mini"),
instruments=[GoogleSearchTools()],
directions="Use outlines to jot down articles",
show_tool_calls=True,
markdown=True,
)
image_agent = Agent(
mannequin=OpenAIChat(id="gpt-4o"),
instruments=[DalleTools()],
description=dedent("""
You're an skilled AI artist with experience in numerous inventive kinds,
from photorealism to summary artwork. You could have a deep understanding of composition,
colour idea, and visible storytelling.
"""),
directions=dedent("""
As an AI artist, observe these pointers:
1. Analyze the consumer's request rigorously to know the specified model and temper
2. Earlier than producing, improve the immediate with inventive particulars like lighting, perspective, and environment
3. Use the `create_image` device with detailed, well-crafted prompts
4. Present a quick clarification of the inventive selections made
5. If the request is unclear, ask for clarification about model preferences
At all times intention to create visually hanging and significant photos that seize the consumer's imaginative and prescient!
"""),
markdown=True,
show_tool_calls=True,
)
Mix right into a Group
agent_team = Agent(
staff=[web_agent, writer_agent, image_agent],
mannequin=OpenAIChat(id="gpt-4o"),
directions=["Give historical information", "Use outlines to write articles","Generate Image"],
show_tool_calls=True,
markdown=True,
)
Run the Entire Factor
agent_team.print_response("Write an article on Eiffel towar and generate picture", stream=True)
Output
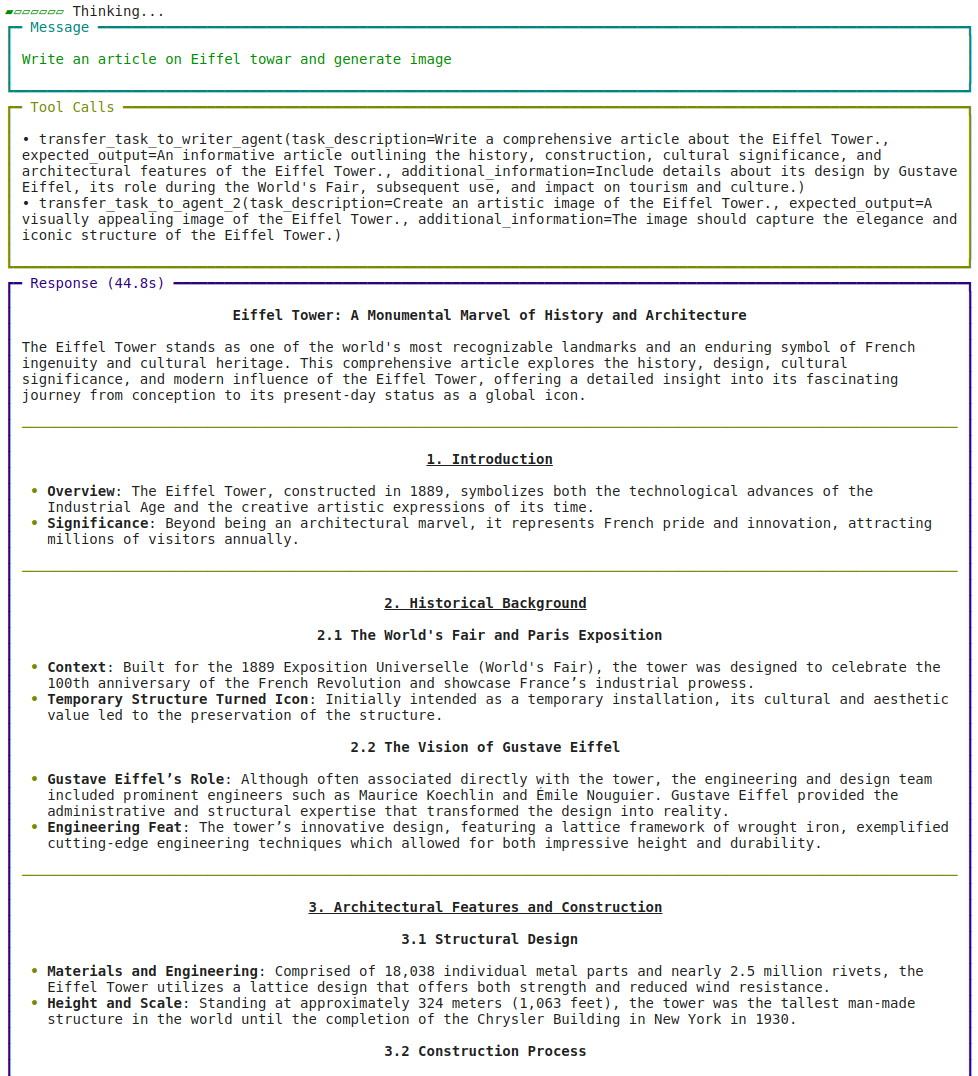
Continued Output
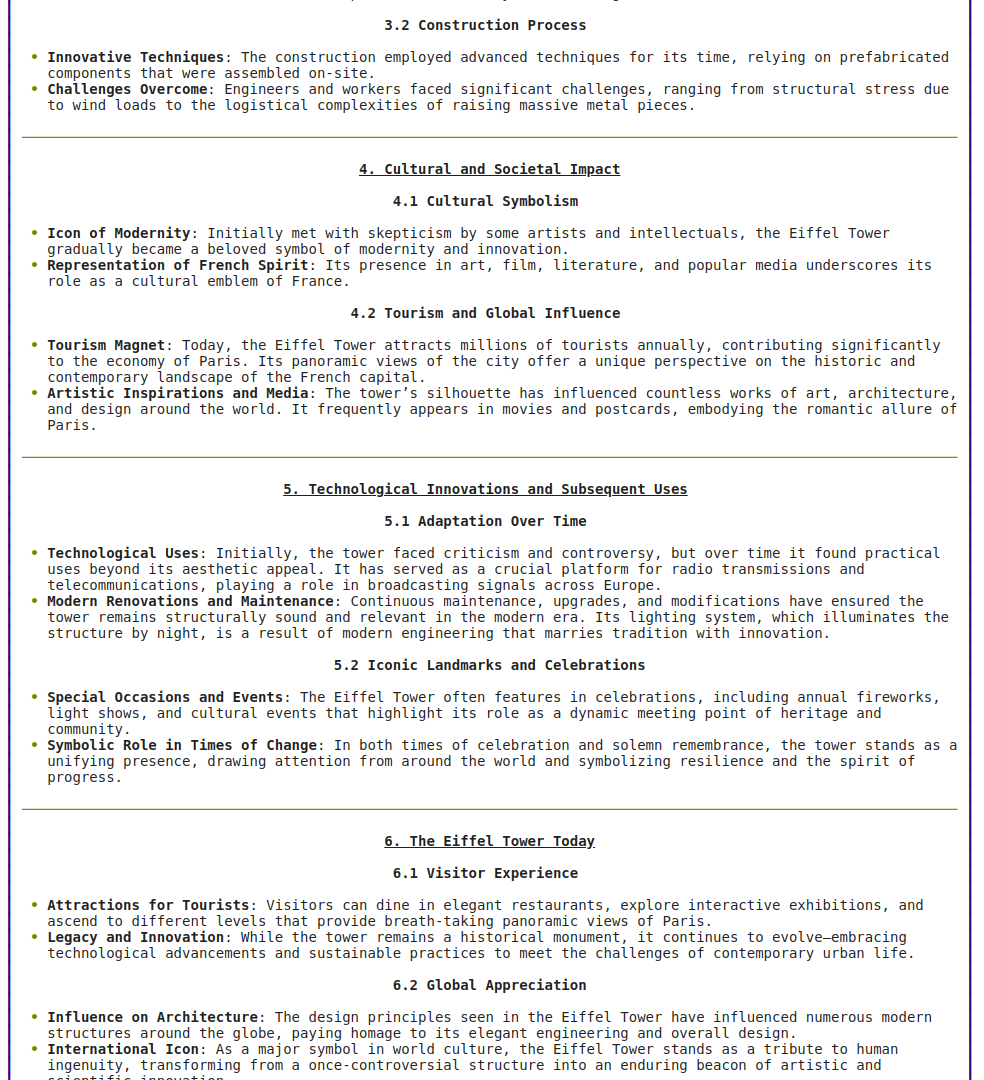
Continued Output
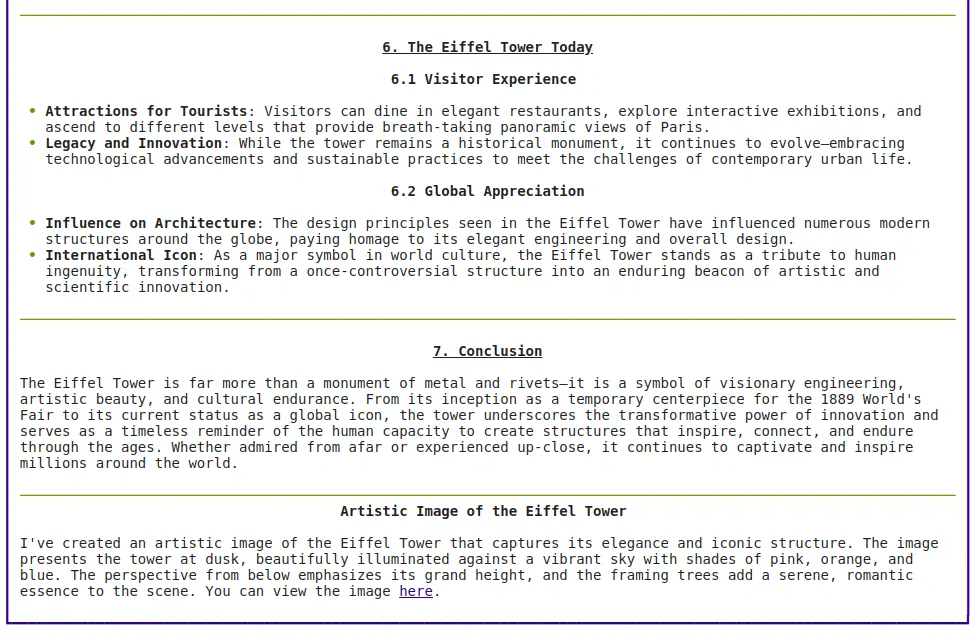
I've created a sensible picture of the Eiffel Tower. The picture captures the
tower's full peak and design, ┃
┃ fantastically highlighted by the late afternoon solar. You possibly can view it by
clicking right here.
Picture Output
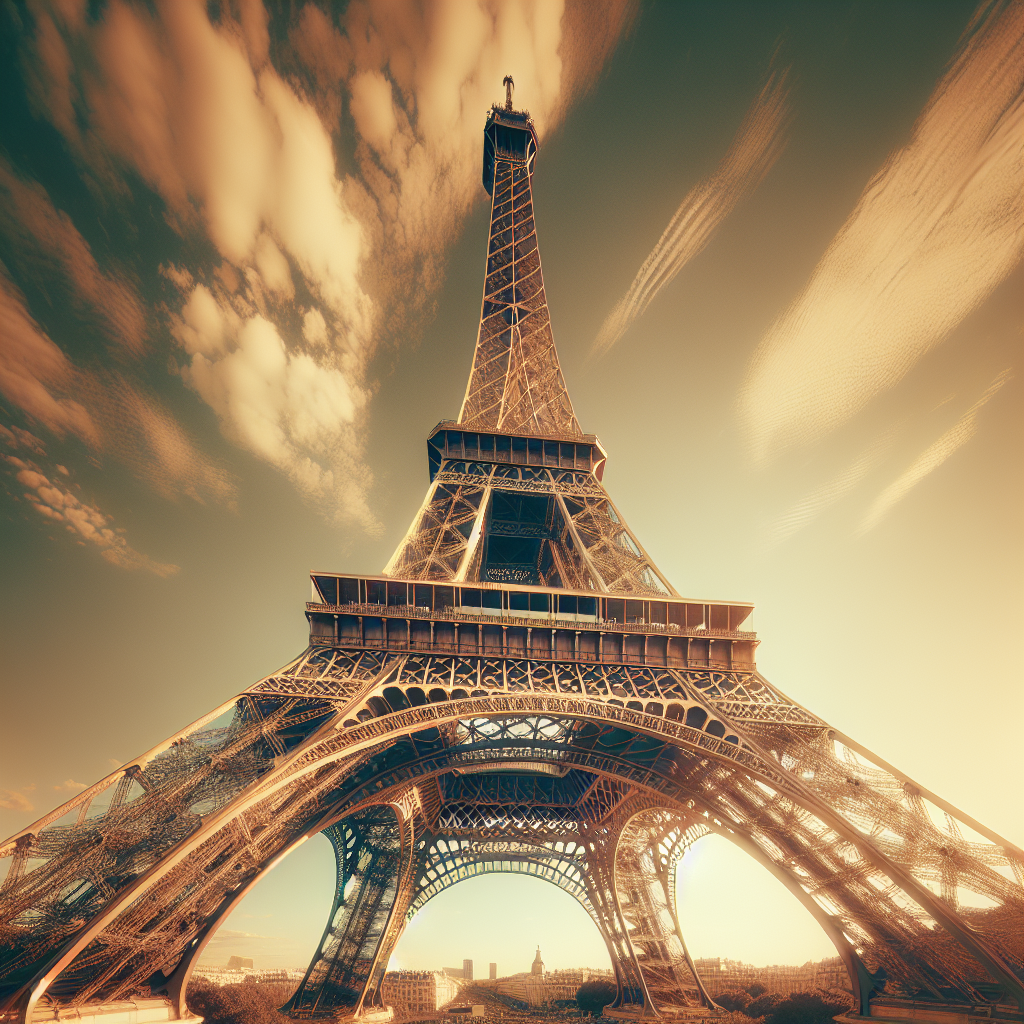
Fingers-On with Agno: Constructing Market Analyst Agent
This Market Analyst Agent is a team-based system utilizing Agno, combining a Internet Agent for real-time information by way of DuckDuckGo and a Finance Agent for monetary information by way of Yahoo Finance. Powered by OpenAI fashions, it delivers market insights and AI firm efficiency utilizing tables, markdown, and source-backed content material for readability, depth, and transparency.
from agno.agent import Agent
from agno.fashions.openai import OpenAIChat
from agno.instruments.duckduckgo import DuckDuckGoTools
from agno.instruments.yfinance import YFinanceTools
from agno.staff import Group
web_agent = Agent(
title="Internet Agent",
function="Search the net for data",
mannequin=OpenAIChat(id="o3-mini"),
instruments=[DuckDuckGoTools()],
directions="At all times embody sources",
show_tool_calls=True,
markdown=True,
)
finance_agent = Agent(
title="Finance Agent",
function="Get monetary information",
mannequin=OpenAIChat(id="o3-mini"),
instruments=[YFinanceTools(stock_price=True, analyst_recommendations=True, company_info=True)],
directions="Use tables to show information",
show_tool_calls=True,
markdown=True,
)
agent_team = Agent(
staff=[web_agent, finance_agent],
mannequin=OpenAIChat(id="gpt-4o"),
directions=["Always include sources", "Use tables to display data"],
show_tool_calls=True,
markdown=True,
)
agent_team.print_response("What is the market outlook and monetary efficiency of high AI corporations of the world?", stream=True)
Output
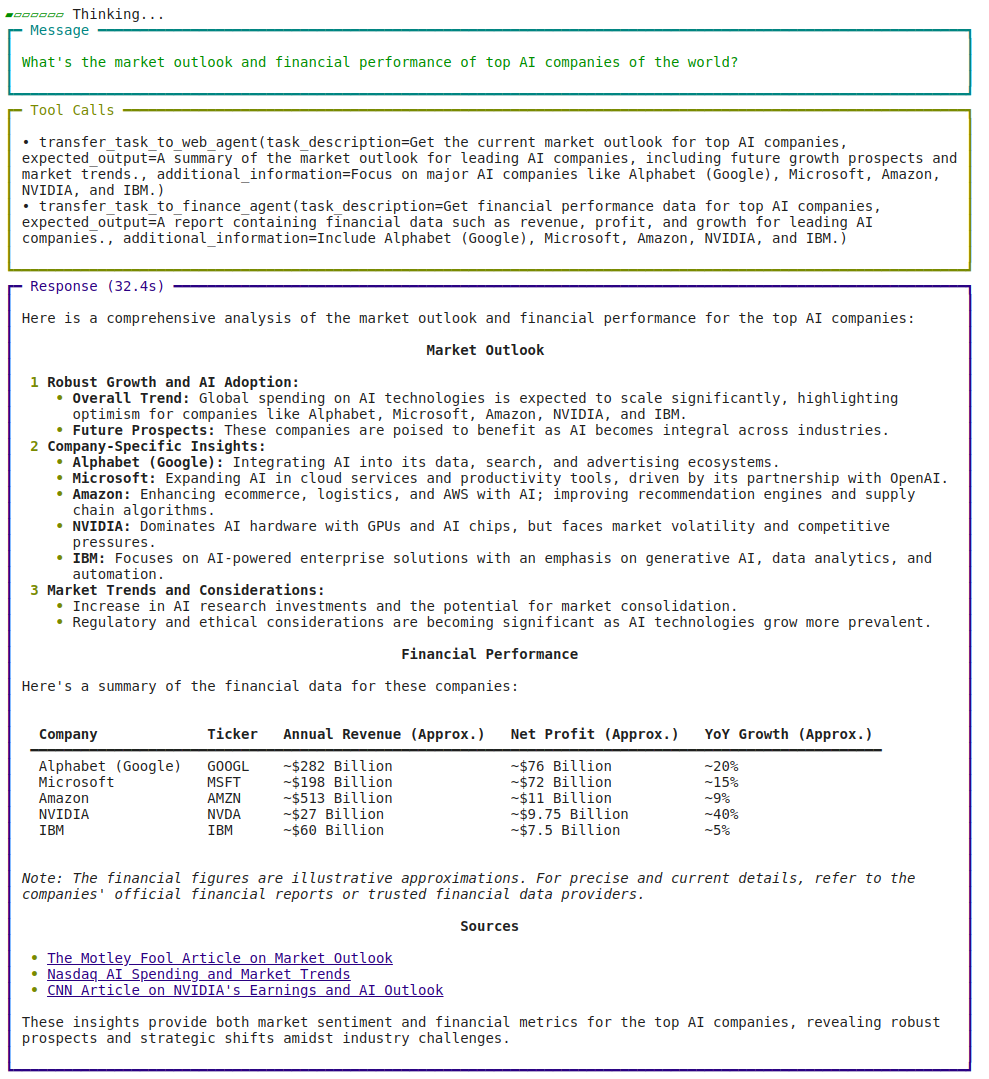
Agno vs LangGraph: Efficiency Showdown
Let’s get into specifics and it’s all included within the official documentation of Agno:
Metric | Agno | LangGraph | Issue |
---|---|---|---|
Agent Instantiation Time | ~2μs | ~20ms | ~10,000x sooner |
Reminiscence Utilization per Agent | ~3.75 KiB | ~137 KiB | ~50x lighter |
- The efficiency testing was accomplished on an Apple M4 MacBook Professional utilizing Python’s tracemalloc for reminiscence profiling.
- Agno measured the typical instantiation and reminiscence utilization over 1000 runs, isolating the Agent code to get a clear delta.
This type of velocity and reminiscence effectivity isn’t nearly numbers—it’s the important thing to scalability. In real-world agent deployments, the place hundreds of brokers could must spin up concurrently, each millisecond and kilobyte issues.
LangGraph, whereas highly effective and extra structured for sure flow-based purposes, tends to battle below this type of load except closely optimized. That may not be a problem for low-scale apps, however it turns into costly quick when operating production-scale brokers.
So… Is Agno Higher Than LangGraph?
Not essentially. It will depend on what you’re constructing:
- In the event you’re engaged on flow-based agent logic (assume: directed graphs of steps with high-level management), LangGraph may provide a extra expressive construction.
- However for those who want ultra-fast, low-footprint, multimodal agent execution, particularly in high-concurrency or dynamic environments, Agno wins by a mile.
Agno clearly favours velocity and system-level effectivity, whereas LangGraph leans into structured orchestration and reliability. That stated, Agno’s builders themselves acknowledge that accuracy and reliability benchmarks are simply as essential—and so they’re presently within the works. Till these are out, we will’t conclude correctness or resilience below edge circumstances.
Additionally learn: Smolagents vs LangGraph: A Complete Comparability of AI Agent Frameworks
Conclusion
From a hands-on perspective, Agno feels prepared for actual workloads, particularly for groups constructing agentic methods at scale. It’s real-time efficiency monitoring, assist for structured output, and talent to plug in reminiscence + vector data make it a compelling platform for constructing strong purposes rapidly.
LangGraph isn’t out of the race—its energy lies in clear, flow-oriented management logic. However for those who’re hitting scaling partitions or must run hundreds of brokers with out melting your infrastructure, Agno is value a severe look.
Login to proceed studying and revel in expert-curated content material.