Introduction
Ever needed to take away an merchandise from a listing however not simply any merchandise, particularly the one at a sure index? Enter Python pop() technique. This built-in perform helps you obtain precisely that. It removes a component from a listing primarily based on its index and, most significantly, returns the eliminated component, providing you with management over your information buildings. Whether or not you’re managing dynamic lists, dealing with consumer enter, or manipulating arrays, understanding python pop() can prevent effort and time. Let’s discover this helpful instrument in depth.
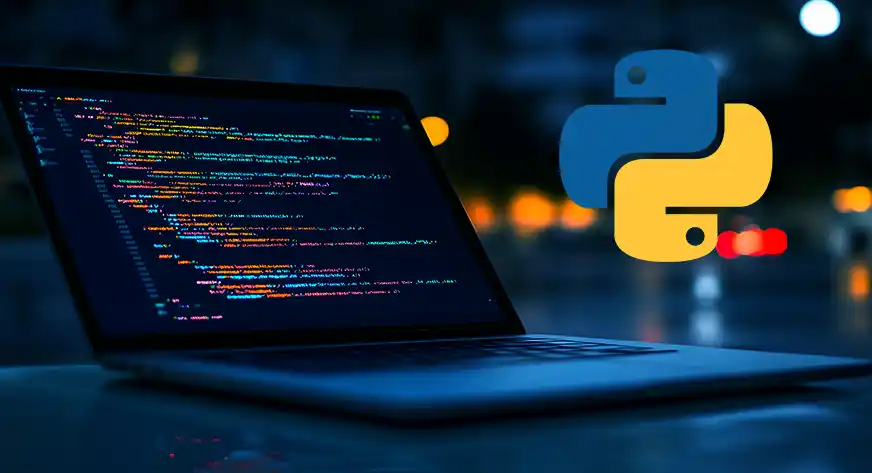
Studying Outcomes
- Perceive the aim and syntax of the
pop()
technique in Python. - Discover ways to take away parts from a listing utilizing
pop()
. - Know the way to work with the index parameter in
pop()
to take away particular parts. - Perceive error dealing with when
pop()
is used incorrectly. - See sensible examples of utilizing
pop()
in varied situations.
What’s the pop()
Methodology in Python?
The pop()
technique in Python is used to take away an merchandise from a checklist at a specified place (index). It additionally returns the worth of the eliminated component. In contrast to strategies like take away()
, which require the worth of the component, pop()
works with indices, providing you with extra management over which merchandise you wish to eradicate from the checklist.
Syntax:
checklist.pop(index)
- checklist: The checklist you wish to take away a component from.
- index (non-obligatory): The index of the component to take away. If no index is offered,
pop()
will take away the final component by default.
How Does the pop()
Methodology Work?
The pop()
technique in Python works by instantly altering the checklist in place and returning the component it removes. This technique gives flexibility in eradicating parts both by specifying their place within the checklist or by defaulting to the final component. Under are detailed explanations of how pop()
behaves in varied situations:
Index Specified
In case you use the pop() technique and specify an index, this perform deletes a component by this index and brings the worth of the deleted component. The checklist could be additionally modified in place, that implies that the unique checklist is altering its content material.
The way it works?
- The component to be deleted is searched utilizing the assistance of the desired index.
- After the component is discovered, it’s deleted and all of the others to the precise of the index are moved one step to the left to take the place of the deleted component.
- The eliminated component is returned: it may be saved in a variable or used with out being assigned to one thing.
Instance:
my_list = ['apple', 'banana', 'cherry', 'date']
removed_element = my_list.pop(1) # Removes component at index 1, which is 'banana'
print(removed_element) # Output: 'banana'
print(my_list) # Output: ['apple', 'cherry', 'date']
Index Not Specified
If no index is handed to perform pop(), then it removes the final merchandise of the checklist. That is useful when it’s essential to delete the most recent or the final component with out the necessity for figuring the index.
The way it works?
- As an illustration, if no index has been outlined, then pop() instantly refers back to the final merchandise within the checklist, the identical with (-1).
- The ultimate worth within the checklist is then taken away and the strategy then returns the worth related to such parameter.
Instance:
my_list = [10, 20, 30, 40]
removed_element = my_list.pop() # Removes the final component, 40
print(removed_element) # Output: 40
print(my_list) # Output: [10, 20, 30]
IndexError
Python raises an IndexError
should you try to make use of the pop()
technique on an empty checklist or present an invalid index that’s out of bounds. This habits ensures that the checklist can’t be accessed or modified past its accessible vary.
- Popping from an Empty Checklist: In case you attempt to pop a component from an empty checklist, Python raises an
IndexError
as a result of there are not any parts to take away. This prevents undefined habits and alerts you that the operation is invalid.
Instance:
empty_list = []
empty_list.pop() # Raises IndexError: pop from empty checklist
- Invalid Index: In case you present an index that’s better than or equal to the size of the checklist, Python raises an
IndexError
as a result of that index doesn’t exist inside the checklist’s bounds.
Instance:
my_list = [1, 2, 3]
my_list.pop(10) # Raises IndexError: pop index out of vary
Adverse Indexing Utilizing pop() Perform
Lists in Python have the potential of unfavourable indexing, this simply implies that you’ll be able to depend from the final index backwards. One other technique that helps that is the pop() technique. A unfavourable index can be utilized to go to the pop() perform with a view to delete parts from the checklist by the top.
Instance:
my_list = [100, 200, 300, 400]
removed_item = my_list.pop(-2) # Removes the second-to-last component
print(removed_item) # Output: 300
print(my_list) # Output: [100, 200, 400]
Utilizing pop()
with Dictionaries in Python
The pop() technique works not solely with checklist sort objects in Python, however with dictionaries as properly. Based mostly on that, the pop() technique is utility to dictionaries the place a key and faraway from the checklist and the worth similar to that secret is returned.
That is particularly vital if you must use the ‘pop’ technique to get and delete a component from the dictionary, on the identical time.
When performing pop() on the dictionary, you’ll go the important thing within the dictionary whose worth you want to get hold of and delete directly. If the hot button is current in dictionary then the pop() capabilities will return the worth and likewise eliminates the key-value pair from the dictionary. If the important thing doesn’t exist, you possibly can both make Python throw the KeyError for you or provide a second worth that the perform ought to use if the important thing doesn’t exist within the dictionary.
Examples of Utilizing pop()
with Dictionaries
Allow us to discover totally different examples of utilizing pop() with dictionaries:
Primary Utilization
pupil = {'identify': 'John', 'age': 25, 'course': 'Arithmetic'}
age = pupil.pop('age')
print(age) # Output: 25
print(pupil) # Output: {'identify': 'John', 'course': 'Arithmetic'}
Offering a Default Worth
If the important thing doesn’t exist within the dictionary, you possibly can forestall the KeyError
by offering a default worth.
pupil = {'identify': 'John', 'age': 25, 'course': 'Arithmetic'}
main = pupil.pop('main', 'Not discovered')
print(main) # Output: Not discovered
print(pupil) # Output: {'identify': 'John', 'age': 25, 'course': 'Arithmetic'}
Dealing with KeyError
With out a Default Worth
In case you don’t present a default worth and the hot button is lacking, Python will elevate a KeyError
.
pupil = {'identify': 'John', 'age': 25, 'course': 'Arithmetic'}
grade = pupil.pop('grade') # KeyError: 'grade'
How pop()
Impacts Reminiscence
If a listing is resized and a component is faraway from the checklist utilizing the pop technique then it alters the pointer of the checklist. The lists in Python are dynamic arrays, so manipulating on them comparable to append or pop will really change the reminiscence allocation of the checklist.
Right here’s an in depth rationalization of how pop()
impacts reminiscence:
Dynamic Array and Reminiscence Administration
Python lists are dynamic arrays, which means they’re saved in contiguous blocks of reminiscence. Whenever you take away a component utilizing pop()
, Python should reallocate or shift parts to keep up this contiguous reminiscence construction.
- If the component eliminated is not the final one (i.e., you present a selected index), Python shifts all the weather that come after the eliminated component to fill the hole.
- In case you take away the final component (i.e., no index is offered), there isn’t any want for shifting; Python merely updates the checklist’s inside measurement reference.
Inner Reminiscence Allocation
Lists in Python are over-allocated, which means they reserve further area to accommodate future parts with out requiring speedy reallocation of reminiscence. This minimizes the overhead of incessantly resizing the checklist when parts are added or eliminated. Nonetheless, eradicating parts with pop()
decreases the dimensions of the checklist, and over time, Python would possibly determine to launch unused reminiscence, though this doesn’t occur instantly after a single pop()
operation.
Shifting Parts in Reminiscence
Whenever you take away a component from the center of a listing, the opposite parts within the checklist shift to the left to fill the hole. This course of entails Python shifting information round in reminiscence, which could be time-consuming, particularly if the checklist is giant. Since Python has to bodily shift every component one step over, this will grow to be inefficient because the checklist grows in measurement.
Instance:
my_list = [10, 20, 30, 40, 50]
my_list.pop(1) # Removes the component at index 1, which is 20
print(my_list) # Output: [10, 30, 40, 50]
Reminiscence Effectivity for Giant Lists
When working with giant lists, frequent use of pop()
(particularly from indices apart from the final one) could cause efficiency degradation due to the repeated must shift parts. Nonetheless, eradicating parts from the top of the checklist (utilizing pop()
with no index) is environment friendly and doesn’t contain shifting.
Effectivity of pop()
The effectivity of the pop()
technique is dependent upon the place within the checklist the component is being eliminated. Under are particulars on the time complexity for various situations:
Greatest Case (O(1) – Eradicating the Final Ingredient)
- When no index is specified,
pop()
removes the final component within the checklist. This operation is fixed time, O(1), as a result of no different parts have to be shifted. - Eradicating the final component solely entails reducing the checklist’s measurement by one and doesn’t require modifying the positions of some other parts in reminiscence.
Instance:
my_list = [5, 10, 15, 20]
my_list.pop() # Removes the final component, 20
print(my_list) # Output: [5, 10, 15]
Worst Case (O(n) – Eradicating the First Ingredient)
- When an index is specified (significantly when it’s 0, the primary component),
pop()
turns into a linear time operation, O(n), as a result of each component after the eliminated one should be shifted one place to the left. - The bigger the checklist, the extra parts have to be shifted, making this much less environment friendly for big lists.
Instance:
my_list = [1, 2, 3, 4, 5]
my_list.pop(0) # Removes the primary component, 1
print(my_list) # Output: [2, 3, 4, 5]
Intermediate Instances (O(n) – Eradicating from the Center)
Even, should you take an merchandise out at a few of random place like pop(5), it additionally takes solely O(n) time the place n is the variety of parts after the fifth that they’re compelled to shift. The nearer the worth of the index is to the utmost representing the final component within the checklist, the much less operations that must be carried out to execute it making it much less environment friendly than the straight pop however extra environment friendly if one compares it with a pop entrance.
Instance:
my_list = [10, 20, 30, 40, 50]
my_list.pop(2) # Removes the component at index 2, which is 30
print(my_list) # Output: [10, 20, 40, 50]
Comparability with take away()
The pop()
and take away()
strategies each delete parts from a listing, however they behave in a different way and serve distinct functions. Right here’s an in depth comparability between the 2:
Function | pop() Methodology |
take away() Methodology |
---|---|---|
What It Does | Takes out an merchandise from the checklist and offers it again to you. You possibly can both specify which place (index) or go away it empty to take away the final merchandise. | Appears to be like for a selected worth within the checklist and removes it. No return worth, simply deletes it. |
How It Returns Values | Offers you the eliminated merchandise. | Doesn’t return something – it simply deletes the merchandise. |
Utilizing an Index | Works with indices, letting you choose an merchandise primarily based on its place. | Doesn’t help indices – solely removes primarily based on worth. |
Utilizing a Worth | You possibly can’t take away an merchandise by its worth, solely by its place. | You possibly can take away an merchandise by instantly specifying the worth. |
Errors You May Encounter | If the index is flawed or the checklist is empty, it throws an IndexError . |
If the worth isn’t within the checklist, you’ll get a ValueError . |
When to Use | Nice if you want each to take away an merchandise and use its worth afterward. | Greatest when you recognize the worth you wish to take away, however don’t must get it again. |
Conclusion
The pop() technique is among the most helpful strategies of Python on the subject of erasing objects from a listing. From dealing with checklist stacks, growing your checklist dynamism and even erasing the final merchandise utilizing pop(), all of it will get made simpler by understanding the way to use this function in Python. Watch out with IndexError whereas making an attempt to entry legitimate indices particularly whereas coping with empty lists.
Regularly Requested Questions
pop()
technique?
A. If no index is handed, pop()
removes and returns the final component of the checklist by default.
pop()
on an empty checklist?
A. No, calling pop()
on an empty checklist will elevate an IndexError
. It is best to make sure the checklist is just not empty earlier than utilizing pop()
.
pop()
deal with unfavourable indices?
A. pop()
can work with unfavourable indices. For instance, pop(-1)
removes the final component, pop(-2)
removes the second-to-last component, and so forth.
pop()
be used with strings or tuples?
A. No, pop()
is restricted to lists. Strings and tuples are immutable, which means their parts can’t be modified or eliminated.
pop()
take away all occurrences of a component?
A. No, pop()
solely removes a single component at a selected index, not all occurrences of that component within the checklist. If it’s essential to take away all occurrences, you should use a loop or checklist comprehension.