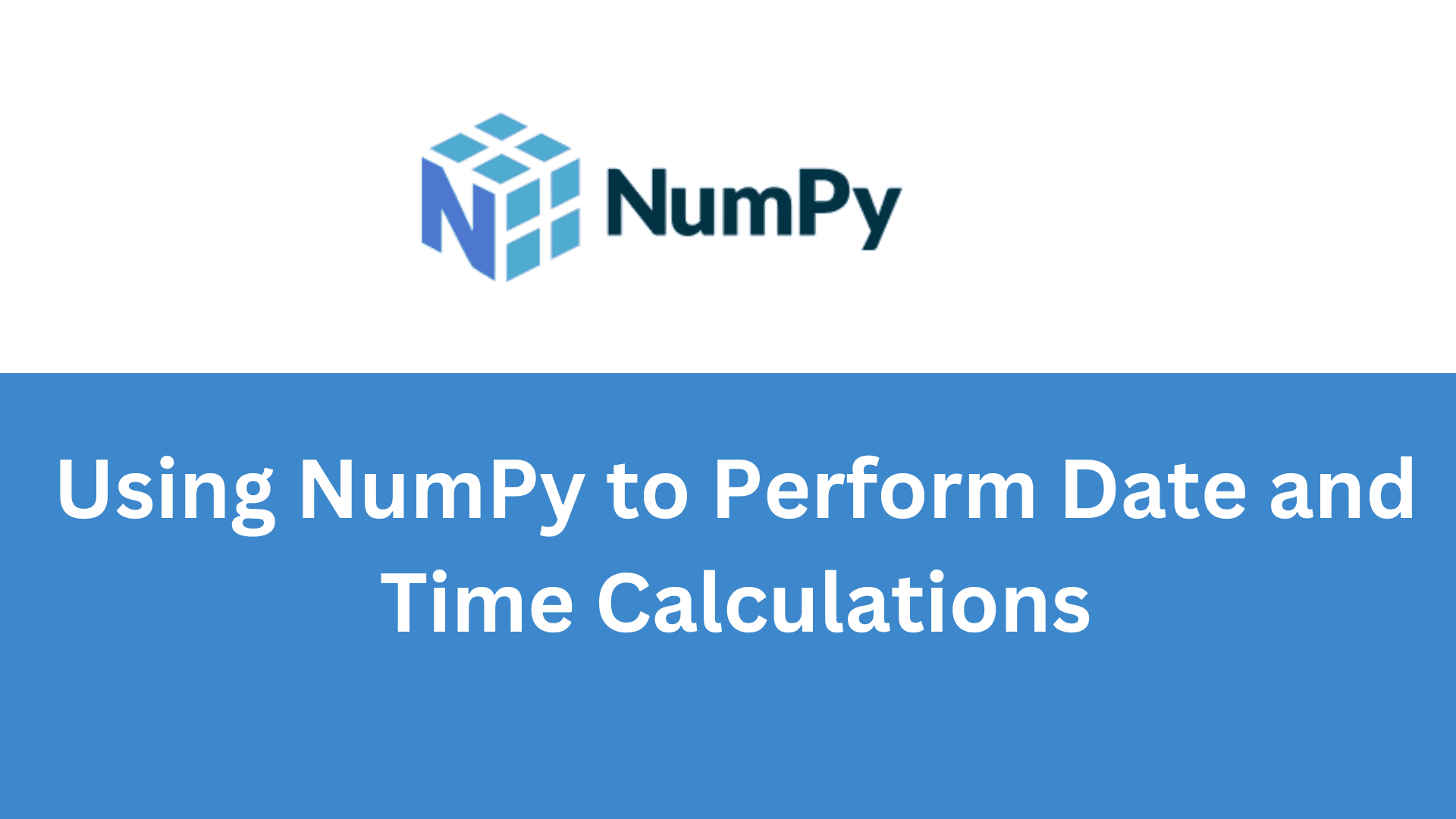
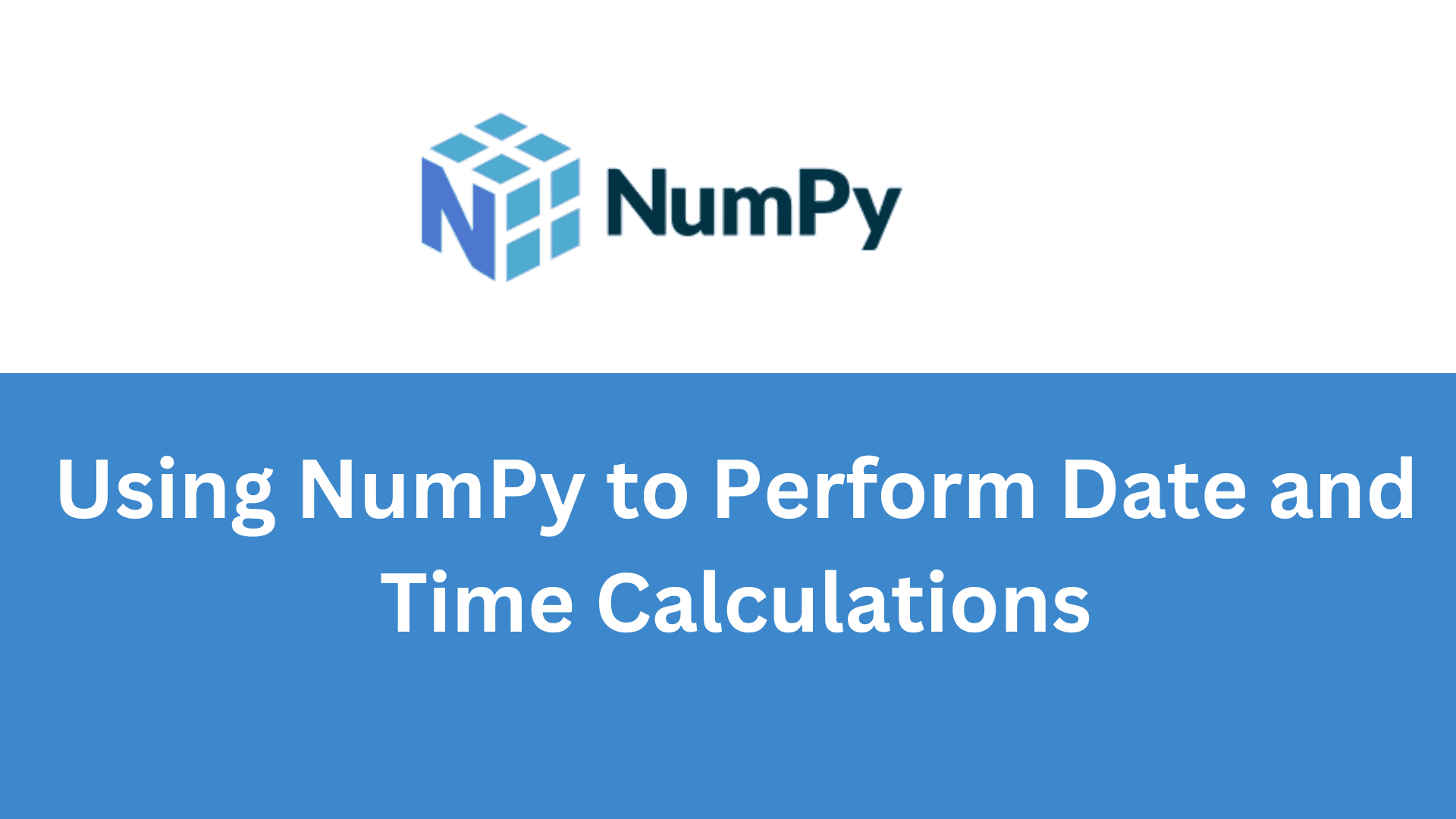
Picture by Writer | Canva
Dates and occasions are on the core of numerous knowledge evaluation duties, from monitoring monetary transactions to monitoring sensor knowledge in real-time. But, dealing with date and time calculations can usually really feel like navigating a maze.
Happily, with NumPy, we’re in luck. NumPy’s strong date and time functionalities take the headache out of those duties, providing a collection of strategies that simplify the method immensely.
As an illustration, NumPy lets you simply create arrays of dates, carry out arithmetic on dates and occasions, and convert between totally different time items with only a few traces of code. Do it’s good to discover the distinction between two dates? NumPy can do this effortlessly. Do you wish to resample your time collection knowledge to a special frequency? NumPy has you lined. This comfort and energy make NumPy a useful device for anybody working with date and time calculations, turning what was a posh problem into an easy activity.
This text will information you thru performing date and time calculations utilizing NumPy. We’ll cowl what datetime is and the way it’s represented, the place date and time are generally used, frequent difficulties and points utilizing it, and finest practices.
What’s DateTime
DateTime refers back to the illustration of dates and occasions in a unified format. It consists of particular calendar dates and occasions, usually all the way down to fractions of a second. This mix is essential for precisely recording and managing temporal knowledge, reminiscent of timestamps in logs, scheduling occasions, and conducting time-based analyses.
Normally programming and knowledge evaluation, DateTime is often represented by specialised knowledge varieties or objects that present a structured method to deal with dates and occasions. These objects enable for simple manipulation, comparability, and arithmetic operations involving dates and occasions.
NumPy and different libraries like pandas present strong assist for DateTime operations, making working with temporal knowledge in numerous codecs and performing advanced calculations straightforward and exact.
In NumPy, date and time dealing with primarily revolve across the datetime64
knowledge kind and related capabilities. You may be questioning why the info kind is known as datetime64. It is because datetime is already taken by the Python normal library.
Here is a breakdown of the way it works:
datetime64 Information Kind
- Illustration: NumPy’s
datetime64
dtype represents dates and occasions as 64-bit integers, providing environment friendly storage and manipulation of temporal knowledge. - Format: Dates and occasions in
datetime64
format are specified with a string that signifies the specified precision, reminiscent ofYYYY-MM-DD
for dates orYYYY-MM-DD HH:mm:ss
for timestamps all the way down to seconds.
For instance:
import numpy as np
# Making a datetime64 array
dates = np.array(['2024-07-15', '2024-07-16', '2024-07-17'], dtype="datetime64")
# Performing arithmetic operations
next_day = dates + np.timedelta64(1, 'D')
print("Unique Dates:", dates)
print("Subsequent Day:", next_day)
Options of datetime64
in NumPy
NumPy’s datetime64
presents strong options to simplify a number of operations. From versatile decision dealing with to highly effective arithmetic capabilities, datetime64
makes working with temporal knowledge simple and environment friendly.
- Decision Flexibility:
datetime64
helps numerous resolutions from nanoseconds to years. For instance,ns (nanoseconds), us (microseconds), ms (milliseconds), s (seconds), m (minutes), h (hours), D (days), W (weeks), M (months), Y (years). - Arithmetic Operations: Carry out direct arithmetic on
datetime64
objects, reminiscent of including or subtracting time items, for instance, including days to a date. - Indexing and Slicing: Make the most of normal NumPy indexing and slicing strategies on
datetime64
arrays.For instance, extracting a variety of dates. - Comparability Operations: Evaluate
datetime64
objects to find out chronological order. Instance: Checking if one date is earlier than one other. - Conversion Features: Convert between
datetime64
and different date/time representations. Instance: Changing adatetime64
object to a string.
np.datetime64('2024-07-15T12:00', 'm') # Minute decision
np.datetime64('2024-07-15', 'D') # Day decision
date = np.datetime64('2024-07-15')
next_week = date + np.timedelta64(7, 'D')
dates = np.array(['2024-07-15', '2024-07-16', '2024-07-17'], dtype="datetime64")
subset = dates[1:3]
date1 = np.datetime64('2024-07-15')
date2 = np.datetime64('2024-07-16')
is_before = date1 < date2 # True
date = np.datetime64('2024-07-15')
date_str = date.astype('str')
The place Do You Are inclined to Use Date and Time?
Date and time can be utilized in a number of sectors, such because the monetary sector, to trace inventory costs, analyze market developments, consider monetary efficiency over time, calculate returns, assess volatility, and determine patterns in time collection knowledge.
You too can use Date and time in different sectors, reminiscent of healthcare, to handle affected person information with time-stamped knowledge for medical historical past, remedies, and drugs schedules.
State of affairs: Analyzing E-commerce Gross sales Information
Think about you are a knowledge analyst working for an e-commerce firm. You have got a dataset containing gross sales transactions with timestamps, and it’s good to analyze gross sales patterns over the previous 12 months. Right here’s how one can leverage datetime64
in NumPy:
# Loading and Changing Information
import numpy as np
import matplotlib.pyplot as plt
# Pattern knowledge: timestamps of gross sales transactions
sales_data = np.array(['2023-07-01T12:34:56', '2023-07-02T15:45:30', '2023-07-03T09:12:10'], dtype="datetime64")
# Extracting Particular Time Intervals
# Extracting gross sales knowledge for July 2023
july_sales = sales_data[(sales_data >= np.datetime64('2023-07-01')) & (sales_data < np.datetime64('2023-08-01'))]
# Calculating Every day Gross sales Counts
# Changing timestamps to dates
sales_dates = july_sales.astype('datetime64[D]')
# Counting gross sales per day
unique_dates, sales_counts = np.distinctive(sales_dates, return_counts=True)
# Analyzing Gross sales Tendencies
plt.plot(unique_dates, sales_counts, marker='o')
plt.xlabel('Date')
plt.ylabel('Variety of Gross sales')
plt.title('Every day Gross sales Counts for July 2023')
plt.xticks(rotation=45) # Rotates x-axis labels for higher readability
plt.tight_layout() # Adjusts format to stop clipping of labels
plt.present()
On this state of affairs, datetime64
lets you simply manipulate and analyze the gross sales knowledge, offering insights into every day gross sales patterns.
Widespread difficulties When Utilizing Date and Time
Whereas NumPy’s datetime64
is a robust device for dealing with dates and occasions, it’s not with out its challenges. From parsing numerous date codecs to managing time zones, builders usually encounter a number of hurdles that may complicate their knowledge evaluation duties. This part highlights a few of these typical points.
- Parsing and Changing Codecs: Dealing with numerous date and time codecs might be difficult, particularly when working with knowledge from a number of sources.
- Time Zone Dealing with:
datetime64
in NumPy doesn’t natively assist time zones. - Decision Mismatches: Totally different elements of a dataset might have timestamps with totally different resolutions (e.g., some in days, others in seconds).
Learn how to Carry out Date and Time Calculations
Let’s discover examples of date and time calculations in NumPy, starting from primary operations to extra superior situations, that will help you harness the complete potential of datetime64
in your knowledge evaluation wants.
Including Days to a Date
The purpose right here is to exhibit how you can add a particular variety of days (5 days on this case) to a given date (2024-07-15)
import numpy as np
# Outline a date
start_date = np.datetime64('2024-07-15')
# Add 5 days to the date
end_date = start_date + np.timedelta64(5, 'D')
print("Begin Date:", start_date)
print("Finish Date after including 5 days:", end_date)
Output:
Begin Date: 2024-07-15
Finish Date after including 5 days: 2024-07-20
Clarification:
- We outline the
start_date
utilizingnp.datetime64
. - Utilizing
np.timedelta64
, we add 5 days (5, D) tostart_date
to getend_date
. - Lastly, we print each
start_date
andend_date
to look at the results of the addition.
Calculating Time Distinction Between Two Dates
Calculate the time distinction in hours between two particular dates (2024-07-15T12:00 and 2024-07-17T10:30)
import numpy as np
# Outline two dates
date1 = np.datetime64('2024-07-15T12:00')
date2 = np.datetime64('2024-07-17T10:30')
# Calculate the time distinction in hours
time_diff = (date2 - date1) / np.timedelta64(1, 'h')
print("Date 1:", date1)
print("Date 2:", date2)
print("Time distinction in hours:", time_diff)
Output:
Date 1: 2024-07-15T12:00
Date 2: 2024-07-17T10:30
Time distinction in hours: 46.5
Clarification:
- Outline
date1
anddate2
utilizingnp.datetime64
with particular timestamps. - Compute
time_diff
by subtractingdate1
fromdate2
and dividing bynp.timedelta64(1, 'h')
to transform the distinction to hours. - Print the unique dates and the calculated time distinction in hours.
Dealing with Time Zones and Enterprise Days
Calculate the variety of enterprise days between two dates, excluding weekends and holidays.
import numpy as np
import pandas as pd
# Outline two dates
start_date = np.datetime64('2024-07-01')
end_date = np.datetime64('2024-07-15')
# Convert to pandas Timestamp for extra advanced calculations
start_date_ts = pd.Timestamp(start_date)
end_date_ts = pd.Timestamp(end_date)
# Calculate the variety of enterprise days between the 2 dates
business_days = pd.bdate_range(begin=start_date_ts, finish=end_date_ts).measurement
print("Begin Date:", start_date)
print("Finish Date:", end_date)
print("Variety of Enterprise Days:", business_days)
Output:
Begin Date: 2024-07-01
Finish Date: 2024-07-15
Variety of Enterprise Days: 11
Clarification:
- NumPy and Pandas Import: NumPy is imported as
np
and Pandas aspd
to make the most of their date and time dealing with functionalities. - Date Definition: Defines
start_date
andend_date
utilizing NumPy’s code model=”background: #F5F5F5″ < np.datetime64 to specify the beginning and finish dates (‘2024-07-01‘ and ‘2024-07-15‘, respectively). - Conversion to pandas Timestamp: This conversion converts
start_date
andend_date
fromnp.datetime64
to pandas Timestamp objects (start_date_ts
andend_date_ts
) for compatibility with pandas extra superior date manipulation capabilities. - Enterprise Day Calculation: Makes use of
pd.bdate_range
to generate a variety of enterprise dates (excluding weekends) betweenstart_date_ts
andend_date_ts
. Calculate the dimensions (variety of components) of this enterprise date vary (business_days
), representing the depend of enterprise days between the 2 dates. - Print the unique
start_date
andend_date
. - Shows the calculated variety of enterprise days (
business_days
) between the desired dates.
Greatest Practices When Utilizing datetime64
When working with date and time knowledge in NumPy, following finest practices ensures that your analyses are correct, environment friendly, and dependable. Correct dealing with of datetime64
can forestall frequent points and optimize your knowledge processing workflows. Listed here are some key finest practices to remember:
- Guarantee all date and time knowledge are in a constant format earlier than processing. This helps keep away from parsing errors and inconsistencies.
- Choose the decision (‘D‘, ‘h‘, ‘m‘, and so forth.) that matches your knowledge wants. Keep away from mixing totally different resolutions to stop inaccuracies in calculations.
- Use
datetime64
to signify lacking or invalid dates, and preprocess your knowledge to handle these values earlier than evaluation. - In case your knowledge consists of a number of time zones, Standardize all timestamps to a typical time zone early in your processing workflow.
- Examine that your dates fall inside legitimate ranges for `datetime64` to keep away from overflow errors and sudden outcomes.
Conclusion
In abstract, NumPy’s datetime64
dtype gives a strong framework for managing date and time knowledge in numerical computing. It presents versatility and computational effectivity for numerous functions, reminiscent of knowledge evaluation, simulations, and extra.
We explored how you can carry out date and time calculations utilizing NumPy, delving into the core ideas and its illustration with the datetime64
knowledge kind. We mentioned the frequent functions of date and time in knowledge evaluation. We additionally examined the frequent difficulties related to dealing with date and time knowledge in NumPy, reminiscent of format inconsistencies, time zone points, and backbone mismatches
By adhering to those finest practices, you may be certain that your work with datetime64
is exact and environment friendly, resulting in extra dependable and significant insights out of your knowledge.
Shittu Olumide is a software program engineer and technical author captivated with leveraging cutting-edge applied sciences to craft compelling narratives, with a eager eye for element and a knack for simplifying advanced ideas. You too can discover Shittu on Twitter.