Introduction
In the case of working with Massive Language Fashions (LLMs) like GPT-3 or GPT-4, immediate engineering is a game-changer. Have you ever ever questioned the best way to make your interactions with AI extra detailed and arranged? Enter the Chain of Image technique—a cutting-edge approach designed to do exactly that. On this paper, we’ll dive into what this technique is all about, how you should use it, and the way it can improve your AI-powered duties. Let’s discover this fascinating strategy collectively!
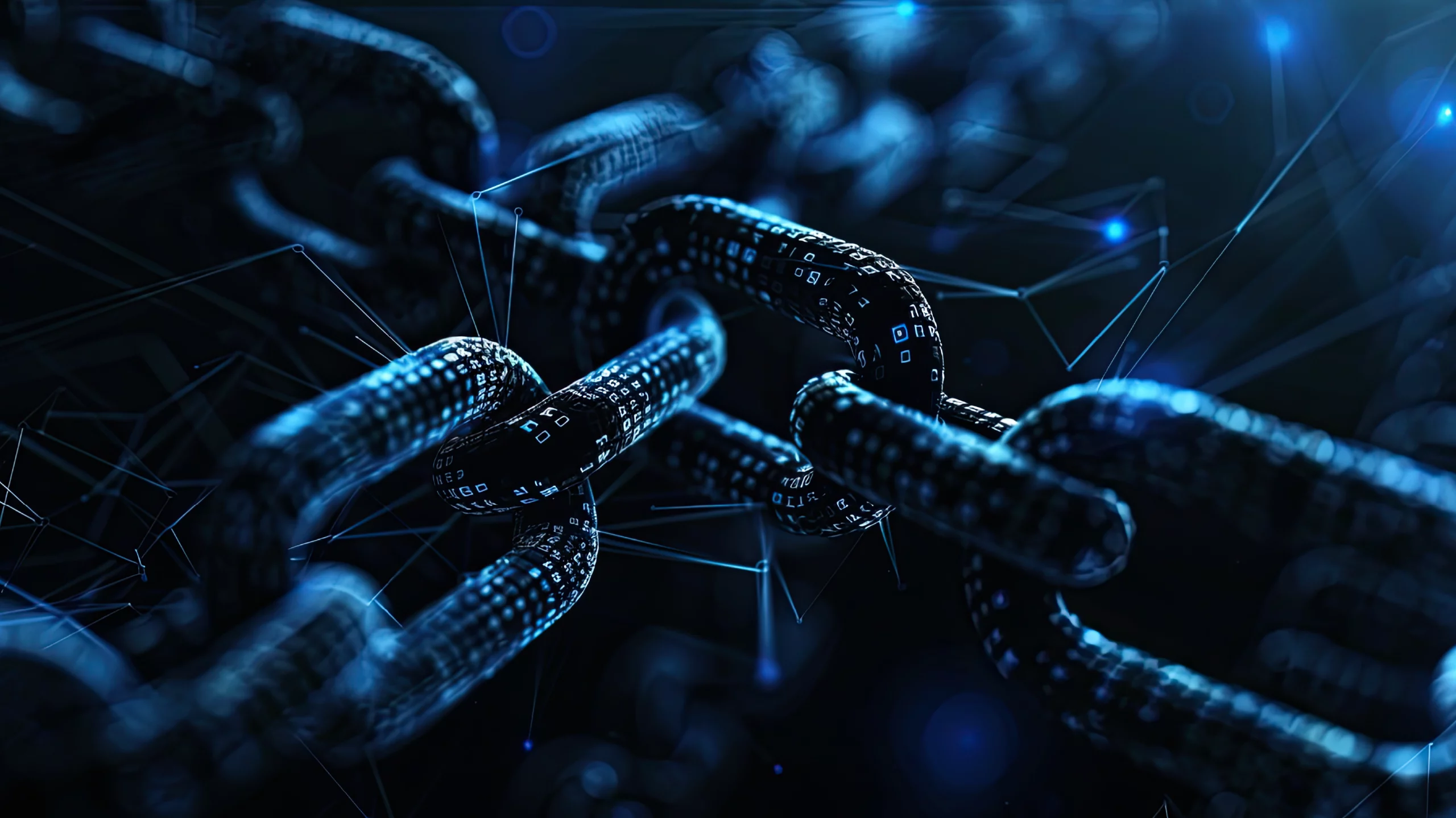
Overview
- Perceive the idea and construction of the Chain of Image approach in immediate engineering.
- Implement a fundamental Chain of Image strategy utilizing Python and an AI API (e.g., OpenAI’s GPT fashions).
- Analyze the advantages and challenges of utilizing the Chain of Image technique for complicated AI duties.
- Design a Chain of Image construction for a selected utility, comparable to story technology or problem-solving.
- Consider the effectiveness of the Chain of Image strategy in comparison with conventional immediate engineering strategies.
Understanding the Chain of Image Idea
In immediate engineering, the phrase “Chain of Image” describes a immediate structuring approach that makes use of a sequence of symbolic representations to direct the AI’s response. This method makes multi-step reasoning and activity completion attainable and offers extra precise management over the output.
The basic idea is to divide an intricate endeavor into extra manageable segments, every denoted by a logo. These symbols information the AI by means of a sure course of or cognitive course of by serving as anchors or checkpoints throughout the immediate.
Key Elements of Chain of Image
- Symbols: distinct markers for each thought or section within the sequence.
- Directions: Clearly outlined directions linked to each image.
- Context: Background particulars or limitations for each motion.
- Placeholders for Output: Particular areas the place the AI can enter its solutions.
Implementing Chain of Image
Set up of dependencies
!pip set up openai --upgrade
#Importing libraries :
import os
from openai import OpenAI
#Setting Api key configuration
os.environ["OPENAI_API_KEY"]= “Your open-API-Key”
consumer = OpenAI()
Let’s take a look at a sensible implementation of the Chain of Image strategy. We’ll use a Python script to generate a narrative utilizing this method.
import os
import openai
from IPython.show import show, Markdown, Picture as IPImage
from PIL import Picture, ImageDraw, ImageFont
import textwrap
# Outline the Chain of Image construction
story_chain = {
"Ω": {
"instruction": "Generate a fundamental premise for a science fiction story",
"context": "Consider a singular idea involving house exploration or superior know-how",
"output": ""
},
"Δ": {
"instruction": "Develop the primary character based mostly on the premise",
"context": "Think about their background, motivations, and challenges",
"output": ""
},
"Φ": {
"instruction": "Create a plot define",
"context": "Embody a starting, center, and finish. Introduce battle and backbone",
"output": ""
},
"Ψ": {
"instruction": "Write the opening paragraph",
"context": "Set the tone and introduce the primary components of the story",
"output": ""
}
}
def generate_story_element(immediate):
response = consumer.chat.completions.create(
messages=[
{"role": "system", "content": "You are a creative writing assistant. Format your responses in Markdown."},
{"role": "user", "content": prompt + " Provide your response in Markdown format."}
],
mannequin="gpt-3.5-turbo",
)
return response.selections[0].message.content material.strip()
def text_to_image(textual content, filename, title):
# Create a brand new picture with white background
img = Picture.new('RGB', (800, 600), shade="white")
d = ImageDraw.Draw(img)
# Draw the title
d.textual content((10, 10), title, fill=(0, 0, 0))
# Wrap the textual content
wrapped_text = textwrap.wrap(textual content, width=70)
# Draw the textual content
y_text = 50
for line in wrapped_text:
d.textual content((10, y_text), line, fill=(0, 0, 0))
y_text += 20
# Save the picture
img.save(filename)
# Course of every step within the chain
for image, content material in story_chain.gadgets():
immediate = f"Image: {image}nInstruction: {content material['instruction']}nContext: {content material['context']}n"
if image != "Ω":
immediate += f"Based mostly on the earlier: {story_chain[list(story_chain.keys())[list(story_chain.keys()).index(symbol) - 1]]['output']}n"
immediate += "Output:"
content material['output'] = generate_story_element(immediate)
# Show the output
show(Markdown(f"### {image}:n{content material['output']}"))
# Create and save a picture for this step
text_to_image(content material['output'], f"{image}.png", image)
# Show the saved picture
show(IPImage(filename=f"{image}.png"))
# Compile the ultimate story
final_story = f"""
## Premise:
{story_chain['Ω']['output']}
## Important Character:
{story_chain['Δ']['output']}
## Plot Define:
{story_chain['Φ']['output']}
## Opening Paragraph:
{story_chain['Ψ']['output']}
"""
# Show the ultimate story
show(Markdown("# FINAL STORY ELEMENTS:n" + final_story))
# Create and save a picture for the ultimate story
text_to_image(final_story, "final_story.png", "FINAL STORY ELEMENTS")
# Show the ultimate story picture
show(IPImage(filename="final_story.png"))
print("Photographs have been saved as PNG information within the present listing.")
Output
Ω (Omega)
This part incorporates the premise of the science fiction story. It introduces “The Omega Expedition,” a mission to research a mysterious sign within the type of an Omega image on the galaxy’s edge.
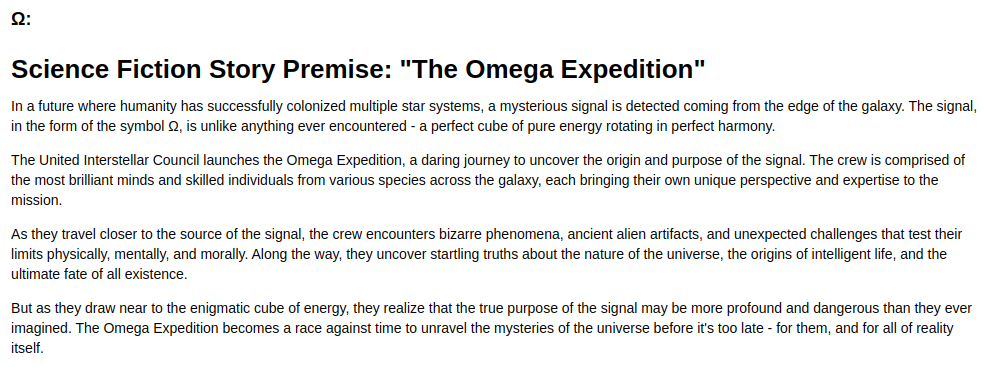
Δ (Delta)
This half describes the primary character, Dr. Elena Novak. It offers her background as a xeno-archaeologist, her motivations for becoming a member of the expedition, and the challenges she’s prone to face.
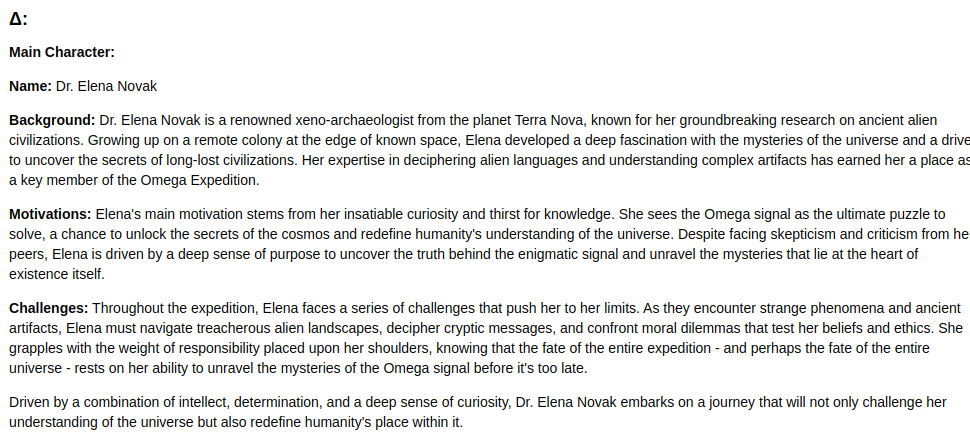
Φ (Phi)
This part outlines the plot of the story, divided into starting, center, and finish. It offers a broad overview of Dr. Novak’s journey and the challenges she’ll face through the expedition.
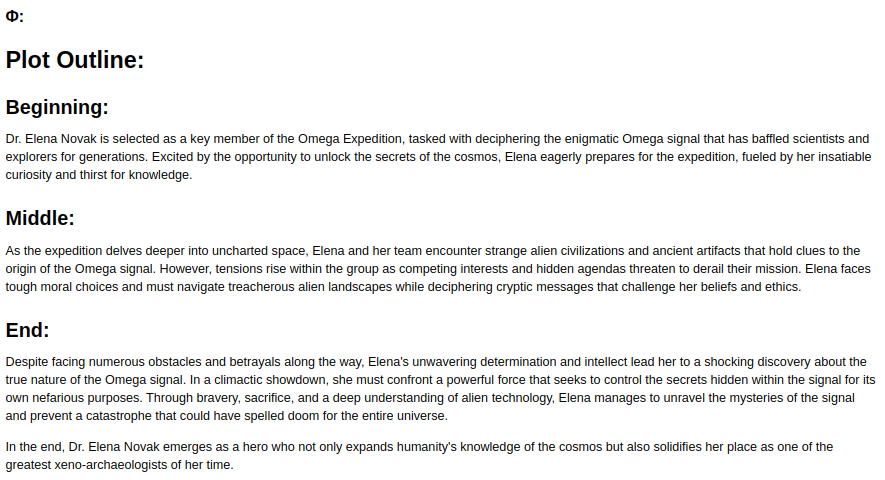
Ψ (Psi)
That is the opening paragraph of the story, setting the tone and introducing Dr. Elena Novak as she embarks on the Omega Expedition.
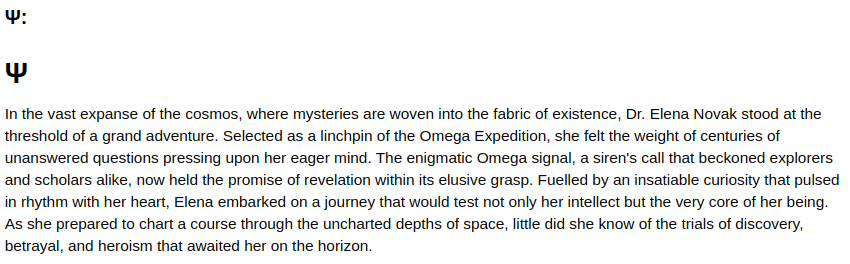
Ultimate Story Components
This half compiles all of the earlier sections into an entire overview of the story, together with the premise, primary character particulars, plot define, and opening paragraph.
For Ultimate Story Components, go to this hyperlink: GitHub Hyperlink.
- We outline a dictionary `story_chain` the place every key’s a logo (Ω, Δ, Φ, Ψ) representing a step within the story creation course of.
- Every image has related directions, context, and an output placeholder.
- The `generate_story_element` perform sends a immediate to the OpenAI API and retrieves the response.
- We iterate by means of every image within the chain, establishing a immediate that features:
- The present image
- The instruction for that step
- The context for that step
- The output from the earlier step (aside from step one)
- After producing content material for every step, we compile the ultimate story utilizing the outputs from every image.
- Structured Considering: By dividing up tough duties into symbolic steps, we assist the AI suppose extra systematically.
- Higher Management: Each image serves as a checkpoint, enabling extra precise management over the AI’s output at each section.
- Context Preservation: The chain ensures that the context from earlier phases is sustained, preserving the coherence of multi-step initiatives.
- Flexibility: The symbolic construction is well expandable or adjusted to assist extra intricate workflows or a wide range of activity sorts.
- Debugging and Iteration: If the end result is just not adequate, it’s easier to find out which step requires modification.
- Nested Chains: Hierarchical activity constructions are attainable, with symbols serving as sub-chain representations.
- Conditional Branches: Based mostly on intermediate outputs, dynamic chains might be created by implementing if-then logic.
- Recursive Chains: For duties requiring iterative refinement, create chains that may name themselves.
- Immediate Size: Lengthy prompts surpassing sure AI fashions’ token restrictions would possibly end result from complicated sequences.
- Deciphering Symbols: To stop confusion, make sure the symbols and their meanings are well-defined.
- Error Propagation: Errors within the chain’s early phases can worsen in subsequent phases.
- Over-structuring: A too tight framework might restrict AI’s capability for creativity or problem-solving.
Clarification of the Code
Right here is the reason of the code:
Related Reads for you:
Article | Supply |
Implementing the Tree of Ideas Technique in AI | Hyperlink |
What are Delimiters in Immediate Engineering? | Hyperlink |
What’s Self-Consistency in Immediate Engineering? | Hyperlink |
What’s Temperature in Immediate Engineering? | Hyperlink |
Chain of Verification: Immediate Engineering for Unparalleled Accuracy | Hyperlink |
Mastering the Chain of Dictionary Approach in Immediate Engineering | Hyperlink |
Test extra articles right here – Immediate Engineering.
Advantages of the Chain of Image Method
Listed here are the advantages of the chain of image strategy:
Superior Functions of Chain of Image Method
The Chain of Image strategy might be prolonged to extra complicated eventualities:
In Multi-Agent Methods, discrete symbols might stand in for numerous AI “consultants,” every with a selected space of experience.
Challenges and Issues of Chain of Image
Whereas highly effective, the Chain of Image strategy does have some challenges:
Conclusion
The Chain of Image strategy in speedy engineering is a potent approach for organizing intricate interactions with AI fashions. By segmenting actions into symbolic phases, actions might be extra successfully guided, context might be maintained throughout phases, and extra managed and coherent outputs might be obtained.
As AI applied sciences advance, strategies such because the chain of symbols will turn into more and more essential in using huge language fashions for complicated, multi-step processes totally. Understanding and implementing this technique will significantly enhance your immediate engineering expertise, whether or not you’re creating AI-powered writing aids, problem-solving programs, or inventive instruments.
Incessantly Requested Questions
Ans. The Chain of Image is a technique of structuring prompts utilizing a sequence of symbolic representations to information AI responses by means of multi-step reasoning and activity completion.
Ans. It divides complicated duties into manageable segments, every denoted by a singular image, offering extra exact management over the AI’s output at every stage.
Ans. The important thing elements are symbols (distinct markers for every step), directions (linked to every image), context (background info), and output placeholders.
Ans. Whereas it may be used with numerous giant language fashions, it’s essential to think about the token limitations of particular fashions when implementing complicated chains.
Ans. Superior purposes embody nested chains for hierarchical duties, conditional branches for dynamic chains, recursive chains for iterative refinement, and multi-agent programs the place symbols signify completely different AI “consultants.”