Introduction
Consider a maze during which you at the moment are alone; your goal is to return out as quicker as attainable however what number of methods are there? Now think about, if you’re given a map the place you’ll be able to spotlight areas that are price pursuing and which of them usually are not! That’s precisely the half heuristic features serve in algorithms of synthetic intelligence. These clever devices help the AI programs to reach at higher and extra immediate selections, thus deeply simplifying the efficiency complexity. On this article, we will focus on the idea of Heuristic perform and its place in AI and the way these make a large distinction within the time taken to resolve issues by how a lot they improve the effectivity – making them indispensable within the shelf of instruments that coming with Synthetic Intelligence.
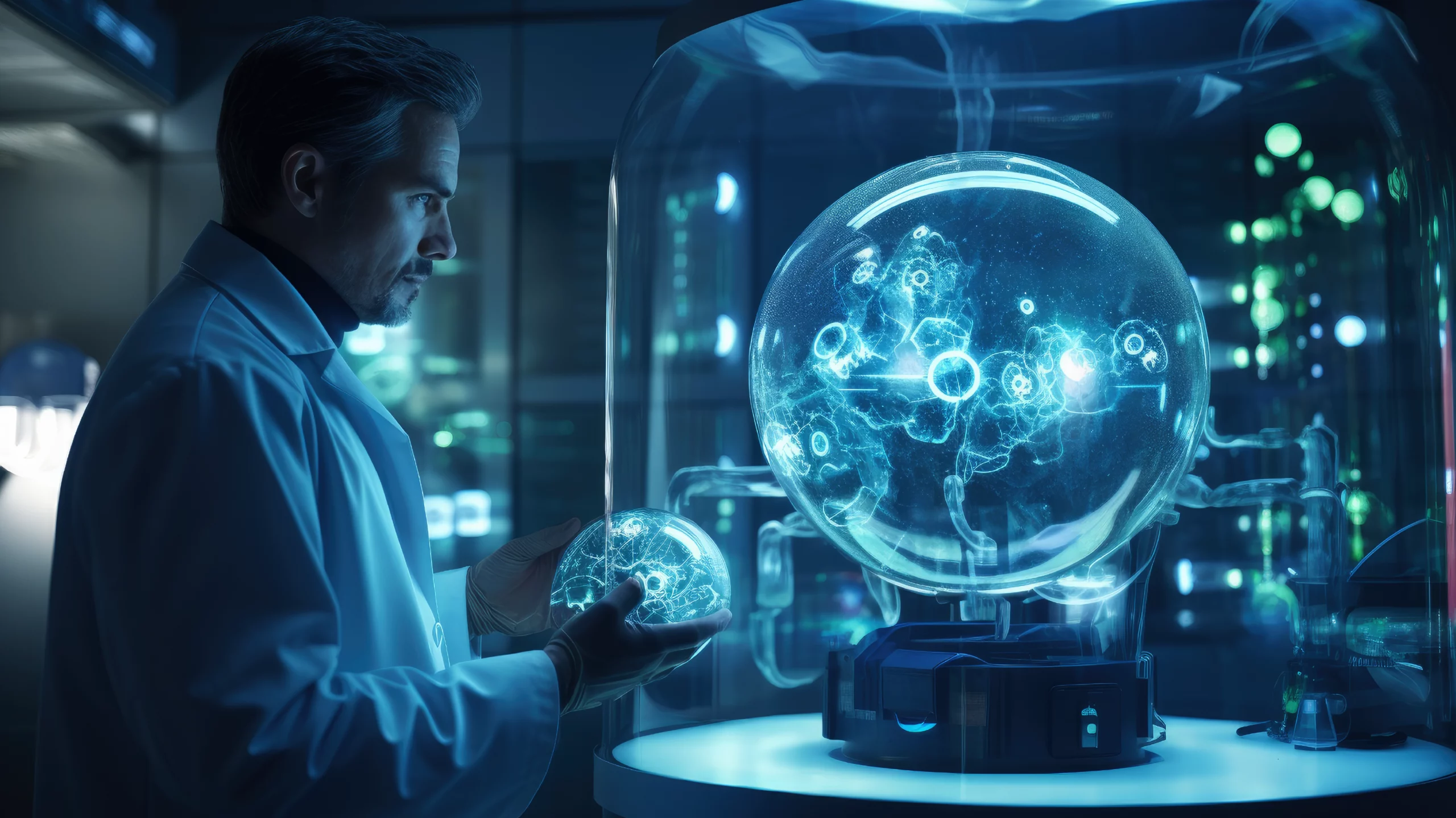
Studying Outcomes
- Comprehend how heuristic features work with AI and its function in search algorithms.
- Learn the way AI downside fixing is improved by way of heuristic features.
- See what forms of heuristic features could be present in AI and the way they’re used.
- Reveal the problems and downsides associated to heuristic features.
- Perceive methods of analysis and optimization of heuristic features in AI.
What’s a Heuristic Perform?
A heuristic perform estimates the fee or distance between a particular state and the purpose in a search technique. It supplies a strategy to choose probably the most promising paths, growing the chance of an efficient answer. In different phrases, a heuristic perform provides the algorithm steering on which course to take, serving to it attain the purpose with fewer steps. By doing so, it minimizes the search house and improves the effectivity of the search course of.
Sorts of Heuristic Capabilities
Heuristic features are available numerous kinds relying on their means to estimate the fee and their influence on algorithm efficiency. Let’s discover these varieties intimately:
Admissible Heuristics
An admissible heuristic is one which by no means overestimates the precise price of reaching the purpose. It all the time supplies a decrease or equal estimate, making certain the algorithm can discover the shortest path. One of these heuristic is essential in algorithms like A*, the place discovering the optimum answer is important.
Instance: Within the A* algorithm, a heuristic that estimates the straight-line distance (Euclidean distance) from one node to a different is admissible, because it doesn’t overestimate the true path.
Inadmissible Heuristics
Exogenous inadmissible heuristics can overestimate the fee wanted to succeed in the purpose. Though they might not all the time present the most effective options, they’re precious when pace is extra essential than high quality.
Instance: There are some circumstances the place an inadmissible heuristic could be helpful by way of the quantity of computation carried out, and acquire a non-optimal answer.
Constant (or Monotonic) Heuristics
A heuristic is admissible if, for each node, the estimated price to the purpose node is not more than the price of shifting from the node to an adjoining node after which to the purpose node from the neighbor. Admissible heuristics embrace constant heuristics and assure that the estimated price decreases because the algorithm progresses in the direction of the purpose.
Instance: In a maze-solving downside, the price of getting from one room to an adjoining room ought to show prohibitively excessive, a minimum of as in contrast with getting from the earlier room after which into the purpose room.
Dominating Heuristics
A dominating heuristic is a stronger heuristic perform that dominates one other if it supplies increased values with out overestimating the fee. The higher the heuristic, the less paths the algorithm must discover.
Instance: In graph traversal, a heuristic estimating each straight-line distance and terrain issue would dominate a heuristic that solely considers straight-line distance.
Path Discovering with Heuristic Capabilities
Heuristic features are fairly important in path discovering algorithms such because the A* (A-star) which finds nice software in GPS navigation, robotics, and gaming amongst others. Now, let’s illustrate using heuristic perform within the A* algorithm for path discovering step-by-step. We’ll describe it with code pattern and additional describe what heuristic features do to make the search higher.
Downside Setup
We’ll encode a grid the place empty areas are represented by 0 and partitions or any type of obstruction is represented by 1. The aim of the duty is to go from the preliminary place (the highest left of the graph) to the ultimate state (the underside proper of the graph) whereas being unable to cross by obstacles. This heuristic perform will probably be used to regulate the trail that the algorithm will select.
Heuristic Perform: Euclidean Distance
On this instance, we use the Euclidean distance as our heuristic. This heuristic estimates the fee from the present node to the purpose node because the straight-line distance, calculated as:

This perform provides the algorithm an estimate of how far a node is from the purpose, serving to it prioritize which node to discover subsequent.
Detailed Walkthrough of the A Algorithm with Heuristic Perform*
Allow us to discover primary steps of A* algorithm heuristic perform.
Step1: Heuristic Perform
The heuristic perform (Euclidean distance) is essential within the A* algorithm. It helps estimate the “distance” from the present node to the purpose. By utilizing the heuristic worth, the algorithm can prioritize nodes which can be extra prone to result in the purpose, lowering the full variety of nodes explored.
Step2: Exploring Neighbors
The A* algorithm explores neighboring nodes by checking every attainable motion course. If a neighbor is throughout the bounds of the grid and isn’t blocked by an impediment, it’s added to the open_list
for additional exploration.
Step3: Prioritizing Nodes
The open_list
is a precedence queue that retains observe of nodes primarily based on their complete estimated price (f = g + h
). This ensures that nodes nearer to the purpose (by way of the heuristic) are explored first.
Step4: Path Reconstruction
As soon as the algorithm reaches the purpose node, it traces the trail again to the beginning node utilizing the came_from
dictionary. This supplies the shortest path, which is then printed.
Grid Illustration and Heuristic Perform
First, we characterize the grid and outline the heuristic perform, which estimates the fee from the present node to the purpose node. We’ll use the Euclidean distance as our heuristic.
import math
# Heuristic perform: Euclidean distance
def heuristic(node, purpose):
return math.sqrt((purpose[0] - node[0])**2 + (purpose[1] - node[1])**2)
# Instance grid (0 = free, 1 = impediment)
grid = [
[0, 0, 0, 1, 0],
[0, 1, 0, 1, 0],
[0, 1, 0, 0, 0],
[0, 0, 1, 1, 0],
[1, 0, 0, 0, 0]
]
begin = (0, 0) # Start line (top-left nook)
purpose = (4, 4) # Purpose level (bottom-right nook)
A Algorithm Setup*
Subsequent, we arrange the A* algorithm by initializing the mandatory variables and buildings:
- Precedence Queue (
open_list
): This shops nodes that should be explored. - Value Monitoring (
cost_so_far
): This dictionary retains observe of the fee from the beginning node to every explored node. - Path Monitoring (
came_from
): This helps reconstruct the trail as soon as we attain the purpose.
import heapq
# A* algorithm implementation
def astar(grid, begin, purpose):
# Instructions for motion (up, down, left, proper, and diagonals)
instructions = [(-1, 0), (1, 0), (0, -1), (0, 1), (-1, -1), (-1, 1), (1, -1), (1, 1)]
# Precedence queue to retailer nodes for exploration
open_list = []
heapq.heappush(open_list, (0 + heuristic(begin, purpose), 0, begin))
# Monitoring the most cost effective price to succeed in every node
came_from = {}
cost_so_far = {begin: 0}
# A* algorithm loop
whereas open_list:
# Get the node with the bottom complete price (g + h)
current_f, current_g, current_node = heapq.heappop(open_list)
# Examine if we have reached the purpose
if current_node == purpose:
return reconstruct_path(came_from, begin, purpose)
# Discover neighbors
for course in instructions:
neighbor = (current_node[0] + course[0], current_node[1] + course[1])
# Examine if the neighbor is inside bounds and never an impediment
if 0 <= neighbor[0] < len(grid) and 0 <= neighbor[1] < len(grid[0]) and grid[neighbor[0]][neighbor[1]] == 0:
new_cost = cost_so_far[current_node] + 1 # Assume motion price is 1
# If the neighbor is unvisited or a less expensive path is discovered
if neighbor not in cost_so_far or new_cost < cost_so_far[neighbor]:
cost_so_far[neighbor] = new_cost
f_score = new_cost + heuristic(neighbor, purpose)
heapq.heappush(open_list, (f_score, new_cost, neighbor))
came_from[neighbor] = current_node
return [] # Return an empty checklist if no path is discovered
Reconstructing the Path
As soon as we attain the purpose, we have to reconstruct the trail from the begin to the purpose utilizing the came_from
dictionary. This dictionary tracks the place we got here from for every node.
# Perform to reconstruct the trail from begin to purpose
def reconstruct_path(came_from, begin, purpose):
present = purpose
path = [current]
whereas present != begin:
present = came_from[current]
path.append(present)
path.reverse()
return path
Operating the A* Algorithm
Lastly, we execute the A* algorithm utilizing the astar()
perform and print the trail discovered.
# Operating the A* algorithm to search out the trail
path = astar(grid, begin, purpose)
if path:
print("Path discovered:", path)
else:
print("No path discovered.")
Instance Output:
Path discovered: [(0, 0), (1, 0), (2, 0), (3, 1), (4, 2), (4, 3), (4, 4)]
Position of Heuristic Capabilities in AI
Heuristic features play a essential function in AI, particularly in search algorithms the place they information the decision-making course of. Right here’s a breakdown of their key roles:
Guiding Search Algorithms
Heuristic features act as a compass for search algorithms by estimating the fee from the present state to the purpose. This estimation helps algorithms give attention to extra promising paths, lowering the effort and time required to discover much less fruitful choices. In algorithms like A*, the heuristic perform considerably accelerates the search by prioritizing nodes which can be prone to result in an answer.
Lowering Computational Complexity
Heuristic features are essential as failure might happen during which a number of choices exist and the quantity will increase exponentially with the growth of the issue. Heuristics cut back this downside by sampling solely probably the most believable paths of search house since arbitrary paths result in arbitrary options. This discount in complexity is essential particularly within the functions requiring actual time options corresponding to robotics.
Enhancing Downside-Fixing Effectivity
Heuristic features assist search algorithms by offering particular details about the issue. This permits the algorithm to make educated guesses slightly than making an attempt all attainable options. Because of this, it results in extra environment friendly problem-solving in real-world conditions. Heuristics are particularly essential in large-scale AI challenges like video games, navigation, and optimization. They play a significant function in tackling advanced issues extra successfully.
Balancing Accuracy and Pace
Typically, heuristic features are designed to be much less correct however quicker than different algorithms. Whereas admissible heuristics assure the identification of the shortest path, inadmissible heuristics present near-optimal options extra rapidly. Certainly, this stability of optimization almost about pace is especially related in conditions during which it’s important to discover a answer quick slightly than to give attention to reaching an optimum answer.
Adapting to Area-Particular Challenges
Heuristic features are normally outlined primarily based on the precise downside area. They depend on data in regards to the issues and targets of the duty. Due to this, they’re helpful in lots of AI functions. They assist with route planning in design AIs and assessing choices in sport AIs.
Significance of Heuristic Capabilities in AI
Heuristic features are important to AI, particularly when fixing issues that contain giant search areas. With out heuristics, search algorithms must discover each attainable answer, inflicting an exponential improve in time and computational assets. Right here’s why they’re essential:
- Effectivity: Heuristic features dramatically cut back the variety of paths an algorithm wants to guage. By guiding the algorithm towards probably the most promising routes, they minimize down each time and house complexity, permitting AI programs to search out options quicker.
- Scalability: In case of precise functions like route discovering, video games and optimization, the scale of the search area could be huge. Approximations help within the scaling of the algorithms in the direction of different bigger issues for they solely discover paths that can probably present options slightly than exploring the entire search house.
- Downside-Particular Perception: Heuristic features leverage data of the issue area to turn out to be extremely efficient for particular points. For instance, in sport AI, builders create heuristics to guage strikes primarily based on sport guidelines, thereby enhancing decision-making throughout gameplay.
Purposes of Heuristic Capabilities
Allow us to discover functions of Heuristic Capabilities under:
- Pathfinding Algorithms: Heuristic features are extensively utilized in pathfinding algorithms like A* and Dijkstra’s algorithm. In these algorithms, heuristics assist estimate the shortest path between two factors in a graph, making them important in functions like GPS navigation programs.
- Sport AI: In video games like chess, heuristic features consider the potential outcomes of various strikes, serving to the AI select probably the most strategic choices. These features are essential in situations the place calculating all attainable strikes is computationally infeasible.
- Optimization Issues: Heuristics are employed in optimization issues, such because the touring salesman downside, to search out near-optimal options inside an affordable timeframe. Whereas these features might not assure the optimum answer, they typically present options which can be shut sufficient for sensible functions.
- Constraint Satisfaction Issues: In issues like scheduling and useful resource allocation, heuristic features information the seek for options that fulfill all constraints, enhancing the effectivity of the search course of.
Challenges and Limitations
Regardless of their effectiveness, heuristic features include challenges that restrict their software:
Design Complexity
One of many greatest challenges is designing an efficient heuristic. A heuristic should precisely estimate the fee to the purpose with out being too conservative or too aggressive. A poorly designed heuristic can result in inefficient searches or suboptimal options.
Downside-Particular Nature
Heuristic features are sometimes tailor-made to particular issues, which limits their generalization. A heuristic that works effectively for a selected situation might not be relevant in a unique context, requiring the design of recent heuristics for every downside.
Computational Overhead
Whereas heuristics cut back search house, calculating a fancy heuristic at every step can introduce computational overhead. If the price of computing the heuristic outweighs its advantages, it might not enhance general efficiency.
Danger of Suboptimal Options
Inadmissible heuristics, whereas quicker, threat resulting in suboptimal options. AI functions that require precision should fastidiously think about the trade-off between pace and accuracy.
Conclusion
Heuristic features are essential in AI. They type the spine of many search algorithms and problem-solving methods. By providing knowledgeable estimates, they assist algorithms navigate advanced search areas effectively. This makes AI programs more practical and sensible in real-world functions. Nonetheless, designing and optimizing heuristic features require cautious thought. Their effectiveness can significantly influence the efficiency of AI algorithms.
Continuously Requested Questions
A. In AI, a heuristic perform estimates the fee or distance from a present state to a purpose state, guiding search algorithms of their decision-making.
A. Heuristic features are essential as a result of they assist AI algorithms effectively navigate advanced search areas by prioritizing probably the most promising paths.
A. Admissible heuristics are features that by no means overestimate the fee to succeed in the purpose, making certain that the algorithm finds the shortest path.
A. Not all the time. Whereas admissible heuristics assure optimum options, inadmissible heuristics might result in suboptimal options however can supply quicker leads to sure situations.
A. Folks generally use heuristic features in pathfinding, sport AI, optimization issues, and constraint satisfaction issues.